Functions in JavaScript

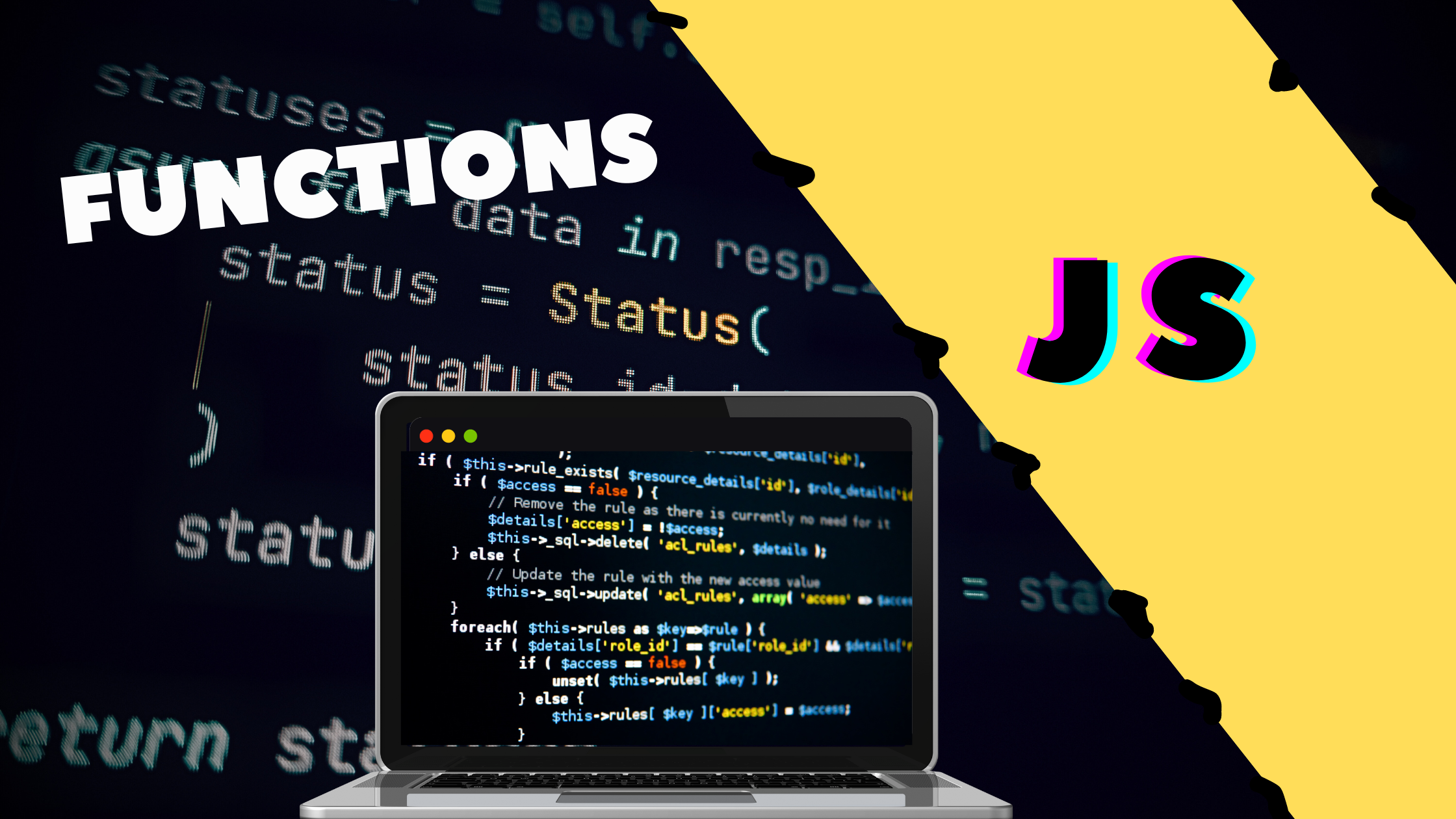
Note: Try to code by yourself and understand the output
Introduction
Functions in JavaScript are blocks of code that can be used to perform a specific task.
Functions can be used to simplify code, make it more reusable, and improve readability.
To create a function in JavaScript, you can use the function keyword.
To perform some common or repeated tasks, we used functions.
Instead of writing code again and again, call the defined functions many times.
Example:-
// * Function Basics in JavaScript *
// 1.Every function begins with function keyword.
// 2.In JavaScript, function can be assign as variable value.
// 3.Syntax:
// function funcName(){
// }
// Function Definition or Function Declaration
function sayMyName() {
console.log("John Doe");
}
console.log(sayMyName); // This is just a Reference of a function
// console.log(sayMyName());
sayMyName(); // calling function
// <=======######=======>
Understand function with parameters
// functions with parameters
function addNumbers(num1, num2) {
// NOTE => Before using parameters always remember to check parameter
// datatype
console.log(num1 + num2); // without returning
// let result = num1 + num2;
// return result; // return result
// OR
return num1 + num2; // Direct return
}
const result = addNumbers(5, 5); // output: 10
// console.log("Result: ", result); // output: Result: undefined (without returning)
// why undefined in above console
// => Output is 'undefined' because we are not returning the output,
// we just printing the output'
// Solution for 'undefined'
// We need to use return statement before printing the value
console.log(result); // output: 10
// <=======######=======>
function greetings(username) {
return `Hello ${username}, Nice to meet you`;
}
greetings(); //output: <= Blank because func returning something and we are just calling function not printing
console.log(greetings()); //output: Hello undefined, Nice to meet you
// output is 'undefined' because we are passing empty argument in the function
console.log(greetings("Jack")); // output: Hello Jack, Nice to meet you
// <=======######=======>
function greetings2(username) {
if (!username) {
console.log("Please enter a username");
return;
}
return `Hello ${username}, Nice to meet you`;
}
greetings2(); //output: Please enter a username
console.log(greetings2());
// Expected output: Please enter a username
// undefined <= why undefined because we are passing nothing, so there is nothing to print or returning undefined
console.log(greetings2("Conway")); // output: Hello Conway, Nice to meet you
// Print Default Value
function greet(username = "User") {
return `Hello ${username}, Nice to meet you`;
}
console.log(greet());
console.log(greet("Steve")); //output: Hello Steve, Nice to meet you <= overwrite the value
// <=======######=======>
// Rest Operator (...parameter)
// Que : How to pass multiple arguments in function with only 1 parameter
// OR
// Convert multiple arguments passed as a 1 parameter into array
function calculateTotalCartPrice(val1, val2, ...num) {
// ...parameter <= rest operator
return num;
}
// console.log(calculateTotalCartPrice(100, 200, 300, 400)); // output: 100 (without rest operator)
// console.log(calculateTotalCartPrice(100, 200, 300, 400)); // output: [ 100, 200, 300, 400 ]
console.log(calculateTotalCartPrice(100, 200, 300, 400)); // output: [300, 400] (with val1, val2)
// val1 = 100, val2 = 200, ...num = [300, 400]
// <=======######=======>
Pass Object as a Parameter
// Pass Object as a parameter
const user = {
username: "MS Dhoni",
age: "42",
};
function handleObject(anyobject) {
console.log(`Username is ${anyobject.username} and age is ${anyobject.age}`);
}
handleObject(user);
//pass object directly
handleObject({
username: "John Doe",
age: "25",
});
// <=======######=======>
Pass Array as a Parameter
// Pass Array as a parameter
const arr = [100, 200, 300, 400];
function returnSecondVaue(getArray){
return getArray[1];
}
console.log(returnSecondVaue(arr)); // output: 200
console.log(returnSecondVaue([400, 500, 600, 700])); // output: 500
// <=======######=======>
'this' Keyword in JavaScript (Important Topic)
Note: Information about the 'this' keyword is limited in this blog(check out the references at the end).
// NOTE: Information about 'this' keyword is limited in this blog
// 'this' Keyword in JS
// => 'this' keyword always holds a reference to a current context
const user = {
username: "John",
price: 900,
welcomeMessage: function () {
console.log(`Welcome ${this.username}!`);
console.log(this); // shows current context
}
}
user.welcomeMessage();
user.username = "Jinny";
user.welcomeMessage();
// console.log(this); // {} empty object (shows current context)
// 'this' keyword in NodeJS acts as a Empty Object.
// 'this' keyword in Browser Console acts as a Windows Object.
// <=======######=======>
Three ways of writing functions in JavaScript
Function Declaration
Function Expression
Arrow Function
Function Declaration:
Declare a function with a 'function' keyword.
It must have a function name.
Example:-
//Syntax
//function declaration or function definition
//function funName(){
//
//}
//call function
//funName();
// Regular Function
function hello() {
let username = "John Doe";
console.log(`Hello, ${this.username}`);
}
// hello(); //output: Hello, undefined
// Function Declaration or Regular Function
function Person(name) {
this.name = name;
}
const john = new Person("John Doe")
// console.log(john.name); //output: John Doe
// <=======######=======>
Function Expression:
A function expression is a JavaScript function that is created and assigned to a variable in a single statement.
Function expressions are often used to create anonymous functions, which are functions that do not have a name.
Example:-
//Syntax
//function declaration or function definition
//let varName = function(){
//
//}
// Function Expression: (Take function in variable)
const hello = function () {
let username = "John Doe";
console.log(`Hello, ${this.username}`);
}
// hello(); //output: Hello, undefined
// <=======######=======>
Arrow Function:
Arrow functions are a new feature in JavaScript that was introduced in ES6.
A more concise way to write anonymous functions.
Arrow functions are typically used for one-liners or for functions that are only used within a single scope.
They are also useful for creating callback functions.
Example:-
//Syntax
//function declaration or function definition
//let function_name = (argument1, argument2 ,..) => expression
// Understand Arrow Functions
// Basic Arrow Function
const hello = () => {
let username = "John Doe";
console.log(`Hello, ${this.username}`);
}
// hello(); // Hello, undefined
const add = (num1, num2) => {
return num1 + num2;
}
// console.log(5, 5); //output: 10
// <=======######=======>
// Different way to use arrow function
// Implicit Function (No use of return keyword)
// Explicit Function (Use of return keyword)
// 1 way
const addTwo = (num1, num2) => num1 + num2; // As function is one linear so no need to return
// console.log(addTwo(5, 5)); //output: 10
// 2 way
const addThree = (num1, num2, num3) => (num1 + num2 + num3);
// console.log(addThree(5, 5, 5)); //output: 15
//Advantage of () paranthesis
const objFunc = () => ({ username: "John Doe" }); // to use object inside function
// console.log(objFunc());
// <=======######=======>
Immediately Invoked Function Expression (IIFE)
Function to execute immediately.
Sometimes global scope gives problems in function scope so to remove global scope variables, declaration, or pollution we use IIFE.
// IMMEDIATELY INVOKED FUNCTION EXPRESSIONS (IIFE)
// 1. Function to execute immediately
// 2. Sometimes Global scope give problem in function scope so to remove
// global scope variable, declaration or pollution we use IIFE.
//syntax
// (function definition)(function call)
(function DBstatus() { // Named IIFE
console.log(`DB Connected`);
})(); // ';' SEMICOLON is very important to stop function execution else it won't run and will give error
(() => { //Unnamed IIFE OR Simple IIFE
console.log(`DB Connected Successfully`);
})();
// pass argument
((name) => {
console.log(`Hii, ${name}`);
})('Binod')
//(function definition)(function execution)
//function definition
//function random = () => {
// console.log(`Hii, ${}`);
// }
//function execution
//random("Binod")
// <=======######=======>
Subscribe to my newsletter
Read articles from Pranay Sanjule directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranay Sanjule
Pranay Sanjule
Learner, Tech-Enthusiast