Getting Started with OAuth 2.0

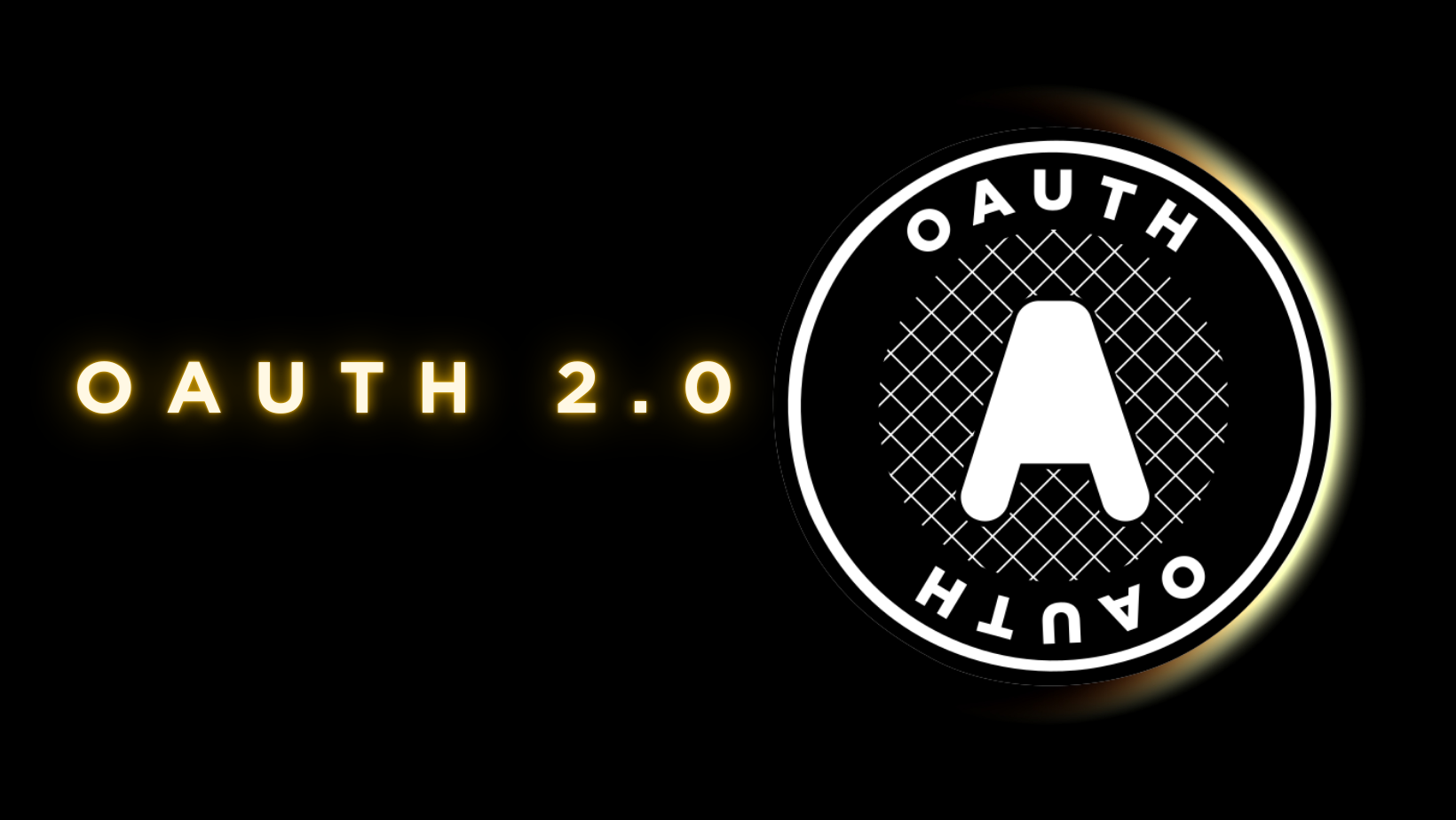
Before learning about OAuth, let me explain these two basic terms:
Authentication and Authorization
Authentication simply means "Tell me who you are," which involves verifying your identity. The system checks if your username and password match, ensuring only you can access your account.
Once you're authenticated, the system determines what you're allowed to do. This is where authorization comes in. It decides which parts of the system you can access—like what features or data you're permitted to use.
OAuth 2.0
OAuth 2.0 simplifies authorization. It allows you to log into an app using services like Google or Facebook without needing to enter a password.
But exactly How?
So basically OAuth uses access tokens to grant specific permissions. For example, when you log into a third-party app with Google, Google gives the app an access token that allows it to view certain parts of your account (like your contacts), but it doesn’t share your password.
Why Use OAuth?
OAuth is a secure and straightforward way to log into various platforms without needing to manage multiple passwords. It lets you give apps controlled access to your data without sharing sensitive information.
Let’s try to set up OAuth 2.0 in a Node.js based web application
We’ll be using Passport.js as middleware to handle user authentication, Express.js to set up our web server and routes, and the Google Developer Console to get the credentials needed for Google OAuth 2.0 authentication.
Go to the Google Developer Console.
Create a new project.
Go to “APIs & Services” > “Credentials”.
Click “Create Credentials”, and pick “OAuth 2.0 Client IDs”.
Fill out the form, choose Web Application, and add the Authorized Redirect URI (like
http://localhost:3000/auth/google/callback
).You'll get a Client ID and Client Secret—keep them aside for now.
In your Node.js project folder, run these commands:
npm init -y # To initialize your project if you haven't already
npm install express passport passport-google-oauth20 express-session
These commands will install express
passport
passport-google-oauth20
express-session
in your project folder, we’ll be using them later on.
- In your
app.js
file, set up the server and authentication like this:
const express = require('express');
const passport = require('passport');
const session = require('express-session');
const GoogleStrategy = require('passport-google-oauth20').Strategy;
const app = express();
// Session setup
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true
}));
// Initialize passport
app.use(passport.initialize());
app.use(passport.session());
// Save user info in the session
passport.serializeUser((user, done) => done(null, user));
passport.deserializeUser((obj, done) => done(null, obj));
// Google OAuth Strategy
passport.use(new GoogleStrategy({
clientID: 'YOUR_GOOGLE_CLIENT_ID', // Replace with your Google Client ID
clientSecret: 'YOUR_GOOGLE_CLIENT_SECRET', // Replace with your Google Client Secret
callbackURL: '/auth/google/callback'
},
(accessToken, refreshToken, profile, done) => {
return done(null, profile); // In a real app, you'd save the user profile to the database
}
));
- Add these routes in your
app.js
to handle the login and profile display:
// Route to start the Google login process
app.get('/auth/google',
passport.authenticate('google', { scope: ['profile', 'email'] }));
// Callback route for Google after the login
app.get('/auth/google/callback',
passport.authenticate('google', { failureRedirect: '/' }),
(req, res) => {
res.redirect('/profile'); // Redirect to profile page on successful login
}
);
// Route to display user's profile
app.get('/profile', (req, res) => {
if (!req.isAuthenticated()) {
return res.redirect('/');
}
res.send(`<h1>Welcome, ${req.user.displayName}</h1>`);
});
// Logout route
app.get('/logout', (req, res) => {
req.logout(() => {
res.redirect('/');
});
});
// Home route
app.get('/', (req, res) => {
res.send('<h1>Welcome</h1><a href="/auth/google">Login with Google</a>');
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on http://localhost:${PORT}`);
});
- Start your server using :
node app.js
Go to
http://localhost:3000
in your browser. You'll see a link to log in with Google.After logging in with Google, you’ll be redirected to the profile page, which will show your Google username.
To log out, just hit
/logout
.
What now?
All of these steps and concepts can feel overwhelming, especially if you're working with them for the first time.
For a better approach, take some time to read the documentation for each component we've discussed. This will help you wrap your head around the concepts before you start working with them. It not only clears up any confusion but also reduces the chances of bugs in your application.
Plus, as I mentioned earlier, understanding these elements better will benefit you in the long run. Even when it comes to fixing bugs, you’ll be able to find solutions more quickly.
Subscribe to my newsletter
Read articles from Sakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sakshi
Sakshi
I love working with both design and technology, understanding front-end and back-end development, and solving problems as I go. What I enjoy most, though, is sharing what I’ve learned and helping build a supportive community. I believe that by learning and growing together, we can make the tech world better.