Client-Side Routing: What You Need to Know to Work with React Router

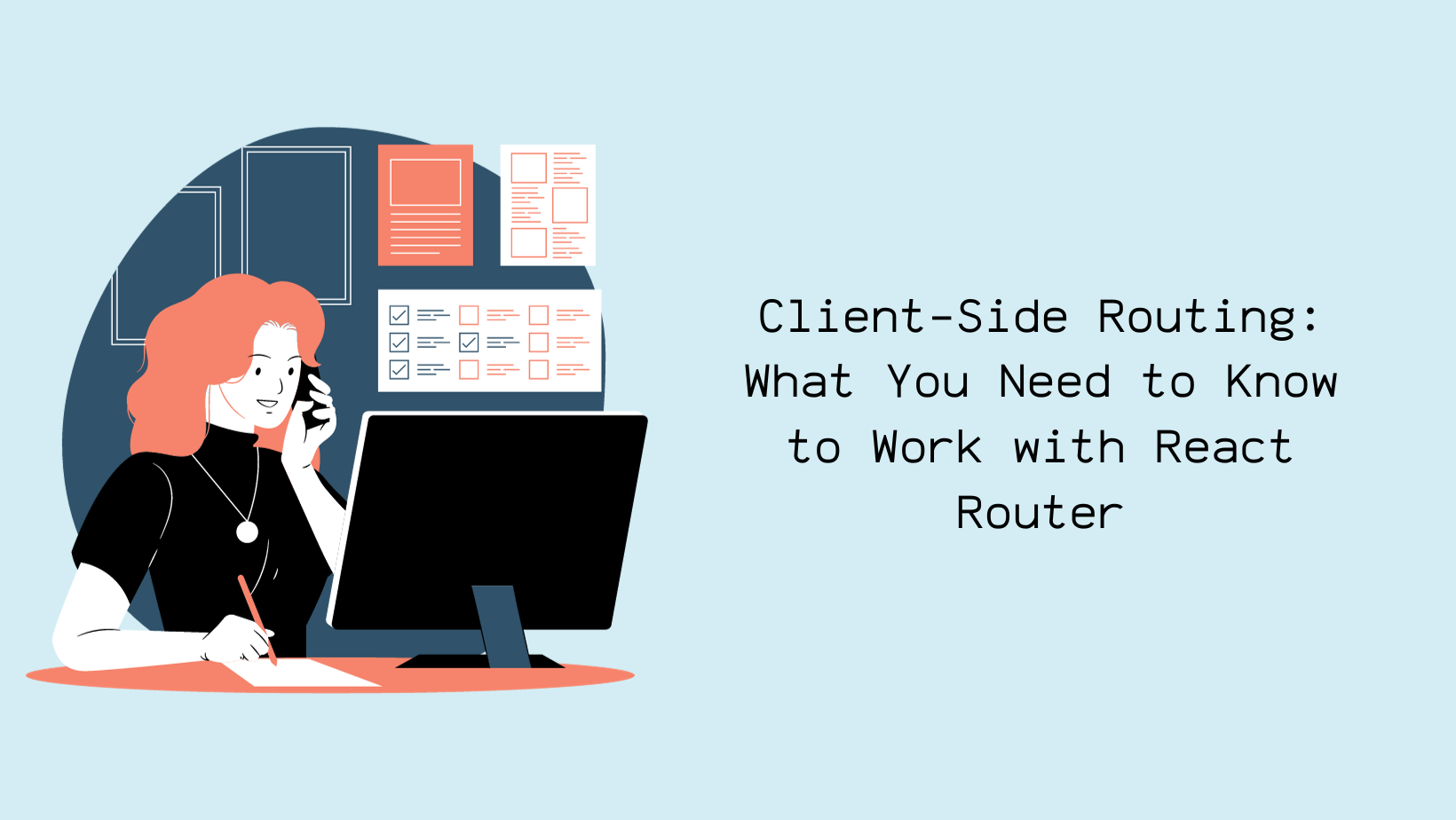
Whether you're building a single-page app or a complex web platform, effective navigation is essential. As a competent and modern developer, you should always ensure your website is user-friendly. So, how can we separate our components into different "pages", each with its unique URL, if we have a single-page application? This is where client-side routing comes in; React Router is the library that makes this possible. In this post, we'll break client-side routing down and see what you need to know to use React Router more efficiently.
Basics of Client-Side Routing
Traditionally, when a user clicks on a link or enters a URL, the browser sends a request to the server. In response, the server will provide a new HTML page. This traditional method is called server-side routing.
Client-side routing, on the other hand, is designed to handle routing logic only through JavaScript, eliminating the need for additional GET requests to fetch new HTML documents.
Client-side routing offers many benefits:
It improves the user experience by quickly updating content without reloading the entire page, making navigation faster and easier.
Users can easily bookmark or share country-specific URLs, which saves context and promotes efficient navigation.
Client interactions are faster, reducing server load and ultimately improving overall performance.
However, this has its drawbacks:
Client-side routing can impact the loading time on the first page as all required items must be loaded first.
SEO can be complicated by client-side routing because search engines can need help crawling and indexing JavaScript-heavy content, which can affect your site's search engine rankings.
Client-side routing relies heavily on JavaScript, making your site dependent.
React Router
React Router is a popular library for handling client-side routing in React applications. In the dynamic content panel, you can define routers and their corresponding components, making it easy to navigate single-page applications.
Setting Up React Router
Before diving into the specifics of React Router, you'll need to install it in your project. You can do this by running the following command:
$ npm install react-router-dom@6
This command installs the React Router library, designed for web applications that use the DOM.
Importing vital components
To start implementing routes, we first need to import createBrowserRouter and RouterProvider from react-router-dom:
import { createBrowserRouter, RouterProvider } from "react-router-dom";
createBrowserRouter is used to create the router for our application.
const router = createBrowserRouter([
{
path: "/",
element: <Home />
}
]);
The RouterProvider provides the router designed by createBrowserRouter to our application to use React-Router's client-side routing.
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(<RouterProvider router={router} />);
Navigating Between Routes
Once you've defined your routes, you'll want to create links to navigate between them. React Router provides the Link and NavLink components, making it easy to create navigation elements.
Link is a fundamental component used to create links for navigation between different paths. It provides an anchor element (<a>) that, when clicked, changes the URL and refreshes the view without reloading the entire page.
import { Link } from 'react-router-dom';
// Usage
<Link to="/about">About</Link>
NavLink is an advanced version of Link that adds formatting and functionality to existing URLs. It allows you to use CSS classes or dynamic link styles, making it ideal for navigation menus.
import { NavLink } from 'react-router-dom';
// Usage with custom styling for the active link
<NavLink to="/home" activeClassName="home">Home</NavLink>
The main difference is that NavLink provides additional functionality to manage "active" links using classes (or other styles). This is useful for improving the navigation menu options by displaying the current page in the navigation menu.
Dynamic routes and URL parameters
Dynamic routes and URL parameters allow you to create routes that match different URL patterns and pass dynamic values as parameters to components.
Imagine the situation: in an e-commerce website, you want to implement product pages. Each product has a unique ID. Using dynamic routes and URL parameters like /products/:id, users can view detailed information about a specific product by clicking on its card in the product catalog.
Dynamic routes are defined with placeholders in the route path, denoted by :
. These placeholders can match any value in the URL segment.
const router = createBrowserRouter([{
path: "/product/:id",
element: <ProductProfile />
}]);
URL parameters are values passed within the URL, usually associated with dynamic routes. They can be accessed using the useParams hook.
import { useParams } from "react-router-dom";
function UserProfile() {
const params = useParams();
console.log(params);
}
Nested Routes
React Router supports nested routes, allowing you to create more complex application structures. Nested routes are routes within routes. They help create layouts that have consistent elements across multiple pages.
Conclusion
The React Router revolutionized modern web development by providing the ability to create dynamic user interfaces and optimize navigation in single-page applications. This is achieved by managing routing via JavaScript, eliminating the need for additional requests to the server. There are many benefits, including faster content updates, shared URLs, and a better user experience.
Subscribe to my newsletter
Read articles from Khrystyna Klapushchak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
