[ T.E ] CN16 - DNS Lookup
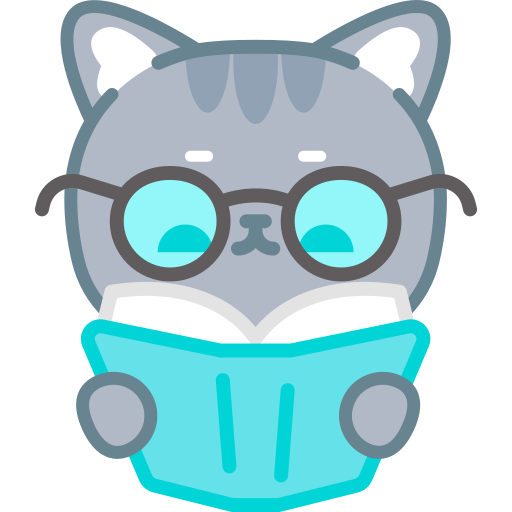
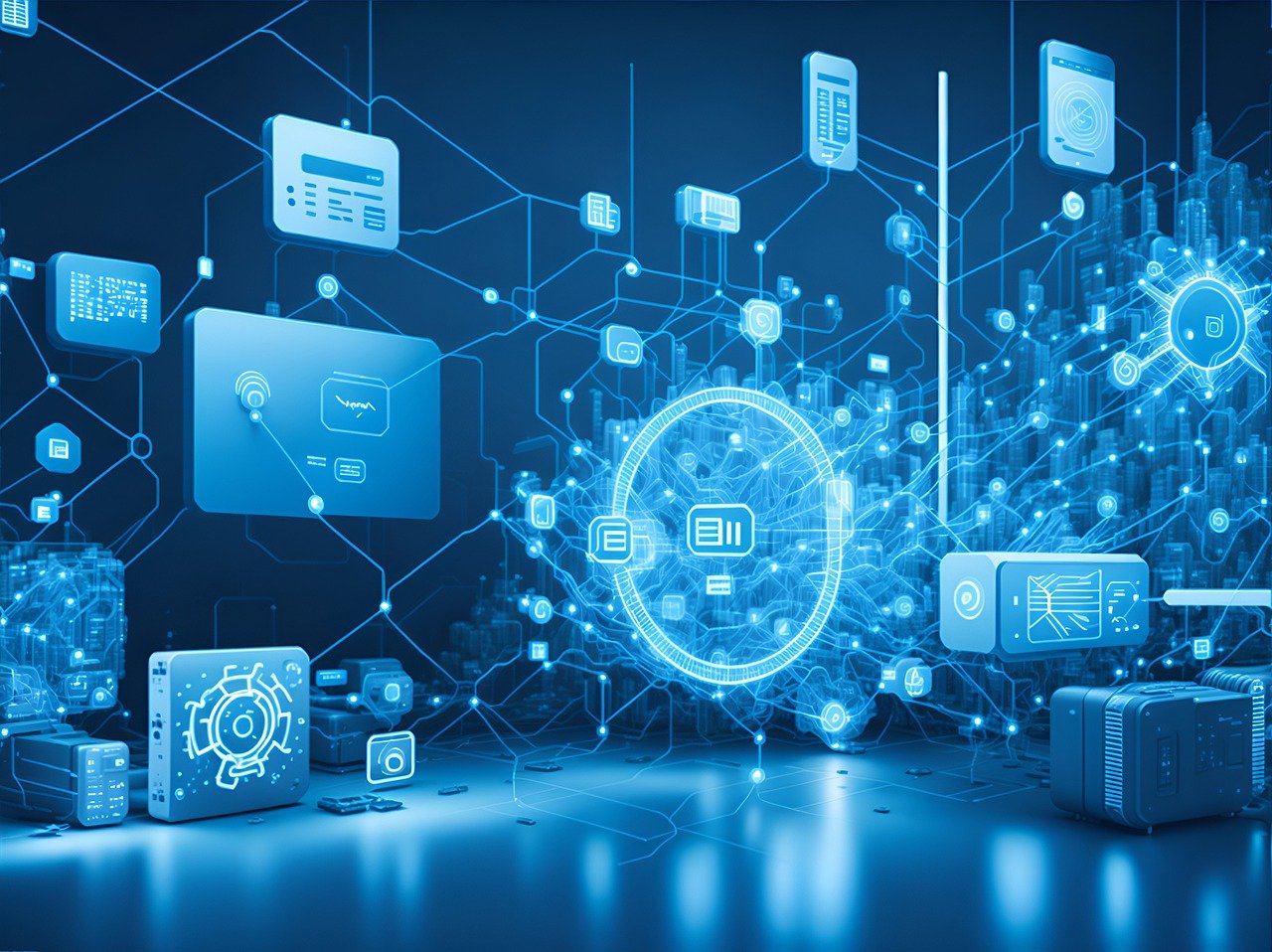
Problem Statement
Write a program for DNS lookup. Given an IP address input, it should return the URL and vice versa.
What is DNS?
DNS stands for Domain Name System. DNS is the system that translates the human-friendly domain name into the actual IP address of the server where the website is hosted.
It comes under the Application Layer.
DNS is a process of translating a human-readable domain name into a computer-readable IP address, allowing us to navigate the internet using easy-to-remember names instead of numerical addresses.
How DNS works?
DNS Query -
- A user types a website address [ name ] into the web browser.
DNS Query Initiation -
- The computer needs to find the IP address associated with the name, so it sends the DNS Query to a DNS server
DNS Server Lookup -
- A DNS Server is like a big directory that knows the IP addresses associated with various domain names. It looks up the IP address for the name provided.
IP Address Retrieval -
- The DNS Server finds and sends the associated IP address back to the computer.
Accessing the Website -
- With the IP address computer can connect to the web server.
CODE
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netdb.h>
#include <arpa/inet.h>
int main() {
struct hostent *host_info;
struct in_addr *address;
char input[50];
int choice;
// Asking the user for their choice
printf("Choose an option:\n");
printf("1. Enter a domain name\n");
printf("2. Enter an IP address\n");
printf("Enter your choice (1 or 2): ");
scanf("%d", &choice);
// Checking the user's choice
if (choice == 1) {
// User wants to input a domain name
printf("Enter the domain name: ");
scanf("%s", input);
printf("Will do a DNS query on: %s\n", input);
host_info = gethostbyname(input);
if (host_info != NULL) {
address = (struct in_addr *)(host_info->h_addr);
printf("%s has address %s\n", input, inet_ntoa(*address));
} else {
herror("Error getting host information");
}
} else if (choice == 2) {
// User wants to input an IP address
printf("Enter the IP address: ");
scanf("%s", input);
struct in_addr addr;
inet_pton(AF_INET, input, &addr);
host_info = gethostbyaddr(&addr, sizeof(struct in_addr), AF_INET);
if (host_info != NULL) {
printf("%s has domain name %s\n", input, host_info->h_name);
} else {
herror("Error getting host information");
}
} else {
printf("Invalid choice. Please enter 1 or 2.\n");
}
return 0;
}
/*
Commands to run the code on Linux -
gcc dns.c
./a.out
*/
Code with line-by-line explanation
// Header Files
// Used for socket programming, providing socket-related functions [ socket(), bind(), listen() ]
#include<sys/socket.h>
// Contains structures and constants for internet address families, particulary for IPv4 and IPv6
#include<netinet/in.h>
// Includes functions and structures for DNS, allowing hostname to IP add convo
#include<netdb.h>
// Provides functions for manipulating IP add, such as converting binary IP add to human-readable strings and vice versa
#include<arpa/inet.h>
// Standard Input-Output functions
#include<stdio.h>
// String Manipulation
#include<string.h>
// Standard library functions, used for memory allocation and deallocation
#include<stdlib.h>
int main(){
// - hostent structure is used to store info about a host, including its name and associated IP addresses
struct hostent *host_info;
// - in_addr structure represents an IPv4 address
struct in_addr *address;
// The above 2 pointers are used for storing info obtained from a DNS query, where
// host_info would contain info about a host
// address would store its IP address
// To store domainName or IP address provided by the user
char input[50];
int choice;
// Asking the user for their choice
printf("Choose an Option : \n");
printf("1. Enter a Domain Name \n");
printf("2. Enter an IP address \n");
// Getting the users input
scanf("%d",&choice);
// Acting according to the users choice
if(choice == 1){
printf("Enter the Domain Name : ");
scanf("%s",input);
printf("Will do a DNS query on : %s \n",input);
/*
- gethostbyname() : Is used to perform a DNS query* and retrieve info about the host [ whos websiteName was provided by the user ]
- The retrieve info of the host is stored in the hostent structure and host_info is the pointer to that hostent structure
*/
host_info = gethostbyname(input);
if(host_info != NULL){
/*
- Performs a typecast operation
- host_info-h_addr contains the pointer to the first IP address returned by the gethostbyname function
- Tht pointer is of type struct hostent, which is typecasted into struct in_addr and is stored in the variable address
*/
address = (struct in_addr *)(host_info->h_addr);
/*
- Prints the result of DNS query
- inet_ntoa() function converts the IP address from its binary representation [ stored in address ] to a human-readable string [ eg. 192.168.10.1 ]
*/
printf("%s has address %s \n",input,inet_ntoa(*address));
}
else{
// Used to print an error message
herror("Error getting the host information ");
}
}
else if(choice == 2){
printf("Enter the IP Address : ")
// Getting the IP address from the user in string format
scanf("%s",input);
// structure that represents [ can store ] IPv4 Address
struct in_addr addr;
/*
- inet_pton()
- Used to convert the IP address in string format to its numeric format and store it in a specified memory location
- AF_INET
- This specifies that we are working with IPv4 addresses. It stands for Address Family - Internet Protocol version 4.
- input : contains the IP address in string format
- addr : the numeric form of IP address will be stored in addr
*/
inet_pton(AF_INET, input, &addr);
/*
- gethostbyaddr :
- Function used to retrieve host information associated with the provided IPv4 address.
- addr
- A pointer to the binary representation of the address.
- sizeof(struct in_addr)
- The length of the address.
- AF_INET
- The address family type, AF_INET for IPv4.
*/
host_info = gethostbyaddr(&addr, sizeof(struct in_addr), AF_INET);
if(host_info != NULL){
// Prints the respective domain name
printf("%s has domain name %s \n",input,host_info->h_name);
}
else{
herror("Error getting host information ");
}
}
else{
printf("Invalid Choice !!");
}
return 0;
}
Output
Using Domain Name :
Using IP address :
Subscribe to my newsletter
Read articles from DataGeek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
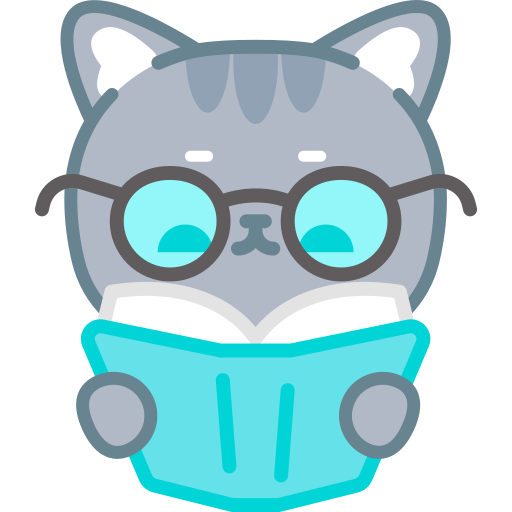
DataGeek
DataGeek
Data Enthusiast with proficiency in Python, Deep Learning, and Statistics, eager to make a meaningful impact through contributions. Currently working as a R&D Intern at PTC. I’m Passionate, Energetic, and Geeky individual whose desire to learn is endless.