Longest Common Prefix

1 min read
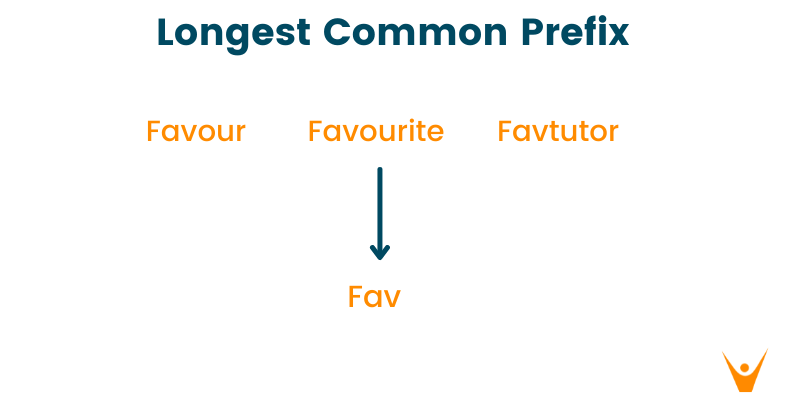
The longest common prefix is a problem in that we can find the prefix in every string of the array.
str = [ "flower", "flow", "flight" ]
output: fl (every string in the array contains the "fl" as its prefix.
public class LogestCommonPrefix {
public static void main(String[] args) {
String[] strs = {"flower","flow","flight"};
System.out.println(Arrays.toString(strs));
int len = strs.length;
String answer="";
if(len==1){
answer = strs[0];
}
Arrays.sort(strs);
int minValue = Math.min(strs[0].length(), strs[len-1].length());
int i=0;
while(i<minValue && strs[0].charAt(i) == strs[len-1].charAt(i)){
i++;
}
answer = strs[0].substring(0,i);
System.out.println("Common prefix: "+answer);
}
}
[flower, flow, flight]
Common prefix: fl
creating external method (OOPS):
public class LogestCommonPrefix {
public static void main(String[] args) {
String[] strs = {"flower","flow","flight"};
System.out.println(Arrays.toString(strs));
System.out.println("Common prefix: "+longestCommonPrefix(strs));
}
public static String longestCommonPrefix(String[] strs)
{
int len = strs.length;
if(len==0){
return "";
}
if(len==1){
return strs[0];
}
Arrays.sort(strs);
int minValue = Math.min(strs[0].length(), strs[len-1].length());
int i=0;
while(i<minValue && strs[0].charAt(i) == strs[len-1].charAt(i)){
i++;
}
String str = strs[0].substring(0,i);
return str;
}
}
[flower, flow, flight]
Common prefix: fl
Thank you for reading :)
0
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
