Constructor Function in Javascript

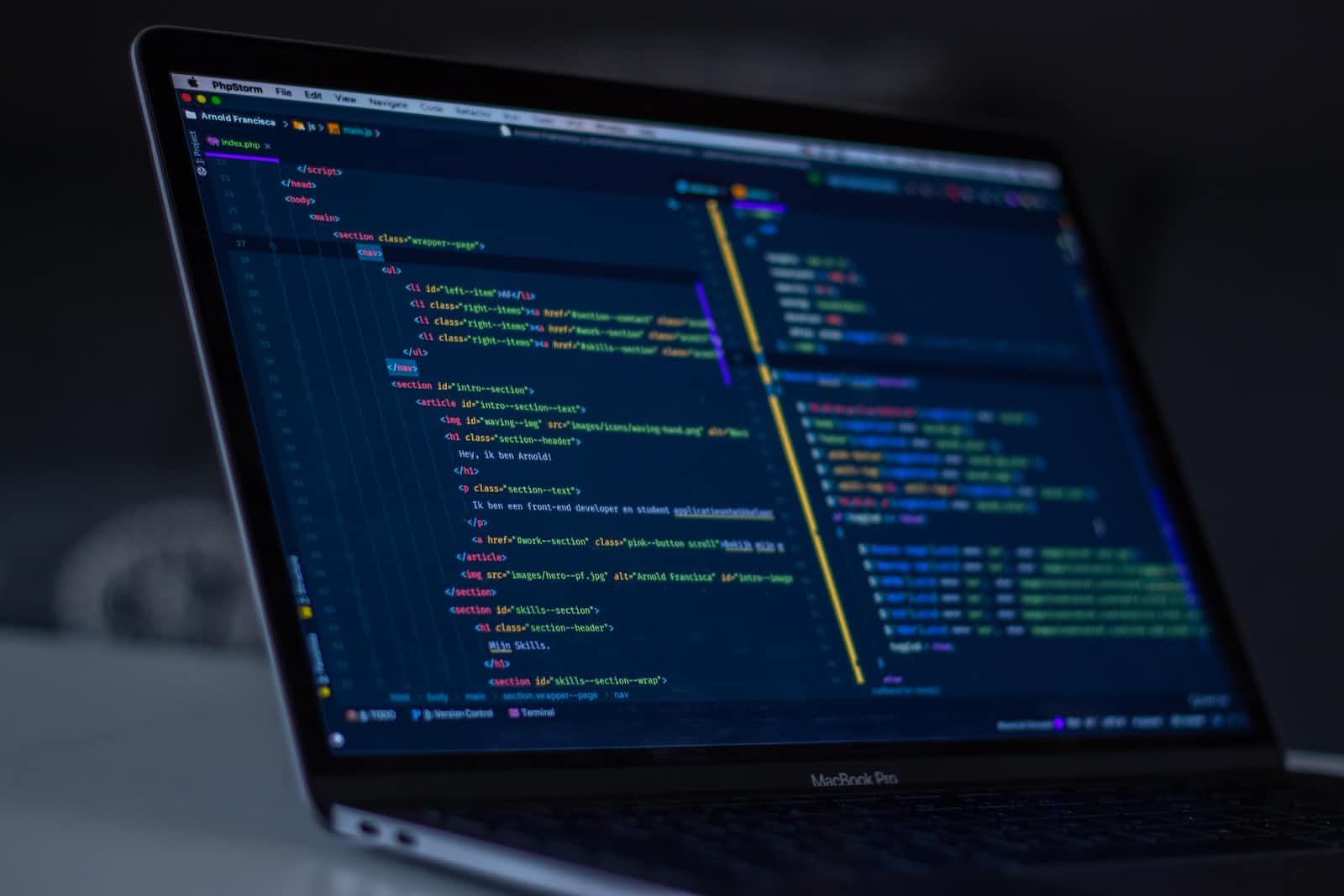
Introduction
In Javascript, there are multiple ways to create an object.
Simply declare a variable & assign an object to it (a.k.a. Object Literal
), use Object.
create
method or use Class
The Constructor Function is just another way to create objects.
So why use it?
They are particularly useful when we want to create multiple objects of similar type.
They can also be thought of as an alternative to the traditional Class based syntax.
Understanding Fundamentals
Well, constructor functions are technically regular functions with a few conventions to be followed.
They are named with the first letter as a capital letter
function Contructorfunction () { // ...rest of content }
They should be called only with
"new"
operator.function Contructorfunction () { // ...rest of content } const constructorFunctionObject = new ConstructorFunction();
💡Calling constructor functions withoutnew
keyword is as good as calling a regular function & it kills the whole point of using the Constructor Function 🤪
What magic does new
keyword do?
A new empty object is created and assigned to
this
.When the function is called the function body executes & value of
this
is returned.
What if we accidentally call the function without new
?
We do have an inbuilt validation available for such a scenario.
function Contructorfunction () {
if (!new.target) { // if you run me without new
// handle in your custom way
}
// ...rest of content
}
An alternate syntax
we can omit parentheses after new
function Contructorfunction () {
// ...rest of content
}
const constructorFunctionObject = new ConstructorFunction;
Good To Know
Consider this example,
function Person (name, age) {
this.name = name,
this.age = age,
}
const person1 = new Person('John', 22);
const person2 = new Person('Max', 25);
Although person1
& person2
has similar properties, the instances are separate.
We can also add new properties & methods to a particular object without affecting other instances of the function.
function Person (name, age) {
this.name = name,
this.age = age,
}
const person1 = new Person('John', 22);
const person2 = new Person('Max', 25);
// adding new property to person1
person1.email = 'john@gmail.com';
// try to console log person2.email.
// It will throw error
// Property email is only available for person1
// Similar rules apply for methods
Subscribe to my newsletter
Read articles from Varun Kelkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Varun Kelkar
Varun Kelkar
Javascript | React | Node | Lit Web Components | Microfrontends