Basics of Python Programming language.

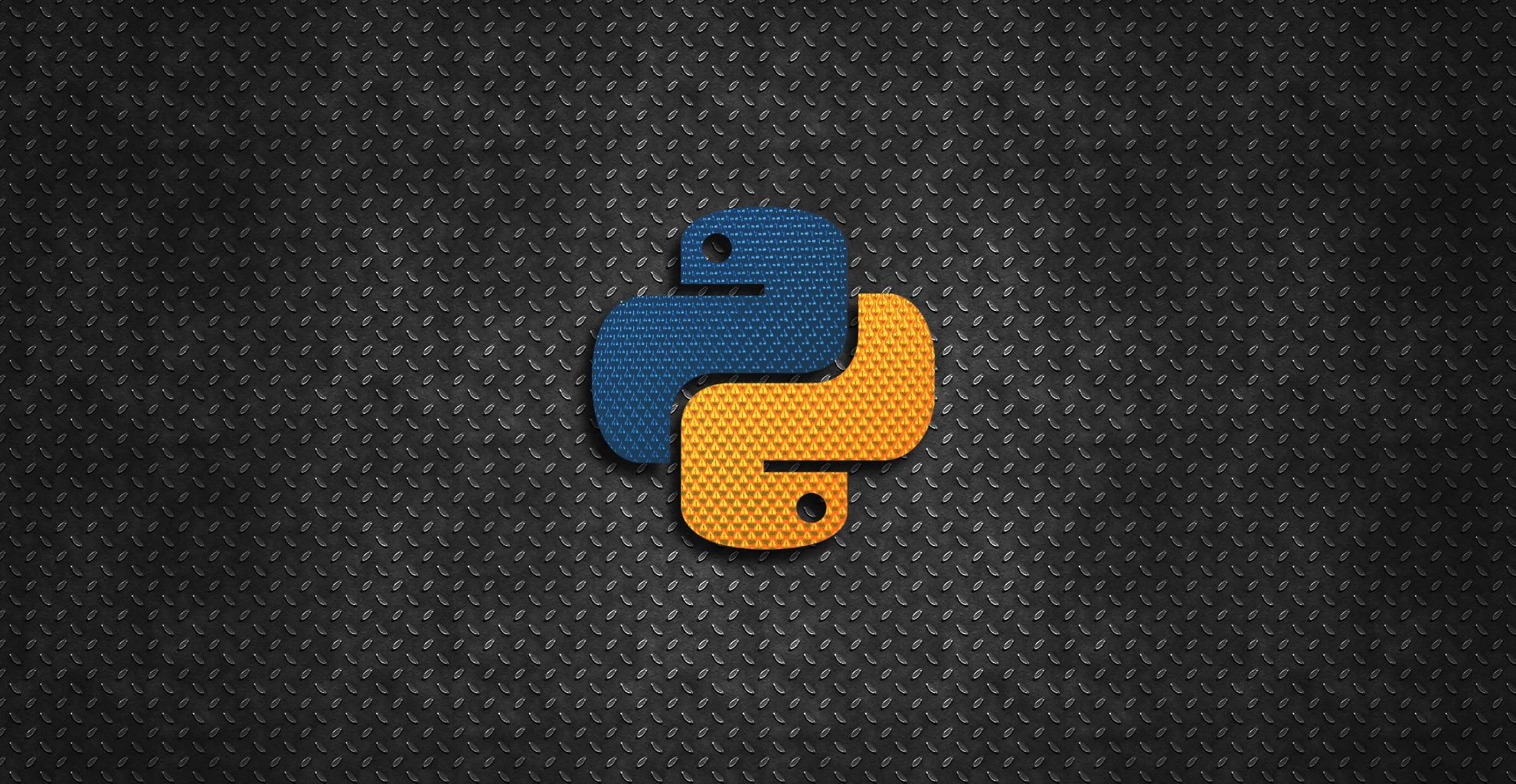
What is Python?
Python is an Open source, general-purpose, high-level, and object-oriented programming language.
It was created by Guido van Rossum
Python consists of vast libraries and various frameworks like Django, Tensorflow, Flask, Pandas, Keras, etc.
Python is one of the most popular general-purpose programming languages in modern times.
The term “general-purpose” simply means that Python can be used for a variety of applications and does not focus on any one aspect of programming.
Python falls under the category of high-level, interpreted languages. A high-level language, which cannot be understood directly by our machine.
Machines are generally designed to read machine code, but high-level syntax cannot be directly converted to machine code.
Python code must first be converted to bytecode which is then converted to machine code before the program can be executed.
Python is an interpreted language because, during execution, each line is interpreted to the machine language on the go.
Python’s rapid growth is the simplicity of its syntax, python is a very efficient language that is used in almost every sphere of modern computing.
Python 2.7 was widely used for a very long time, even after the release of newer versions.
Python 2.7 has been deprecated as of January 2020, and replaced completely by
3.xx
versions, known as Python 3. The differences between Python 2.7 and Python 3 are minute, but important nonetheless.Since Python is one of the most readable languages out there, we can print data on the terminal by simply using the
print
statement. We can even print multiple things in a singleprint
command; we just have to separate them using commas, like print(a,b,c).
How to Install Python:
You can install Python in your System whether it is Windows, MacOS, ubuntu, centos, etc. Below are the links for the installation:
Windows Installation - https://www.python.org/downloads/
Ubuntu: apt-get install python3.6
Data types and Variables:
The data type of an item defines the type and range of values that an item can have.
The concept of data types can be found in the real world. There are numbers, alphabets, characters, etc., that all have unique properties due to their classification.
Variables: A variable is simply a name to which a value can be assigned. Variables allow us to give meaningful names to data. The simplest way to assign a value to a variable is through the =
operator. A big advantage of variables is that they allow us to store data so that we can use it later to perform operations in the code. Variables are mutable. Hence, the value of a variable can always be updated or replaced.
Naming convention:
There are certain rules we have to follow when picking the name for a variable:
The name can start with an upper or lowercase alphabet, for example, you can define your income variable as
Income
orincome
, both are valid.All the names are case-sensitive, for example,
Income
andincome
are two different variables and not one.A number can appear in the name, but not at the beginning, for example,
12income
is not a valid name butincome12
orin12come
are valid.The
_
character can appear anywhere in the name, for example,_income
orincome_
are valid names.Spaces are not allowed. Instead, we must use snake_case to make variable names readable, For example,
monthly_income
is a valid name.The name of the variable should be something meaningful that describes the value it holds, instead of being random characters.
TASK 1:
- Install Python in your respective OS, and check the version.
Data types in Python: There are different types of data types in Python. Some built-in Python data types are:
Numeric data type:
Python is one of the most powerful languages when it comes to manipulating numerical data, It is equipped with support for several types of numbers, along with utilities for performing computations on them, There are three main types of numbers in Python:
a. Integers: The integer data type is comprised of all the positive and negative whole numbers, the amount of memory an integer occupies depends on its value. ex: 0= 24Bytes and 1= 28Bytes etc.
b. Floating point: Floating-point numbers, or floats, refer to positive and negative decimal numbers, python allows us to create decimals up to a very high decimal place, which ensures accurate computations for precise values, float occupies 24 bytes of memory.
c. Complex numbers: Python also supports complex numbers, or numbers made up of a real and an imaginary part, just like the
print()
statement is used to print values,complex()
is used to create complex numbers, It requires two values. The first one will be the real part of the complex number, while the second value will be the imaginary part.Syntax: complex(real, imaginary).
Complex numbers are useful for modeling physics and electrical engineering models in Python. While they may not seem very relevant right now, a complex number usually takes up 32 bytes of memory.
Boolean Data type: The Boolean (also known as bool) data type allows us to choose between two values: true and false, the first letter of a bool needs to be capitalized in Python, and a Boolean is used to determine whether the logic of an expression or a comparison is correct. It plays a huge role in data comparisons.
String data type: A string is a sequence of characters represented by single(') or double quotes ("), A string can also contain a single character or be entirely empty, a blank space inside the string quotation marks is also considered to be a character, to add a multi-line string we can use triple quotes, the length of a string can be found using the
len()
built-in function. This length indicates the number of characters in the string.In a string, every character is given a numerical index based on its position., a string in Python is indexed from
0
ton-1
wheren
is its length. This means that the index of the first character in a string is0
. Each character in a string can be accessed using its index. The index must be closed within square brackets,[]
, and appended to the string.List data type: A list is a collection of similar or different types of Items separated by commas and enclosed with bracket []
a. List Integer:
b. List string:
c. List of Integers and String:
Tuples: It's an ordered sequence of items same as a list. the difference is it is immutable. Data in a tuple is written using parenthesis ( ) and commas.
Thank you for reading this blog....!!
Happy Learning :)
Subscribe to my newsletter
Read articles from RAKESH REVASHETTI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

RAKESH REVASHETTI
RAKESH REVASHETTI
I'm Rakesh, a DevOps engineer who enjoys automation, continuous integration and deployment. With good hands-on experience in DevOps & cloud technology, i'm proficient in various tools and technologies related to infrastructure automation, containerization, cloud platform, monitoring and CI/CD.