In Pursuit of Model Excellence: The Ultimate Guide to Hyperparameter Tuning
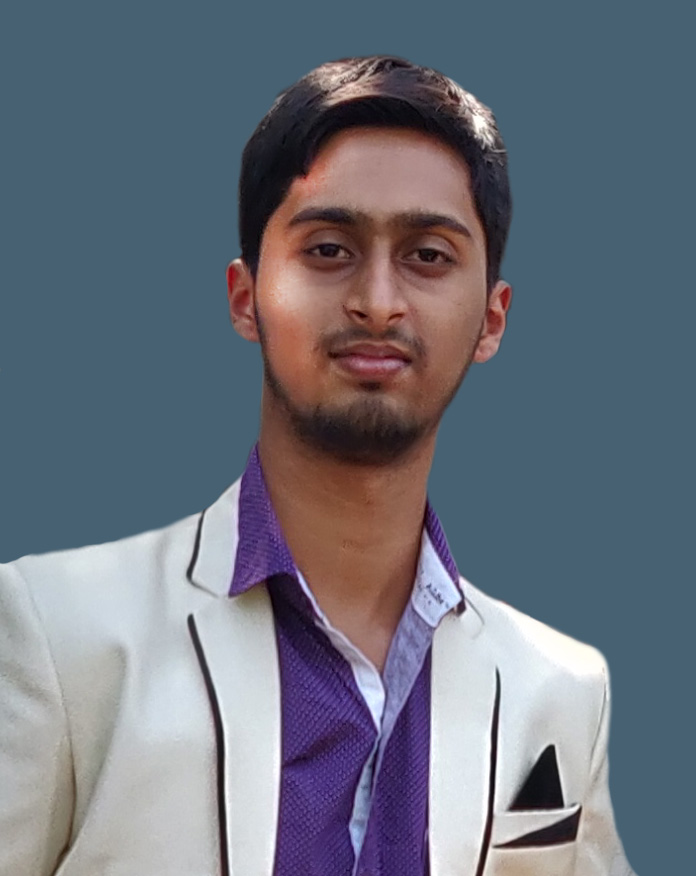
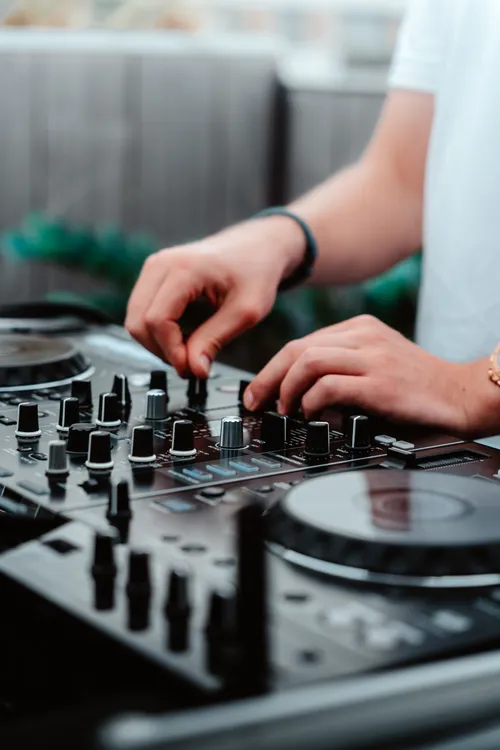
Introduction:
Hyperparameter tuning plays a pivotal role in optimizing machine learning models for better performance. The selection of appropriate hyperparameters significantly influences a model's ability to generalize to new, unseen data. In this blog, we'll explore various hyperparameter tuning techniques, each with its unique approach. Let's dive in!
Why Hyperparameter Tuning?
Machine learning models have hyperparameters, which are external configuration settings that are not learned from the data. Optimizing these hyperparameters is essential for achieving the best model performance. Here are some reasons why hyperparameter tuning is crucial:
Enhanced Model Performance: Fine-tuning hyperparameters can significantly boost a model's accuracy and generalization capabilities.
Avoiding Overfitting: Proper tuning helps prevent overfitting or underfitting, leading to a more balanced and robust model.
Improved Resource Utilization: Optimized hyperparameters often result in models that require fewer computational resources.
Hyperparameter Tuning Techniques:
1. GridSearchCV:
Explanation: GridSearchCV exhaustively searches through a specified hyperparameter grid, evaluating the model's performance for each combination.
Python Code:
from sklearn.model_selection import GridSearchCV from sklearn.ensemble import RandomForestClassifier # Define hyperparameter grid param_grid = { 'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20], 'min_samples_split': [2, 5, 10], } # Instantiate RandomForestClassifier clf = RandomForestClassifier() # Use GridSearchCV grid_search = GridSearchCV(clf, param_grid, cv=5) grid_search.fit(X, y)
2. RandomizedSearchCV:
Explanation: RandomizedSearchCV randomly samples from a specified hyperparameter space, providing a more computationally efficient alternative to GridSearchCV.
Python Code:
from sklearn.model_selection import RandomizedSearchCV from scipy.stats import randint from sklearn.ensemble import RandomForestClassifier # Define hyperparameter distributions param_dist = { 'n_estimators': randint(50, 200), 'max_depth': [None, 10, 20], 'min_samples_split': randint(2, 10), } # Instantiate RandomForestClassifier clf = RandomForestClassifier() # Use RandomizedSearchCV random_search = RandomizedSearchCV(clf, param_distributions=param_dist, n_iter=10, cv=5) random_search.fit(X, y)
3. Bayesian Optimization using HyperOpt:
Explanation: HyperOpt uses Bayesian optimization to efficiently explore the hyperparameter space based on the model's performance. This method is far better than the random search CV and grid search CV. This technique can be broken down into 3 important components: 1) Objective function: Defines model and calculates the metrics specified. 2) Space: stores all the possible parameter values 3) Optimization algorithm: specify which algorithm to use to improve the metrics for our problem
Python Code:
from hyperopt import hp, fmin, tpe from sklearn.model_selection import cross_val_score from sklearn.ensemble import RandomForestClassifier # Define objective function def objective(params): clf = RandomForestClassifier(**params) return -cross_val_score(clf, X, y, cv=5, scoring='accuracy').mean() # Define hyperparameter space space = { 'n_estimators': hp.choice('n_estimators', range(50, 200)), 'max_depth': hp.choice('max_depth', [None, 10, 20]), 'min_samples_split': hp.choice('min_samples_split', range(2, 10)), } # Perform Bayesian Optimization best = fmin(fn=objective, space=space, algo=tpe.suggest, max_evals=10)
4. Sequential Model-based Optimization:
Explanation: A more advanced optimization technique that models the objective function to determine the next set of hyperparameters to evaluate.
Python Code:
- This technique often involves the use of specialized libraries like sci-kit-optimize, which provide Bayesian optimization methods.
5. Optuna:
Explanation: Optuna is a hyperparameter optimization framework that employs a tree-structured Parzen Estimator algorithm. The study stands for space for training. The objective function is the core part of this Optuna in which we pass the trial object.
Python Code:
import optuna from sklearn.model_selection import cross_val_score from sklearn.ensemble import RandomForestClassifier # Define objective function def objective(trial): clf = RandomForestClassifier( n_estimators=trial.suggest_int('n_estimators', 50, 200), max_depth=trial.suggest_categorical('max_depth', [None, 10, 20]), min_samples_split=trial.suggest_int('min_samples_split', 2, 10), ) return -cross_val_score(clf, X, y, cv=5, scoring='accuracy').mean() # Perform Optuna Optimization study = optuna.create_study(direction='maximize') study.optimize(objective, n_trials=10)
6. Genetic Algorithms:
- Explanation: Genetic algorithms use principles inspired by natural selection to evolve a population of hyperparameter sets over multiple generations. For example, if we are considering 50 models initially we will only select the top 25 best-performing models and then we will create offspring based on these models having good accuracies
Conclusion:
Hyperparameter tuning is a critical step in the machine learning pipeline, ensuring that models perform optimally. The techniques discussed, along with their Python implementations, offer a range of approaches suitable for various scenarios. As the field of hyperparameter optimization continues to evolve, staying informed about these techniques will empower data scientists to build more accurate and efficient models.
Subscribe to my newsletter
Read articles from Saurabh Naik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
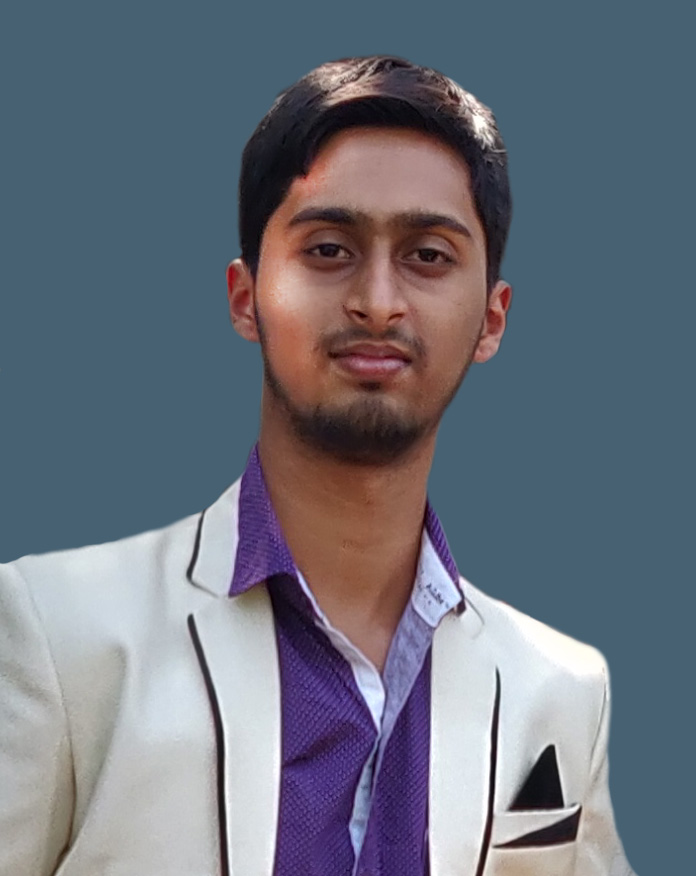
Saurabh Naik
Saurabh Naik
๐ Passionate Data Enthusiast and Problem Solver ๐ค ๐ Education: Bachelor's in Engineering (Information Technology), Vidyalankar Institute of Technology, Mumbai (2021) ๐จโ๐ป Professional Experience: Over 2 years in startups and MNCs, honing skills in Data Science, Data Engineering, and problem-solving. Worked with cutting-edge technologies and libraries: Keras, PyTorch, sci-kit learn, DVC, MLflow, OpenAI, Hugging Face, Tensorflow. Proficient in SQL and NoSQL databases: MySQL, Postgres, Cassandra. ๐ Skills Highlights: Data Science: Statistics, Machine Learning, Deep Learning, NLP, Generative AI, Data Analysis, MLOps. Tools & Technologies: Python (modular coding), Git & GitHub, Data Pipelining & Analysis, AWS (Lambda, SQS, Sagemaker, CodePipeline, EC2, ECR, API Gateway), Apache Airflow. Flask, Django and streamlit web frameworks for python. Soft Skills: Critical Thinking, Analytical Problem-solving, Communication, English Proficiency. ๐ก Initiatives: Passionate about community engagement; sharing knowledge through accessible technical blogs and linkedin posts. Completed Data Scientist internships at WebEmps and iNeuron Intelligence Pvt Ltd and Ungray Pvt Ltd. successfully. ๐ Next Chapter: Pursuing a career in Data Science, with a keen interest in broadening horizons through international opportunities. Currently relocating to Australia, eligible for relevant work visas & residence, working with a licensed immigration adviser and actively exploring new opportunities & interviews. ๐ Let's Connect! Open to collaborations, discussions, and the exciting challenges that data-driven opportunities bring. Reach out for a conversation on Data Science, technology, or potential collaborations! Email: naiksaurabhd@gmail.com