Day 71 Task : Interview Questions of Terraform
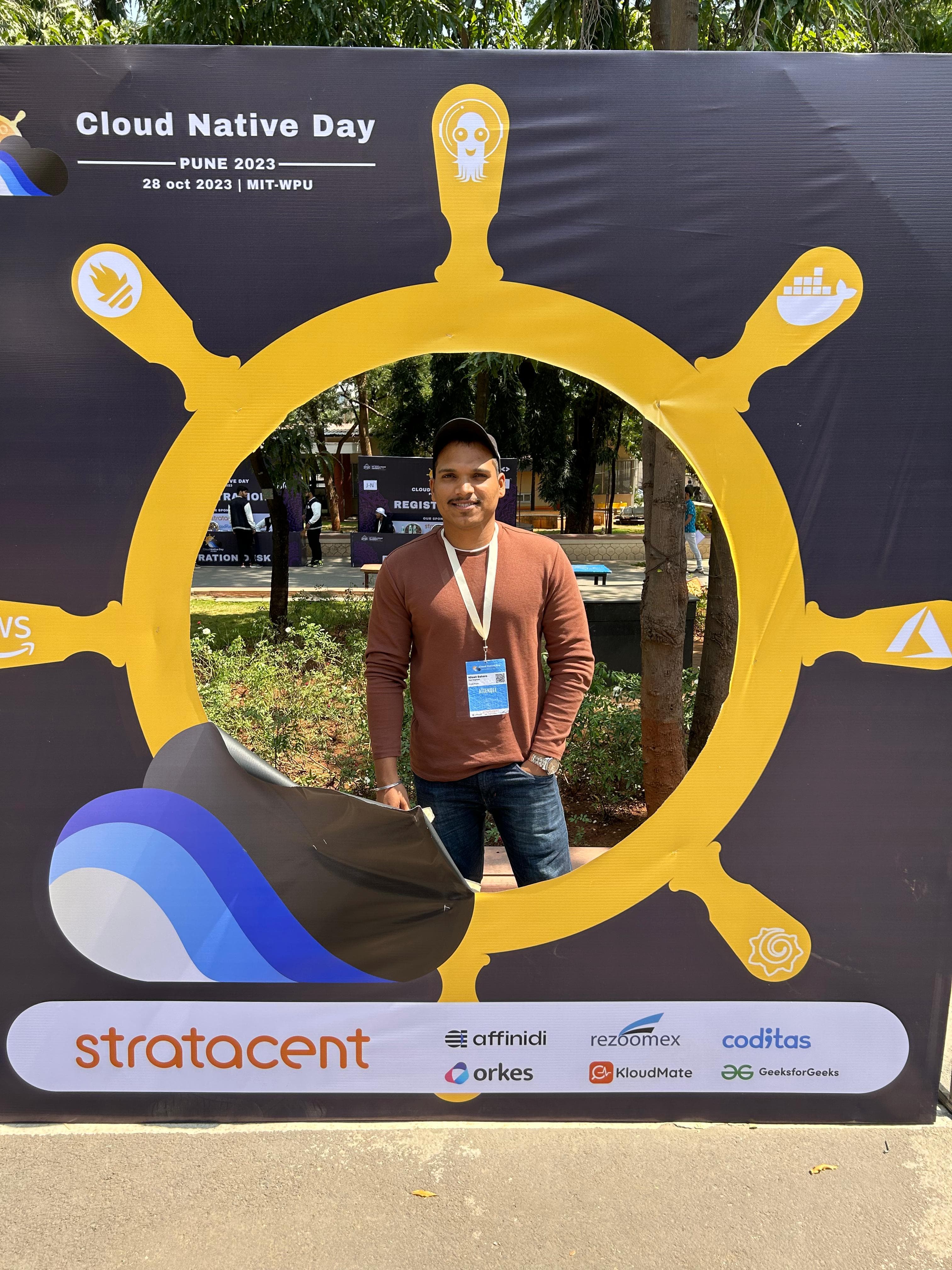
Table of contents
- 1. What is Terraform and how it is different from other IaaC tools?
- 2. How do you call a main.tf module?
- 3. What exactly is Sentinel? Can you provide a few examples that we can use for Sentinel policies?
- 4. You have a Terraform configuration file that defines an infrastructure deployment. However, there are multiple instances of the same resource that need to be created. How would you modify the configuration file to achieve this?
- 5. You want to know from which paths Terraform is loading providers referenced in your Terraform configuration (*.tf files). You need to enable debug messages to find this out. Which of the following would achieve this?
- 6. The below command will destroy everything that is being created in the infrastructure. Tell us how would you save any particular resource while destroying the complete infrastructure.
- 7. Which module is used to store the .tfstate file in S3?
- 8. How do you manage sensitive data in Terraform, such as API keys or passwords?
- 9. You are working on a Terraform project that needs to provision an S3 bucket, and a user with read and write access to the bucket. What resources would you use to accomplish this, and how would you configure them?
- 10. Who maintains Terraform providers?
- 11. How can we export data from one module to another?

1. What is Terraform and how it is different from other IaaC tools?
Terraform is an IaC tool by HashiCorp. Unlike others, it uses a declarative syntax where you describe your desired infrastructure state, letting Terraform handle the "how." It's cloud-agnostic, supports multi-cloud environments, promotes code reusability with resource modules, maintains infrastructure state, excels in parallel execution, and has a robust community. This makes it efficient, reliable, and versatile for managing infrastructure at scale.
2. How do you call a main.tf module?
In Terraform, we don't explicitly call a "main.tf" module. Instead, Terraform automatically processes all ".tf" files in the working directory as part of its configuration. So, the main configuration module is essentially the collection of all these ".tf" files. This approach simplifies module organization and reduces the need for explicit calls.
3. What exactly is Sentinel? Can you provide a few examples that we can use for Sentinel policies?
Sentinel is a policy as a code framework developed by HashiCorp. It's used to enforce policies and governance across infrastructure as code (IaC) deployments, especially in Terraform. Sentinel policies are written in a high-level language and can be used to prevent or warn about certain actions during Terraform runs. For example, we can create policies to ensure that only approved AWS instance types are used, or to restrict the creation of public S3 buckets. These policies help maintain compliance, security, and operational standards within our infrastructure.
4. You have a Terraform configuration file that defines an infrastructure deployment. However, there are multiple instances of the same resource that need to be created. How would you modify the configuration file to achieve this?
To create multiple instances of the same resource in Terraform, you can use resource "count" or resource "for_each" depending on your use case. Here's how you can modify the Terraform configuration file:
Using count
resource "aws_instance" "example" {
count = 3 # This will create 3 instances
ami = "ami-12345678"
instance_type = "t2.micro"
}
In this example, it will create three AWS instances based on the specified AMI and instance type.
5. You want to know from which paths Terraform is loading providers referenced in your Terraform configuration (*.tf files). You need to enable debug messages to find this out. Which of the following would achieve this?
A. Set the environment variable TF_LOG=TRACE
B. Set verbose logging for each provider in your Terraform configuration
C. Set the environment variable TF_VAR_log=TRACE
D. Set the environment variable TF_LOG_PATH
The correct option to enable debug messages and determine from which paths Terraform is loading providers referenced in your configuration is:
A. Set the environment variable TF_LOG=TRACE
Setting TF_LOG=TRACE
will enable detailed debug logging for Terraform, including information about provider loading and configuration. This will help you see the paths from which Terraform is loading providers.
The other options (B, C, and D) do not directly control the level of logging or provide information about provider loading paths.
6. The below command will destroy everything that is being created in the infrastructure. Tell us how would you save any particular resource while destroying the complete infrastructure.
terraform destroy
To save a particular resource while using the terraform destroy
command to destroy the complete infrastructure, you can use the -target
flag to specify the specific resource you want to retain. Here's how you can do it:
terraform destroy -target=resource_type.resource_name
Replace resource_type
with the type of resource (e.g., aws_instance
, aws_s3_bucket
) and resource_name
with the name of the resource you want to save.
7. Which module is used to store the .tfstate file in S3?
The module used to store the .tfstate
file in an S3 bucket is called the "S3 backend." It's a configuration option in Terraform that allows you to store your state file remotely in an S3 bucket instead of locally on your machine. This is a common practice in Terraform to enable state sharing and collaboration among team members.
To configure an S3 backend in Terraform, you typically include a block like the following in your configuration:
terraform {
backend "s3" {
bucket = "your-terraform-state-bucket"
key = "path/to/your/terraform.tfstate"
region = "us-east-1"
encrypt = true
}
}
In this configuration, you specify the S3 bucket where the state file will be stored, the path to the state file within the bucket, the AWS region of the bucket, and whether to encrypt the state file at rest. This configuration ensures that your Terraform state is stored securely and can be accessed by your team members when needed.
8. How do you manage sensitive data in Terraform, such as API keys or passwords?
In Terraform, it's essential to manage sensitive data, such as API keys or passwords, securely to maintain the integrity and security of your infrastructure. There are several best practices for handling sensitive data:
Use Environment Variables: Store sensitive information like API keys, secret keys, and passwords in environment variables on your local machine or CI/CD pipeline. Terraform can access these variables using the
os
package in your configuration files.provider "aws" { region = "us-east-1" access_key = var.AWS_ACCESS_KEY secret_key = var.AWS_SECRET_KEY }
Variable Files: Store sensitive values in separate variable files that are not committed to your version control system (e.g., Git). Instead, share these files securely with authorized team members or systems.
variable "database_password" {}
Terraform Input Variables: Use input variables to pass sensitive data into your Terraform modules securely. Input variables can be defined in a separate variables file or passed in during runtime.
variable "database_password" { type = string default = "" }
State Management: Store Terraform state files securely. Avoid storing sensitive data within the Terraform state. Use a backend like S3 with encryption to store your state file.
HashiCorp Vault: Consider using tools like HashiCorp Vault to manage and retrieve secrets securely. Vault allows you to store, retrieve, and manage sensitive data separately from your Terraform configuration.
Encryption: Ensure that sensitive data, such as passwords or keys, is transmitted and stored securely. Utilize encryption mechanisms provided by cloud providers or third-party solutions.
Access Controls: Implement strict access controls and policies for who can access and modify your Terraform configurations and state files. Use IAM roles and policies to restrict access to sensitive resources.
Audit Trails: Enable auditing and monitoring to track changes to your infrastructure and detect any unauthorized access to sensitive data.
Remember that security is a critical aspect of infrastructure as code (IaC), and it's essential to follow security best practices to protect sensitive information when working with Terraform.
9. You are working on a Terraform project that needs to provision an S3 bucket, and a user with read and write access to the bucket. What resources would you use to accomplish this, and how would you configure them?
To provision an S3 bucket and grant a user read and write access to the bucket in Terraform, you would typically use the following resources:
S3 Bucket Resource (
aws_s3_bucket
): This resource defines the S3 bucket you want to create. You can configure it with properties such as the bucket name, access control settings, versioning, and more.resource "aws_s3_bucket" "example_bucket" { bucket = "my-terraform-bucket" acl = "private" versioning { enabled = true } }
IAM User Resource (
aws_iam_user
): This resource defines the IAM user you want to create. You can configure it with the user's name and other optional properties.resource "aws_iam_user" "example_user" { name = "my-terraform-user" }
IAM User Policy Attachment (
aws_iam_policy_attachment
): To grant the user read and write access to the S3 bucket, you would attach an IAM policy to the user. You can use an existing IAM policy or create a custom one with the necessary permissions.resource "aws_iam_policy" "s3_access_policy" { name = "s3-access-policy" description = "Allows read and write access to S3 bucket" policy = jsonencode({ Version = "2012-10-17", Statement = [ { Action = ["s3:GetObject", "s3:PutObject"], Effect = "Allow", Resource = aws_s3_bucket.example_bucket.arn, }, ], }) } resource "aws_iam_policy_attachment" "attach_s3_policy" { policy_arn = aws_iam_policy.s3_access_policy.arn users = [aws_iam_user.example_user.name] }
In the code above:
We create an S3 bucket named "my-terraform-bucket" with versioning enabled and set its ACL to "private."
We create an IAM user named "my-terraform-user."
We define an IAM policy named "s3-access-policy" that allows the user to perform
s3:GetObject
ands3:PutObject
actions on the S3 bucket.We attach the IAM policy to the user "my-terraform-user."
By doing this, the IAM user will have read and write access to the specified S3 bucket. Make sure to adjust the permissions and policy statements to match your specific requirements and security constraints.
10. Who maintains Terraform providers?
Terraform providers are typically maintained by the organizations or individuals that provide the underlying infrastructure or services being managed by Terraform. These maintainers are responsible for developing, updating, and ensuring the compatibility of Terraform providers with Terraform itself and the services they represent.
11. How can we export data from one module to another?
In Terraform, you can export data from one module to another using output variables in the source module and referencing those outputs in the calling module. Here's a step-by-step approach:
Source Module (exporting data):
- Define an output variable in your source module's
outputs.tf
file, specifying the data you want to export. For example:
output "exported_data" {
value = "This is the data you want to export."
}
- Apply the Terraform configuration for the source module using
terraform apply
. This will create the necessary resources and make the output variable available.
Calling Module (importing data):
- In your calling module, define a data source that references the source module. Create a
.tf
file with the following content:
data "terraform_remote_state" "source_module" {
backend = "local"
config = {
path = "../path/to/source_module"
}
}
Make sure to adjust the backend
configuration to match your actual setup. In a production environment, you'd typically use a remote backend for better state management.
- Access the exported data from the source module using the
data
block:
resource "some_resource" "example" {
some_attribute = data.terraform_remote_state.source_module.outputs.exported_data
}
Here, some_attribute
in the some_resource
will receive the value of exported_data
from the source module.
- Run
terraform apply
in the calling module to create or update resources, including the one that uses the exported data.
By following these steps, you can effectively export data from one Terraform module and import it into another, allowing for modular and organized infrastructure management.
Happy Learning :)
If you find my blog valuable, I invite you to like, share, and join the discussion. Your feedback is immensely cherished as it fuels continuous improvement. Let's embark on this transformative DevOps adventure together! ๐ #devops #90daysofdevop #AWS
Subscribe to my newsletter
Read articles from Nilesh Sahare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
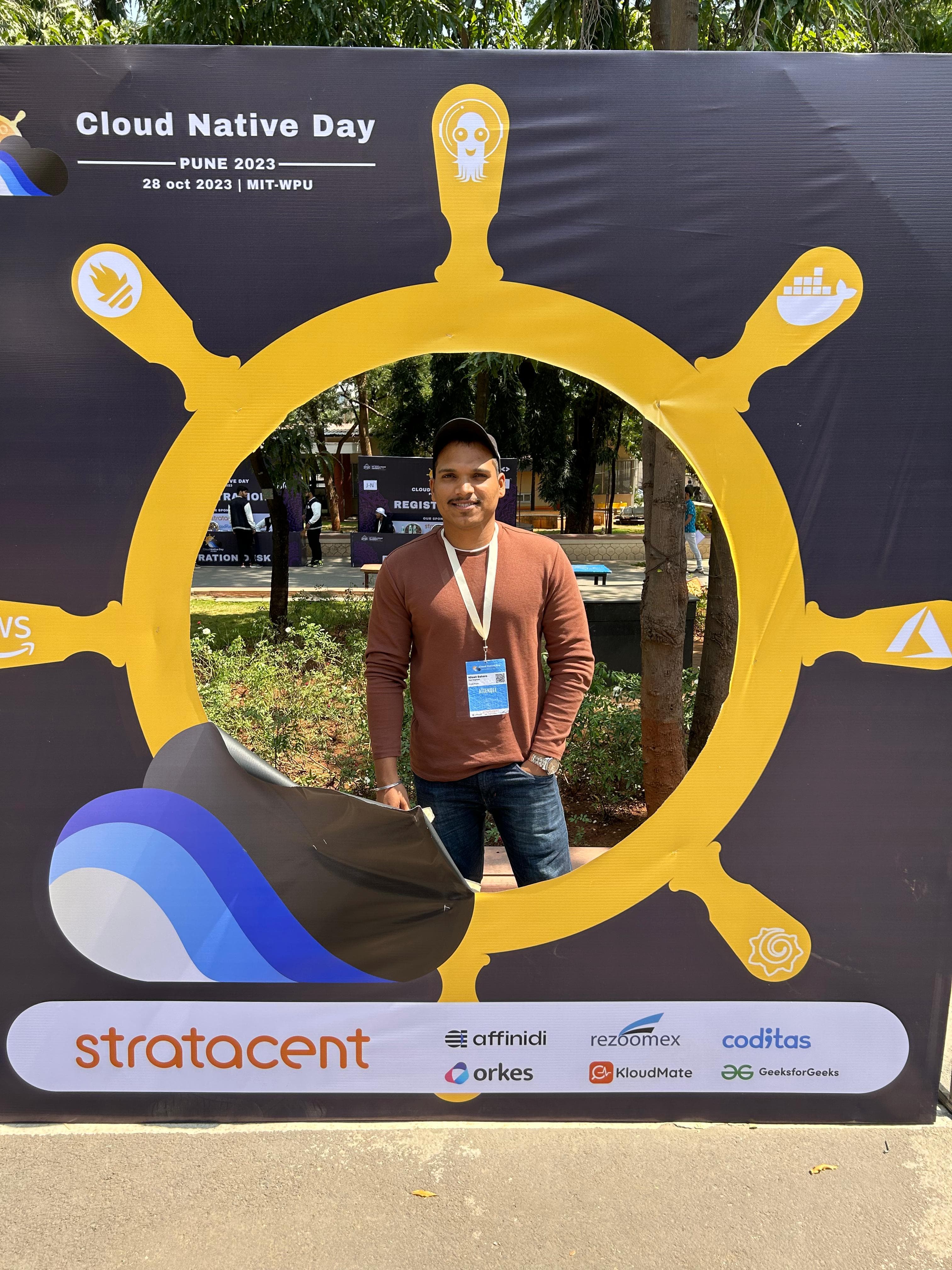
Nilesh Sahare
Nilesh Sahare
๐ I hold a Post Graduate Diploma in Advanced Computing, which has equipped me with a strong foundation in cutting-edge computing technologies like C++ Programming (VS Code editor), MySQL Database (MySQL 8 on both Command Line client and Workbench), Data Structures and Algorithms using Java (Eclipse IDE), HTML 5, CSS 3, JavaScript (VS Code editor), MERN (VS Code editor), Ms.Net Framework with C# Programming (Visual Studio .Net 2019), Software Development Methodologies (SDLC, STLC, JIRA, POSTMAN), JDBC , Servlet, Hibernate, Spring Boot, JSP (J2EE, STS IDE) LINUX Operating system and shell scripting. My academic journey has been marked by a thirst for knowledge and a passion for solving complex problems. ๐ Current Role: Test Engineer | 13 Months and Counting ๐งช In my current role as a Test Engineer, I thrive on the challenges of ensuring software quality and reliability. Automation and Manual Testing Tools: Selenium, Jira, TestRail, Postman, JUnit, . ๐ ๏ธ Learning the DevOps Way: Building Bridges ๐ My journey doesn't stop here. I'm on a continuous learning path, actively acquiring knowledge and hands-on experience with DevOps tools streamline collaboration between development and operations teams, encompassing popular solutions like Jenkins for continuous integration, Docker for containerization, Kubernetes for orchestration, Terraform for infrastructure as code, and Git for version control. ๐ค Let's Connect and Collaborate! ๐