Go's Building Blocks: Structs and Custom Data Structures
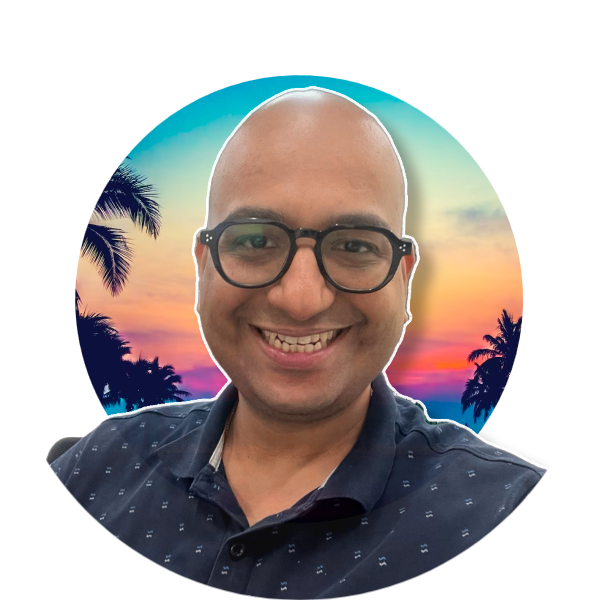
Table of contents
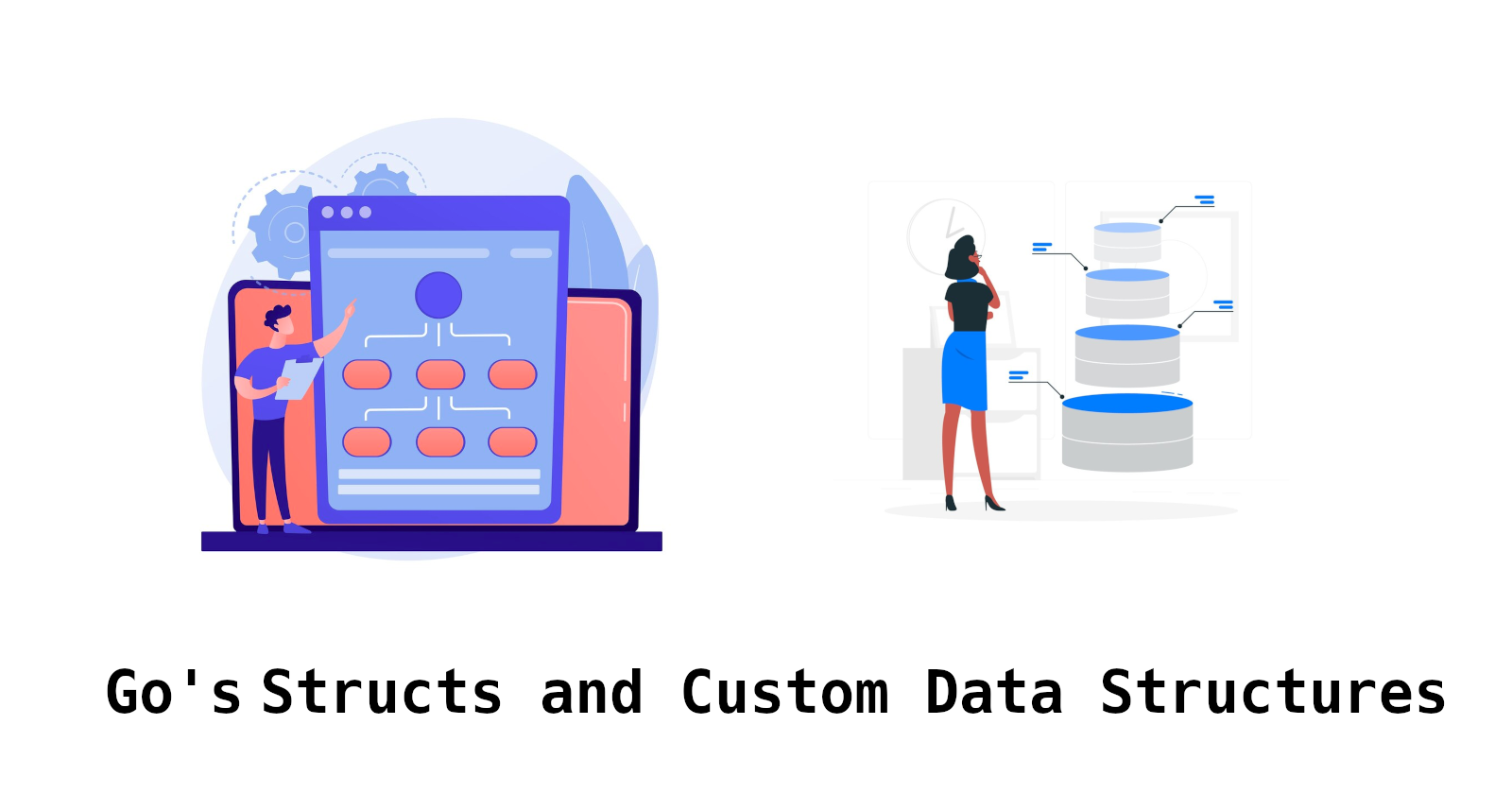
Introduction
Welcome to the world of Go's structured data! In this guide, we'll delve into structs and custom data structures, the cornerstones of organized data in Go. Understanding how to create and use these structures is essential for building complex and well-organized Go applications. Whether you're an experienced Python developer or new to programming, Go's simplicity and flexibility will make this journey exciting.
Simpler Explanation
Imagine structs as containers where you can neatly organize different types of information. Think of custom data structures as unique Lego sets you create for your specific needs, combining different pieces for a particular purpose.
Sample Code
Structs in Go:
package main
import "fmt"
// Define a struct for a person
type Person struct {
FirstName string
LastName string
Age int
}
func main() {
// Create an instance of the Person struct
person := Person{
FirstName: "John",
LastName: "Doe",
Age: 30,
}
// Access and print fields of the struct
fmt.Printf("Name: %s %s, Age: %d\n", person.FirstName, person.LastName, person.Age)
}
Creating Custom Data Structures:
package main
import "fmt"
// Define a custom data structure
type Point struct {
X, Y int
}
func main() {
// Create instances of the custom Point data structure
p1 := Point{X: 2, Y: 5}
p2 := Point{X: -1, Y: 3}
// Perform operations with custom data structures
result := Point{X: p1.X + p2.X, Y: p1.Y + p2.Y}
// Print the result
fmt.Printf("Resulting Point: (%d, %d)\n", result.X, result.Y)
}
In the struct example, we define a
Person
struct, create an instance and access its fields.In the custom data structure example, we define a
Point
data structure and perform operations on instances.
Conclusion
You've now embarked on a journey into the world of Go's structured data, where structs and custom data structures are your tools for organized information. Structs help you define structured data, and custom data structures allow you to create tailored solutions for your needs. Armed with this knowledge, you're well-equipped to design and manage data effectively in your Go applications. Continue exploring, experimenting, and building to become a proficient Gopher!
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
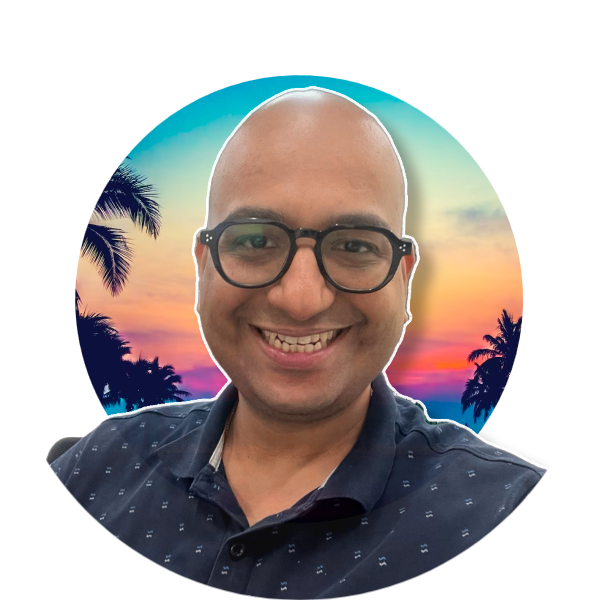
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.