Hoisting in JavaScript: Demystifying the 'Lift and Shift'
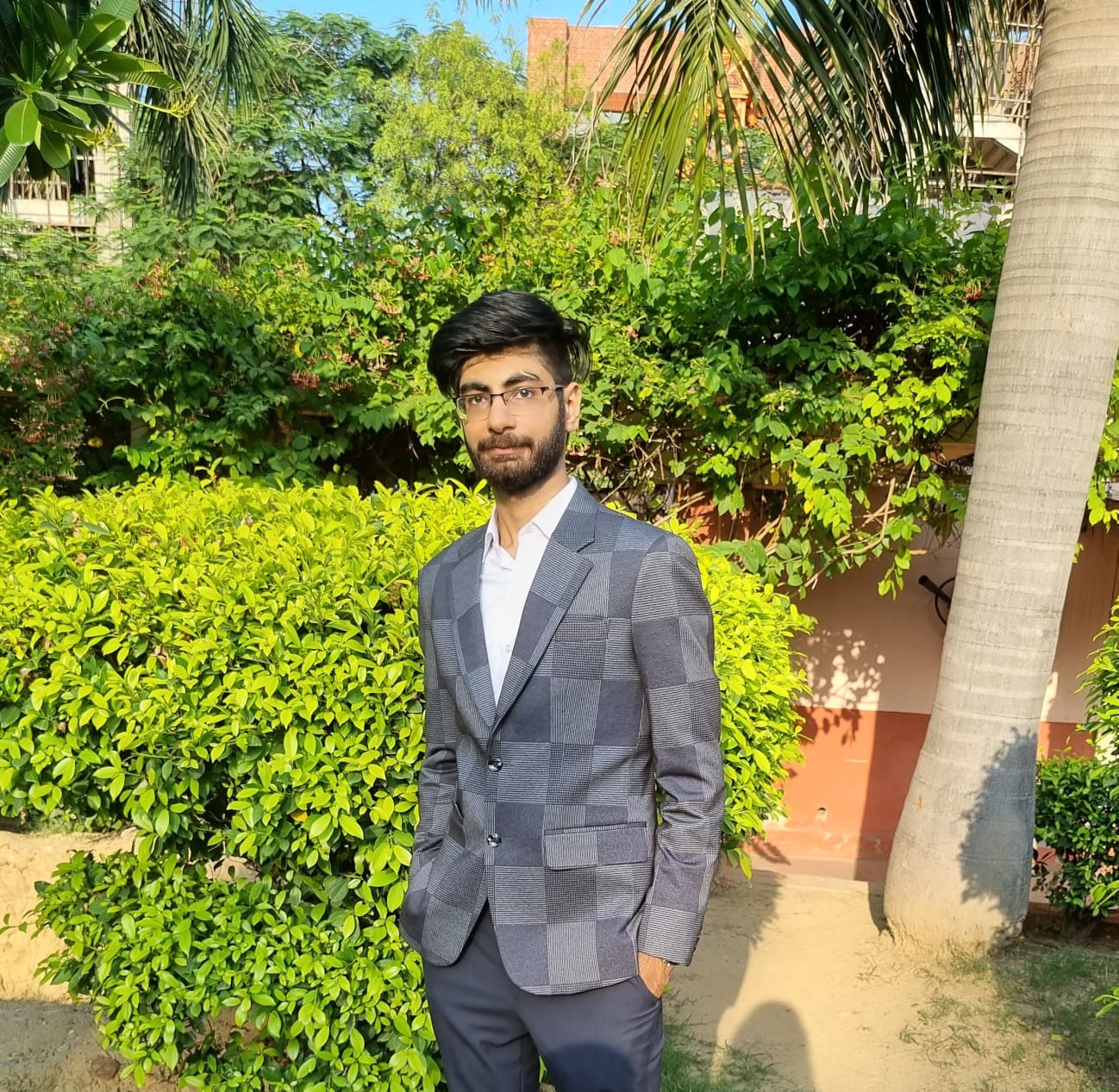
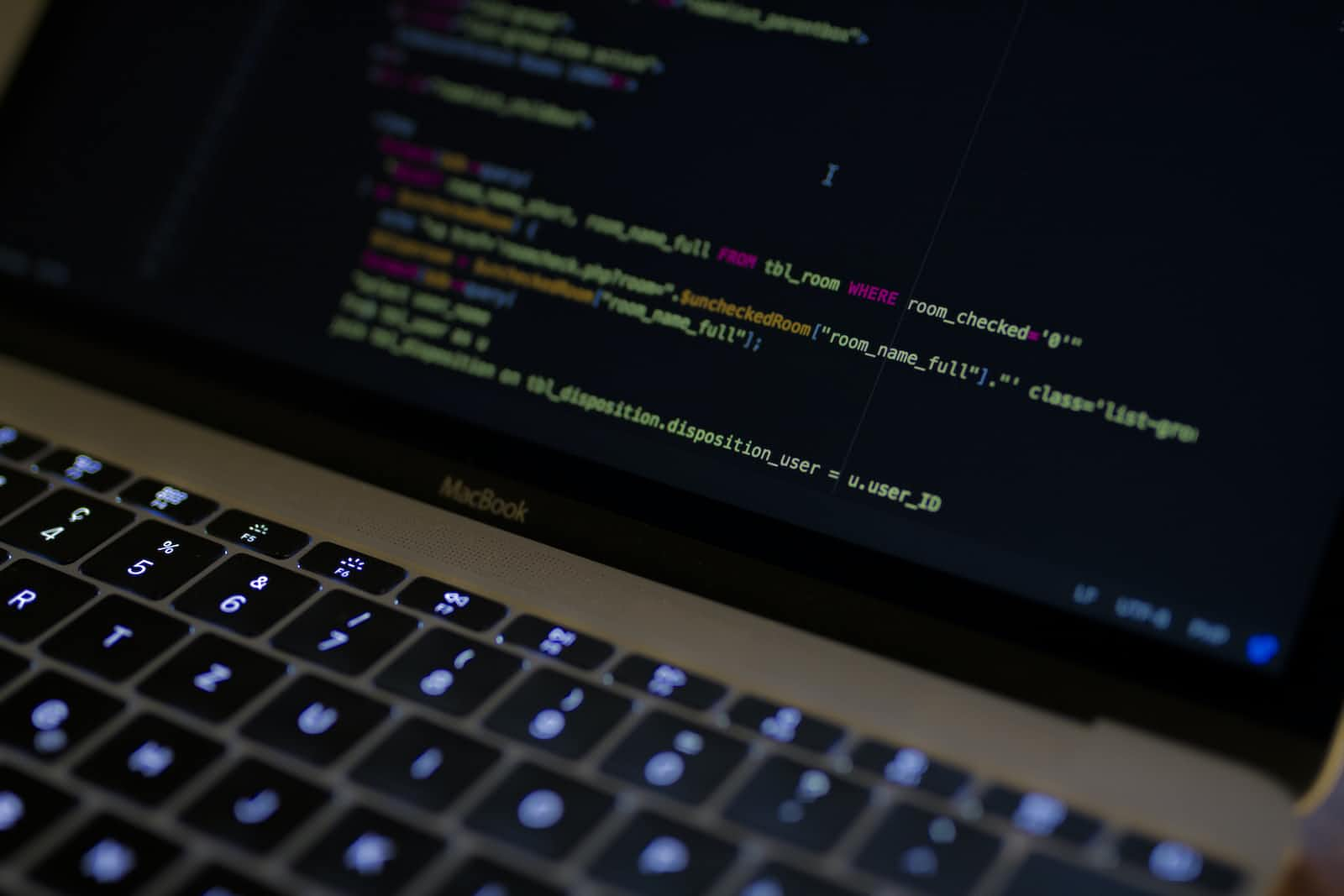
JavaScript, the language of the web, has its share of enchanting mysteries. One such mystery is "hoisting." Imagine it as a magical 'Lift and Shift' that happens behind the scenes. Let's unravel this wizardry in the world of JavaScript and make it as simple as your morning coffee routine.
What is Hoisting, Anyway? Hoisting is like that friend who shows up early to the party, lifting things to the top. In JavaScript, during the compilation phase, variable and function declarations are lifted to the top of their containing scope. Sounds confusing? Let's break it down with a tale of two examples.
Example 1: The Variable Waltz
console.log(x); // Outputs: undefined
var x = 5;
Here, the variable x
is lifted to the top, but its value isn't. So, when we try to log it, we get a friendly "undefined." JavaScript says, "Hey, I know x
exists, but I haven't gotten to the part where it's assigned yet!"
Example 2: The Function Tango
sayHello(); // Outputs: "Hello, World!"
function sayHello() {
console.log("Hello, World!");
}
In this dance, the entire function is lifted, allowing us to call it before the actual declaration. JavaScript says, "No worries, I've got the steps for sayHello
right here!"
The Dance of Scopes In the world of JavaScript, we have two types of scopes: block scope (with let
and const
) and function scope (with var
). Hoisting behaves differently in these dances.
Block Scope:
console.log(city); // Throws an error - city is not defined
let city = "New York";
Block-scoped variables don't get the VIP hoisting treatment. JavaScript says, "Sorry, I can't lift city
to the top; it's only available from the point it's declared."
Function Scope:
console.log(animal); // Outputs: undefined
var animal = "Tiger";
Function-scoped variables, on the other hand, get the 'Lift and Shift' magic. We can access animal
even before its declaration, getting an "undefined" as JavaScript's way of saying, "It exists, but I'm not there yet."
Best Practices: How to Dance with Hoisting
Use
let
andconst
: Embrace the modern block-scoped variables to avoid unexpected hoisting surprises.Declare at the Top: Declare your variables and functions at the top of their scope for clarity and to match JavaScript's dance steps.
In Conclusion: The Hoisting Magic
So, that's the secret behind hoisting in JavaScript - it's like a behind-the-scenes helper that lifts things up, so your code runs smoothly. Understanding this magic trick helps you write code that works well and makes your JavaScript journey more fun. Next time you see 'Lift and Shift' in your code, just know it's JavaScript doing its thing to keep everything in order. Happy coding! ๐โจ
Subscribe to my newsletter
Read articles from Ojas Elawadhi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
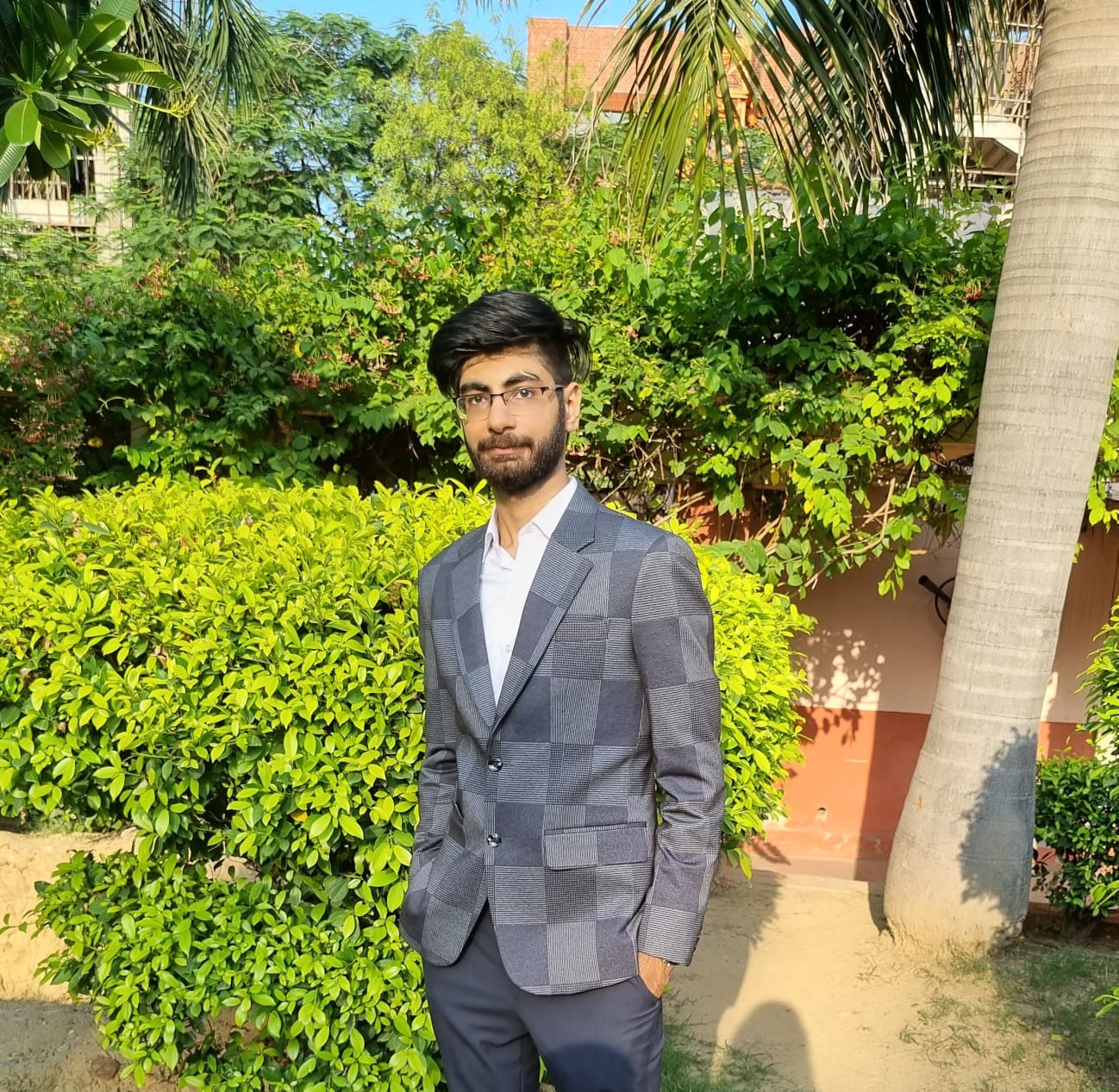