MIME stands for "Multipurpose Internet Mail Extensions.

Table of contents
- MIME stands for "Multipurpose Internet Mail Extensions.
- 1. Understand the Basics:
- 2. Study MIME Types:
- 3. Explore MIME Headers:
- 4. Learn MIME in Emails:
- 5. HTTP Content Negotiation:
- 6. File Uploads and MIME Validation:
- 7. Security Considerations:
- 8. Implement MIME Handling in Programming Languages:
- 9. Stay Updated:
- 10. Real-world Applications:
- 1. Email Systems:
- 2. Web Development - File Uploads:
- 3. Content Management Systems (CMS):
- 4. APIs and Web Services:
- 5. Rich Text Editors:
- 6. Web Browsers - Content Rendering:
- 7. Content Delivery Networks (CDN):
- 8. Document Management Systems:
- 9. Digital Signatures and Encryption:
- 10. Media Streaming Services:
- 11. Community Participation:
- 1. Join Online Forums and Groups:
- 2. Engage on Social Media:
- 3. Participate in Mailing Lists:
- 4. Contribute to Open Source Projects:
- 5. Attend Meetups and Conferences:
- 6. Write Blog Posts or Tutorials:
- 7. Create and Share Resources:
- 8. Teach or Mentor:
- 9. Ask Questions and Seek Guidance:
- 10. Collaborate on Research:
- 11. Contribute to Standards Bodies:
- 12. Organize Community Events:
- 13. Provide Feedback to Developers:
- 12. Read RFCs:
- 1. Identify Relevant RFCs:
- 2. Understand the Structure:
- 3. Read the Abstract and Introduction:
- 4. Focus on Key Concepts:
- 5. Pay Attention to Syntax and Semantics:
- 6. Explore Examples:
- 7. Follow References:
- 8. Take Notes:
- 9. Join Discussions:
- 10. Apply Knowledge:
- 11. Stay Updated:
- 12. Combine with Practical Experience:
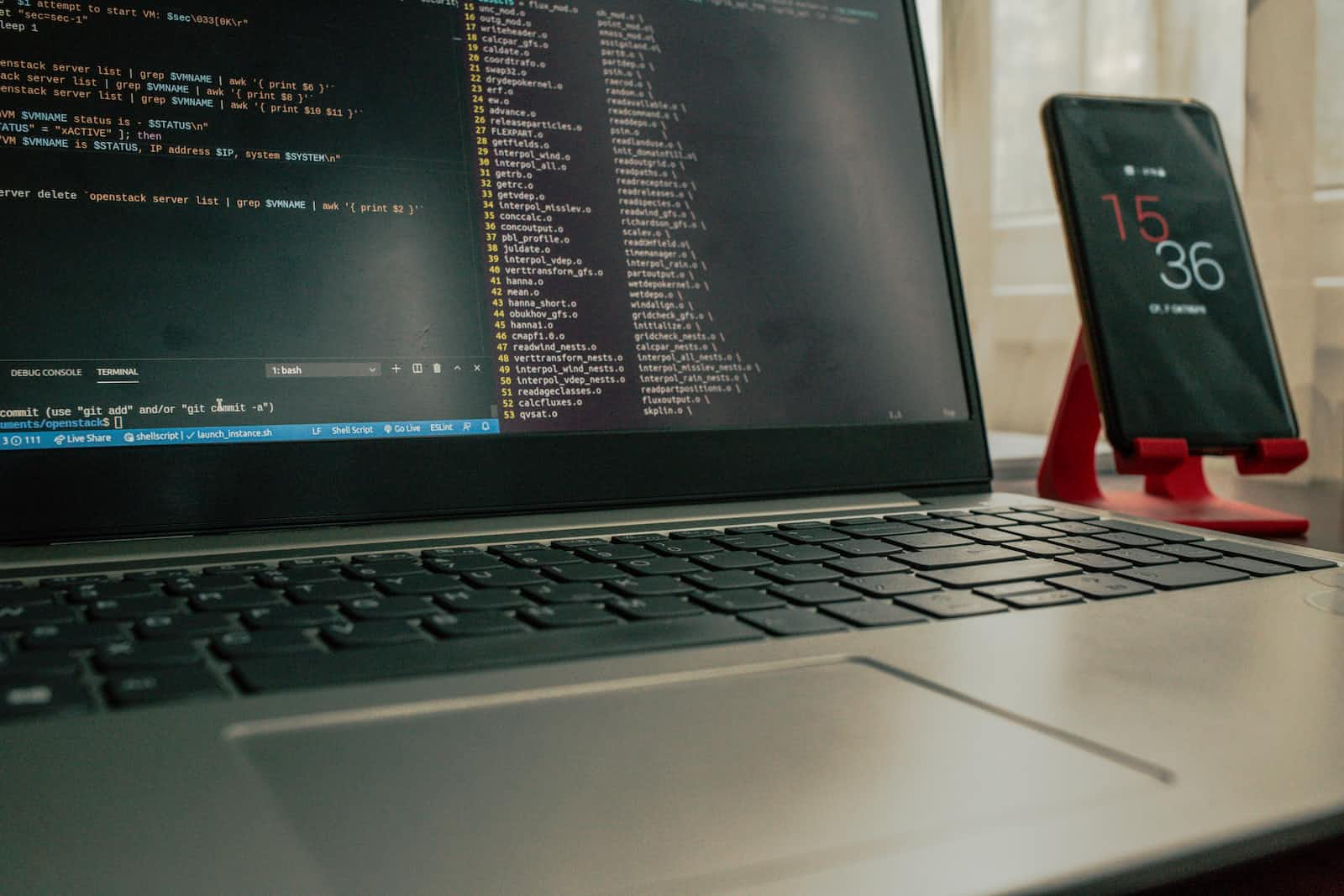
MIME stands for "Multipurpose Internet Mail Extensions.
MIME stands for "Multipurpose Internet Mail Extensions." MIME is a standard that extends the format of email messages to support text in character sets other than ASCII, as well as attachments of audio, video, images, and application programs. It was originally developed to extend the capabilities of email for sending multimedia content and non-text attachments.
In the context of web development and file uploads, MIME types are used to identify the type of content that a file contains. Each MIME type is associated with a unique identifier, and it helps browsers and servers understand how to handle and interpret different types of files. For example, common MIME types include "text/plain" for plain text files, "image/jpeg" for JPEG images, "application/pdf" for PDF documents, and so on.
When you upload a file through a web form, the browser sends information about the file's MIME type along with the file data. This information is crucial for servers to validate the type of file being uploaded and to take appropriate actions, such as allowing or rejecting the upload, based on security and application requirements.
1. Understand the Basics:
Understanding the Basics of MIME (Multipurpose Internet Mail Extensions):
1. MIME Overview:
- Purpose: MIME is a standard that enhances email messages' format to handle various types of content beyond simple ASCII text. It allows emails to support character sets other than ASCII and facilitates the inclusion of multimedia attachments such as audio, video, images, and even application programs.
2. MIME in Email:
Character Set Support: Before MIME, emails were limited to ASCII characters. MIME allows emails to support a wide range of character sets, enabling the exchange of messages in languages with non-ASCII characters, such as accented characters in European languages.
Multimedia Attachments: MIME introduces a mechanism for attaching multimedia content to email messages. This includes images, audio files, video files, and even binary application data. Each type of content is associated with a specific MIME type.
Multipart Messages: MIME introduces the concept of multipart messages, allowing different content types to coexist within a single email. For example, an email can contain both plain text and an attached image.
3. MIME in HTTP:
Media Type Definition: MIME is also used in the HTTP protocol to define media types or content types. This is crucial for web communication, ensuring that servers and clients understand the type of data being exchanged.
Content-Type Header: In HTTP, the
Content-Type
header is used to specify the MIME type of the data being sent in the response. This header informs the client about how to interpret and display the received data.
4. Facilitating Data Exchange:
Universal Standard: MIME provides a universal standard for encoding and exchanging different types of data on the internet. Whether it's sending an email with attachments or delivering multimedia content via HTTP, MIME ensures a standardized approach to data representation.
Extensibility: MIME is extensible, allowing for the inclusion of new content types as technologies evolve. This flexibility is crucial for adapting to the changing landscape of internet communication.
5. Practical Example in PHP (Email):
$to = 'recipient@example.com';
$subject = 'Birthday Greeting';
$message = 'Happy Birthday! 🎉';
$headers = "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: text/plain; charset=utf-8\r\n";
mail($to, $subject, $message, $headers);
This PHP example creates a simple plain text email but utilizes MIME headers (MIME-Version
and Content-Type
) to specify the character set and content type.
6. Practical Example in HTTP (PHP):
// PHP script serving an image with MIME type specified
$imagePath = 'path/to/image.jpg';
header('Content-Type: image/jpeg');
readfile($imagePath);
In this PHP example, the server sends an image in the response with the appropriate MIME type specified in the Content-Type
header.
Conclusion:
Understanding the basics of MIME is crucial for anyone involved in internet communication, whether it's sending diverse email content or serving various media types over HTTP. MIME's role in defining standards for data exchange contributes to the interoperability and richness of content on the internet.
2. Study MIME Types:
Let's delve into the study of MIME types with explanations and examples in both PHP and JavaScript.
MIME Types Structure:
MIME types are a way to describe the nature and format of a file or content on the internet. They are structured as "type/subtype" and are used to indicate the type of data being sent. The "type" represents the general category, and the "subtype" provides more specific information about the data.
Examples of Common MIME Types:
text/plain:
- Represents plain text data without any specific formatting. It's commonly used for simple text files.
PHP Example:
$mime_type = 'text/plain';
JavaScript Example:
// JavaScript doesn't have direct MIME type declarations, but in a server environment (Node.js), you might use:
const mimeType = 'text/plain';
image/jpeg:
- Represents JPEG image files. This MIME type is used for images with the JPEG format.
PHP Example:
$mime_type = 'image/jpeg';
JavaScript Example:
// JavaScript doesn't have direct MIME type declarations, but in a server environment (Node.js), you might use:
const mimeType = 'image/jpeg';
application/json:
- Represents JSON (JavaScript Object Notation) data. It's commonly used for transmitting data between a server and a web application.
PHP Example:
$mime_type = 'application/json';
JavaScript Example:
// JavaScript doesn't have direct MIME type declarations, but in a server environment (Node.js), you might use:
const mimeType = 'application/json';
Why MIME Types are Important:
MIME types play a crucial role in communication between clients and servers. When a client requests a resource (e.g., a file) from a server, the server responds with the appropriate MIME type in the Content-Type header. This informs the client about the type of content it is receiving, allowing it to interpret and render the data correctly.
Setting MIME Types in HTTP Headers:
In both PHP and JavaScript (Node.js), you may encounter scenarios where you need to set MIME types in HTTP headers. This is commonly done when serving files or generating responses dynamically.
Setting MIME Type in PHP:
header("Content-Type: image/jpeg");
Setting MIME Type in JavaScript (Node.js):
// In a Node.js environment, using the Express framework as an example
app.get('/image', (req, res) => {
res.header('Content-Type', 'image/jpeg');
// Other response handling here
});
MIME Type Detection:
In some cases, you might need to determine the MIME type of a file dynamically, especially when handling file uploads.
PHP Example:
$file_path = 'path/to/file.jpg';
$mime_type = mime_content_type($file_path);
JavaScript Example:
// JavaScript doesn't have a built-in function like PHP's mime_content_type.
// In a Node.js environment, you might use a library like 'mime-types.'
const mime = require('mime-types');
const file_path = 'path/to/file.jpg';
const mime_type = mime.contentType(file_path);
Understanding MIME types is fundamental for web development, ensuring that data is interpreted correctly across different platforms and applications. Whether you are working with static files, dynamic responses, or file uploads, being familiar with MIME types is essential for effective communication on the web.
3. Explore MIME Headers:
Exploring MIME Headers:
**1. Content-Type Header in Email (PHP Example):
- In email, the
Content-Type
header specifies the type of content being sent. It includes information about the character set and the type of data.
$to = 'recipient@example.com';
$subject = 'Important Document';
$message = 'Please find the attached document.';
$headers = "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: application/pdf; charset=utf-8\r\n";
$headers .= "Content-Disposition: attachment; filename=important_document.pdf\r\n";
// Attach the PDF file
$attachment = file_get_contents('path/to/important_document.pdf');
mail($to, $subject, $message, $headers, $attachment);
In this PHP example, the Content-Type
header indicates that the email contains a PDF attachment (application/pdf
). The Content-Disposition
header specifies that the attachment should be treated as a file, and the filename is provided.
2. Content-Type Header in HTTP (PHP Example):
- In HTTP, the
Content-Type
header is crucial for indicating the type of data being sent in the response. It ensures that the client interprets the data correctly.
// PHP script serving JSON data with the appropriate Content-Type header
$data = ['message' => 'Hello, world!'];
header('Content-Type: application/json');
echo json_encode($data);
In this PHP example, the server sends JSON data with the Content-Type
header set to application/json
. This informs the client that the response body contains JSON-encoded data.
3. Content-Disposition Header (Email):
- In email, the
Content-Disposition
header is often used to specify how the content should be handled by the recipient's email client. It can indicate whether the content is an attachment or inline.
$headers .= "Content-Disposition: inline; filename=inline_image.png\r\n";
In this PHP example, the Content-Disposition
header indicates that the attached image should be displayed inline (e.g., embedded in the email) and provides the filename.
4. Handling MIME Headers in JavaScript (Node.js):
- In a Node.js environment, you might handle MIME headers when serving content using a web framework like Express.
const express = require('express');
const app = express();
// Handling JSON response
app.get('/data', (req, res) => {
const data = { message: 'Hello, world!' };
res.setHeader('Content-Type', 'application/json');
res.send(JSON.stringify(data));
});
// Serving an image with specified MIME type
app.get('/image', (req, res) => {
const imagePath = 'path/to/image.jpg';
res.setHeader('Content-Type', 'image/jpeg');
res.sendFile(imagePath);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this Node.js example, the Express framework is used to handle routes, and res.setHeader
is used to set the Content-Type
header based on the type of content being served.
5. Importance of MIME Headers:
MIME headers are crucial for ensuring proper communication between servers and clients. They provide essential information about the type and handling of the content, allowing recipients to interpret and display data accurately.
Properly setting MIME headers is especially important in scenarios where content types vary, such as serving different file types over HTTP or attaching various types of files in emails.
Understanding and correctly using MIME headers contribute to the seamless exchange and interpretation of diverse content on the internet, enhancing the overall user experience.
4. Learn MIME in Emails:
Learning MIME in Emails:
1. MIME Multipart Email Example (PHP):
- MIME allows emails to contain multiple parts, each with its own content type. A common use case is sending both plain text and HTML versions of an email.
$to = 'recipient@example.com';
$subject = 'MIME Multipart Email';
// Define the boundary for separating different parts
$boundary = uniqid('boundary');
// Email headers
$headers = "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: multipart/alternative; boundary=\"$boundary\"\r\n";
// Plain text version
$textMessage = "Hello, this is the plain text version of the email.";
$textPart = "--$boundary\r\n";
$textPart .= "Content-Type: text/plain; charset=utf-8\r\n";
$textPart .= "\r\n$textMessage\r\n";
// HTML version
$htmlMessage = "<p>Hello, this is the <strong>HTML</strong> version of the email.</p>";
$htmlPart = "--$boundary\r\n";
$htmlPart .= "Content-Type: text/html; charset=utf-8\r\n";
$htmlPart .= "\r\n$htmlMessage\r\n";
// Combine parts
$emailBody = "$textPart$htmlPart--$boundary--";
// Send the email
mail($to, $subject, $emailBody, $headers);
In this PHP example, a MIME multipart email is created with both plain text and HTML versions. The Content-Type
header indicates that it is a multipart/alternative message, and each part is separated by a unique boundary.
2. Handling MIME Multipart Emails in JavaScript (Node.js):
- In a Node.js environment, handling MIME multipart emails can be achieved using libraries like
nodemailer
.
const nodemailer = require('nodemailer');
// Create a transporter
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your-email@gmail.com',
pass: 'your-password'
}
});
// Define email content
const mailOptions = {
from: 'your-email@gmail.com',
to: 'recipient@example.com',
subject: 'MIME Multipart Email',
text: 'Hello, this is the plain text version of the email.',
html: '<p>Hello, this is the <strong>HTML</strong> version of the email.</p>'
};
// Send the email
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.error(error);
} else {
console.log('Email sent: ' + info.response);
}
});
This Node.js example uses the nodemailer
library to create a MIME multipart email with both plain text and HTML versions.
3. Key Concepts:
MIME-Version Header: Specifies the MIME version being used. Commonly set to "1.0" for compatibility.
Content-Type Header: In a multipart email, the
Content-Type
header is crucial. It specifies that the email is multipart and defines the boundary that separates different parts.Boundary: A unique string that marks the beginning and end of each part in a multipart email. It should not appear in the content of the parts.
Content-Disposition Header: Specifies how the content should be displayed or processed. For email attachments, it may indicate that the content should be treated as an attachment.
Content-Transfer-Encoding Header: Specifies the encoding method used for transferring data. Common values include "7bit" and "base64."
4. Practical Use Case:
- MIME multipart emails are commonly used when you want to provide both a plain text version (for simplicity) and an HTML version (for richer formatting) to accommodate a variety of email clients.
Understanding MIME in emails allows you to create versatile and well-structured emails that can include different types of content to cater to various client capabilities.
5. HTTP Content Negotiation:
HTTP Content Negotiation with MIME Types:
1. Content Negotiation in HTTP (PHP):
- Content negotiation allows a client to specify the desired content type in the
Accept
header of an HTTP request. The server can then respond with the appropriate representation based on what the client can accept.
// Extracting Accept header from the HTTP request
$acceptHeader = $_SERVER['HTTP_ACCEPT'];
// Checking if the client accepts JSON
if (strpos($acceptHeader, 'application/json') !== false) {
$data = ['message' => 'This is a JSON response'];
header('Content-Type: application/json');
echo json_encode($data);
} else {
// If JSON is not accepted, provide an HTML response
echo '<p>This is an HTML response</p>';
}
In this PHP example, the server checks the Accept
header to determine if the client prefers JSON (application/json
). If so, it responds with a JSON-encoded message; otherwise, it provides an HTML response.
2. Content Negotiation in JavaScript (AJAX):
- In JavaScript, content negotiation is often used in AJAX requests to indicate the desired content type.
// AJAX request with content negotiation
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data');
// Set the Accept header to indicate JSON preference
xhr.setRequestHeader('Accept', 'application/json');
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE) {
if (xhr.status === 200) {
console.log(JSON.parse(xhr.responseText));
}
}
};
xhr.send();
In this JavaScript example, an AJAX request is made to a hypothetical API (https://api.example.com/data
). The Accept
header is set to indicate that the client prefers JSON. The server can use this information to send an appropriate response.
3. Importance of Content Negotiation:
Flexible Responses: Content negotiation allows the server to respond with different representations of the same resource based on the client's preferences. For example, it can provide JSON or XML data based on what the client can accept.
Efficient Resource Usage: By tailoring responses to the client's needs, content negotiation helps in optimizing bandwidth usage and reducing unnecessary data transfer.
Improved User Experience: Clients can receive data in a format they can process efficiently, enhancing the overall user experience. For instance, a web browser may prefer HTML for rendering, while an API client may prefer JSON for easy parsing.
4. Key Concepts:
Accept Header: The
Accept
header in an HTTP request specifies the content types that the client can understand. It can include multiple types, weighted with a quality value indicating preference.Content-Type Header: In the server's response, the
Content-Type
header indicates the type of data being sent. It allows the client to interpret and display the content correctly.Quality Values: Quality values (q-values) in the
Accept
header indicate the client's preference for a particular content type. For example,application/json;q=0.8
means JSON is accepted with a preference of 80%.
5. Practical Use Case:
- Content negotiation is often used in RESTful APIs where clients, such as web browsers or mobile apps, may request data in different formats (JSON, XML) based on their capabilities and requirements.
Understanding and implementing content negotiation ensure that web servers can provide the most suitable representation of resources based on client preferences, promoting efficient and flexible communication between clients and servers.
6. File Uploads and MIME Validation:
Let's go through examples of file uploads and MIME validation using PHP and Express.js. In these examples, we'll focus on a basic image upload scenario, and the MIME validation will ensure that only JPEG and PNG images are accepted.
PHP File Upload and MIME Validation:
<?php
$allowedMimeTypes = ['image/jpeg', 'image/png'];
$uploadDirectory = 'uploads/';
if ($_FILES['file']['error'] === UPLOAD_ERR_OK) {
$uploadedFile = $_FILES['file'];
$fileMimeType = mime_content_type($uploadedFile['tmp_name']);
if (in_array($fileMimeType, $allowedMimeTypes)) {
// Valid MIME type, move the file to the desired location
move_uploaded_file($uploadedFile['tmp_name'], $uploadDirectory . $uploadedFile['name']);
echo 'File uploaded successfully.';
} else {
// Invalid MIME type, reject the file
echo 'Invalid file type. Please upload a JPEG or PNG image.';
}
}
?>
In this PHP example:
$allowedMimeTypes
specifies the allowed MIME types (JPEG and PNG).mime_content_type
is used to determine the MIME type of the uploaded file.If the MIME type is valid, the file is moved to the
uploads/
directory; otherwise, an error message is displayed.
Express.js File Upload and MIME Validation:
const express = require('express');
const multer = require('multer');
const upload = multer({ dest: 'uploads/' });
const app = express();
const allowedMimeTypes = ['image/jpeg', 'image/png'];
app.post('/upload', upload.single('file'), (req, res) => {
const uploadedFile = req.file;
if (allowedMimeTypes.includes(uploadedFile.mimetype)) {
// Valid MIME type, move the file to the desired location
res.send('File uploaded successfully.');
} else {
// Invalid MIME type, reject the file
res.status(400).send('Invalid file type. Please upload a JPEG or PNG image.');
}
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
In this Express.js example:
Multer is used for handling file uploads.
The
upload.single('file')
middleware is used to process a single file upload with the name 'file'.The
allowedMimeTypes
array specifies the allowed MIME types (JPEG and PNG).If the uploaded file has a valid MIME type, the file is considered valid; otherwise, a 400 Bad Request response is sent.
Important Notes:
Always implement server-side validation for security. Client-side validation can enhance user experience but should not be solely relied upon.
The PHP and Express.js examples are meant to handle file uploads on the server side. Make sure your HTML form or client-side application interacts correctly with these server-side scripts.
These examples showcase the basic concept of handling file uploads and performing MIME type validation in both PHP and Express.js. Always remember to implement robust server-side validation for security considerations.
7. Security Considerations:
Security Considerations in MIME Handling:
Handling MIME types correctly is crucial for web security. Incorrect MIME type handling can lead to security vulnerabilities, such as Cross-Site Scripting (XSS) attacks. Here are some security considerations and best practices:
1. MIME-Based Attacks:
- XSS Attacks: Incorrectly handling or trusting user-supplied MIME types can lead to XSS attacks. For example, an attacker might upload a file with a manipulated MIME type that gets processed as HTML, allowing them to inject malicious scripts into the web application.
2. File Upload Security:
Validate MIME Types: When allowing file uploads, always validate and verify that the uploaded file's MIME type matches the expected content type. This helps prevent attackers from uploading files with malicious content.
Use Whitelists: Instead of checking for disallowed MIME types, use whitelists of allowed MIME types. This approach is more secure as it explicitly defines the types that are permitted.
Server-Side Validation: Perform MIME type validation on the server side. Client-side validation can be bypassed, so server-side validation is crucial for security.
3. Content-Disposition Header:
- Be Cautious with Inline Content: When using the
Content-Disposition
header in emails or HTTP responses, be cautious with inline content. Ensure that user-supplied content is sanitized to prevent potential security issues.
4. Sanitize User Input:
- Escape and Sanitize: When dealing with user input, especially if it affects the generation of dynamic content, always escape and sanitize the input to prevent XSS attacks. Use libraries or frameworks that provide secure escaping functions.
5. Implement a Content Security Policy (CSP):
- Use Content Security Policy: Implement a Content Security Policy (CSP) on your web application. A CSP helps mitigate the risk of XSS attacks by defining which content sources are allowed, including restrictions on script execution.
6. Keep Software Updated:
- Regularly Update Libraries and Frameworks: Ensure that you are using the latest versions of libraries and frameworks that handle MIME types. Developers often release updates to address security vulnerabilities.
7. Educate Developers and Users:
- Training and Awareness: Educate developers about the importance of secure MIME type handling and the potential risks associated with incorrect handling. Users should also be aware of safe file upload practices.
8. Logging and Monitoring:
- Log and Monitor Activities: Implement logging and monitoring mechanisms to detect and investigate any suspicious activities related to MIME types. This helps in identifying potential security incidents.
9. Regular Security Audits:
- Conduct Security Audits: Regularly conduct security audits of your web application to identify and address potential vulnerabilities, including issues related to MIME type handling.
10. Collaborate with the Security Community:
- Engage with the Security Community: Stay informed about the latest security best practices and vulnerabilities related to MIME types. Engage with the security community to learn from experiences and share insights.
By following these security considerations and best practices, you can enhance the security of your web application and mitigate the risks associated with incorrect MIME type handling. Always prioritize security in the development and maintenance of web applications.
8. Implement MIME Handling in Programming Languages:
To implement MIME handling in programming languages, let's explore how you can work with MIME types in both PHP and JavaScript. We'll cover basic scenarios such as creating and parsing MIME messages, as well as handling MIME types in HTTP headers.
PHP - Creating and Parsing MIME Messages:
1. Creating a MIME Message:
<?php
// Create a simple MIME message with text/plain content
$to = 'recipient@example.com';
$subject = 'Hello MIME!';
$message = 'This is a MIME message.';
// MIME headers
$headers = "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: text/plain; charset=utf-8\r\n";
// Send the email
mail($to, $subject, $message, $headers);
?>
In this PHP example, a basic MIME message is created with a text/plain
content type. The MIME-Version
and Content-Type
headers are included.
2. Parsing a MIME Message:
<?php
// Assume $rawMimeMessage contains the raw MIME message
$rawMimeMessage = file_get_contents('example.eml');
// Parse the MIME message
$mimeParser = new MimeMailParser();
$mimeParser->setText($rawMimeMessage);
// Get parts of the MIME message
$parts = $mimeParser->getParts();
// Access individual parts
foreach ($parts as $part) {
echo "Content Type: " . $part->getContentType() . "\n";
echo "Content:\n" . $part->getRawContent() . "\n";
}
?>
In this example, we use a library like MimeMailParser
to parse a raw MIME message and extract its parts. The library provides methods to access various attributes of each part, including content type and raw content.
JavaScript (Node.js) - Handling MIME Types in HTTP:
1. Setting MIME Types in HTTP Headers:
const http = require('http');
const server = http.createServer((req, res) => {
// Set the Content-Type header based on file extension
const filePath = 'path/to/file.html';
const fileExtension = filePath.split('.').pop();
const mimeTypes = {
'html': 'text/html',
'css': 'text/css',
'js': 'application/javascript',
'png': 'image/png',
'jpg': 'image/jpeg',
};
const contentType = mimeTypes[fileExtension] || 'application/octet-stream';
res.setHeader('Content-Type', contentType);
// Read and send the file
const fs = require('fs');
fs.readFile(filePath, (err, data) => {
if (err) {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
} else {
res.writeHead(200);
res.end(data);
}
});
});
const port = 3000;
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
In this Node.js example, the server sets the Content-Type
header based on the file extension when serving files. This is a basic example, and in a production environment, you might use a library like mime
for more accurate MIME type determination.
2. Parsing MIME Types from HTTP Requests:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.text({ type: '*/*' }));
app.post('/process-mime', (req, res) => {
const rawMimeMessage = req.body;
// Parse the MIME message
// Use a library like 'mailparser' for more advanced parsing
console.log('Received MIME message:', rawMimeMessage);
res.send('MIME message received.');
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
In this Express.js example, the server receives a raw MIME message in the HTTP request body. This is a basic illustration, and for more advanced parsing, you might use a library like mailparser
.
Remember to install necessary libraries using tools like Composer (for PHP) or npm (for Node.js) before running the examples. Additionally, always consider security best practices, especially when dealing with user inputs or handling files.
9. Stay Updated:
Staying Updated on MIME Standards:
As with any internet standard, staying informed about updates and changes to MIME (Multipurpose Internet Mail Extensions) is crucial. The Internet Assigned Numbers Authority (IANA) is a primary source for MIME-related information. Here's how you can stay updated:
1. Check IANA Website:
Website: IANA MIME Media Types
IANA maintains the official registry of MIME media types. Regularly check their website for updates, new registrations, and changes to existing media types.
2. Review RFC Documents:
Read and review the relevant Request for Comments (RFC) documents that define MIME standards. These documents provide in-depth details about MIME and any updates to the standard.
3. Subscribe to Mailing Lists:
- IETF MIME-related Mailing Lists: The Internet Engineering Task Force (IETF) discusses MIME-related topics. Subscribing to relevant mailing lists allows you to stay informed about ongoing discussions, proposed changes, and updates.
4. Follow Industry Blogs and Forums:
- Blogs and Forums: Follow industry blogs, forums, and discussion groups related to internet standards and MIME. Engaging with the community can provide insights into real-world challenges and solutions.
5. Monitor Updates in Programming Languages:
- PHP and JavaScript Documentation: Check the official documentation of programming languages like PHP and JavaScript for any updates related to MIME handling. Language updates may include improvements or changes in MIME-related functionalities.
6. Join Online Communities:
- Stack Overflow, Reddit, and Other Platforms: Join online communities like Stack Overflow and Reddit, where developers often discuss MIME-related issues and solutions. Participate in discussions, ask questions, and share your experiences.
7. Follow MIME-related News:
- Tech News Outlets: Stay informed about MIME updates through technology news outlets. Articles and news updates may highlight significant changes or advancements in MIME standards.
8. Subscribe to Newsletters:
- Subscribe to Newsletters: Subscribe to newsletters from organizations, websites, or individuals who specialize in internet standards and protocols. Newsletters often provide curated content and updates.
9. Attend Conferences and Webinars:
- Industry Conferences: Attend conferences and webinars focused on internet standards, protocols, and web development. These events often feature discussions on MIME and related technologies.
10. Follow Official Repositories:
- GitHub and GitLab Repositories: Follow official repositories on platforms like GitHub or GitLab that are related to MIME handling in programming languages. Developers often contribute to and discuss improvements in these repositories.
By actively engaging with these sources and staying involved in the community, you'll be well-equipped to adapt to changes in MIME standards and ensure that your knowledge and practices remain up-to-date. Keeping abreast of developments in internet standards is fundamental for maintaining the security and efficiency of web applications.
10. Real-world Applications:
Real-world applications of MIME (Multipurpose Internet Mail Extensions) span a wide range of scenarios, given its role in facilitating the exchange of different types of data on the internet. Let's explore some practical applications where MIME is crucial:
1. Email Systems:
Scenario: MIME is extensively used in email systems to enable the exchange of multimedia content.
Implementation: MIME allows emails to include attachments, inline images, and formatted text. For example, an email can have both plain text and HTML versions.
2. Web Development - File Uploads:
Scenario: Websites that allow users to upload files need to handle MIME types to ensure the expected content.
Implementation: Web servers often perform MIME validation when users upload files. This helps in preventing the upload of malicious or inappropriate content.
3. Content Management Systems (CMS):
Scenario: CMS platforms handling diverse types of media content need to manage and serve content with appropriate MIME types.
Implementation: When serving media files, CMS systems set the correct MIME types in HTTP headers to ensure proper rendering in browsers.
4. APIs and Web Services:
Scenario: APIs and web services exchanging data between different systems need to agree on content types.
Implementation: HTTP headers, including
Content-Type
andAccept
, are crucial for content negotiation. MIME types specify the format of data being sent or expected.
5. Rich Text Editors:
Scenario: Applications providing rich text editing capabilities need to handle various content types.
Implementation: MIME types play a role in specifying the format of content within the editor. For instance, distinguishing between plain text and formatted HTML.
6. Web Browsers - Content Rendering:
Scenario: Browsers need to interpret and render content received from web servers correctly.
Implementation: The
Content-Type
header in HTTP responses guides browsers on how to handle and display the received content. Incorrect MIME types may lead to rendering issues.
7. Content Delivery Networks (CDN):
Scenario: CDNs distributing content globally need to ensure proper handling of diverse media types.
Implementation: CDNs set appropriate MIME types to optimize content delivery. For example, images may have different MIME types like
image/jpeg
orimage/png
.
8. Document Management Systems:
Scenario: Systems managing documents of various formats need to handle MIME types for proper rendering and processing.
Implementation: When retrieving documents, MIME types specify how the content should be interpreted. For instance, distinguishing between PDFs and Word documents.
9. Digital Signatures and Encryption:
Scenario: Secure communication protocols often use MIME types for encrypted and signed messages.
Implementation: MIME types may indicate that the content is encrypted or signed, guiding the recipient on how to process the secure message.
10. Media Streaming Services:
Scenario: Platforms streaming audio and video content need to handle MIME types for compatibility with various devices.
Implementation: MIME types in HTTP responses help in identifying the type of media being streamed, ensuring proper playback.
In each of these scenarios, understanding and correctly implementing MIME is essential for seamless communication, data integrity, and user experience. Whether you are developing web applications, designing APIs, or managing email systems, MIME plays a fundamental role in ensuring the interoperability of diverse content types on the internet.
11. Community Participation:
Community participation is a valuable aspect of staying engaged, learning from others, and contributing to the collective knowledge within the field of MIME (Multipurpose Internet Mail Extensions) and internet standards. Here are ways you can actively participate in the community:
1. Join Online Forums and Groups:
Examples: Stack Overflow, Reddit (e.g., r/webdev), specialized forums.
Participate in discussions, share your experiences, and seek advice on MIME-related topics. Answering questions from others can deepen your understanding.
2. Engage on Social Media:
Platforms: Twitter, LinkedIn.
Follow relevant hashtags and accounts, share interesting articles, and engage in conversations. Social media platforms are excellent for real-time updates and networking.
3. Participate in Mailing Lists:
IETF Mailing Lists: Subscribe to mailing lists related to MIME and internet standards.
Engage in discussions, stay informed about ongoing developments, and contribute your insights.
4. Contribute to Open Source Projects:
GitHub, GitLab: Contribute to projects related to MIME handling in programming languages or web development.
By contributing code, documentation, or reporting issues, you actively participate in improving tools and libraries.
5. Attend Meetups and Conferences:
Local Meetups, Industry Conferences: Attend events related to web development, internet standards, or MIME.
Networking at these events can lead to valuable connections, and you can learn from the experiences of others.
6. Write Blog Posts or Tutorials:
Personal Blog, Medium: Share your knowledge by writing blog posts or tutorials.
Explaining concepts or sharing practical experiences can benefit others in the community.
7. Create and Share Resources:
GitHub Repositories, Gists: Create and share code snippets, examples, or tools related to MIME.
Providing useful resources can be a valuable contribution to the community.
8. Teach or Mentor:
Online Courses, Coding Bootcamps: Offer your expertise by teaching or mentoring.
Helping others learn about MIME and related topics contributes to the growth of the community.
9. Ask Questions and Seek Guidance:
Online Forums, Q&A Platforms: Don’t hesitate to ask questions when you encounter challenges.
Seeking guidance from the community can lead to solutions and new insights.
10. Collaborate on Research:
Academic Journals, Research Groups: Collaborate on research related to MIME or internet standards.
Academic contributions can advance the understanding and development of MIME-related technologies.
11. Contribute to Standards Bodies:
IETF, W3C: Contribute to internet standards bodies.
Active participation in standardization efforts ensures that your expertise contributes to the evolution of MIME.
12. Organize Community Events:
Meetups, Workshops: Take the initiative to organize local or online events.
Hosting events allows you to bring the community together and share knowledge.
13. Provide Feedback to Developers:
GitHub Issues, Bug Reports: If you use libraries or tools related to MIME, provide constructive feedback to developers.
Your feedback helps improve the quality of tools used by the community.
Participating in the community is a reciprocal process where you both contribute and gain knowledge. It fosters a collaborative environment and ensures that expertise is shared for the benefit of all practitioners in the field.
12. Read RFCs:
Reading Request for Comments (RFC) documents is essential for gaining an in-depth understanding of standards like MIME (Multipurpose Internet Mail Extensions). RFCs provide detailed specifications and guidelines. Here's how you can approach reading RFCs:
1. Identify Relevant RFCs:
MIME RFCs: Focus on RFCs specific to MIME, such as RFC 2045, RFC 2046, RFC 2047, and RFC 2049.
These RFCs define the MIME standard and its various aspects.
2. Understand the Structure:
- Sections and Headers: RFCs are typically organized into sections with specific headers. Understand the structure, including the abstract, introduction, and key sections.
3. Read the Abstract and Introduction:
Abstract: Provides a concise summary of the document's purpose.
Introduction: Sets the context and explains the motivation behind the standard.
4. Focus on Key Concepts:
Content Types: Understand how MIME defines content types and subtypes.
Multipart Messages: Explore how MIME enables the packaging of multiple entities within a single message.
5. Pay Attention to Syntax and Semantics:
Grammar and Syntax: RFCs often include formal grammar or syntax specifications. Pay attention to these for a precise understanding.
Semantics: Understand the meaning and intended use of different elements and attributes.
6. Explore Examples:
Practical Examples: RFCs often include examples to illustrate concepts.
Implementation Examples: Look for examples that demonstrate how MIME is implemented in various scenarios.
7. Follow References:
References Section: RFCs cite other relevant documents. Follow these references for a more comprehensive understanding.
Dependency Chains: Understand dependencies between RFCs to grasp the broader context.
8. Take Notes:
Document Key Points: Summarize key points and concepts as you read.
Annotations: Annotate sections that may require further exploration or clarification.
9. Join Discussions:
IETF Mailing Lists: Participate in discussions on IETF mailing lists related to MIME.
Clarify Doubts: If you have questions or uncertainties, the community can provide clarifications.
10. Apply Knowledge:
Implement Concepts: Apply the concepts you've learned by implementing them in practical scenarios.
Build Projects: Consider building projects that involve MIME handling to solidify your understanding.
11. Stay Updated:
- Monitor Updates: RFCs are periodically updated. Stay informed about revisions and updates to ensure your knowledge aligns with the latest standards.
12. Combine with Practical Experience:
Hands-On Practice: Apply what you learn from RFCs in real-world scenarios.
Experiment: Experiment with MIME in email systems, web development, or other relevant projects.
Reading RFCs can be challenging due to their technical nature, but it's a valuable skill for anyone working with internet standards. Break down the content, focus on key concepts, and use the information to enhance your practical skills in MIME handling.
Remember that the examples provided are simplified and might need adaptation based on your specific use case and environment.
Subscribe to my newsletter
Read articles from Saifur Rahman Mahin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saifur Rahman Mahin
Saifur Rahman Mahin
I am a dedicated and aspiring programmer with a strong foundation in JavaScript, along with proficiency in key web development technologies like React, Next JS, Vue JS, Express JS, PHP, Laravel, MongoDB, and MySQL. I have a passion for creating interactive and dynamic web applications, and I'm committed to continuous learning and improvement in the ever-evolving world of programming. With my skills and enthusiasm, I'm excited to contribute to exciting projects and explore new opportunities in the field of web development.