Understanding Basic Data Types in Go

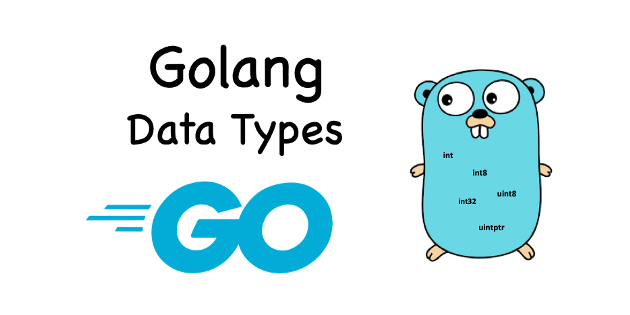
Go, also known as Golang, is a statically typed programming language that emphasizes simplicity and efficiency. At the foundation of this language are basic data types that form the building blocks of Go programs. Let's dive into these fundamental types and understand how they work.
Introduction to Data Types
In Go, data types specify the kind of data that any variable can hold. When you declare a variable in Go, you not only give it a name but also define what type of data it is going to store. This helps the compiler understand how to handle variables in your program.
The Basic Data Types
Go has several basic data types which include:
1. Booleans
The boolean data type is represented by the keyword bool
. It can hold one of two values: true
or false
.
Example:
var isActive bool = true
2. Numeric Types
Go has a rich numeric system that includes:
Integers: Signed (
int8
,int16
,int32
,int64
,int
) and unsigned (uint8
,uint16
,uint32
,uint64
,uint
) integers.Floating-Point Numbers:
float32
andfloat64
.Complex Numbers:
complex64
andcomplex128
for numbers with real and imaginary parts.
Example:
var age int = 30
var price float32 = 99.99
3. Strings
A string in Go is a sequence of bytes represented by string
. It's immutable, meaning once created, it cannot be changed.
Example:
var name string = "Jane Doe"
4. Bytes and Runes
Bytes: An alias for
uint8
, used to handle raw data.Runes: Represents a Unicode code point and is an alias for
int32
.
Example:
var letter rune = 'A'
Type Conversion
In Go, you must explicitly convert types when necessary, as it does not support automatic type conversion (coercion).
Example:
var integer int = 42
var floatNumber float64 = float64(integer)
Conclusion
Understanding the basic data types in Go is crucial for writing effective and efficient programs. These types are the building blocks that will allow you to define the behavior and functionality of your Go applications.
With this foundation, you're now ready to start experimenting with Go's data types in your programs. Remember, practice is key to mastering Go's type system!
Subscribe to my newsletter
Read articles from Arslan Ametov directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arslan Ametov
Arslan Ametov
Coder, creator, community mentor. I simplify software engineering into insightful articles and engaging videos. Let's decode tech together!