Day 8: Working with Lists (Part 1) in Python for DevOps
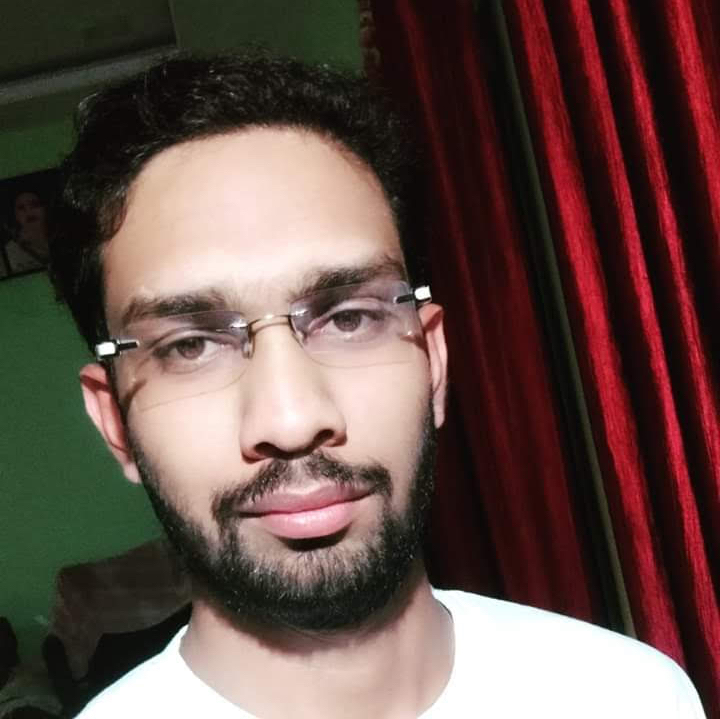

Welcome back to our Python for DevOps series! In today's edition, we'll embark on a journey into the realm of lists—an essential data structure for managing and manipulating collections of items in your DevOps scripts.
🔶 Understanding Lists and the List Data Structure 🔶
🔶 What are Lists?
In Python, a list is a versatile and dynamic data structure used to store an ordered collection of items. Lists can contain elements of different data types, making them ideal for various DevOps scenarios.
🔶 List Creation:
# Creating a list
my_list = [1, 2, 3, 'DevOps', True]
🔶 List Manipulation and Common Operations
🔶 Accessing List Elements:
# Accessing elements by index
first_element = my_list[0]
last_element = my_list[-1]
🔶 Modifying List Elements:
# Modifying elements
my_list[2] = 'Automation'
🔶 Adding Elements to a List:
# Appending an element to the end
my_list.append('Python')
🔶 Removing Elements from a List:
# Removing an element by value
my_list.remove(2)
# Removing an element by index
removed_element = my_list.pop(1)
🔶 List Slicing:
# Extracting a sublist
subset = my_list[0:3]
🔶 Common List Operations:
Finding the length of a list:
len(my_list)
Concatenating lists:
new_list = my_list + another_list
subset = my_list[0:3] print(subset) another_list =[ "hello", "Chandresh"] # new list created new_list = [my_list , " ", another_list] #Concatenated print(new_list)
🔶 What are tuples?
A tuple in Python is an immutable, ordered collection of elements. "Immutable" means that once a tuple is created, you cannot modify its contents – you cannot add, remove, or change elements. "Ordered" implies that the elements have a specific order, and this order is maintained throughout the tuple's existence.
Tuples are created using parentheses ()
and can contain elements of different data types, including numbers, strings, and other tuples. Here's a basic syntax for creating a tuple:
my_tuple = (1, "hello", 3.14)
You can also create an empty tuple:
empty_tuple = ()
To create a tuple with a single element, you need to include a trailing comma:
single_element_tuple = (42,)
You can access elements in a tuple using indexing, similar to lists:
print(my_tuple[0]) # Output: 1
print(my_tuple[1]) # Output: hello
Since tuples are immutable, you cannot modify, add, or remove elements after the tuple is created. This immutability provides a level of data integrity and makes tuples useful in situations where the data should remain constant.
🔶 Practice Exercises and Examples
Example: Managing a List of User Accounts in a DevOps Script
# DevOps script to manage user accounts
# Create an empty list to store user accounts
user_accounts = []
# Add user accounts
user_accounts.append('user1')
user_accounts.append('user2')
user_accounts.append('user3')
# Display the current user accounts
print("Current user accounts:", user_accounts)
# Remove a user account
user_to_remove = 'user2'
if user_to_remove in user_accounts:
user_accounts.remove(user_to_remove)
print(f"User account '{user_to_remove}' removed.")
else:
print(f"User account '{user_to_remove}' not found.")
# Update a user account
user_to_update = 'user1'
new_username = 'new_user1'
if user_to_update in user_accounts:
index = user_accounts.index(user_to_update)
user_accounts[index] = new_username
print(f"User account '{user_to_update}' updated to '{new_username}'.")
else:
print(f"User account '{user_to_update}' not found.")
# Display the final user accounts
print("Final user accounts:", user_accounts)
🔶 Conclusion
Lists are powerful tools for managing collections of data in your DevOps scripts. With their flexibility and range of operations, lists enable you to build dynamic and efficient automation solutions. Stay tuned for Part 2 of Working with Lists, where we'll explore more advanced list operations.
Note: I am following Abhishek Verraamalla's YouTube playlist for learning.
GitHub Repo: https://github.com/Chandreshpatle28/python-for-devops-av
Happy Learning :)
Stay in the loop with my latest insights and articles on cloud ☁️ and DevOps ♾️ by following me on Hashnode, LinkedIn (https://www.linkedin.com/in/chandreshpatle28/), and GitHub (https://github.com/Chandreshpatle28).
Thank you for reading! Your support means the world to me. Let's keep learning, growing, and making a positive impact in the tech world together.
#Git #Linux Devops #Devopscommunity #PythonforDevOps #python
Subscribe to my newsletter
Read articles from CHANDRESH PATLE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
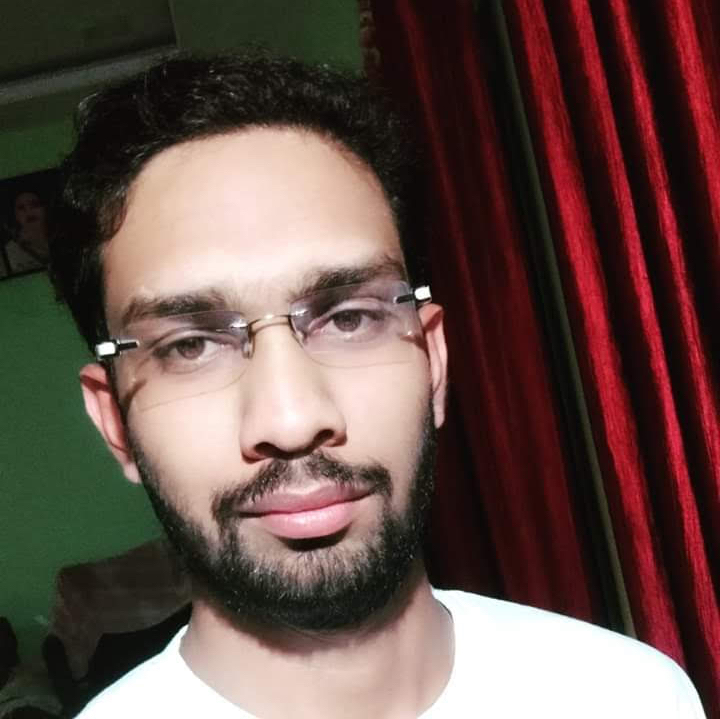
CHANDRESH PATLE
CHANDRESH PATLE
Hi, I'm Chandresh Patle, an aspiring DevOps Engineer with a diverse background in field supervision, manufacturing, and service consulting. With a strong foundation in engineering and project management, I bring a unique perspective to my work. I recently completed a Post Graduate Diploma in Advanced Computing (PG-DAC), where I honed my skills in web development, frontend and backend technologies, databases, and DevOps practices. My proficiency extends to Core Java, Oracle, MySQL, SDLC, AWS, Docker, Kubernetes, Ansible, Linux, GitHub, Terraform, Grafana, Selenium, and Jira. I am passionate about leveraging technology to drive efficient and reliable software delivery. With a focus on DevOps principles and automation, I strive to optimize workflows and enhance collaboration among teams. I am constantly seeking new opportunities to expand my knowledge and stay up-to-date with the latest industry trends. If you have any questions, collaboration ideas, or professional opportunities, feel free to reach out to me at patle269@gmail.com. I'm always open to connecting with fellow tech enthusiasts and exploring ways to contribute to the DevOps community. Let's build a better future through innovation and continuous improvement!