Python Libraries for DevOps - JSON and YAML Handling
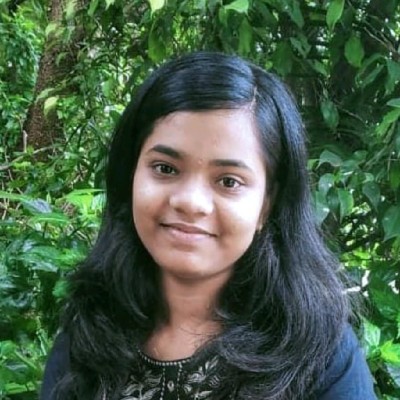
Hey everyone! ๐ Today's task in the DevOps journey involves handling JSON and YAML files in Python.
๐ Python Libraries :
json:
Purpose: Simplifies handling JSON data.
Usage: Effortless processing of JSON.
Why It Matters: Easy integration with web APIs, configuration files, and data interchange.
yaml:
Purpose: Makes YAML file handling a breeze.
Usage: Effortless management of YAML files.
Why It Matters: Smooth interaction with configuration files, particularly in DevOps tasks.
Tasks:
1. Writing to JSON:
Create a Python dictionary.
Use the json library to write it to a JSON file.
Code :-
import json
my_dict = {"cloud_provider": "AWS", "service": "EC2"} with open("output.json", "w") as json_file: json.dump(my_dict, json_file)
2. Reading JSON File:
Read a JSON file (services.json) containing cloud service provider information.
Extract and print the service names.
Code:-
with open("services.json", "r") as json_file: data = json.load(json_file)
for provider, service in data.items():
print(f"{provider}: {service}")
3. YAML to JSON:
Read a YAML file (services.yaml) and convert its contents to JSON.
Code:-
import yaml
with open("services.yaml", "r") as yaml_file: yaml_data = yaml.safe_load(yaml_file)
Assuming you have a function to convert YAML to JSON
json_data = convert_yaml_to_json(yaml_data) print(json_data)
Subscribe to my newsletter
Read articles from Aakanksha Deshmukh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
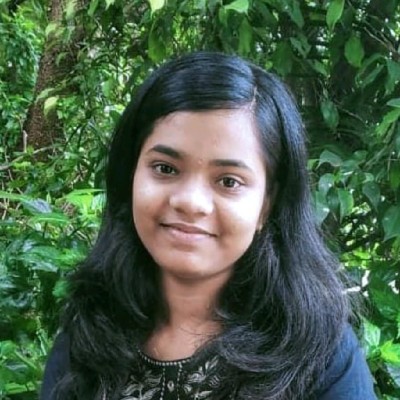
Aakanksha Deshmukh
Aakanksha Deshmukh
I am a Devops Engineer