Unveiling the Magic of JavaScript Array Methods: A Journey Through Elegance and Efficiency

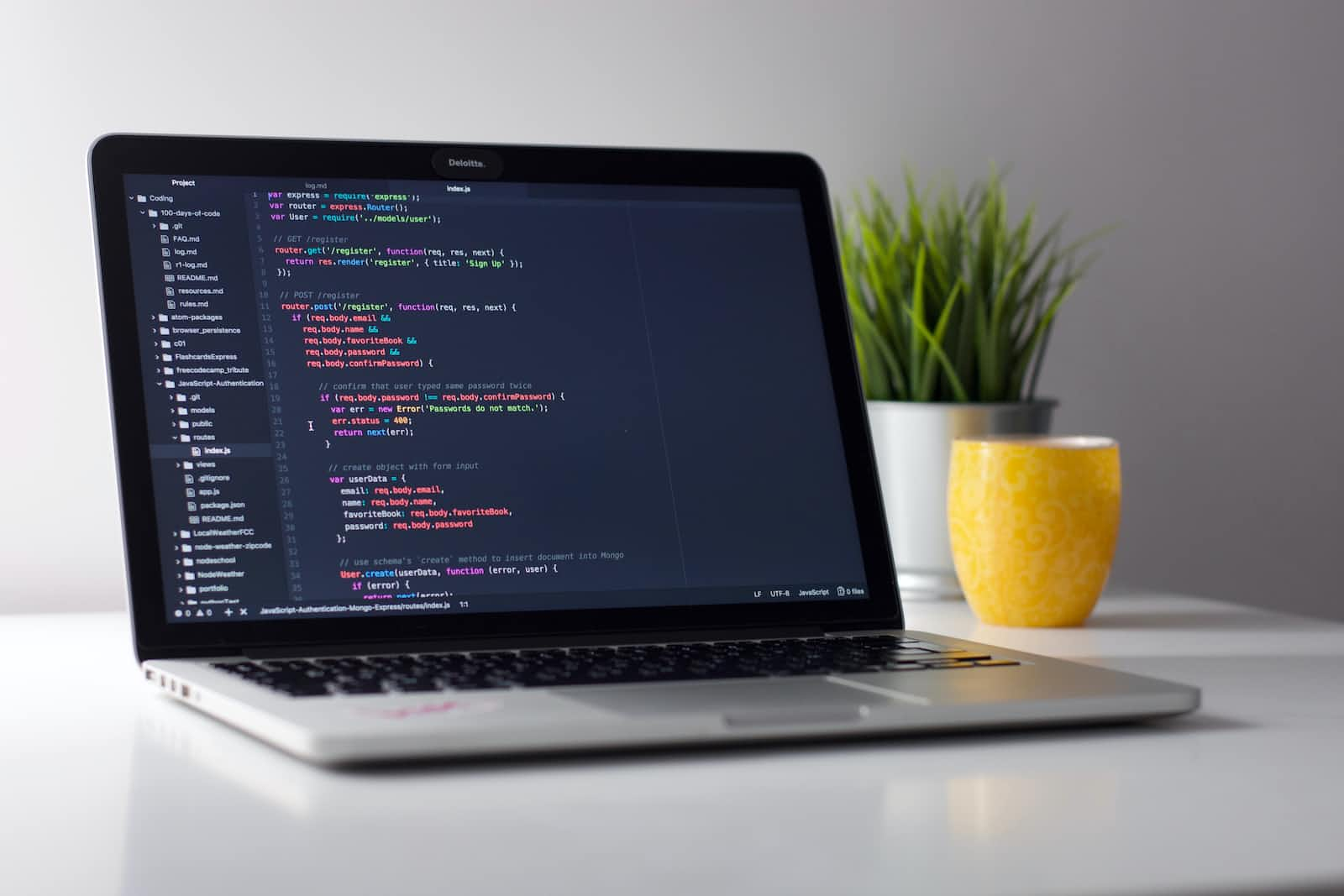
Introduction:
In the enchanting realm of JavaScript, arrays play a pivotal role, serving as the backbone of countless operations. However, it’s the array of methods that truly elevate the language’s allure, weaving a tapestry of elegance and efficiency. Join me on this exhilarating expedition as we unravel the enchanting array methods that adorn the language, transforming mundane code into a symphony of functionality and grace.
Array.map(): Unleashing the Power of Transformation
Let’s begin our odyssey with the illustrious “map” method, a maestro of transformation. Imagine an artist meticulously reshaping a canvas, stroke by stroke, to create a masterpiece. Similarly, the “map” method elegantly transmutes each element of an array, molding it into a new form, breathing life into your code.
// Example: Converting Fahrenheit temperatures to Celsius
const fahrenheitTemperatures = [32, 45, 68, 72, 87];
const celsiusTemperatures = fahrenheitTemperatures.map((temp) => ((temp - 32) * 5) / 9);
// Output: [0, 7.2222, 20, 22.2222, 30.5556]
Array.filter(): The Art of Selective Elegance
Step into the realm of “filter,” where elements harmoniously align themselves based on specific criteria, much like a skilled curator meticulously selecting artworks for an exhibition. With grace and precision, the “filter” method skims through arrays, handpicking elements that satisfy the condition, creating a refined and purposeful collection.
// Example: Filtering out even numbers from an array
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
// Output: [2, 4, 6, 8, 10]
Array.reduce(): The Symphony of Aggregation
Behold the enchanting “reduce” method, akin to an expert conductor skillfully orchestrating various musical elements into a harmonious composition. With finesse and precision, “reduce” amalgamates array elements, meticulously chiseling a singular value that encapsulates the essence of the entire array, transcending mere data to create a masterpiece of computation.
// Example: Summing all elements of an array
const numbersToSum = [1, 2, 3, 4, 5];
const sum = numbersToSum.reduce((total, num) => total + num, 0);
// Output: 15
Array.forEach(): Embracing the Rhythms of Iteration
Envision a seasoned storyteller narrating each chapter of a riveting tale with passion and fervor. Much like this, the “forEach” method embraces each element of an array, invoking a sequence of actions, infusing your code with a narrative that unfolds with each iteration.
// Example: Displaying each element of an array
const fruits = ['apple', 'banana', 'cherry', 'date'];
fruits.forEach((fruit) => {
console.log(`I love ${fruit}s!`);
});
// Output: I love apples! I love bananas! I love cherries! I love dates!
Array.find(): Discovering the Gems Within
Imagine embarking on a treasure hunt, where each step unearths hidden gems that shimmer with brilliance. The “find” method serves as your guide, leading you to the first element in the array that satisfies your quest, revealing a jewel amidst an array of possibilities.
// Example: Finding the first even number in an array
const numbersToSearch = [3, 5, 7, 8, 9, 10];
const firstEvenNumber = numbersToSearch.find((num) => num % 2 === 0);
// Output: 8
Array.some() and Array.every(): The Gates of Validation
Imagine a pair of sentinels guarding the gates of a majestic citadel, one allowing passage to those deemed worthy, the other denying entry to those who fall short. The “some” and “every” methods serve as these guardians, validating elements within arrays based on specific criteria, determining whether any or all meet the required conditions.
// Example: Validating if any element in the array is greater than 10
const numbersToCheckSome = [5, 8, 12, 3, 9];
const anyGreaterThanTen = numbersToCheckSome.some((num) => num > 10);
// Output: true// Example: Validating if all elements in the array are greater than 5
const numbersToCheckEvery = [6, 8, 12, 7, 9];
const allGreaterThanFive = numbersToCheckEvery.every((num) => num > 5);// Output: true
Array.includes(): Embracing the Quest for Presence
Envision a seasoned explorer navigating through uncharted territories, searching for traces of a hidden relic. The “includes” method serves as the compass, guiding your path as you seek the presence of a specific element within the array, unraveling the mysteries of its existence.
// Example: Checking if an array contains a specific element
const numbersToCheck = [1, 3, 5, 7, 9];
const includesSeven = numbersToCheck.includes(7);
// Output: true
Embrace the elegance and versatility of these array methods, weaving a tapestry of functionality and finesse within your JavaScript code. With each method adding its own unique flair, your programming journey will ascend to new heights, enriched by the profound beauty and efficiency of these exquisite constructs.
Array.slice(): Crafting the Essence of Subsets
Imagine a skilled artisan delicately carving out a distinct section from a larger masterpiece, creating a standalone work that encapsulates the essence of the whole. Much like this, the “slice” method carves out a portion of an array, crafting a new array that captures the spirit of the original, allowing you to work with a subset of elements with ease.
// Example: Extracting a subset of elements from an array
const originalArray = [1, 2, 3, 4, 5];
const slicedArray = originalArray.slice(1, 3);
// Output: [2, 3]
Array.splice(): The Art of Surgical Extraction and Insertion
Picture a master surgeon delicately removing a specific section of tissue and seamlessly integrating a new component, enhancing the vitality of the whole. In a similar vein, the “splice” method surgically excises elements from an array while seamlessly integrating new elements, transforming the array with surgical precision.
// Example: Removing and inserting elements within an array
const fruitsArray = ['apple', 'banana', 'cherry', 'date'];
fruitsArray.splice(2, 1, 'grape', 'kiwi');
// Output: ['apple', 'banana', 'grape', 'kiwi', 'date']
Array.concat(): The Symphony of Unification
Envision a symphony conductor skillfully merging the harmonious notes of multiple instruments into a grand orchestral masterpiece. In a similar fashion, the “concat” method seamlessly unites multiple arrays, weaving them together into a single harmonious composition, creating a unified array that resonates with cohesion.
// Example: Concatenating multiple arrays into one
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = array1.concat(array2);
// Output: [1, 2, 3, 4, 5, 6]
Array.reverse(): Embracing the Art of Mirroring
Imagine standing before a mirror that reflects your image in perfect reverse, offering a unique perspective on your essence. The “reverse” method bestows this mirror-like quality upon arrays, gracefully reversing the order of elements, granting you a new viewpoint on the structure of your data.
// Example: Reversing the order of elements in an array
const originalOrder = [1, 2, 3, 4, 5];
const reversedOrder = originalOrder.reverse();
// Output: [5, 4, 3, 2, 1]
Array.join(): The Weaving of Strings into Tapestries
Envision a skilled weaver intertwining a myriad of colorful threads into a breathtaking tapestry, each string adding its unique hue to the fabric of the whole. In a similar manner, the “join” method weaves the elements of an array into a cohesive string, creating a seamless narrative that unites individual elements into a unified whole.
// Example: Joining elements of an array into a string
const elementsToJoin = ['JavaScript', 'is', 'amazing'];
const joinedString = elementsToJoin.join(' ');
// Output: 'JavaScript is amazing'
Array.sort(): Orchestrating Elements into Harmony
Imagine a skilled conductor bringing together a dissonant orchestra, guiding each musician to play in perfect unison, transforming chaos into a harmonious melody. Much like this, the “sort” method orchestrates the elements of an array, arranging them in ascending or descending order, creating a symphony of organized data.
// Example: Sorting elements in an array
const unsortedArray = [3, 1, 4, 1, 5, 9, 2, 6, 5];
const sortedArray = unsortedArray.sort();
// Output: [1, 1, 2, 3, 4, 5, 5, 6, 9]
Array.indexOf() and Array.lastIndexOf(): Navigating the Array’s Coordinates
Envision a seasoned cartographer plotting a course across uncharted territories, meticulously marking key locations on the map. The “indexOf” and “lastIndexOf” methods serve as your navigational tools, revealing the precise coordinates of specific elements within the array, guiding you to their exact positions.
// Example: Finding the index of a specific element in an array
const fruitsList = ['apple', 'banana', 'cherry', 'date', 'apple'];
const firstAppleIndex = fruitsList.indexOf('apple');
const lastAppleIndex = fruitsList.lastIndexOf('apple');
// Output: firstAppleIndex = 0, lastAppleIndex = 4
Array.every(): Embracing the Quest for Truth
Envision a relentless truth-seeker navigating through a labyrinth of assertions, diligently examining each claim for authenticity. The “includes” method serves as your guide, unveiling the truth behind the presence of a specific element within the array, bringing clarity to your quest for veracity.
// Example: Checking if all elements in an array meet a certain condition
const numbersToCheckEvery = [6, 8, 12, 7, 9];
const allGreaterThanFive = numbersToCheckEvery.every((num) => num > 5);
// Output: true
Array.from(): The Art of Transformation
Imagine a master shapeshifter effortlessly assuming various forms, adapting to the demands of different situations with fluid grace. Similarly, the “from” method metamorphoses non-array objects or iterables into arrays, allowing you to seamlessly navigate between different data structures with grace and ease.
// Example: Creating an array from a string
const stringToConvert = 'JavaScript';
const arrayFromString = Array.from(stringToConvert);
// Output: ['J', 'a', 'v', 'a', 'S', 'c', 'r', 'i', 'p', 't']
Subscribe to my newsletter
Read articles from ROSHAN CSE0026 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ROSHAN CSE0026
ROSHAN CSE0026
Result Driven Engineer