Mastering Solidity: Requirements and Custom Errors in Ethereum Contracts
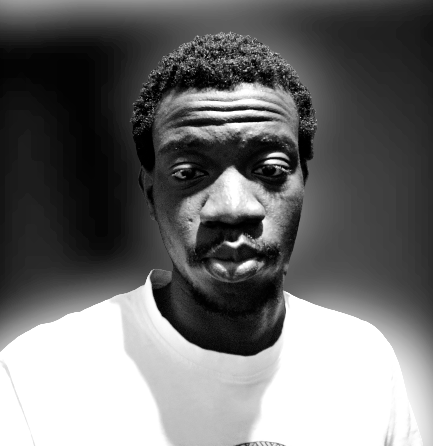
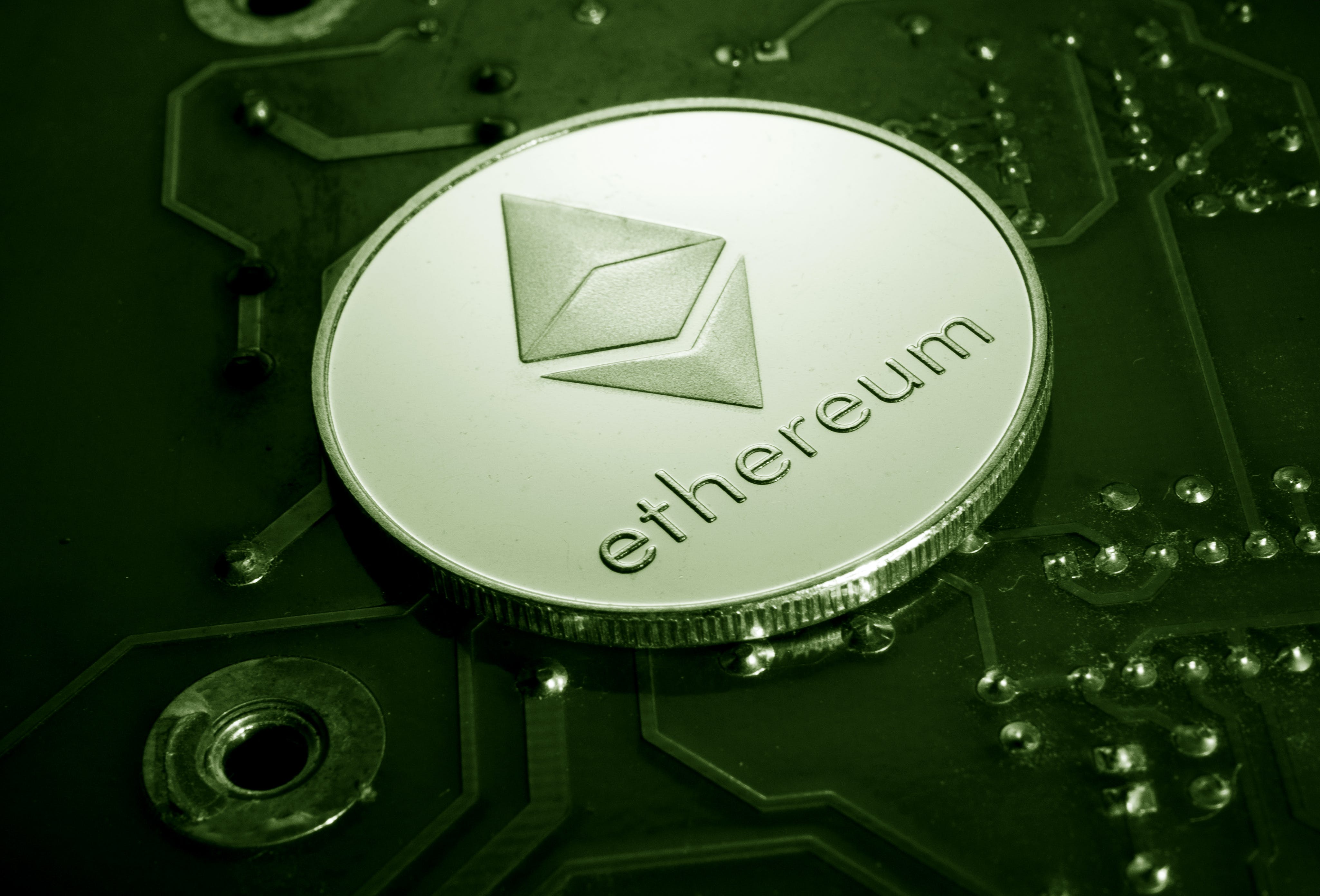
Introduction
Solidity is a high-level, object-oriented programming language for implementing smart contracts. Smart contracts are programs that govern the behaviour of accounts in the Ethereum network. Solidity is a curly bracket language designed to target the Ethereum Virtual Machine (EVM). Among the essential tools in a Solidity developer's arsenal are the require
statement and the ability to create custom errors.
In Solidity, the require
statement is used to validate conditions in smart contracts. The require
statement basic syntax involves checking a condition, and if the condition is false
, it reverts the transaction.
Custom errors are defined using the error
statement in Solidity. They can be used inside and outside of contracts (including interfaces and libraries). They help in debugging and understanding the reason for the revert during contract execution.
What is the require Statement in Solidity?
The require
statement is used in Solidity to ensure that certain conditions in smart contracts are met. It is often used to validate input or execution requests before executing additional code. If the condition provided to require
evaluates to false
, it reverts the transaction, and any state changes will be undone.
The general syntax for using the require
statement in Solidity is:
require(condition, errorMessage);
Below is an explanation of the code above:
condition:
This is the condition that needs to be true for the function to execute successfully. If the condition is false, the function will revert, and any state changes will be undone.errorMessage:
This is an optional error message that will be displayed if therequire
condition evaluates tofalse
. It helps provide context as to why the condition failed in our code.
Here's an example demonstrating the general syntax of the require
statement:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract RequireStatement {
function withdraw(uint amount) public {
require(amount > 0, "Amount should be greater than zero");
// Further code for withdrawal
// ...
}
}
In the code example above, the require
statement ensures that the amount
provided is greater than zero. If the condition (amount > 0
) is not met, the transaction will be reverted, and the error message "Amount should be greater than zero" will be displayed.
Best Practices for using the require Statement in Solidity
Using the require
statement in Solidity is essential for ensuring the integrity and security of your smart contracts. Here are some of the best practices for using the require
statement:
Provide Informative Error Messages: Include descriptive error messages to provide more context when a
require
condition fails. It helps developers and users understand why the function execution reverted, aiding in debugging.Keep Conditions Simple and Readable: Write clear and concise conditions that are easy to understand. Complex conditions might make the code harder to read and maintain.
Use
require
Moderately: Reverting a transaction can be costly, so it is important to use therequire
statement only when necessary.
Benefits of Using the require Statement in Solidity
Using the require
statement in Solidity offers several benefits that contribute to the security, integrity, and clarity of smart contract development. Some of the key benefits include:
Precondition Checks:
require
statement allows for the verification of input parameters or state conditions before executing critical parts of the code. This helps to ensure that the function or contract is operating under expected circumstances.Security Enhancement:
require
statements are important for preventing unwanted state changes and ensuring that the contract state remains consistent. They assist in guarding against unexpected or malicious behaviour that could compromise the integrity of smart contracts.Code Readability and Maintenance: By using
require
statements for precondition checks, the code becomes more readable and self-explanatory. It improves maintainability and makes the code easier to understand for developers, auditors, and anyone reviewing the code.
What are Custom Errors in Solidity?
Solidity v0.8.4 brought the introduction of custom errors. They allow developers to define their own custom error types and messages. They are used to provide more descriptive and specific information when something goes wrong during the execution of a function in a contract
Custom errors can be defined using the error
statement followed by a unique name for the error along with specific error messages or error codes to provide additional context about the error. Custom errors are more gas-efficient than using strings in error messages, and they can also be used to pass parameters to error messages.
In Solidity, you can define custom errors using the error
statement within the contract. Here's the syntax for defining custom errors in Solidity:
error MyCustomError(string message);
Below is an explanation of the code above:
The
error
statement in the code example is a keyword used to define custom errors in Solidity.The
MyCustomError
is the name given to the custom error. You can replace the nameMyCustomError
with your desired name.The
string message
specifies the type of parameter to be included with the error. This parameter can be used to pass information regarding the error.
Here's an example demonstrating the general syntax of the error
statement:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract CustomErrorExample {
error MyCustomError(uint value, string message);
function checkValue(uint _value) public {
if (_value < 10) {
revert MyCustomError(_value, "Value is too low");
}
// Additional code if the value is acceptable
}
}
In the code example above, the MyCustomError
error statement is defined to handle cases where a value is too low. Within the checkValue
function, if the provided _value
is less than 10, it will revert and display the error MyCustomError
, passing the _value
and the error message "Value is too low". This allows for a more descriptive and informative error message when the condition is not met.
Defining custom errors enables Solidity developers to create specific error types with additional information, aiding in better error handling and debugging within smart contracts.
Best Practices for Using Custom Errors in Solidity
When working with custom errors in Solidity, following these best practices is important to ensure clarity, security, and proper error handling in smart contracts. Here are some best practices for using custom errors:
Use Descriptive Names: Name your custom errors descriptively to easily indicate what happened. Also, you should include detailed explanations of the error in the error message.
Use Custom Errors to Represent Different Types of Errors. This will make your code easier to read and maintain. For example, you could have a custom error for insufficient funds, a custom error for unauthorized access, and a custom error for invalid input.
By following these best practices, you can use custom errors to write more secure, reliable, and efficient Solidity contracts.
Benefits of Using Custom Errors in Solidity
Using custom errors in Solidity offers several advantages and benefits for smart contract development and error handling. Some of the key benefits include:
Reduced Gas Costs: Custom errors save more gas than using strings in error messages. This is because the error message is encoded into the bytecode of the contract, so it does not need to be stored on the blockchain.
Improved Code Readability and Maintainability: Custom errors can make code more readable and maintainable by explicitly stating the assumptions that the code is making.
Conclusion
The require
statement is often used to check input parameters, verify certain conditions before executing critical parts of the code, or ensure the contract state is as expected before proceeding with further operations. This helps to prevent erroneous or malicious actions that could result in undesirable or inconsistent states of the blockchain.
Custom errors are useful for providing more meaningful feedback to users interacting with the contract, allowing them to understand why a transaction failed and providing more transparency in the smart contract's behaviour.
If you find this blog post helpful, please clap, share, and follow me to learn more about the blockchain network, Ethereum Virtual Machine, Solidity, and a whole lot more. Thank you for sticking around till the end, and see you on the next one.
Subscribe to my newsletter
Read articles from Wasiu Akindoyin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
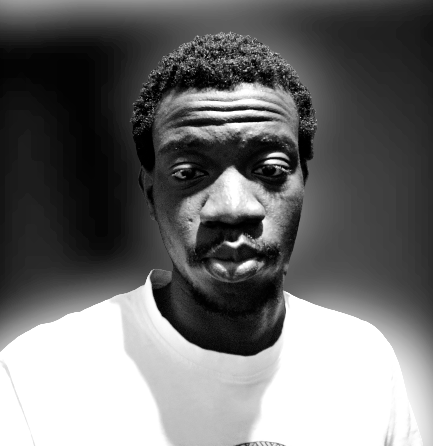