Palindrome or not

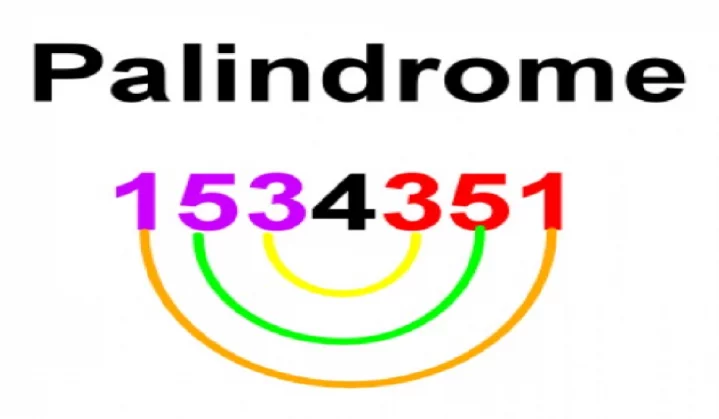
A palindrome is a word derived from the Greek meaning "running back again".
A string is called a palindrome string if the reverse of that string is the same as the original string. Example lol, madam, level.
Eg: original string = mom
after reversing the original string = mom
original == reversed string then the string is palindrome.
1. Check palindrome string
For reversing the string you can refer to my blog (I used 3 different approaches for reversing the string with an explanation).
public class Palindrome {
public static void main(String[] args) {
String str = "madam";
String reverseStr="";
String s = str.toLowerCase();
System.out.println("Original:"+s);
for(int i=s.length()-1;i>=0;i--){
reverseStr += s.charAt(i);
}
System.out.println("Reversed:"+str);
if(s. equals(reverseStr)){
System.out.println(s + " is Palindrome.");
}
else{
System.out.println(s + " is not Palindrome.");
}
}
}
Original:madam
Reversed:madam
madam is Palindrome.
check palindrome string without reversing the string
public class Palindrome {
public static void main(String[] args) {
String str = "madam";
String s = str.toLowerCase();
int start = 0, end = s.length()-1;
while(start < end){
if(s.charAt(start)!=s.charAt(end)){
System.out.println(s + " is not Palindrome.");
System.exit(0);
}
start++;
end--;
}
System.out.println(s + " is Palindrome.");
}
}
madam is Palindrome.
Take the example "Madam"
start =0, end = 5-1 = 4
0 1 2 3 4 <==>m a d a m
check the first and last index of the string
(m == m) => then increment the start and decrease the end
start++ => start = 1
end-- => end = 4-1=3
check until the condition is false(start< end)
if start!=end then the print string is not a palindrome and exits from the program.
2. Check the palindrome number
check palindrome number using string
Convert the number to a string.
String.valueOf(number) => It will convert the number to a string and then follow the same process.
public class Palindrome {
public static void main(String[] args) {
int number = 121;
String string = String.valueOf(number);
String s = string.toLowerCase();
int start = 0, end = s.length()-1;
while(start < end){
if(s.charAt(start)!=s.charAt(end)){
System.out.println(number + " is not Palindrome.");
System.exit(0);
}
start++;
end--;
}
System.out.println(number + " is Palindrome.");
}
}
121 is Palindrome.
check palindrome number without converting to string
public class Palindrome {
public static void main(String[] args) {
int num = 353, reversedNum = 0, rem;
int originalNum = num;
while (num != 0) {
rem = num % 10;
reversedNum = reversedNum * 10 + rem;
num /= 10;
}
if (originalNum == reversedNum) {
System.out.println(originalNum + " is Palindrome.");
}
else {
System.out.println(originalNum + " is not Palindrome.");
}
}
}
353 is Palindrome.
Take number = 353 and store it in originalNum because in the process the number value will change.
reversedNum=0, rem
while loop
num = 353 => rem = num%10 (It will give the reminder of the number)
rem = 3
reversedNum = reversedNum 10 + rem (0*10 +3 = 3)
reversedNum = 3
num = num/10 (num = 353/10 = 35)
num = 35 => rem = num%10 (It will give the reminder of the number)
rem = 5
reversedNum = reversedNum * 10 + rem (3*10 +5 = 35)
reversedNum = 35
num = num/10 (num = 35/10 = 3)
num = 3 => rem = num%10 (It will give the reminder of the number)
rem = 3
reversedNum = reversedNum * 10 + rem (35*10 +3 = 353)
reversedNum = 353
num = num/10 (num = 3/10 = 0)
while(num!=0) condition false
then check the originalNum and reversedNum then print the output.
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
