Sentinel: The Vanguard of Discord Security
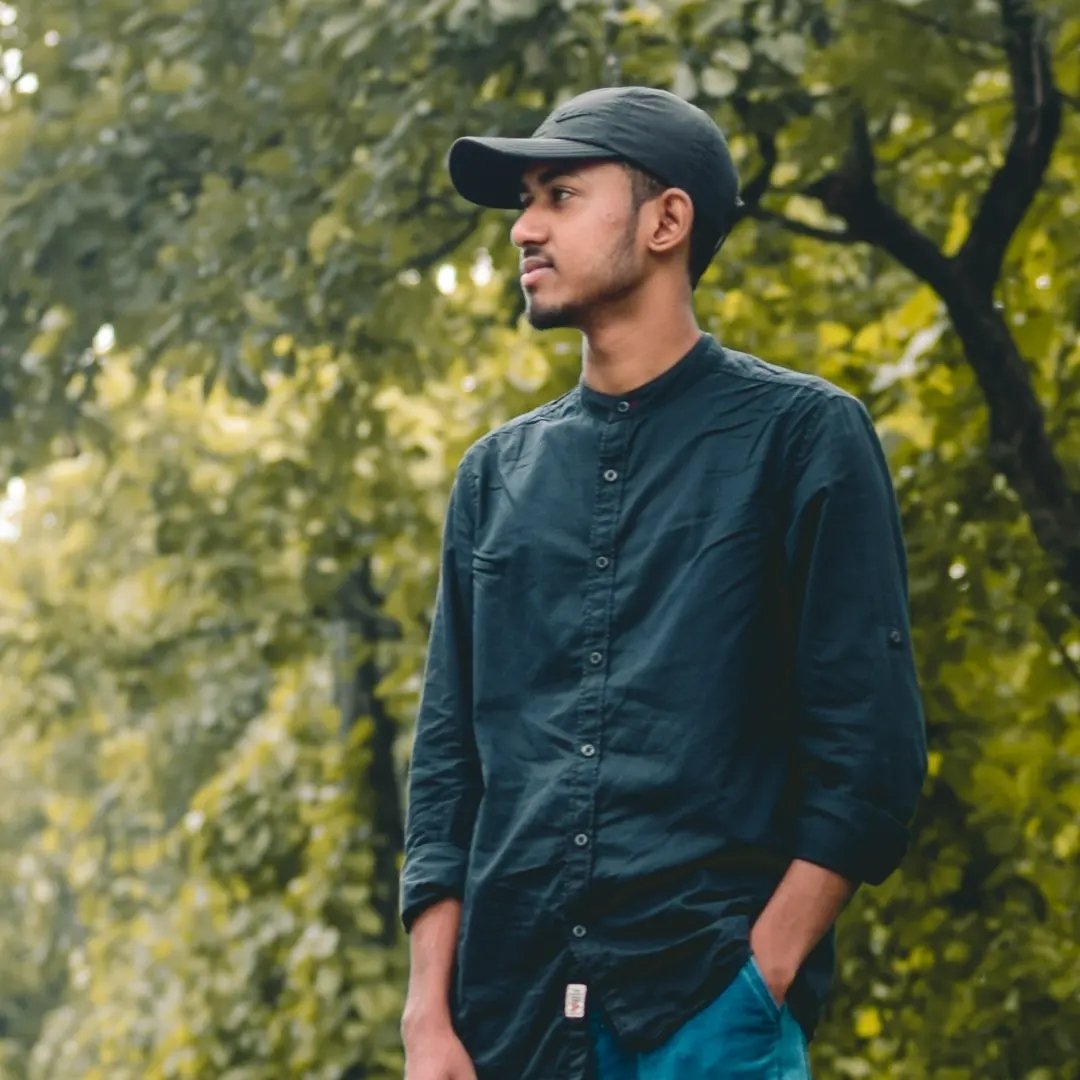
Table of contents
- Preparing for the Bot action
- Understanding the Code:
- Implementing API Key Redaction with Sentinel
- Understanding Pangea: The Backbone of Sentinel's Security
- Integrating Pangea's Redact Service: A Step-by-Step Guide
- Implementing Redaction with Pangea's SDK
- Integrating Redact into Sentinel's Code
- Conclusion: Enhancing Community Security with Sentinel
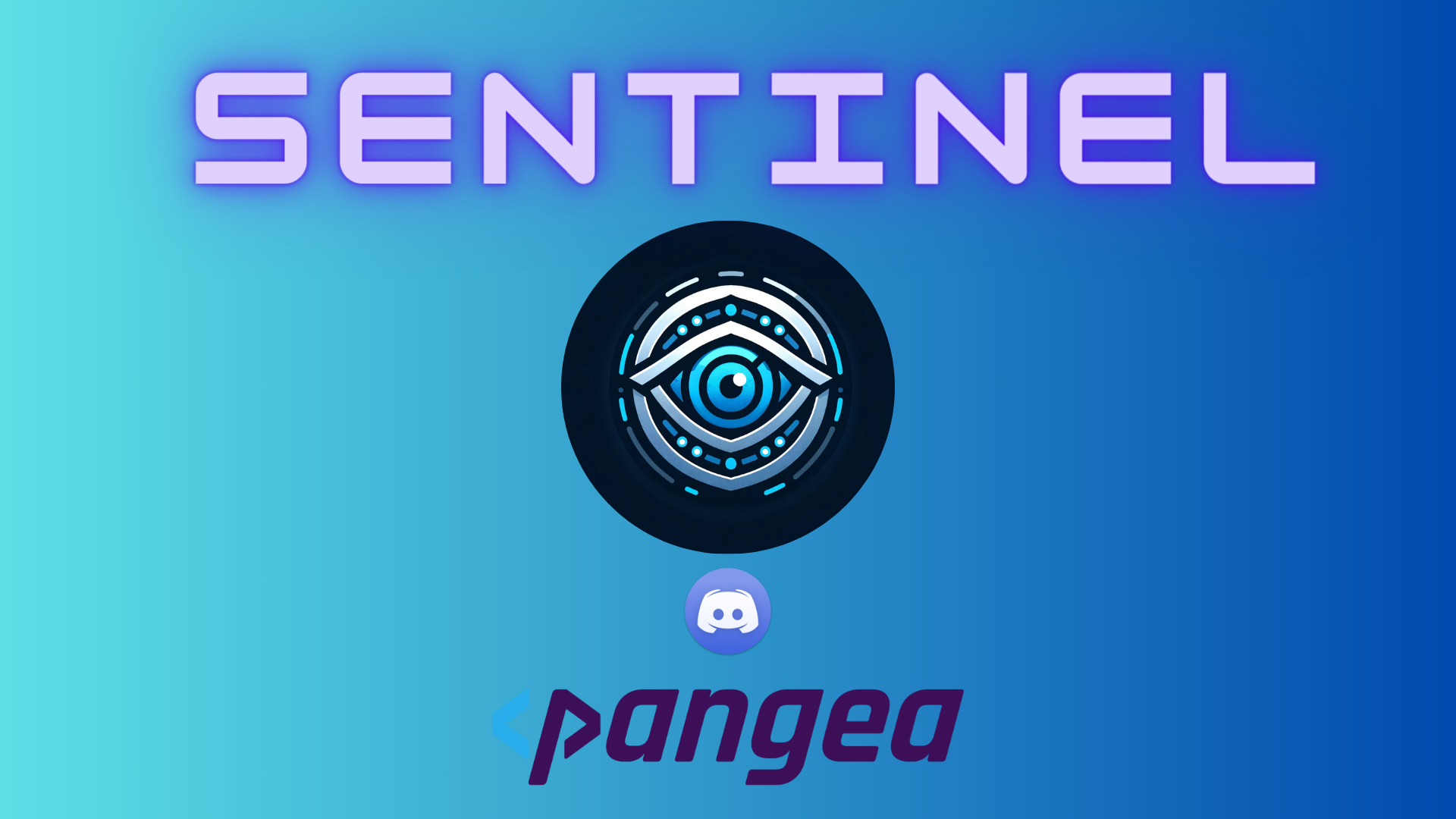
Hello, Discord enthusiasts and developers! Today, let's talk about something really important: making sure our Discord servers are safe and secure. As someone who spends a lot of time in different Discord communities, I've seen how important it is to keep our online spaces safe from any kind of danger.
Discord is more than just a place to chat. It's where developers, businesses, and lots of other people come together. That means we need to be really careful about protecting our shared space. There are many things to watch out for, like harmful links and the risk of data being stolen. I'm writing this blog to share my journey in creating a Discord bot using Pangea. I want to help make our servers the safest and most fun places on the internet.
As I embarked on this exciting project, the first step was to conceptualize a name that encapsulates the bot's core function. After much thought, I settled on "Sentinel," a name that resonates with its role as a vigilant protector of our Discord communities. To visually represent Sentinel, I ventured into the realm of graphic design, using Canva and DALLE 3 to create a straightforward yet striking logo. This logo, which you can view at the top of this article, is designed to visually align with Sentinel's purpose and functionality.
Preparing for the Bot action
For Sentinel, Python is my language of choice, given its versatility and robust support for bot development. If you're new to creating a Discord bot, I highly recommend starting with the Discord.py documentation for a comprehensive guide.
Steps to Set Up Your Bot:
Creating a Bot Account: First things first, you'll need to create a bot account. A detailed guide on this can be found at Creating a Bot Account in the Discord.py documentation.
Setting Up the Bot Application: Here's the process to get your bot up and running:
Create an application: Head to the Discord Developer Portal and set up a new application. Fill in the general information about your bot.
Configuring OAuth2 and Permissions: Navigate to the OAuth2 section and use the URL generator. Here, you'll select the bot's scope and assign the necessary permissions. I’m granting access. Once you've configured the permissions, a link will be generated at the bottom of the page.
Adding the Bot to Your Server: Copy the generated link and paste it into your web browser. This will add the bot to your Discord server.
Enable Message Content Intent: In the Discord Developer Portal, under the "Bot" tab of your application, make sure to enable "MESSAGE CONTENT INTENT". This is crucial for allowing your bot to read and interact with messages on your server.
Once these steps are completed, your bot will appear as 'Offline' in your Discord server. It's now time to breathe life into it.
Setting Up Your Environment: Open your preferred code editor and get ready to write some Python code. If you're not sure which editor to use, some popular choices include Visual Studio Code, PyCharm, or even a simple text editor like Sublime Text.
Creating the Bot File: Start by creating a new file named
bot.py
. This file will house your bot's code.Initial Code and Bot Token: Before writing the bot's functionality, you'll need the bot token, which acts as a key to connect your code to your Discord bot. You can find this token on the "Bot" page in the Discord Developer Portal. Remember, the token is sensitive information; it should be kept private and only viewed when necessary. If it's compromised, you'll need to regenerate it.
import discord
class MyClient(discord.Client):
async def on_ready(self):
print(f'Logged on as {self.user}!')
async def on_message(self, message):
print(f'Message from {message.author}: {message.content}')
intents = discord.Intents.default()
intents.message_content = True
client = MyClient(intents=intents)
client.run('your bot token here')
Understanding the Code:
Class Inheritance: Here, we define
MyClient
, a class inheriting fromdiscord.Client
. This parent class from the Discord library provides essential functionalities to interact with the Discord API.Event Handlers:
on_ready
: This asynchronous method is triggered when the bot successfully logs in. It's a great place to confirm that the bot is up and running.on_message
: This method responds to incoming messages. Every time a message is sent in a server where the bot is present, this method prints the message's content and its author to the console.
Intents Configuration: The
discord.Intents.default()
call sets up the default intents for our bot, which determine what types of events it can listen to. We specifically enablemessage_content
intent to allow the bot to read the content of messages.Running the Bot: Finally, we create an instance of
MyClient
and callrun
, passing in the bot token. This token is like the key that connects our code to the Discord bot, allowing it to log in and start interacting in servers.
This basic setup gets our bot off the ground, ready to be expanded with more advanced features, including the integration of Pangea's Redact API for enhanced security measures.
For a deeper understanding, you might want to explore the quick introduction page in the Discord.py documentation.
Implementing API Key Redaction with Sentinel
Consider this: you're coding, copying, and pasting snippets in a Discord chat, and without realizing it, you've just shared a sensitive API key. It's a scenario many developers encounter, especially when collaborating or seeking assistance in community chats. Unintentionally sharing credentials like API keys is risky, potentially leading to unauthorized use and unexpected charges.
What if there was a safeguard against such slips? This is where Sentinel steps in, with the crucial task of automatically redacting API keys shared in chat.
- How Redaction Enhances Security: Redaction is the process of meticulously removing sensitive data from text, making it unreadable and secure. It's vital for preserving privacy, complying with regulatory standards, and protecting information from unauthorized exposure.
Sentinel's Approach to Redacting Sensitive Information:
Scanning Messages: Sentinel continuously monitors chat messages for the presence of API keys and other sensitive data.
Automatic Redaction: Upon detecting an API key, Sentinel removes it, replacing the sensitive information with a message.
Notifying Users: After redaction, Sentinel informs the chat that "Message from user contained sensitive information and was removed", maintaining transparency and awareness within the community.
To accomplish this, Sentinel harnesses the power of Pangea's Redact service API. This integration enables the bot to efficiently identify and secure sensitive credentials shared in Discord channels, thus playing a pivotal role in protecting the community from potential security breaches.
Understanding Pangea: The Backbone of Sentinel's Security
Pangea stands at the forefront of cloud-based platforms, specifically engineered for application developers. This comprehensive platform offers an extensive suite of security services, each designed to address critical aspects of digital safety and compliance. Here’s what Pangea brings to the table:
Robust Security Services: Ranging from user authentication and authorization to audit logging, Pangea covers all bases. It's a one-stop shop for managing secrets, enforcing entitlements and licensing, and ensuring data privacy.
PII Redaction and More: Particularly vital for Sentinel, Pangea's ability to redact Personally Identifiable Information (PII) is crucial. Beyond this, it also offers intelligent solutions for handling files, IP addresses, URLs, and domains.
Compliance and Reliability: For developers who need to adhere to GDPR and data residency regulations, Pangea emerges as a dependable ally. Its focus on compliance makes it a go-to resource for building secure, regulation-friendly applications.
Getting Started with Pangea: To leverage Pangea’s capabilities, start by signing up and navigating the Pangea Console. Here, you’ll need to obtain two key pieces of information:
Pangea Token: This token serves as your access key to Pangea’s plethora of services.
Domain Information: Essential for configuring your applications to interact with the Pangea Cloud.
These credentials are the linchpins for activating and utilizing Pangea's services, including the Redact service that powers Sentinel’s security features.
Integrating Pangea's Redact Service: A Step-by-Step Guide
Once you're familiar with the basics of Pangea, it's time to delve into one of its key services: Redact. This service is pivotal for Sentinel’s ability to ensure chat security on Discord. To understand more about Redact and how to use it, it’s worthwhile to explore Pangea's documentation.
- Implementing Redact in Your Project: To integrate Redact into your application, follow these steps:
Activate the Redact Service: In the Pangea Console, locate and enable the Redact service. This is the first step in utilizing its capabilities.
Set Up a Token: Generate a unique token for the Redact service. This token will authenticate your requests to Pangea’s Redact API.
Configure Redact Rules: Tailor the Redact settings to meet your specific needs. Pangea allows you to customize the rules based on the type of information you need to redact.
Testing the Service: Before integrating the service into your application, use the Test Rules feature. This helps you understand how the Redact service functions and ensures it aligns with your requirements.
Integration into Your Application: With the token set up and rules configured, integrate the Redact service into your application. This enables your application to automatically redact sensitive information as per the defined rules.
- Understanding Rulesets: Rulesets in Pangea's Redact service are essentially collections of redaction rules categorized for specific types of data. For Sentinel, we focus on the 'Secrets' ruleset, designed to identify and redact commonly used credentials. By testing these rules within Pangea’s framework, you can fine-tune how Sentinel detects and handles sensitive data in Discord chats.
Implementing Redaction with Pangea's SDK
To illustrate how Pangea's Redact service works in practice, I conducted a test using the 'Pangea Token' rule. By inputting a sample token, I could see firsthand how the service adeptly replaced the actual token with <PANGEA_TOKEN>
, effectively redacting the sensitive information.
Incorporating Redact into Sentinel: Now, let's integrate this functionality into our Discord bot using Pangea's Python SDK. The integration is straightforward, as shown in the code snippet below:
pythonCopy coderedact_response = redact.redact(text=text, rulesets=["SECRETS"])
In this implementation, the redact
function is central to our operation. It takes two key arguments:
text: This is the actual text from the Discord chat that we want to scan for sensitive information. In our bot's context, it would be the content of a Discord message.
rulesets: Here, we specify which set of redaction rules to apply. By choosing the
["SECRETS"]
ruleset, we're instructing the service to focus on detecting and redacting typical credentials and sensitive data, as configured in our Pangea console settings.
By integrating this code into Sentinel, the bot is now empowered to automatically scan and redact sensitive data from messages, maintaining a higher standard of security and privacy within the Discord community.
Integrating Redact into Sentinel's Code
With our foundational bot setup and an understanding of Pangea's Redact service, the next step is to integrate the redaction functionality into the bot.py
file. This integration will enable Sentinel to actively scan and redact sensitive information from Discord messages.
Deep Dive into Sentinel's Code: Automating Sensitive Data Redaction
Here's the core code that powers Sentinel, our Discord bot, equipped with Pangea's Redact service to ensure secure communication within Discord communities:
from dotenv import load_dotenv
load_dotenv()
import os
import discord
from discord.ext import commands
import pangea.exceptions as pe
from pangea.config import PangeaConfig
from pangea.services import Redact
# Pangea Redact service setup
token = os.getenv("PANGEA_REDACT_TOKEN")
domain = os.getenv("PANGEA_DOMAIN")
config = PangeaConfig(domain=domain)
redact = Redact(token, config=config)
# Discord bot setup
discord_token = os.getenv("DISCORD_BOT_TOKEN")
intents = discord.Intents.all()
intents.messages = True
bot = commands.Bot(command_prefix="!", intents=intents)
@bot.event
async def on_ready():
print(f"{bot.user} has connected to Discord!")
@bot.event
async def on_message(message):
if message.author == bot.user:
return
try:
# Redact API keys using Pangea Redact service
redact_response = redact.redact(text=message.content)
if redact_response.result.redacted_text != message.content:
await message.delete()
await message.channel.send(f"Message from {message.author} contained sensitive information and was removed.")
print(f"Removed sensitive information from a message by {message.author} in channel {message.channel}.") # Log to console
except pe.PangeaAPIException as e:
print(f"Pangea Redact Error: {e.response.summary}")
for err in e.errors:
print(f"\t{err.detail}")
await bot.process_commands(message)
bot.run(discord_token)
Code Functionality Explained:
Environment Setup: We start by loading environment variables using
load_dotenv()
, which is crucial for securely accessing our tokens and domain information without hardcoding them into the script.Pangea Redact Service Configuration: The bot initializes Pangea's Redact service with the necessary credentials -
PANGEA_REDACT_TOKEN
andPANGEA_DOMAIN
, which are fetched from the environment variables.Discord Bot Initialization: The bot is set up with the Discord token (
DISCORD_BOT_TOKEN
) and configured to use all available intents, including messages, which is necessary for reading message content in chat.Event Handling: Two key events are handled by the bot:
on_ready
: Triggered when the bot successfully connects to Discord, confirming its active status.on_message
: This is where the primary functionality resides. For every message that the bot sees (and that is not sent by the bot itself), the following occurs:The message content is passed to Pangea's Redact service.
If the Redact service finds and redacts any sensitive information (like API keys, phone numbers, credit card numbers, IP addresses, etc.), the original message is deleted from the Discord chat.
A notification is sent to the channel, informing users that a message containing sensitive information was removed. This maintains transparency within the community.
Error Handling: The code is equipped to handle exceptions from Pangea's API, ensuring that any issues during the redaction process are logged for review and troubleshooting.
Running the Bot: Finally, the bot is run using the
bot.run
method with the Discord bot token, bringing Sentinel online in your Discord server.
What this code achieves is a seamless integration of advanced redaction capabilities within a Discord environment. Sentinel actively monitors and sanitizes chat messages, automatically removing sensitive data and thus significantly enhancing the security and privacy of your Discord server.
Securing Sensitive Information with Environment Variables
In Sentinel's development, we prioritize security by using environment variables for storing sensitive data like API keys. This approach keeps these details separate from the code, enhancing security and making it easier to manage and update keys without altering the script. By loading these variables at runtime, Sentinel maintains high security standards while efficiently managing sensitive information.
Conclusion: Enhancing Community Security with Sentinel
In summing up, the security and safety of our Discord servers are crucial for fostering a positive and engaging online community. As we engage in varied discussions and collaborative efforts, it's essential to be aware of the risks, from inadvertent sharing of sensitive data to potential security breaches.
The development of Sentinel, our innovative bot powered by Pangea's Redact service, represents a vital leap towards safeguarding the digital spaces we share. Sentinel diligently works to identify and remove sensitive information, thereby bolstering the security of our Discord servers. This proactive approach not only enhances safety but also contributes to a more welcoming and enjoyable environment for all community members.
As we continue to evolve and improve Sentinel, I invite the community to join in this journey. Your contributions and feedback are invaluable in making Sentinel an even more effective tool, ensuring our Discord servers remain secure and vibrant places for connection and collaboration.
GitHub Repository - Sentinel
RESOURCES
Pangea | Security Services for Developers: Explore Pangea's comprehensive cloud platform, offering a range of security services for developers.
Pangea | Documentation: Access the official documentation for Pangea to learn more about its services and how to integrate them.
discord.py Documentation: Refer to the official documentation for discord.py, which provides guidance on creating Discord bots in Python.
FOLLOW ME FOR MORE
Subscribe to my newsletter
Read articles from SEIKH NABAB UDDIN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
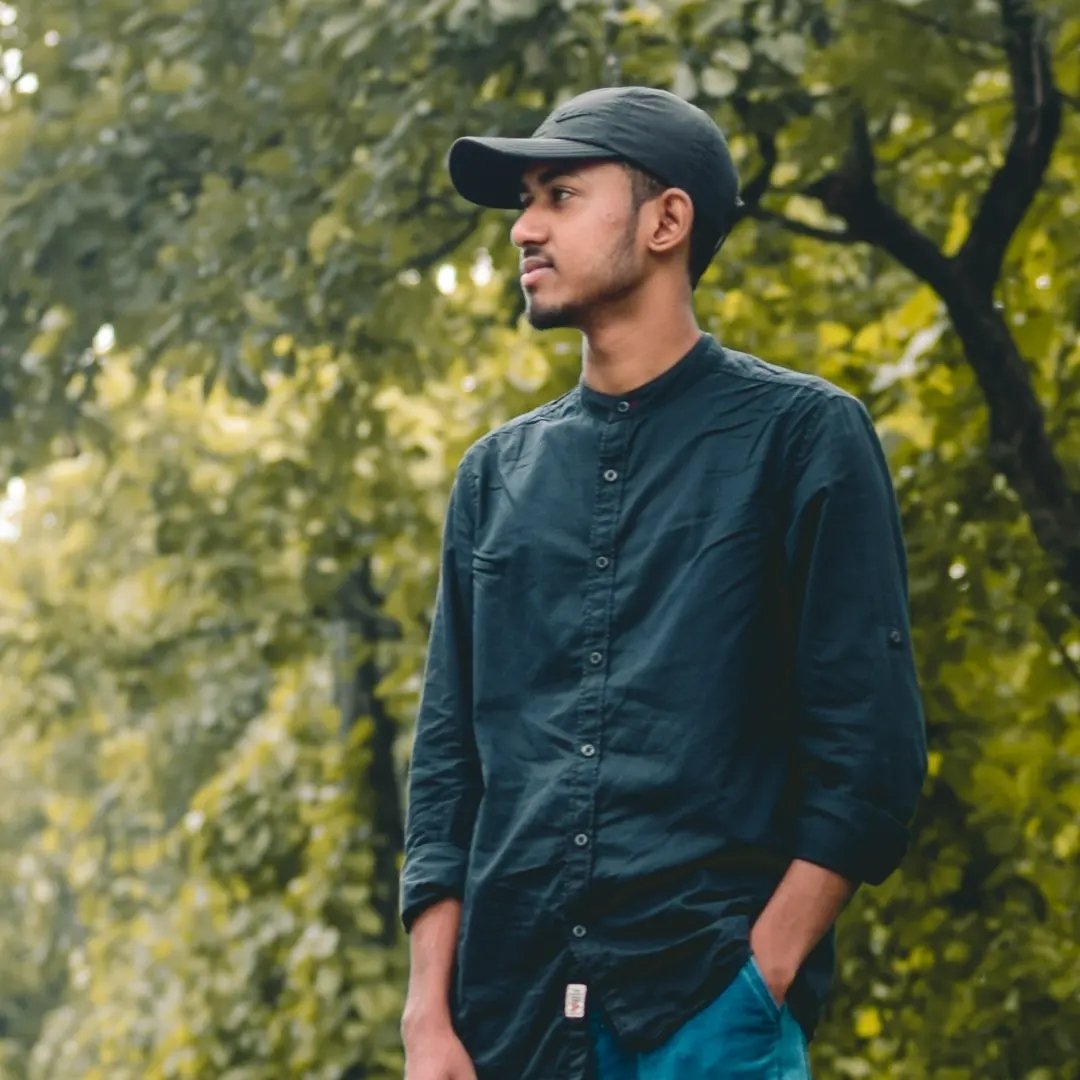
SEIKH NABAB UDDIN
SEIKH NABAB UDDIN
"Hello, I'm Seikh Nabab Uddin, a multi-disciplinary developer specializing in AR Development, Frontend Web Development, 3D Designing with Blender,, VR Environment Development, Python, and Java. With a passion for blending creativity with technology, I thrive on working on innovative projects that push the boundaries of what's possible. In addition to my technical expertise, I also love sharing my knowledge through blogging on my personal website. I'm committed to staying up-to-date with the latest trends and techniques in the industry, ensuring that I deliver high-quality work that exceeds client expectations.