Lets build a Simple P2P Lending Application in Go
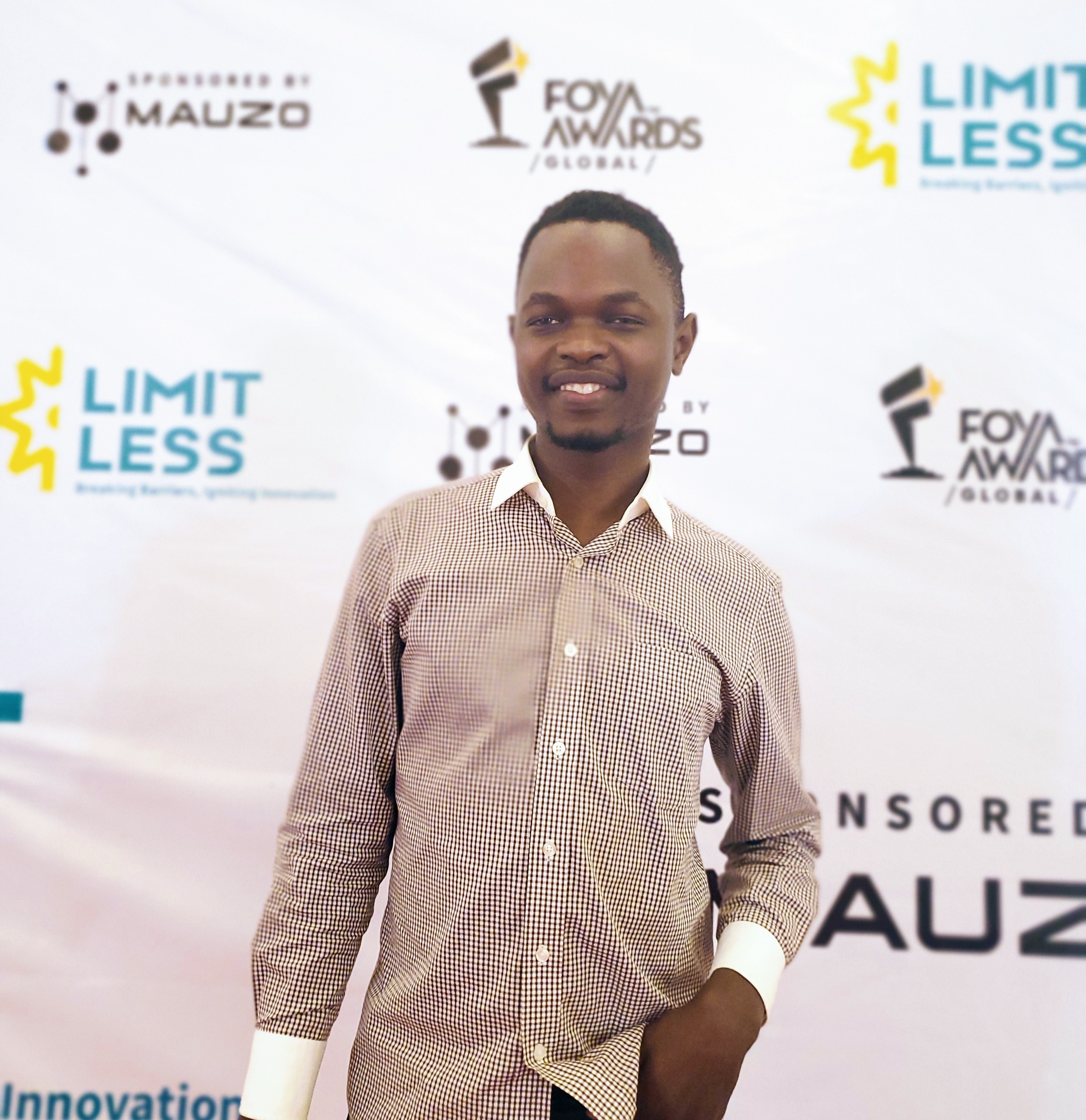
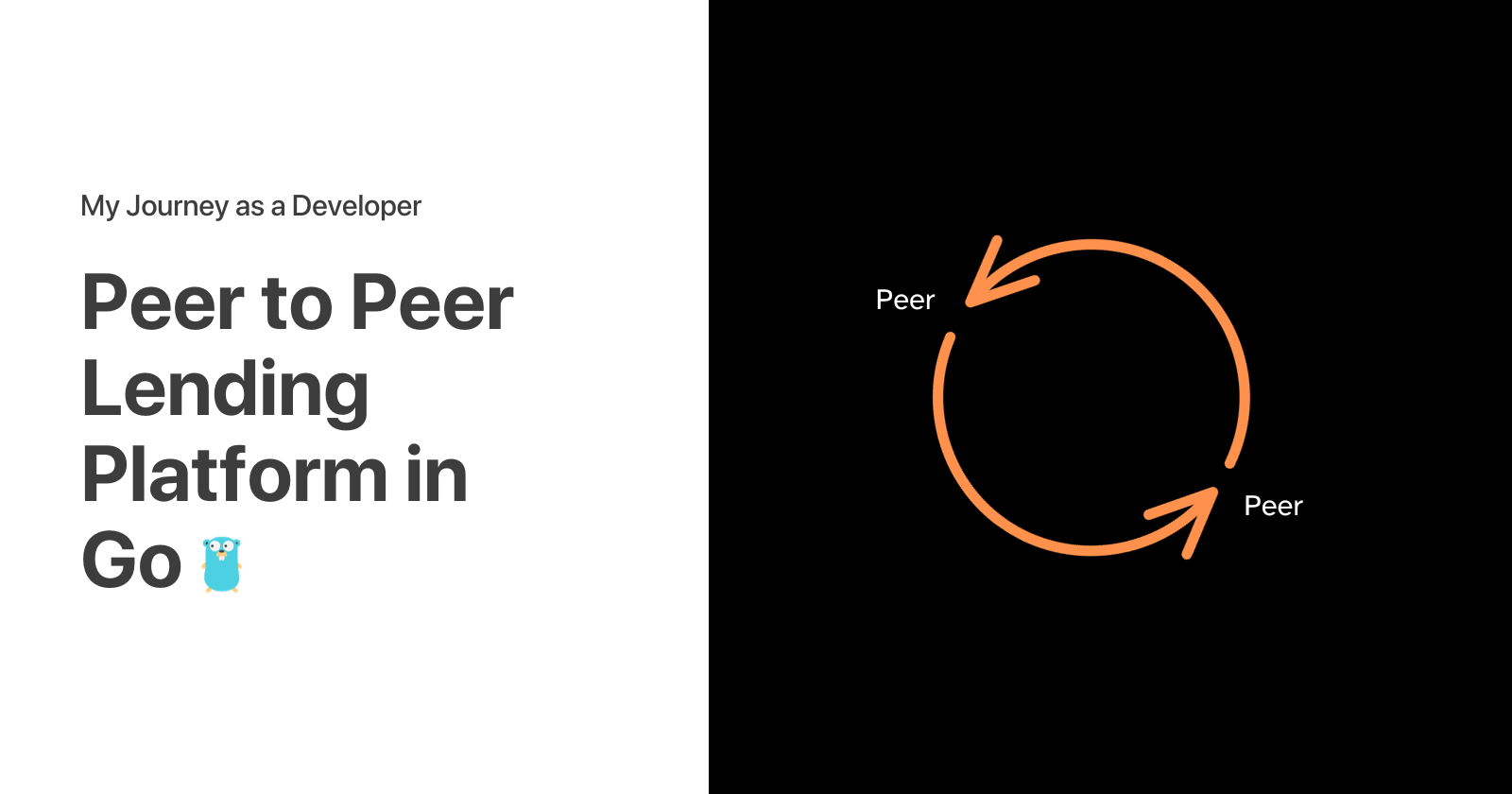
Welcome to the comprehensive tutorial on building a Peer-to-Peer (P2P) Lending Platform using Go. In the ever-evolving landscape of financial technology, P2P lending platforms have gained significant attention for their ability to connect borrowers directly with lenders, cutting out traditional financial intermediaries. In this tutorial, we will guide you through the process of creating a robust and scalable P2P lending platform using the Go programming language.
Whether you're a seasoned Go developer or a newcomer to the language, this tutorial aims to provide clear and detailed steps to help you understand the key components involved in building a P2P lending system. From setting up the initial project structure to implementing essential features and handling HTTP requests, each step is designed to enhance your understanding of Go's capabilities in the context of financial technology.
1. Setting Up Go Project
In this step, you'll set up the main file for your Go project. Create a file named main.go
and initialize your project. This file will handle the initialization of the server, database connection, and route handling.
// main.go
package main
import (
"fmt"
"net/http"
)
func main() {
fmt.Println("P2P Lending Platform")
// Initialize database, router, and other components
initialize()
// Define routes
http.HandleFunc("/borrowers", handleBorrowers)
http.HandleFunc("/lenders", handleLenders)
http.HandleFunc("/loans", handleLoans)
// Start server
http.ListenAndServe(":8080", nil)
}
2. Database Setup (Using GORM)
Create a new file named models.go
to define your data models and database connections. In this step, we are using GORM as the Object Relational Mapper (ORM) for database operations.
// models.go
package main
import (
"gorm.io/driver/sqlite"
"gorm.io/gorm"
)
var db *gorm.DB
func initialize() {
// Open a database connection
database, err := gorm.Open(sqlite.Open("p2p_lending.db"), &gorm.Config{})
if err != nil {
panic("Failed to connect to database")
}
// Migrate the database
database.AutoMigrate(&Borrower{}, &Lender{}, &Loan{})
// Assign the global variable for database access
db = database
}
3. Define Models
Create a new file named models.go
to define your data models. In this step, you are defining three models: Borrower
, Lender
, and Loan
. These models represent the entities in your P2P lending system.
// models.go
package main
import "gorm.io/gorm"
type Borrower struct {
gorm.Model
Name string
Email string
}
type Lender struct {
gorm.Model
Name string
Email string
}
type Loan struct {
gorm.Model
BorrowerID uint
LenderID uint
Amount float64
Status string // Pending, Approved, Rejected, Completed, etc.
}
4. Handling HTTP Requests
Create a new file named handlers.go
to handle HTTP requests. In this step, you are defining functions to handle different routes related to borrowers, lenders, and loans.
// handlers.go
package main
import (
"encoding/json"
"net/http"
)
func handleBorrowers(w http.ResponseWriter, r *http.Request) {
// Handle GET, POST, PUT, DELETE requests for borrowers
}
func handleLenders(w http.ResponseWriter, r *http.Request) {
// Handle GET, POST, PUT, DELETE requests for lenders
}
func handleLoans(w http.ResponseWriter, r *http.Request) {
// Handle GET, POST, PUT, DELETE requests for loans
}
5. Implement Business Logic
In this step, you will implement the business logic of your P2P lending platform. This includes functions for creating loans, approving/rejecting loans, and updating the loan status based on repayment.
6. Frontend (Optional)
If you choose to implement a frontend, create the necessary HTML, CSS, and JavaScript files. You can use a frontend framework like React or Vue.js, or keep it simple with plain HTML/CSS.
Remember to install the required Go packages using the following commands:
go get -u github.com/gin-gonic/gin
go get -u gorm.io/gorm
go get -u gorm.io/driver/sqlite
Conclusion:
Congratulations! You have successfully navigated through the creation of a P2P lending platform using the Go programming language. This tutorial aims to provide you with a solid foundation for building financial applications, emphasizing clean code practices and the efficient use of Go's features.
You can get the source code here https://github.com/eclane/peer_to_peer_lending
Feel free to explore further, leverage additional Go packages, and integrate advanced features to enhance the functionality of your P2P lending platform. Happy coding!
Subscribe to my newsletter
Read articles from Oscar John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
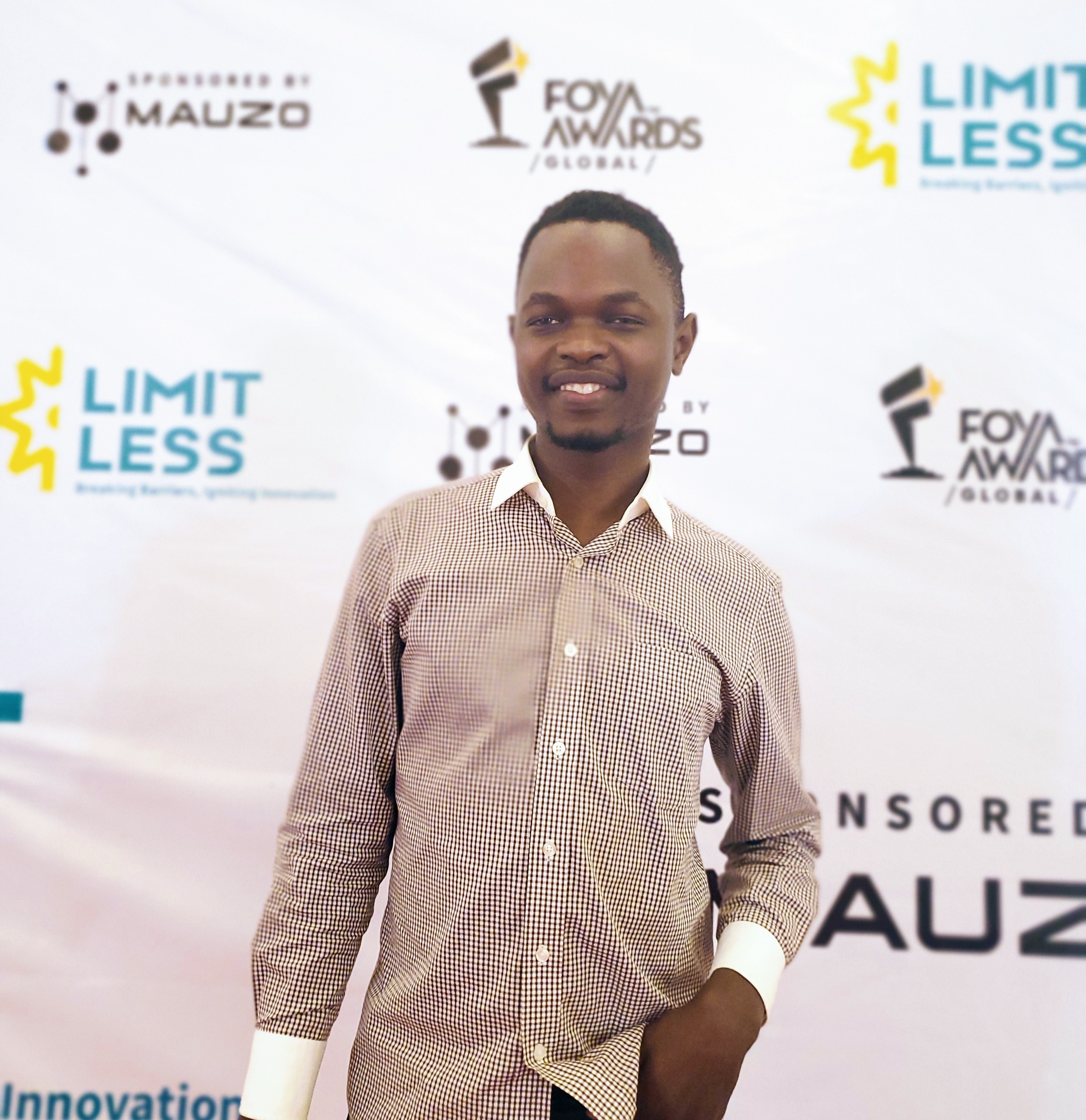
Oscar John
Oscar John
As a dedicated Software Engineer, I've collaborated with numerous startups, contributing to the development of exceptional and scalable products. My passion for software engineering drives me to consistently deliver high-quality solutions that meet and exceed the expectations of clients and end-users alike.