1859. Sorting the Sentence

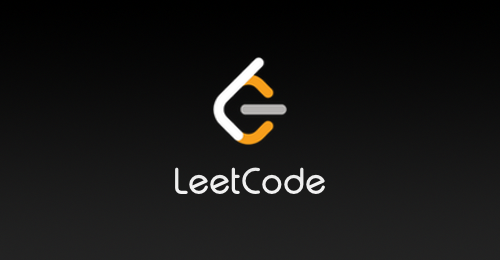
A sentence is a list of words that are separated by a single space with no leading or trailing spaces. Each word consists of lowercase and uppercase English letters.
A sentence can be shuffled by appending the 1-indexed word position to each word and then rearranging the words in the sentence.
- For example, the sentence
"This is a sentence"
can be shuffled as"sentence4 a3 is2 This1"
or"is2 sentence4 This1 a3"
.
Given a shuffled sentence s
containing no more than 9
words, reconstruct and return the original sentence.
Example 1:
Input: s = "is2 sentence4 This1 a3"
Output: "This is a sentence"
Explanation: Sort the words in s to their original positions "This1 is2 a3 sentence4", then remove the numbers.
Example 2:
Input: s = "Myself2 Me1 I4 and3"
Output: "Me Myself and I"
Explanation: Sort the words in s to their original positions "Me1 Myself2 and3 I4", then remove the numbers.
Constraints:
2 <= s.length <= 200
s
consists of lowercase and uppercase English letters, spaces, and digits from1
to9
.The number of words in
s
is between1
and9
.The words in
s
are separated by a single space.s
contains no leading or trailing spaces.
CODE:
public class SortingSentence {
public static void main(String[] args) {
String s = "is2 sentence4 This1 a3";
String[] str = s.split(" ");
int len = str.length;
String[] result = new String[len];
StringBuilder sb = new StringBuilder();
int index =0;
for(String string:str){
int l = string.length();
index = (int)(string.charAt(l-1)-'0');
result[index-1] = string.substring(0, l-1);
}
for(int i=0; i<result.length-1; i++){
sb.append(result[i]).append(" ");
}
sb.append(result[result.length-1]);
System.out.println(sb.toString());
}
}
This is a sentence
Explanation:
step 1:
split the string into a string array.
String[] str = s.split(" ");
s = "is2 sentence4 This1 a3"
str = [ "is2", "sentence4", "This1", "a3" ]
for finding the length of the string array is calculated using the len = str.length;
int index = 0;
for(String string:str){
int l = string.length();
index = (int)(string.charAt(l-1)-'0');
result[index-1] = string.substring(0, l-1);
}
for(String string:str) => It will directly take the string
string = "is2" => Here extract the number from the string.
"is2".length() -> l = 3
index = (int) (string.charAt(l-1) - '0')
unicode of '0' = 48
public class UnicodeExample {
public static void main(String[] args) {
char digitTwo = '0';
// Getting the Unicode value
int unicodeValue = (int) digitTwo;
System.out.println("Unicode value of '0': " + unicodeValue);
}
}
Unicode value of '0': 48
Unicode of '0' = 48
Unicode of '1' = 49
Unicode of '2' = 50
Unicode of '3' = 51 ........
index = (int) (string.charAt(3-1) - '0') => (int) ( '2'-'0' ) => 50-48 => index = 2
int[] result = new int[len];
result[index-1] = str.substring(0,l-1);
result[1] = is
result[3] = sentence
result[0] = This
result[2] = a
StringBuilder sb = new StringBuilder();
for(int i=0; i<result.length-1; i++){
sb.append(result[i]).append(" ");
}
sb.append(result[result.length-1]);
i=0 => sb.append(0).append(" ") => sb = This
i=1 => sb.append(1) => sb = This is
i=2 => sb.append(2) => sb = This is a
3 < 3 condition false loop terminate. If we add the last word the extra space is added at the end.
sb.append(result[3]) => This is a sentence
sb.toString() => "This is a sentence".
Thank you for reading.
Happy Coding :)
Subscribe to my newsletter
Read articles from Sirisha Challagiri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
