Node.js: Basics of Events
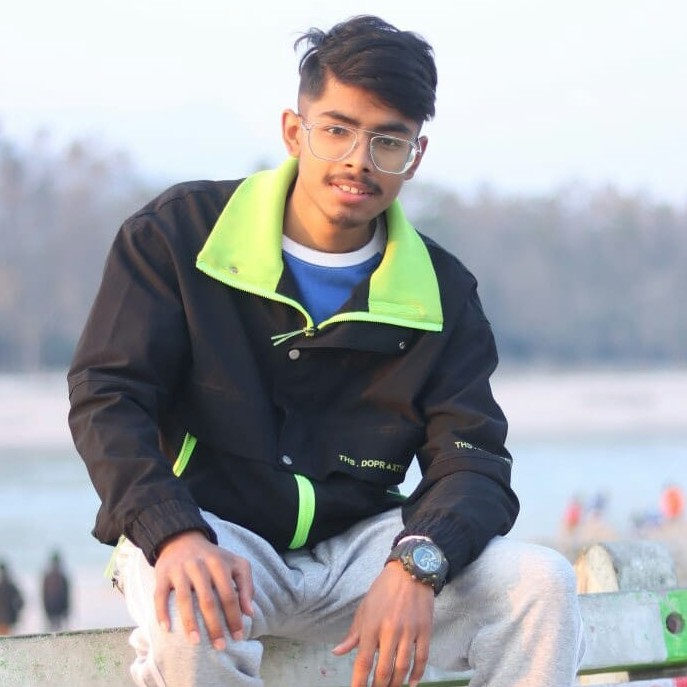
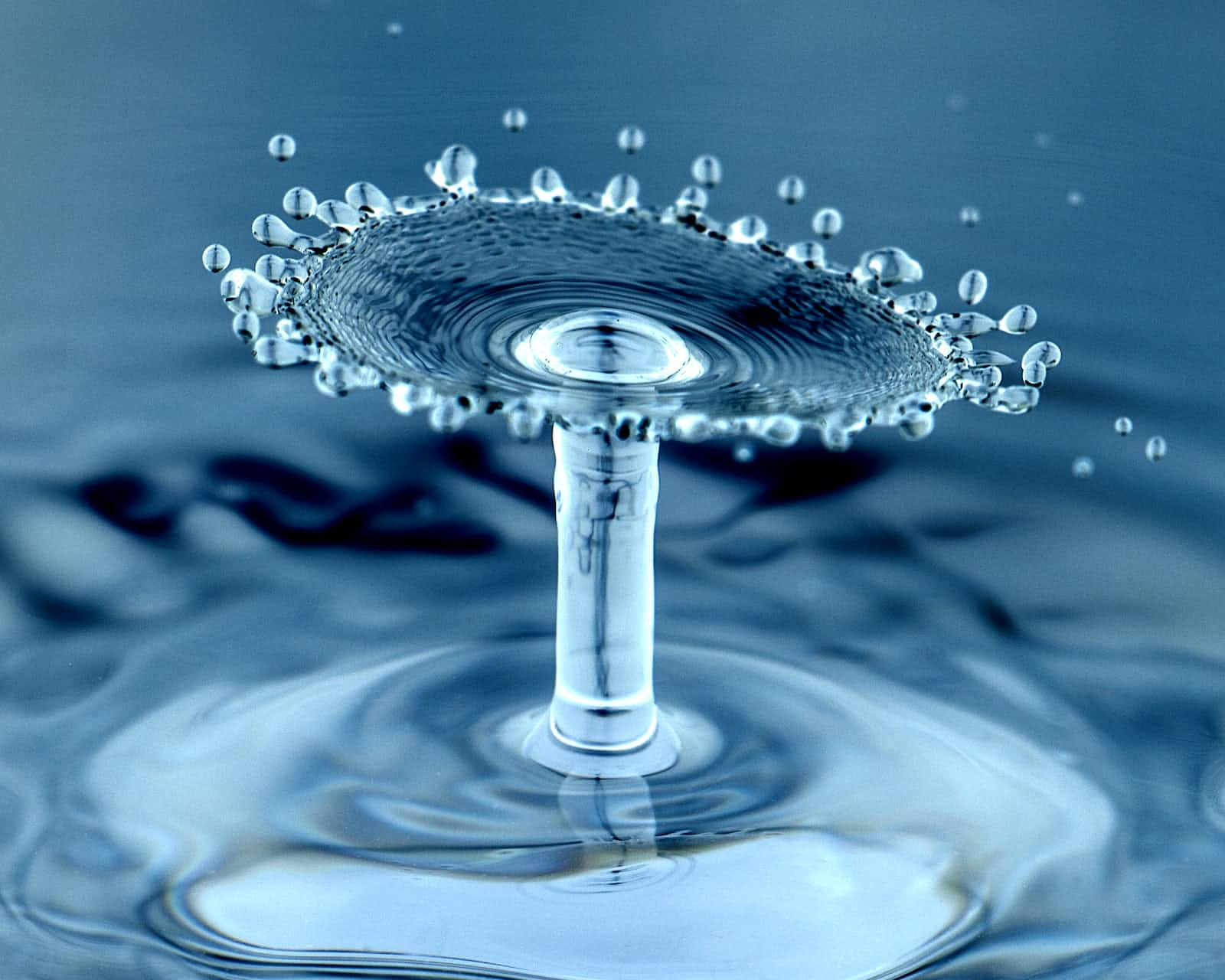
Just like JavaScript has the event-driven programming technique for the browser side, Node.js has it on the server side. Events are used heavily in Node.js. Setting up the event is similar to the browser-side JavaScript:
First, we need to listen to a particular event(predefined/custom).
Then, define a function that runs in response to that event.
To work with custom events, we need the events module:
// Here, 'EventEmitter' is a class
const EventEmitter = require("events");
// Now, 'myEmitter' is an object
const myEmitter = new EventEmitter();
Now, we can use the 'myEmitter' object to set up and fire events.
Let's create our custom event called 'rain', then:
// Adding event listener with the event object
myEmitter.on("rain", (place) => {
console.log("It's raining at", place);
});
// The event is triggered/emitted here
myEmitter.emit("rain", "Kathmandu");
At first, we used the 'on()' method to create a custom event type and defined its corresponding callback function. Then, we manually triggered the event using the 'emit()' method. Generally, we emit events based on some conditions.
Let's see an example of events in the 'http' module:
const http = require("http");
// Now, 'server' is an EventEmitter object in itself
const server = http.createServer((req, res) => {
res.write("Server Stared!");
});
server.listen(5000);
This is the basic setup for creating an HTTP server in NodeJS. Now, let's set up a predefined event of the 'http' module as follows:
// 'request' is a predefined event in 'http' module
server.on("request", () => {
console.log("User is requesting something!");
});
Here, the 'server' is our EventEmitter object and the 'request' is the predefined event that is triggered by the module itself when the user requests on the server.
That's all about events in NodeJS for surface-level understanding. Keep Learning!
Subscribe to my newsletter
Read articles from Giver Kdk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
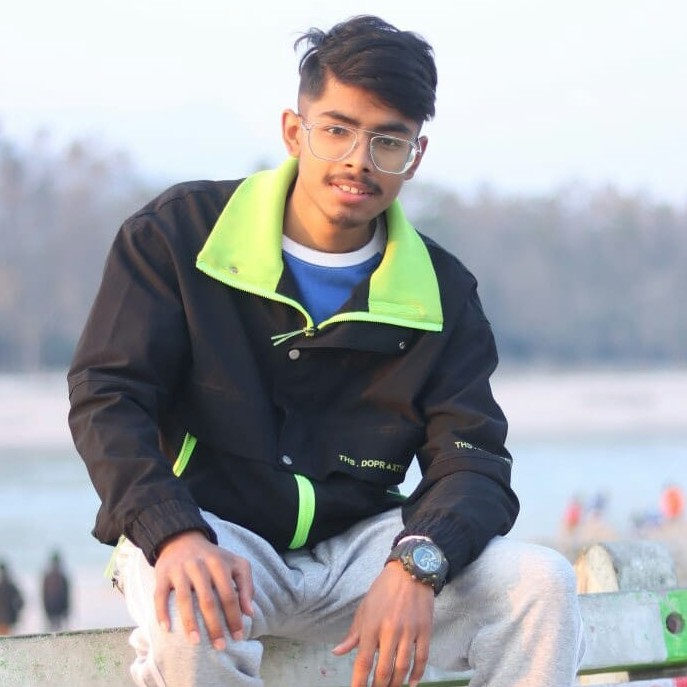
Giver Kdk
Giver Kdk
I am a guy who loves building logic.