1528. Shuffle String

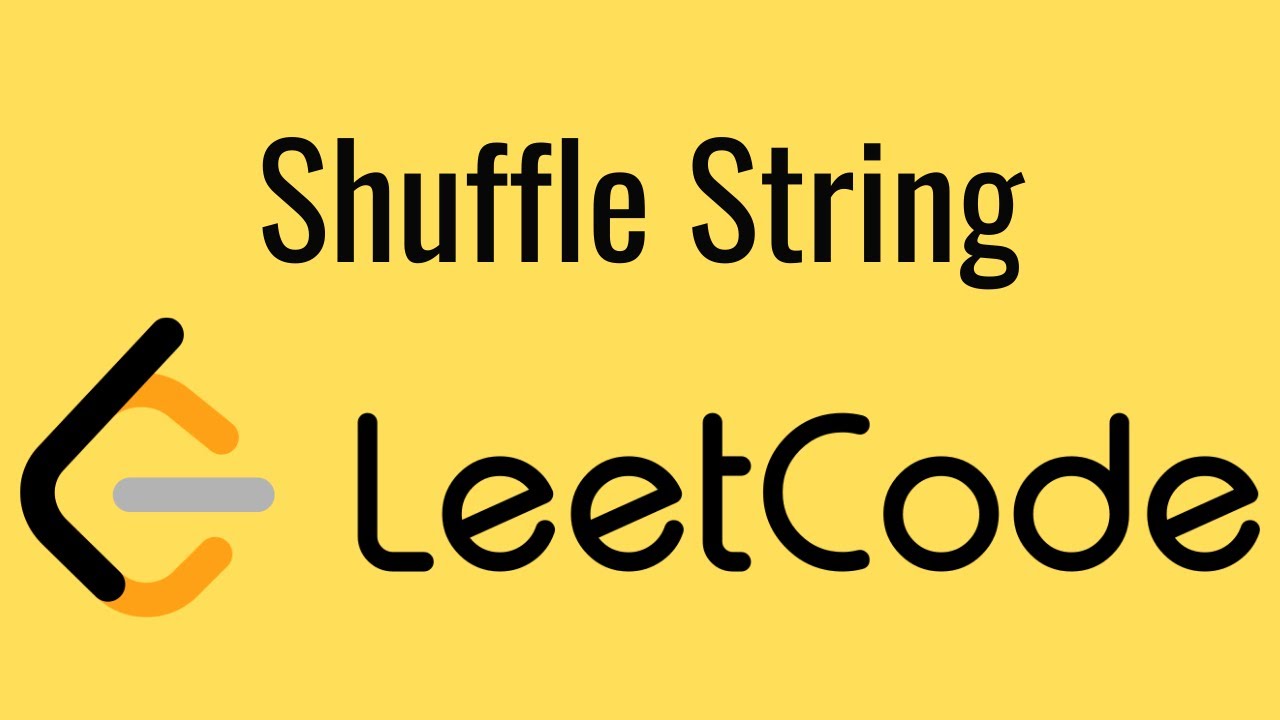
You are given a string s
and an integer array indices
of the same length. The string s
will be shuffled such that the character at the i<sup>th</sup>
position moves to indices[i]
in the shuffled string.
Return the shuffled string.
Example 1:
Example 1:
Input: s = "codeleet", indices = [4,5,6,7,0,2,1,3]
Output: "leetcode"
Explanation: As shown, "codeleet" becomes "leetcode" after shuffling.
Example 2:
Input: s = "abc", indices = [0,1,2]
Output: "abc"
Explanation: After shuffling, each character remains in its position.
The way of approach to the solution. you are given a string and the indices values according to the indices values place the characters accordingly.
4 5 6 7 0 2 1 3
c o d e l e e t
Arrange them as = 0 1 2 3 4 5 6 7 8
at position 0,1,2,3,4,5,6,7 -> leetcode
Solution 1:
public class ShuffleString {
public static void main(String[] args) {
String s = "codeleet";
int[] indices = {4,5,6,7,0,2,1,3};
int len = indices.length;
char[] c = new char[len];
for(int i=0;i<len;i++){
c[indices[i]] = s.charAt(i);
}
String str = new String(c);
System.out.println(str);
//System.out.println(new String(c));
}
}
output:
leetcode
process:
step 1:
Here the length of the string and the indices length are the same.
For finding length we can use both string and array.
For string length() function is used => len = string.length();
For an array of indices length is used => len = array.length;
step 2:
create a new array of indices array of length.
name the new array as you wish and the array stores the characters of the string so we want to create the character array.
char[] c = new char[len];
step 3:
for loop i=0 to i<len
Initially, the character array contains the null value.
c[indices[i]] = s.charAt(i);
charAt(i) = it means it will take the character at position i from string s.
i=0 => c[indices[0]] = s.charAt(0) => c[4] = c => [., ., ., ., c, ., ., .,]
i=1 => c[indices[1]] = s.charAt(1) => c[5] = o => [., ., ., ., c, o, ., .,]
i=2 => c[indices[2]] = s.charAt(2) => c[6] = d => [., ., ., ., c, o, d, .,]
i=3 => c[indices[3]] = s.charAt(3) => c[7] = e => [., ., ., ., c, o, d, e,]
i=4 => c[indices[4]] = s.charAt(4) => c[0] = l => [l, ., ., ., c, o, d, e,]
i=5 => c[indices[5]] = s.charAt(5) => c[2] = e => [l, ., e, ., c, o, d, e,]
i=6 => c[indices[6]] = s.charAt(6) => c[1] = e => [l, e, e, ., c, o, d, e,]
i=7 => c[indices[7]] = s.charAt(7) => c[3] = t => [l, e, e, t, c, o, d, e,]
i++ => i=8
8<8 condition false.
step 4:
converting the character array to a string.
String str = new String(array); then it will convert the array into a string.
Finally, print the string.
Happy Coding :)
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
