Creating Custom Widgets in CesiumJS: A Developer's Toolkit

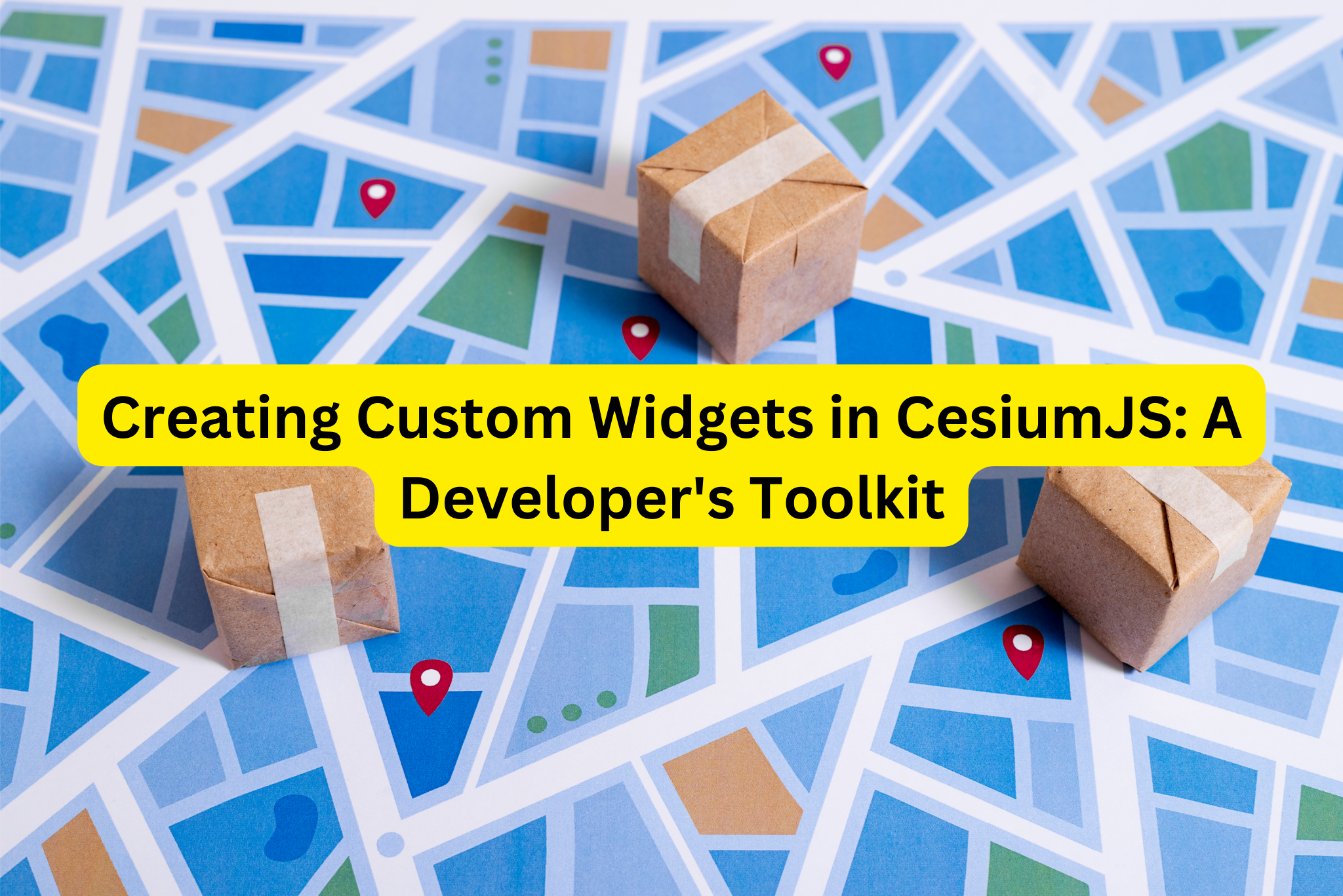
CesiumJS not only provides a rich set of built-in widgets for common tasks but also allows developers to create custom widgets tailored to their specific application needs. In this guide, we'll not only cover the fundamental steps for creating custom widgets but also explore the architecture of CesiumJS widgets and how to publish them for broader reuse.
Widget Architecture in CesiumJS
CesiumJS follows a modular widget architecture, treating each widget as an independent component. The characteristics of a CesiumJS widget include:
Encapsulation of state and functionality.
Exposure of public methods and properties for interaction.
Event handling to notify changes in state.
Styling capabilities using CSS.
Integration with Cesium's scene and primitives.
Creating a Basic Custom Widget
Let's start by creating a simple HelloWidget that displays a greeting:
javascript
class HelloWidget {
constructor(options) {
this._container = document.createElement("div");
this._container.innerText = "Hello World!";
this._container.className = "cesium-helloWidget";
this._container.addEventListener("click", () => {
this._container.innerText = "Goodbye!";
});
}
get container() {
return this._container;
}
}
This widget encapsulates its state, exposes a container, and reacts to a click event to update its content.
Integrating with CesiumViewer
To integrate the widget with a CesiumViewer, pass it as an option during construction:
javascript
const viewer = new Cesium.Viewer("cesiumContainer", {
widget: new HelloWidget()
});
CesiumViewer will handle the automatic appending of the widget's container to the DOM.
Adding Custom Styles and Interactions
Enhance the widget by adding styles using CSS:
css
.cesium-helloWidget {
color: green;
font-size: 2em;
}
Additionally, listen to DOM events and update the widget's state:
javascript
class HelloWidget {
constructor(options) {
this._container = document.createElement("div");
this._container.innerText = "Hello World!";
this._container.className = "cesium-helloWidget";
this._container.addEventListener("click", () => {
this._container.innerText = "Goodbye!";
});
}
get container() {
return this._container;
}
}
Publishing the Widget for Reuse
To share your custom widget, follow these steps:
- Export as a module
javascript
export default HelloWidget;
- Publish on npm
bash
npm publish
- Document API and examples in README
Provide clear documentation on how to use the widget, its API, and include examples in the README file.
- Mention licensing and contribution guidelines
Clearly state the licensing terms and guidelines for contributions.
By following these steps, other developers can easily install and integrate your custom widget into their Cesium applications, fostering collaboration within the CesiumJS community.
Subscribe to my newsletter
Read articles from Phạm Vân Anh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Phạm Vân Anh
Phạm Vân Anh
Currently I am working as Marketing Executive at Bac Ha Software - Leading software development company in Vietnam https://bachasoftware.com/