Loops - Unlocking The Magic of Python's Repetitive Power
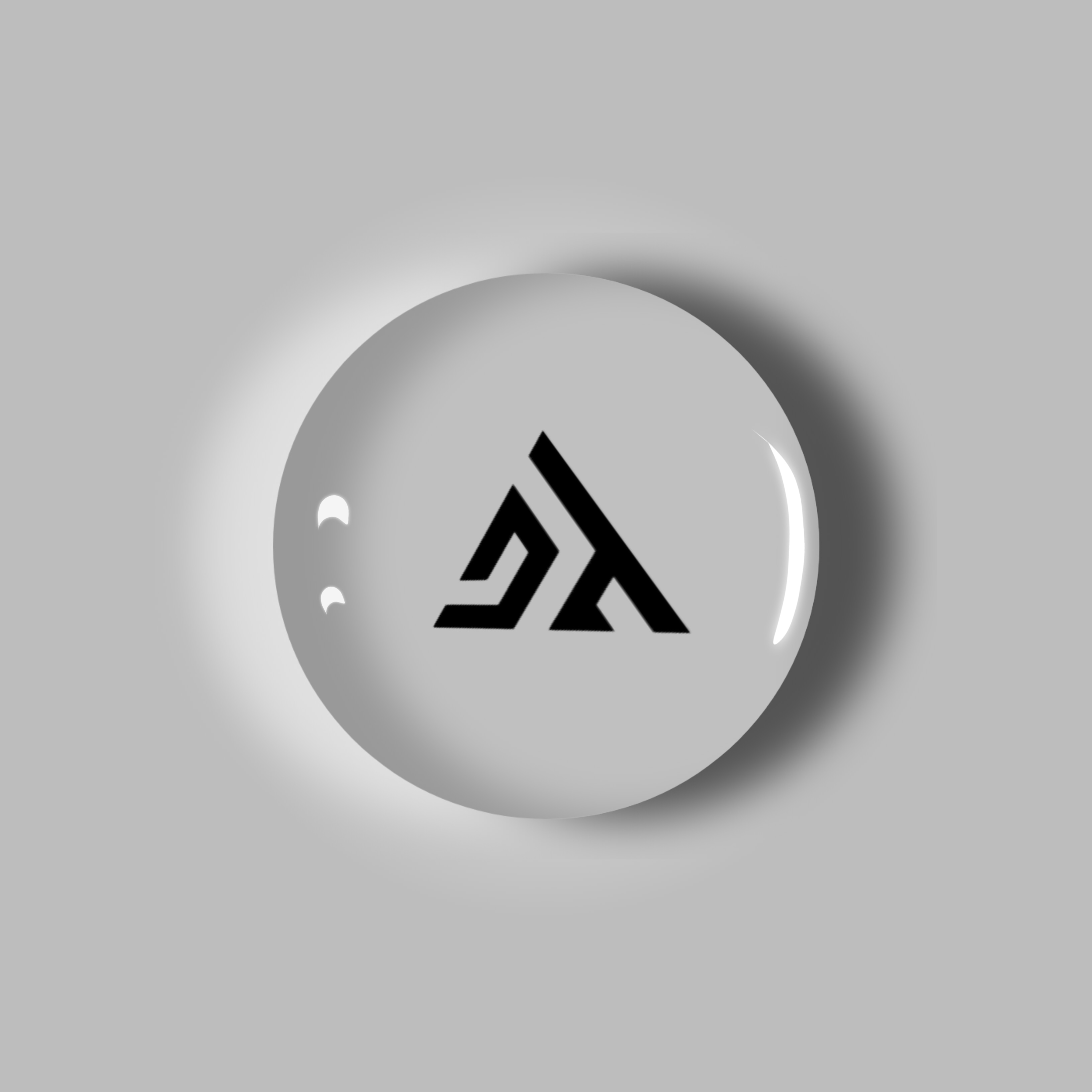
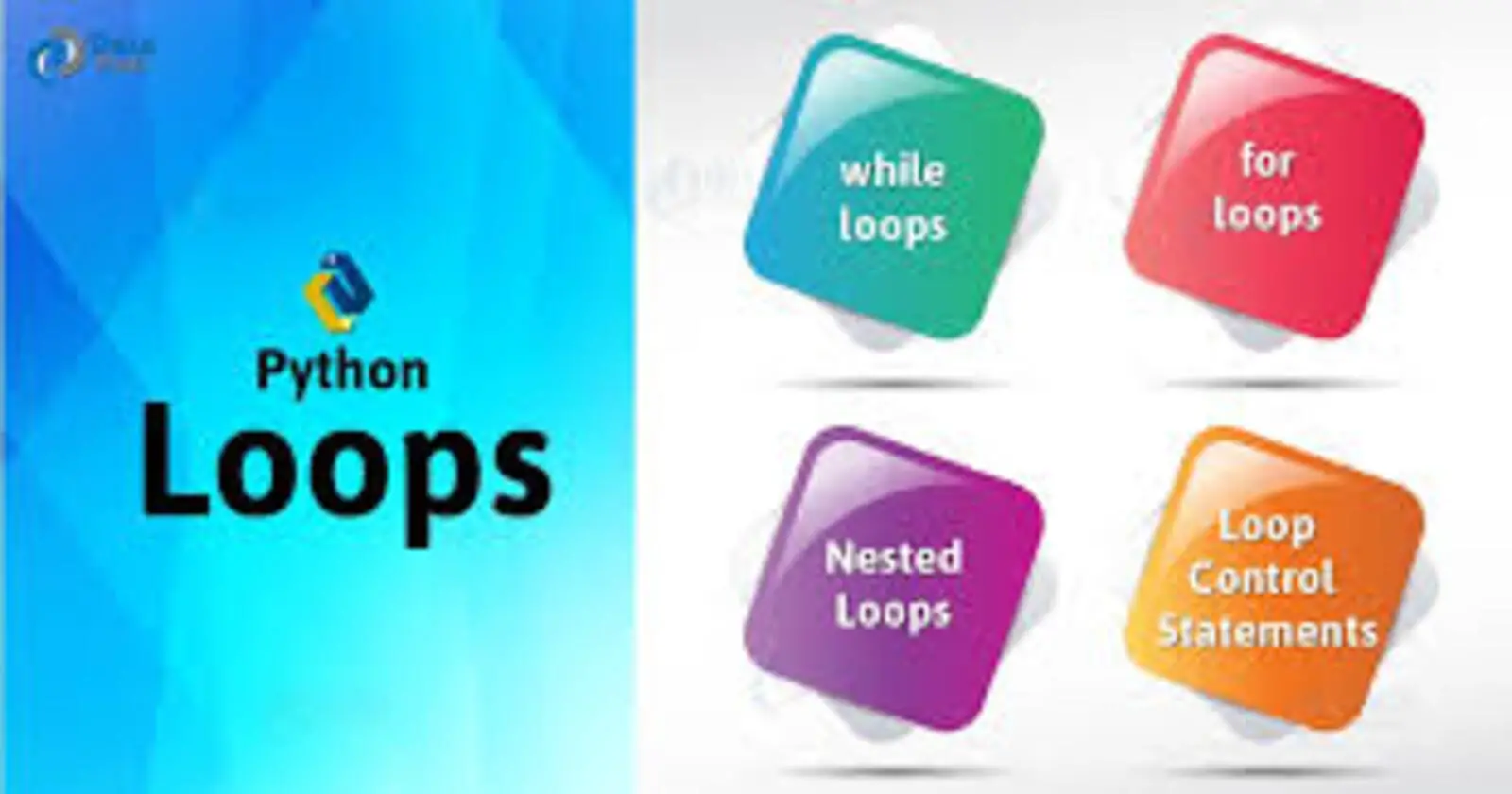
Table of Contents
Introduction
Section 1: for Loops
1.1. Basic for Loop
1.2. Iterating Over Lists and Collections
1.3. Looping Through Strings
1.4. The range()
Function
Section 2: while Loops
2.1. Basic while Loop
2.2. Infinite Loops and Break Statements
Section 3: Nested Loops
3.1. Nested for Loops
3.2. Nested while Loops
Section 4: Loop Control Statements
4.1. continue
Statement
4.2. pass
Statement
4.3. Loop Control with else
Block
Section 5: Best Practices and Tips
5.1. Choosing the Right Loop for the Task
5.2. Optimization and Performance Considerations
5.3. Code Readability and Style
Conclusion
Introduction
Loops are the unsung heroes of programming, the magic incantations that allow us to unleash the repetitive power of Python. They are the essential tools that transform lines of code into automated tasks, enabling us to perform computations, manipulate data, and control the flow of our programs with elegance and efficiency.
In this comprehensive tutorial, we embark on a journey to unravel the art of Python's loops. Whether you're a seasoned developer or a novice coder, understanding and mastering loops is a fundamental skill that will empower you to tackle a wide range of programming challenges. With loops at your disposal, you can traverse data, perform iterative tasks, and breathe life into your code.
This tutorial is your key to unlocking the world of Python's looping constructs. We will explore the ins and outs of both for
and while
loops, uncover the secrets of nested loops, and discover how loop control statements can shape the behavior of your code. Moreover, we will delve into best practices, optimization strategies, and coding style tips to ensure that your loops are not only functional but also maintainable and efficient.
Are you ready to embrace the repetitive power of Python's loops and discover the art of automation? Let's embark on this journey together and uncover the magic that lies within the world of loops.
SECTION 1: For Loops
Python's for
loops are the workhorses of repetitive tasks, and they can be used to iterate through various data structures and sequences. In this section, we will explore the versatility and functionality of for
loops, starting with the basic structure and moving on to more advanced applications.
1.1. Basic for Loop
Syntax and Iteration Mastery
At the heart of every for
loop lies the fundamental structure, which is crucial for iterating over a sequence of elements. In a basic for
loop, you specify the sequence you want to iterate through, and for each item in that sequence, a block of code is executed.
Syntax
for item in sequence:
# Code to execute for each item
Illustration: Let's consider a scenario where you want to print the numbers from 1 to 5 using a for
loop.
for number in [1, 2, 3, 4, 5]:
print(number)
In this example, the for
loop iterates through the list [1, 2, 3, 4, 5]
, and for each item in the list, it executes the code to print the number.
1.2. Iterating Over Lists and Collections
Unlocking Data Structures
for
loops are not limited to simple sequences; they can handle lists, tuples, and even dictionaries. We'll explore how to iterate through these collections effectively.
Illustration: Suppose you have a list of names and you want to greet each person using a for
loop.
names = ["Alice", "Bob", "Charlie", "David"]
for name in names:
print(f"Hello, {name}!")
In this example, the for
loop iterates through the names
list, and for each name in the list, it greets the person.
1.3. Looping Through Strings
Character by Character Exploration
Strings can be thought of as sequences of characters, and for
loops are perfect for traversing them character by character.
Illustration: Let's say you want to count the number of vowels in a given string using a for
loop.
text = "Hello, Python!"
vowel_count = 0
for char in text:
if char in "AEIOUaeiou":
vowel_count += 1
print(f"The text has {vowel_count} vowels.")
In this script, the for
loop goes through each character in the text
string and checks if it's a vowel, updating the vowel_count
accordingly.
1.4. The range()
Function
Generating Number Sequences
The range()
function is a valuable companion for for
loops, enabling you to generate sequences of numbers effortlessly.
Illustration: Let's generate the first five square numbers using a for
loop and the range()
function.
for number in range(1, 6):
square = number ** 2
print(f"The square of {number} is {square}")
In this example, the for
loop works in tandem with the range()
function to iterate from 1 to 5, calculating and printing the squares of the numbers.
SECTION 2: While Loops
While for
loops are excellent for iterating over known sequences, while
loops provide the flexibility to repeat a block of code until a specific condition is no longer true. In this section, we will delve into the intricacies of while
loops, from the basics to handling infinite loops with the strategic use of break
statements.
2.1. Basic while Loop
Looping with a Purpose
The while
loop is a powerful construct that allows you to execute a block of code as long as a certain condition holds true. It's particularly useful when the number of iterations is not predetermined.
Syntax
while condition:
# Code to execute as long as the condition is True
Illustration: Let's consider a scenario where you want to print numbers from 1 to 5 using a while
loop.
counter = 1
while counter <= 5:
print(counter)
counter += 1
In this example, the while
loop continues to execute as long as the counter
is less than or equal to 5. The counter increments with each iteration, ensuring the loop terminates after printing the numbers.
2.2. Infinite Loops and Break Statements
Balancing on the Edge
Infinite loops, where the condition always holds true, are a potential pitfall with while
loops. We'll explore how to handle these situations gracefully using the break
statement.
Illustration: Suppose you want to take user input for a password until it matches a predefined one, and you want to avoid an infinite loop.
correct_password = "secure123"
while True:
user_input = input("Enter the password: ")
if user_input == correct_password:
print("Access granted!")
break
else:
print("Incorrect password. Try again.")
In this script, the while True
creates an infinite loop. The loop continues until the user enters the correct password. Once the condition is met, the break
statement is encountered, and the loop is exited.
SECTION 3: NESTED LOOPS
In Python, the power of loops isn't limited to simple, linear structures. Nested loops introduce a whole new level of sophistication, allowing you to traverse intricate patterns, matrices, and multi-dimensional data structures. In this section, we'll unravel the intricacies of nested loops, exploring both for
and while
loop combinations.
3.1. Nested for Loops
Unveiling the Depths
Nested for
loops provide a mechanism for traversing multiple levels of data structures. They allow you to iterate over each element in one loop while having another loop inside it.
Syntax
for outer_item in outer_sequence:
for inner_item in inner_sequence:
# Code to execute for each inner_item
Illustration: Let's consider an example where you want to print a pattern of stars using nested for
loops.
for i in range(5):
for j in range(i + 1):
print("*", end=" ")
print()
In this script, the outer loop (for i in range(5)
) controls the number of rows, and the inner loop (for j in range(i + 1)
) handles the number of stars in each row.
3.2. Nested while Loops
Layers of Complexity
Just like for
loops, while
loops can also be nested, allowing you to create complex iterations based on changing conditions.
Syntax
outer_condition = True
while outer_condition:
inner_condition = True
while inner_condition:
# Code to execute as long as inner_condition is True
if some_condition:
inner_condition = False
# Code to execute as long as outer_condition is True
if some_other_condition:
outer_condition = False
Illustration: Let's illustrate nested while
loops with a script that generates a number pyramid.
row = 1
while row <= 5:
space = 5
while space > row:
print(" ", end=" ")
space -= 1
number = 1
while number <= row:
print(number, end=" ")
number += 1
print()
row += 1
In this example, the outer while
loop controls the number of rows, and the inner while
loops handle spaces and numbers in each row.
SECTION 4: LOOP CONTROL STATEMENTS
In the realm of loops, control statements serve as instruments of fine-tuning, enabling you to shape the flow of your code with precision. In this section, we will unravel the subtleties of loop control statements—continue
, pass
, and the else
block—providing you with the tools to navigate loops with finesse.
4.1. continue
Statement
Skipping a Beat
The continue
statement allows you to skip the rest of the code inside a loop for the current iteration and move to the next iteration.
Syntax
for item in sequence:
if condition:
continue
# Code to execute if condition is False
Illustration: Let's say you want to print only the even numbers from a list using the continue
statement.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number % 2 != 0:
continue
print(number)
In this script, the continue
statement skips the print
statement for odd numbers, allowing only even numbers to be printed.
4.2. PASS
Statement
Placeholder Harmony
The pass
statement is a no-operation statement; it acts as a placeholder when syntactically some code is required but no action is desired.
Syntax
Illustration: Consider a scenario where you want to print even numbers and do nothing for odd numbers.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number % 2 != 0:
pass
else:
print(number)
In this example, the pass
statement serves as a placeholder, indicating that no specific action is required for odd numbers.
4.3. Loop Control with else
Block
Unexpected Allies
Surprisingly, loops in Python can have an else
block, which is executed when the loop condition becomes False. It's not an else
block for an if
statement but an else
block for a loop.
Syntax
for item in sequence:
# Code to execute for each item
else:
# Code to execute when the loop condition becomes False
Illustration: Let's say you want to search for an element in a list and print a message if it's not found.
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 6:
print("Number found!")
break
else:
print("Number not found!")
In this script, the else
block is executed only if the loop completes without encountering a break
statement.
SECTION 5: BEST PRACTICES AND TIPS
In the final stretch of our journey through the world of Python loops, let's delve into best practices and tips that will not only enhance the efficiency of your code but also make it more readable and maintainable.
5.1. Choosing the Right Loop for the Task
Precision in Selection
When faced with the decision of whether to use a for
or while
loop, consider the nature of your task. for
loops are ideal for iterating over sequences, such as lists, whereas while
loops shine when the number of iterations is unknown. Choose the loop that best aligns with the structure of your data and the logic of your task.
Illustration: Suppose you want to iterate through a list of names to print a greeting. A for
loop would be a natural choice.
names = ["Alice", "Bob", "Charlie"]
for name in names:
print(f"Hello, {name}!")
5.2. Optimization and Performance Considerations
Streamlining Execution
While Python is known for its readability, optimizing loop performance is crucial, especially in large-scale applications. Techniques like list comprehension and built-in functions often outperform traditional loops. Additionally, consider using the timeit
module to profile and benchmark your code for performance improvements.
Illustration: Transforming a list of numbers into their squares using list comprehension.
numbers = [1, 2, 3, 4, 5]
squares = [number ** 2 for number in numbers]
print(squares)
5.3. Code Readability and Style
Crafting Clear and Elegant Code
Maintaining a consistent and readable coding style enhances collaboration and long-term code maintainability. Follow the PEP 8 style guide recommendations for indentation, naming conventions, and other formatting aspects. Use meaningful variable and function names to ensure clarity.
Illustration: Applying PEP 8 recommendations for code readability.
# Good naming conventions
def calculate_area(radius):
pi = 3.14
return pi * radius ** 2
# Poor naming conventions
def cal_a(r):
p = 3.14
return p * r ** 2
CONCLUSION
In this comprehensive exploration of Python loops, you've journeyed from the basics to the nuanced aspects of looping constructs. You've mastered the art of iteration with for
and while
loops, delved into the complexity of nested loops, and harnessed control statements to shape the flow of your code. Understanding best practices has equipped you to write efficient, readable, and maintainable loop structures.
As you venture forth in your Python programming endeavors, remember that loops are not just a means to an end but a powerful tool in your coding arsenal. Choose the right loop for the task, optimize for performance when necessary, and always prioritize code readability and style. With this knowledge, you're well-prepared to tackle a myriad of programming challenges and wield the magic of loops with finesse. Happy coding!
Subscribe to my newsletter
Read articles from Abakpa Dominic directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
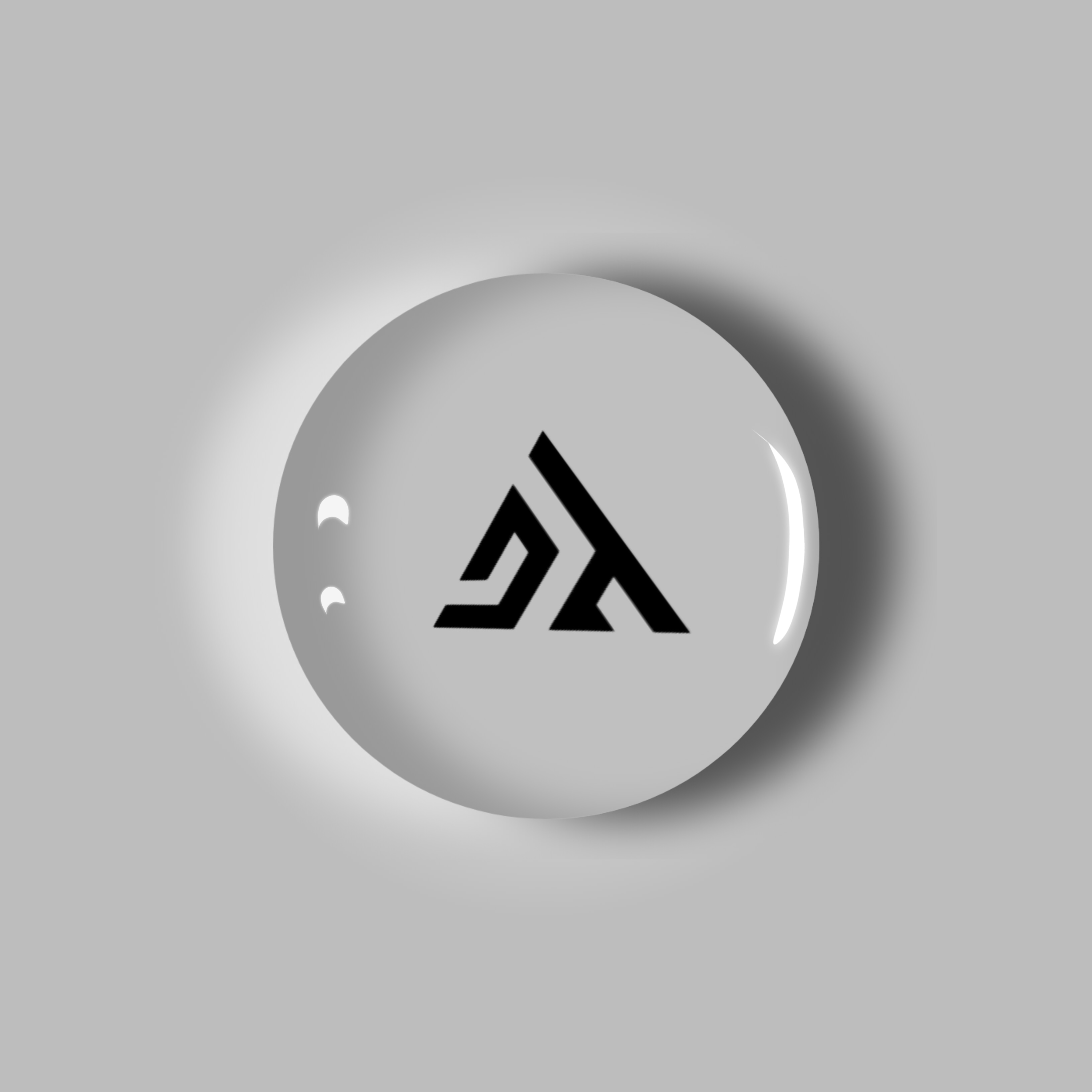
Abakpa Dominic
Abakpa Dominic
👨💻 Backend Dev with a ❤️ for Tech | Solving Problems, One Line of Code at a Time | Embracing Challenges with a Grin 😄 | Coffee in Hand, Debugging in Mind ☕ | Coding by Day, Gaming by Night 🎮 | Join me on this tech adventure! 🚀 #BackendDeveloper #TechLover #ProblemSolver #FunInCoding