Exploring Sealed Interfaces in Java 17
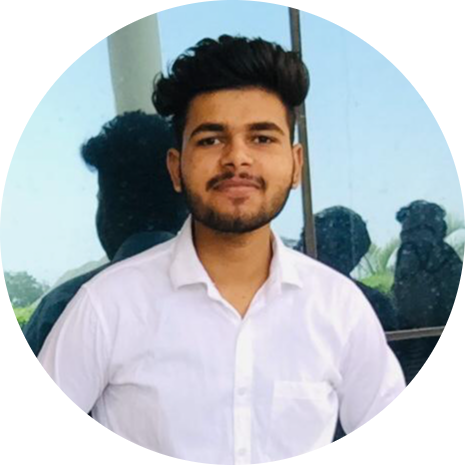
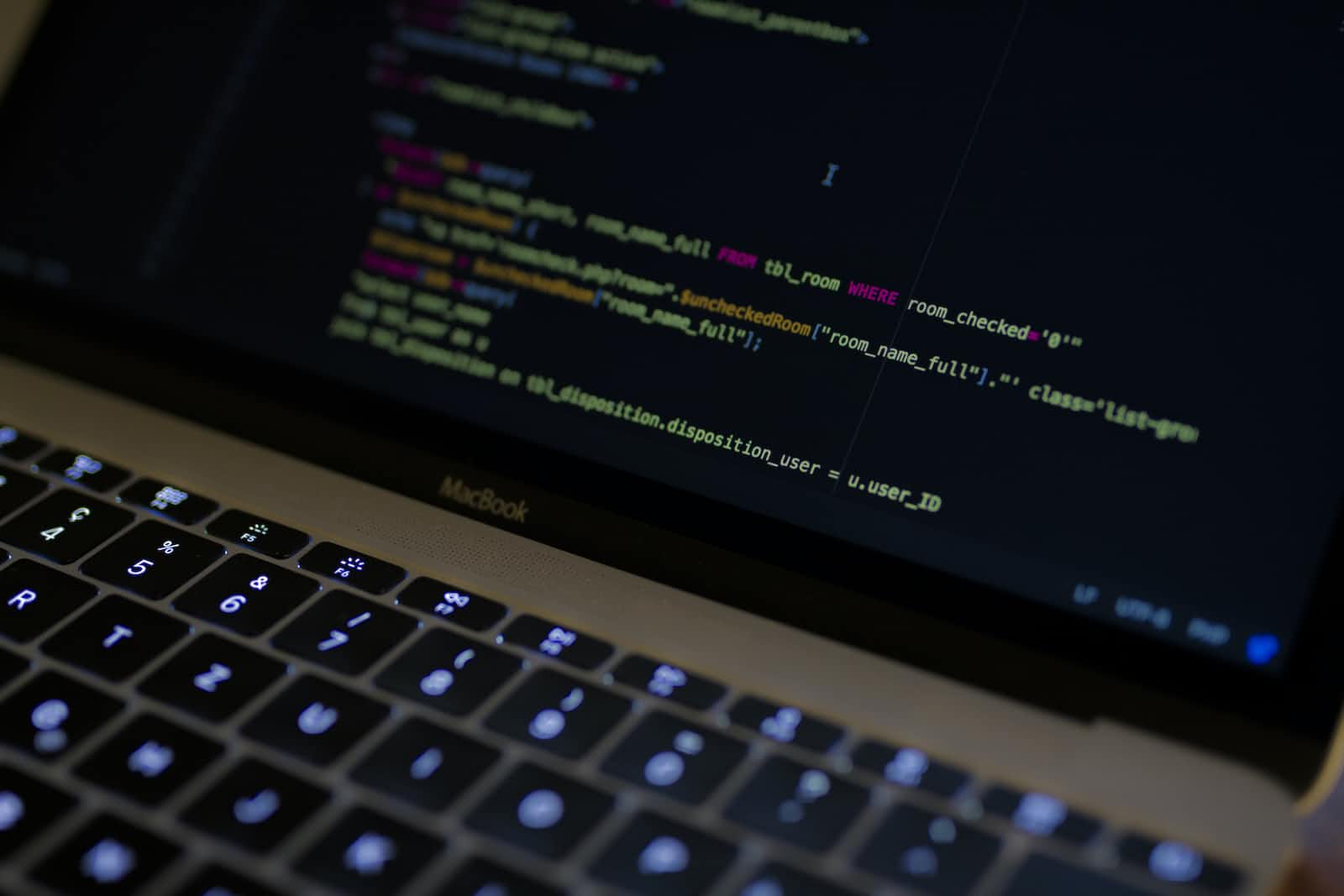
Sealed Interfaces
Java continues to evolve, and with the release of Java 17, we see the introduction of sealed interfaces. Sealed interfaces enhance the way we define and control the inheritance hierarchy in Java, providing a more structured and secure approach. In this blog post, we'll explore sealed interfaces, understand their significance, and learn how to leverage them in our code.
The Need for Sealed Interfaces
Traditionally, interfaces in Java were open for extension by default, meaning any class could implement an interface. While this flexibility is powerful, it can lead to unintended consequences, such as an uncontrolled growth of the implementation classes or potential security issues. Sealed interfaces address these concerns by allowing developers to define a limited set of classes that can implement an interface.
Declaring a Sealed Interface
To declare a sealed interface, use the sealed
modifier followed by the permits
clause, specifying the permitted implementing classes. Let's consider an example:
public sealed interface Shape permits Circle, Square, Triangle {
double calculateArea();
}
In this example, the Shape
interface is sealed and permits only the classes Circle
, Square
, and Triangle
to implement it.
Implementing Sealed Interfaces
When implementing a sealed interface, a class must explicitly declare its intent to implement the interface. For instance:
public final class Circle implements Shape {
private double radius;
// Constructor, methods, etc.
@Override
public double calculateArea() {
// Implementation for calculating the area of a circle
}
}
Attempting to implement the Shape
interface in a class not listed in the permits
clause will result in a compilation error, enforcing the constraints defined by the sealed interface.
Benefits of Sealed Interfaces
1. Controlled Inheritance Hierarchy:
Sealed interfaces provide a clear and controlled inheritance hierarchy by specifying which classes are allowed to implement the interface. This can lead to more maintainable and secure code.
2. Enhanced Readability:
By explicitly listing permitted classes, developers reading the code gain immediate insight into the possible implementations of an interface. This improves code readability and reduces the likelihood of unintentional misuse.
3. API Stability:
Sealed interfaces contribute to API stability. As new classes are added, developers can easily identify which interfaces they are permitted to implement, minimizing the risk of breaking existing code.
Conclusion
Sealed interfaces in Java 17 offer a valuable tool for structuring and securing your codebase. By explicitly defining the allowable implementations, developers can create more robust and readable systems. As you explore Java 17, consider incorporating sealed interfaces into your design to take advantage of these benefits and contribute to the evolution of Java programming.
Happy coding❤️!
Subscribe to my newsletter
Read articles from Mukul kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
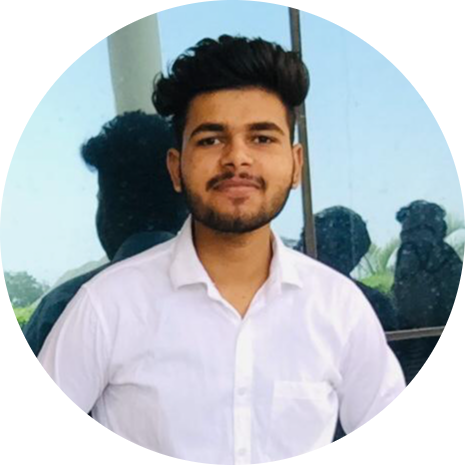
Mukul kumar
Mukul kumar
Hey! I am a software Engineer who is skilled in Java backend and has a good understanding of Spring Boot and Quarkus Frameworks