Mastering Microservices: A Deep Dive into Spring Cloud Netflix Eureka for Seamless Service Registration and Discovery
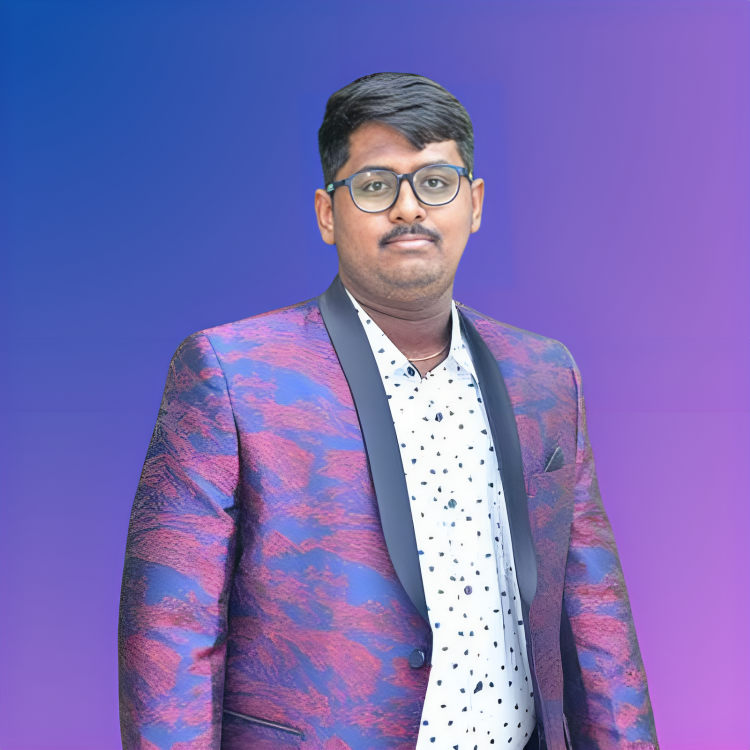
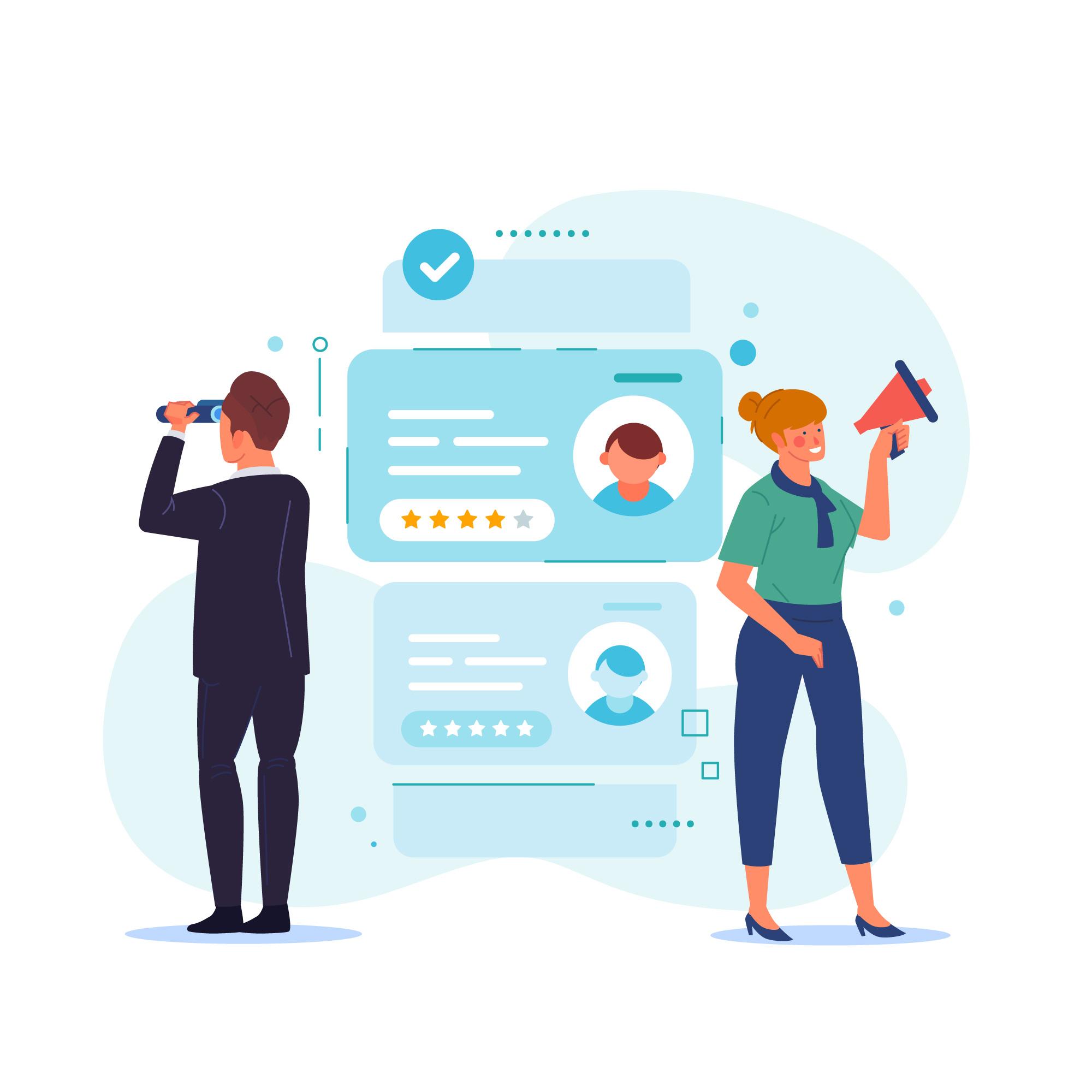
Welcome, everyone! π We're diving into microservices with Spring Boot. Join us as we explore Service Registry and Service Discovery using Spring Cloud Netflix Eureka, showing you how to set up a service registry server for multiple service clients. Ready to begin this microservices journey? Let's go! π
What is a Service Registry?
Service Registry is like a digital directory for microservices in a system. It acts as a central hub where services register themselves and can discover other services, fostering efficient communication and collaboration. This dynamic approach enables seamless interactions between microservices, allowing them to adapt and connect dynamically as the system evolves. In essence, it's a crucial tool in the world of microservices architecture, ensuring that services can find and communicate with each other effortlessly. πβοΈ
Client-Side Service Registry:
Empower your services to take charge! With the client-side approach, each service proudly registers itself, maintaining autonomy and control.
Periodic heartbeats ensure a vibrant and active ecosystem.
Experience the efficiency and simplicity exemplified by Netflix Eureka.
Server-Side Service Registry:
Elevate your orchestration game with the server-side registry, where a dedicated server takes the reins of registration and discovery.
Services communicate with the central hub, fostering a streamlined and centralized management system.
Discover the power of Eureka as it redefines the landscape of service orchestration.
In this dynamic dance of connections, Service Registries emerge as the maestros, conducting the symphony of microservices with finesse and precision. πΆβοΈ Ready to embark on a journey where communication is not just efficient but orchestrated to perfection? Welcome to the Service Registry experience! ππ«
What is Service Discovery?
Service Discovery involves the dynamic detection and connection to available services in a network. It complements the Service Registry by allowing services to find and communicate with each other in a decentralized and adaptive manner. ππ
Types of Service Discovery:
Client-Side Service Discovery:
Microservices are responsible for determining the locations of other services.
Commonly involves the use of load-balancing algorithms.
Examples include Ribbon and Spring Cloud. πΉπ€οΈ
Server-Side Service Discovery:
A central server manages the service discovery process.
Microservices query this server to discover other services.
Examples include Netflix Zuul and API Gateway. ππ
In summary, Service Registry and Service Discovery work hand in hand to create a dynamic and efficient microservices ecosystem, ensuring seamless communication and adaptability in a distributed architecture. πβοΈ
Setting up the Eureka Service Registry for the project:
π± Create a new Spring Boot Project:
Use Spring Initializr or your preferred IDE.
Add dependencies: Eureka Server and any other required dependencies.
The dependencies that are required are:
it's always recommended to use the Spring Initializer or Preferred IDE don't create the project from scratch, for reference the maven repositories for the dependencies are provided.
After adding all the dependencies click on generate which will generate a .zip folder, then place it in the required directory then extract the contents.
Ready to Dive In? Open Your Favourite IDE with a Smile!
Launching your coding sanctuary has never been more delightful! Whether you're a fan of IntelliJ, Eclipse, or any other IDE that sparks joy, here's a quick guide:
IntelliJ IDE brings everything together in one place, making coding a seamless and enjoyable experience! which I love the most and I recommend you use it...π
Now, add @EnableEurekaServer
to the main class of the spring application which might differ depending on the name given while creating a spring application.
What is @EnableEurekaServer
?
@EnableEurekaServer
is an annotation in Spring Cloud that is used to enable the Eureka Server functionality in a Spring Boot application. When applied to a class, it turns the Spring Boot application into an Eureka Service Registry. This means the application will act as a central hub where other microservices can register themselves and discover each other.
@SpringBootApplication
@EnableEurekaServer
public class ServiceRegistryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRegistryApplication.class, args);
}
}
After adding the annotation, let's change the application.properties or application.yml (I recommend you to use the application.yml since it provides a tree-like structure and is easy to configure compared to the application.properties)file, which is the configuration file in spring applications:
server:
port: 8761
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka
register-with-eureka: false
fetch-registry: false
spring:
application:
name: SERVICE-REGISTRY
Here the server port can differ, make sure it's not repeated.
eureka.client.service-url:defaultZone
represents where the Eureka server starts.eureka.client.register-with-eureka
takes a boolean, if true(default? then it registers itself with the service registry, since we are creating the Service Registry it has to be false.eureka.client.fetch-registry
by default is set to true, when set to false the service won't fetch the registry of other services from Eureka Server.spring.application.name
is the name with which the service will register itself with the service registry.
Now, run the application in your IDE, or open the command prompt in the directory where the application is present and execute the following command.
mvn clean install //to build your project and download dependencies
mvn spring-boot:run //to start the application
Now access the URL to check if the service registry is up and running: http://localhost:8761/
if the port number is different then, http://localhost:<port-number>/
Now, that we set up the Service Registry let's build the client in the further topics and get it registered with the service registry that we have built together.
Conclusion
Thanks for reading our latest article on Mastering Microservices: A Deep Dive into Spring Cloud Netflix Eureka for Seamless Service Registration and Discovery with practical usage.
You can get the source code for this tutorial from our GitHub repository.
Happy Coding!!!!π
Subscribe to my newsletter
Read articles from Karthik Kulkarni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
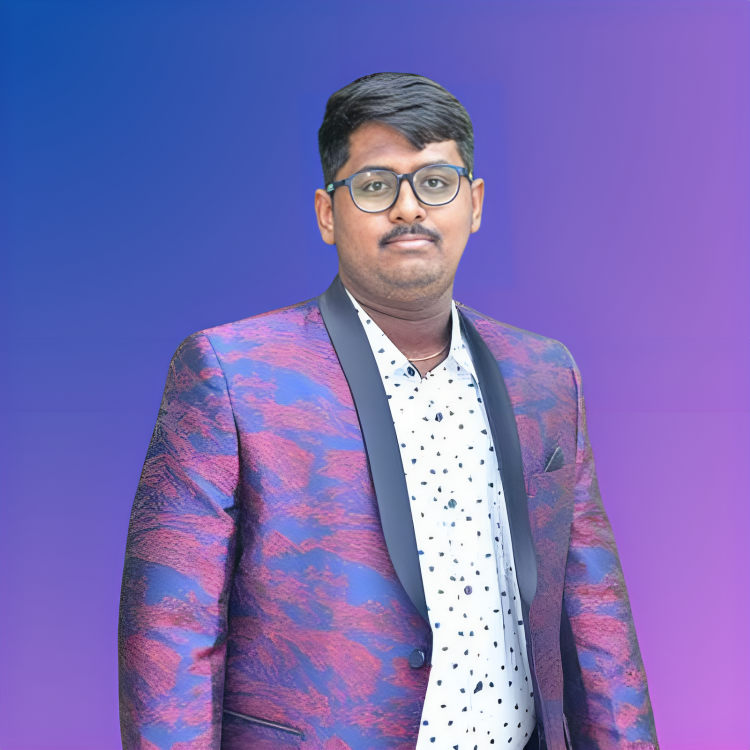
Karthik Kulkarni
Karthik Kulkarni
CSE'23 Grad π | Aspiring Java Developer π | Proficient in Spring, Spring Boot, REST APIs, Postman π» | Ready to Contribute and Grow π