Factorial of a number

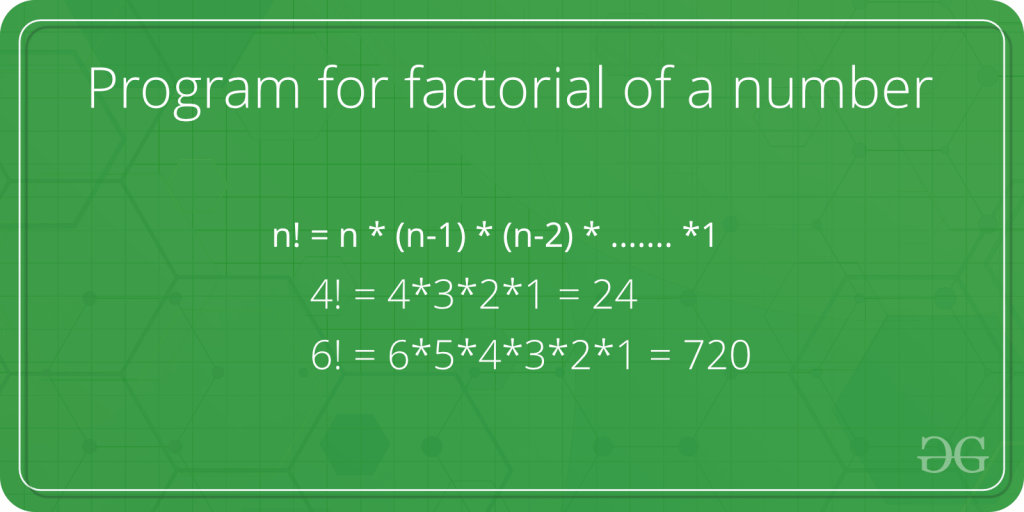
Factorial of a non-negative number is the multiplication of all positive numbers that are smaller than are equal to n.
Formula: n! = n*(n-1)\(n-2)*... 2\1
n=5 => 5*4*3*2*1
n=5 => 1*2*3*4*5
factorial of 5 is 120
0! = 1
Factorial using for loop:
public class Factorial {
public static void main(String[] args) {
int n= 5, fact=1;
for(int i=1;i<=n;i++){
fact = fact * i;
}
System.out.println(n+" Factorial is "+fact);
}
}
5 Factorial is 120
In the for loop we can start the loop from 2 also because 1*n =n. so 1*2= 2.
i=1 => 1<=5 => fact = 1 * 1=1
i=2 => 2<=5 => fact = 1 * 2=2
i=3 => 3<=5 => fact = 2 * 3=6
i=4 => 4<=5 => fact = 6 * 4=24
i=5 => 5<=5 => fact = 24 * 5 = 120
output: 120
Factorial using while loop:
public class Factorial {
public static void main(String[] args) {
int n= 5, fact=1;
//It print the n value and the cursor it at the same line.
System.out.print(n);
while(n!=0){
fact = fact * n;
n--;
}
//In that same line the factorial value is also print
System.out.println(" Factorial is "+fact);
//In every iteration n value decreases
//System.out.println(n+" Factorial is "+fact);
}
}
5 Factorial is 120
For the above while loop, we can also write the code as
public class Factorial {
public static void main(String[] args) {
int n= 6, fact=1, i=1;
while(i<=n){
fact = fact * i;
i++;
}
System.out.println(n+" Factorial is "+fact);
}
}
6 Factorial is 720
Factorial using recursion
Recursion is nothing but calling the function itself again and again.
public class Factorial {
public static void main(String[] args) {
int num = 4;
System.out.println(num+" Factorial is "+fact(num));
}
public static int fact(int num){
if(num==0)
return 1;
return num * fact(num-1);
}
}
4 Factorial is 24
num = 4
4!
fact(4) = 4*fact(3) = 4*6 =24
fact(3) = 3 * fact(2) = 3*2 =6
fact(2) = 2 * fact(1) = 2*1 =2
fact(1) = 1 * fact(0) = 1*1 =1
Here n==0 return 1;
Finally, return the output to the main function call.
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
