Python File Handling: A Beginners Guide

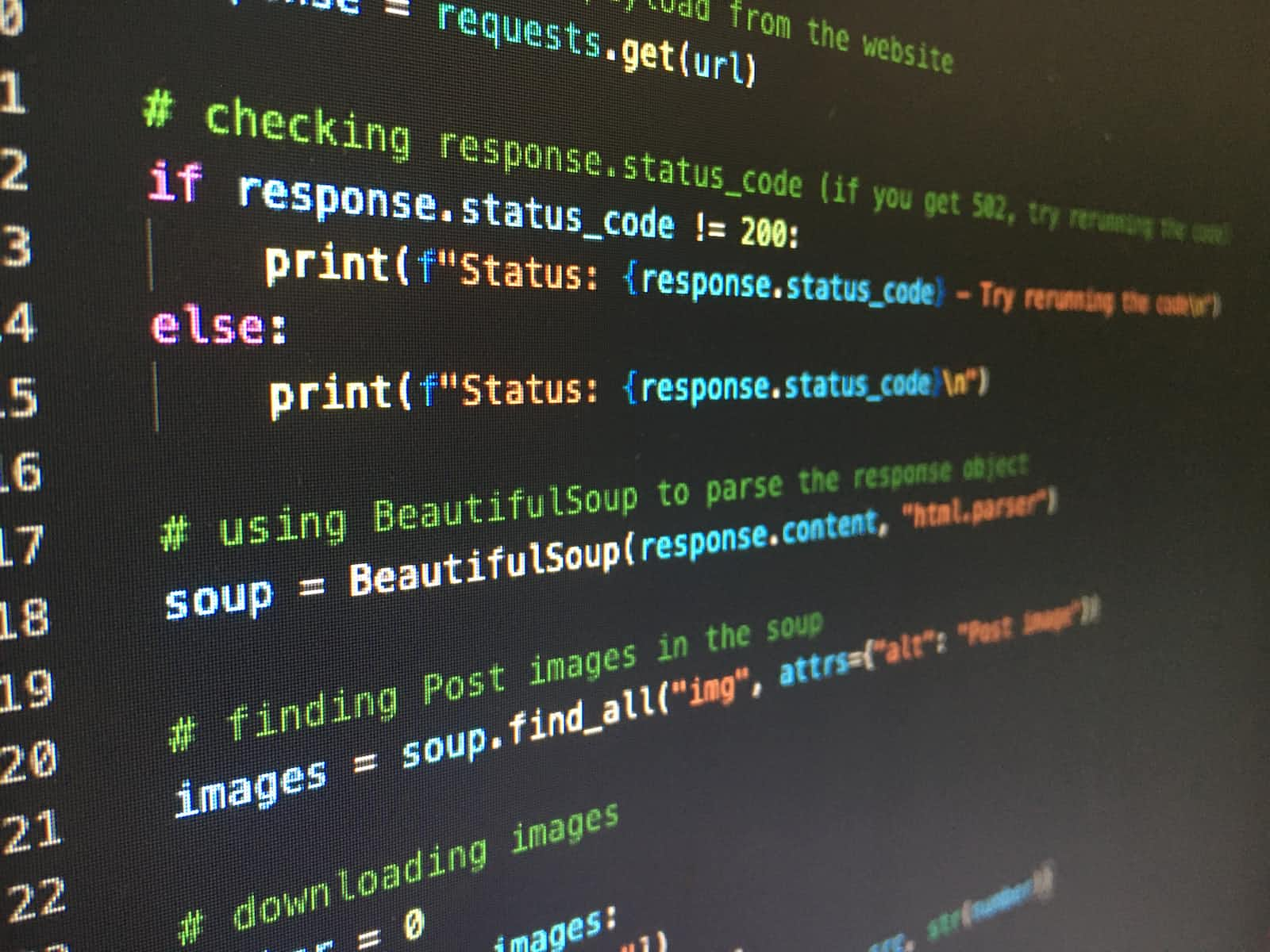
File handling is a fundamental aspect of programming that allows developers to interact with external data. In Python, file handling is made straightforward yet robust, offering a plethora of functionalities to work with various file types.
In this guide, we'll explore the basics of file handling, covering everything from opening and closing files to dealing with different file formats like text files, JSON, and binary files. Let's dive in!
Understanding Files
What is a File?
A file is a collection of data stored on a computer under a specific name and directory. Files come in various types, such as text files, images, videos, and more.
In Python, the built-in open()
function is used to interact with files, allowing you to perform operations like reading, writing, and appending.
Opening and Closing Files
To interact with a file, you must first open it using the open()
function. This function takes two arguments, the file name and the mode, and a keyword argument.
file = open('example.txt', 'r', encoding="utf-8")
The first argument is a string containing the name of the file. The second argument is also a string containing a couple of characters that describe how the file will be used (mode).
Here are different modes you can use to interact with the file:
"r" -> This mode is used to only read into the file.
"w" -> This mode is used to write into a file. If there is an existing file with the same filename then the contents will be overwritten.
"a" -> This mode is used to append to a file. Any data written with this mode will be automatically added at the end of the file.
"r+" -> This mode is used to read and write into a file.
"b" -> This opens files in binary mode
"x" -> opens for exclusive creation, failing if the file already exists
If no mode argument has been specified then "r" will be assumed
The last argument (keyword argument) is the encoding argument. This determines how the bytes in the file are translated into characters, ensuring proper interpretation of the text. In the above example we used encoding="utf-8"
, which is widely used and can represent most characters in the Unicode standard. It is always good practice to specify the encoding otherwise the default is platform dependent.
Always remember to close the file using the close()
method to free up system resources.
file = open("filename", "r", encoding="utf-8")
# Performing operations on the file
# Closing the file
file.close()
Using the with
statement
There is an advantage to using the with
statement when handling file objects. It ensures that the file is automatically closed when you are done, even if an exception is raised at some point.
with open('example.txt', 'r') as file:
file_data = file.read()
# File is automatically closed outside the 'with' block
Reading Files
Reading files is a common operation, and Python provides several methods for doing so.
The
read()
method reads the entire filereadline()
reads one line at a time. If a function returns an empty string ' ' then then the end of file has been reached. A blank line is represented by the '\n' characterreadlines()
returns a list of lines.
with open('example.txt', 'r') as file:
content = file.read() # Read the entire file
line = file.readline() # Read one line
lines = file.readlines() # Read all lines into a list
Let us assume that our example.txt has the following content:
Hello, World!
File handling is fun
You'll get a hang of it!
- If we print out content then our output will be:
Hello, World!
File handling is fun
You'll get a hang of it!
- If we print out line then the output will be:
Hello, World!
- if we print out lines then the output will be:
'Hello, Worls!, 'File handling is fun', 'You'll get a hang of it!'
Writing and Appending to Files
To write to a file, open it in write mode ('w'). Be cautious, as this will overwrite the existing content. To append content, use append mode ('a').
with open('example.txt', 'w') as file:
file.write('Hello, World!')
with open('example.txt', 'a') as file:
file.write('\nAppending to the file.')
After execution of this file, the content in the example.txt file will be:
Hello, World!
Appending to the file.
This is because 'Hello, World!' is written within the file since the mode 'w' is used. 'Appending to the file' is then appended on a new line as specified with the '\n' character.
If the type object is not either a string (for text mode) or a bytes object (for binary mode) then they need to first be converted before writing them.
Working with Different File Types
Text Files
Text files are the simplest form of files, containing plain text. Python makes it easy to work with them using the methods mentioned above.
JSON Files
JSON (JavaScript Object Notation) is a lightweight data interchange format. Python's json
module allows easy serialization and deserialization of JSON data.
Serialization (Python to JSON)
Serialization is the process of converting a Python object into a JSON-formatted string. This is useful when you want to store or transmit data in a format that can be easily read by other programs. The json
module provides the dump()
and dumps()
functions for serialization.
The dump()
function writes a Python object to a file-like object (such as a file or a network socket). Here's an example:
import json
data = {'Name': 'Jane', 'Country': 'Kenya', 'Occupation': 'Software Engineer'}
# Serialize and write to a file
with open('data.json', 'w') as json_file:
json.dump(data, json_file)
This will create a new file 'data.json' with these content:
{"Name": "Jane", "Country": "Kenya", "Occupation": "Software Engineer"}
The dumps()
function, on the other hand, returns a JSON-formatted string without writing it to a file. This is useful when you want to transmit the JSON data over a network or include it in a web response.
import json
# Python object to be serialized
data = {'name': 'John', 'age': 30, 'city': 'New York'}
# Serialize to a JSON-formatted string
json_string = json.dumps(data)
print(json_string)
This will be the output:
{"Name": "Jane", "Country": "Kenya", "Occupation": "Software Engineer"}
Binary File Formats
In addition to text-based formats, Python supports working with binary files. When working with images, audio, or other non-text data, use binary mode ('rb' for reading, 'wb' for writing).
Handling Errors
When working with files, errors can occur, such as attempting to open a non-existent file or insufficient permissions. It's crucial to handle these errors gracefully.
Conclusion
Mastering file handling in Python is a crucial skill for any developer. Whether dealing with text files, JSON or binary formats, Python provides a comprehensive set of tools to make the process efficient and effective. Always prioritize proper file handling, use the with
statement for automatic closing, and handle errors gracefully for robust and reliable file interactions. Happy coding!
Subscribe to my newsletter
Read articles from Tk Codes directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tk Codes
Tk Codes
Hey there, I'm Tracey – a passionate learner who's diving headfirst into the world of programming. As a recent enthusiast in this captivating realm, I've embarked on a thrilling journey of discovery and growth. Why do I write? Well, it's simple: I believe in the power of sharing. By putting my learning experiences into words, I not only solidify my understanding but also aim to provide a helping hand to fellow learners. Join me as I navigate the exciting seas of programming, one keystroke at a time. Let's learn out loud together!