Reverse of a string

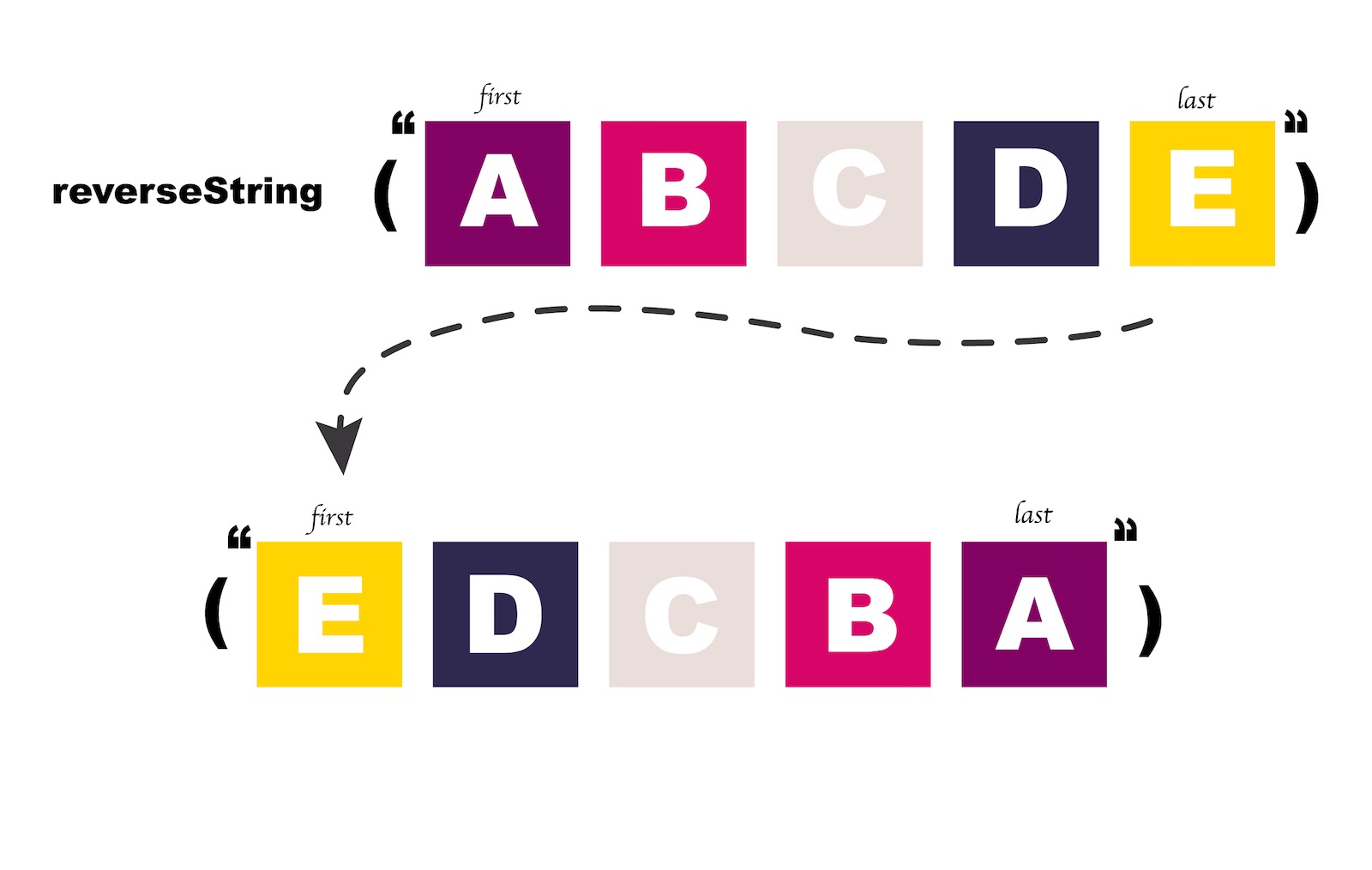
Method 1:
public class StringReverse {
public static void main(String[] args) {
String s = "code with siri";
String str="";
System.out.println("Original"+s);
for(int i=s.length()-1;i>=0;i--){
str = str+s.charAt(i);
}
System.out.println("Reversed"+str);
}
}
Take string s = "code with siri"
we want to reverse the string in "iris htiw edoc"
finding length of the string using length() function: s.length()
for(int i=14-1; i>=0; i--)
i = 13 => str = str + s.charAt(i)
str = ""+i = i
decrementing i = i--
i = 12 => str = str + s.charAt(i)
str = i + r = ir
decrementing i = i--
i = 11 => str = str + s.charAt(i)
str = ir + i = iri
decrementing i = i--
i = 10 => str = str + s.charAt(i)
str = iri + s = iris
decrementing i = i--
i = 9 => str = str + s.charAt(i)
str = iris + " " = iris (added space after s)
decrementing i = i--
this process continued till i=0 Then print output as reversed string.
In this process, every iteration of the last character comes to the first.
Method 2:
public class StringReverse {
public static void main(String[] args) {
String s = "code with siri";
System.out.println("Original"+s);
StringBuilder sb = new StringBuilder(s);
sb.reverse();
System.out.println("Reversed"+sb);
}
}
StringBuilder is mutable. if we make any change in the StringBuilder it directly reflects on the string.
string assign to StringBuilder(s)
StringBuilder sb = new StringBuilder(s);
Then sb. reverse(). It will reverse the string and print the reversed string
Method 3:
public class StringReverse {
public static void main(String[] args) {
String s = "code with siri";
System.out.println(s);
char[] str = s.toCharArray();
//finding the length of the array
int len = str.length;
for(int i=0,j=len-1;i<len/2;i++,j--){
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
String r = new String(str);
System.out.println("Reversed"+r);
}
}
Converting the string into a character array
char[] str = s.toCharArray();
For loop in every iteration, first and last will be swapped and increment the starting index(i++) and decrement the last index(j--)
for(int i=0,j=len-1;i<len/2;i++,j--){
char temp = str[i];
str[i] = str[j];
str[j] = temp;
}
converting the character array to a string
String r = new String(str);
Finally, print the reversed string.
Recursion
Recursion without return:
public class StringReverse {
public static void main(String[] args) {
String s = "siri";
rev(s,s.length()-1);
}
public static void rev(String s,int len){
if(len ==-1){
return;
}
String str = "";
str = str + s.charAt(len);
System.out.print(str);
//recursion call
rev(s,len-1);
}
}
output: iris
Recursion with return:
public class StringReverse {
public static void main(String[] args) {
String s = "siri";
System.out.println(rever(s, s.length()-1));
}
public static String rever(String s,int len){
if(len ==-1){
return "";
}
return s.charAt(len) + rever(s,len-1);
}
}
output: iris
Happy coding :)
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
