Is Object Empty? LeetCode Question: 2727

Table of contents
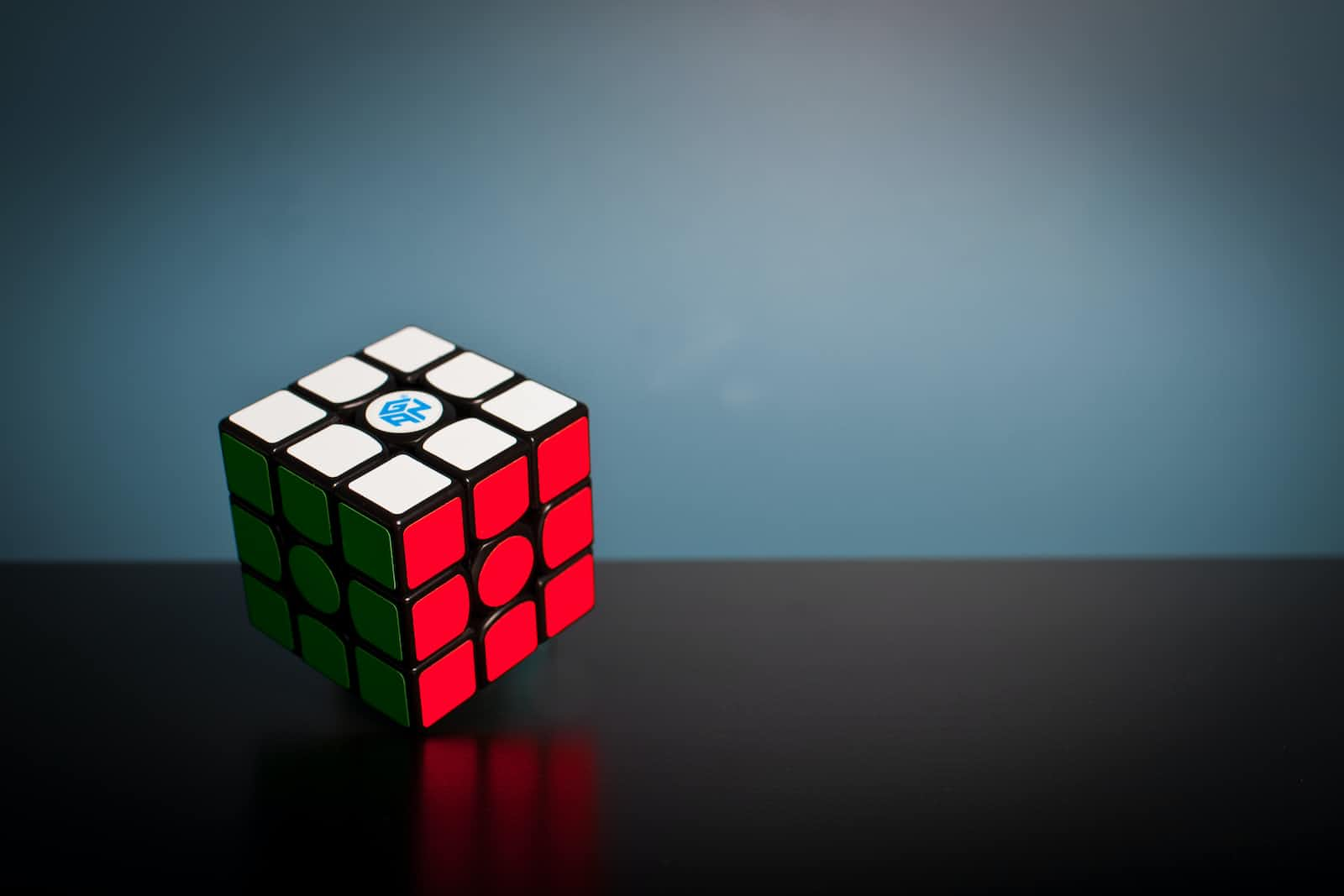
Okay, so let me clear it out: I am not a professional problem solver nor do it regularly, but I randomly came across this leet code question framed 2727. Is Object EmptyI know many of the intermediate or professionals know the answer and can solve this problem in one-liner but I felt the approach was important.
So the question was as follows
Given an object or an array, return if it is empty.
An empty object contains no key-value pairs.
An empty array contains no elements.
You may assume the object or array is the output of
JSON.parse
.Example 1:
Input: obj = {"x": 5, "y": 42} Output: false Explanation: The object has 2 key-value pairs so it is not empty.
Example 2:
Input: obj = {} Output: true Explanation: The object doesn't have any key-value pairs so it is empty.
Example 3:
Input: obj = [null, false, 0] Output: false Explanation: The array has 3 elements so it is not empty.
Before moving on I would like to appreciate the team of @hasnode, this blogging editor is just mind-blowing like just copied the question and example and it automatically formatted it for me🤯🥹🥹
Approach & Solution
So the question asks us to check whether the given array or object is empty or not and return true or false on the basis of it.
Now we all know to check if the array is empty or not we just check the length of array
let arr = [1,2,3,4,5]
console.log(arr.length) // 5
But wait what if the input is Object? For that, we have the Object method. What it does is it converts the given object into an array so that we can apply array methods over the objects, That's it!
So the solution to the problem was
var isEmpty = function(obj) {
if(Object.keys(obj).length===0) return true
else return false
};
Here I directly converted the obj parameter to an array and checked its length.
Note: Object.keys(obj) converts all keys of the object into an array which eventually helps us to check if it is empty or not.
That's it now it will pass all the given test cases and I got the following result
Delighted that you enjoyed the blog! Your support means the world ❤️🌟. Stay tuned for more exciting content and updates—your presence makes it all the more special! 🤗📚 #FollowForMore
Subscribe to my newsletter
Read articles from Atharva Mulgund directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Atharva Mulgund
Atharva Mulgund
Software Developer| Passionate about MERN | React.js | Angular | Javascript | Typescript