Day 27 | All about Docker Network
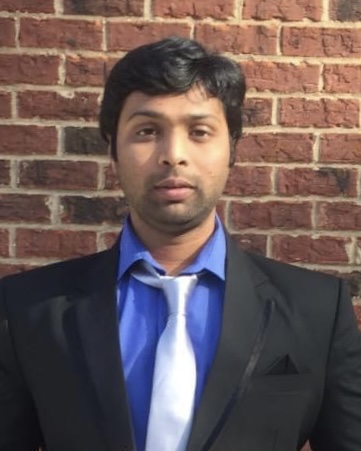
What is Networking in Docker?
Docker networking refers to the mechanisms and technologies Docker uses to enable communication between Docker containers, as well as between containers and the outside world. It plays a crucial role in allowing containers to work together in a networked environment.
Key Aspects of Docker Networking:
Isolation:
- By default, Docker containers are isolated from each other, meaning they run in their own network namespace. This isolation prevents interference between containers and ensures that each container has its own network stack, including its own IP address.
Container Communication:
- Docker containers can communicate with each other using different networking options. Containers on the same Docker network can reach each other by their container names or IP addresses.
Port Mapping:
Docker enables port mapping to expose specific ports on the host machine, making services inside containers accessible from outside the container environment.
bashCopy codedocker run -p 8080:80 my-web-app
In this example, the container's port 80 is mapped to port 8080 on the host.
DNS Resolution:
- Docker provides a built-in DNS server that allows containers to refer to each other by their container names. This DNS resolution simplifies communication between containers.
Custom Bridge Networks:
- Docker allows users to create custom bridge networks with specific configurations, including IP address ranges and custom DNS settings. This helps in organizing and managing container communication.
External Connectivity:
- Containers can connect to external networks, making it possible for them to access external services or the internet.
Different types of networking in Docker Containers
Let's explore different types of networking in Docker containers using a simple analogy involving a neighbourhood and communication between houses.
Bridge Network:
Analogy: A street in a neighbourhood where each house (container) has its address (IP address).
Example:
You have multiple houses (containers) on a street (bridge network).
Each house has a unique address (IP address) within the street.
Houses can communicate with each other by their addresses (IP addresses).
# Creating a container on the default bridge network
docker run -d --name house1 my-app
# Creating another container on the same bridge network
docker run -d --name house2 my-app
Host Network:
Analogy: All houses (containers) share the same street address (IP address) as the neighbourhood (host machine).
Example:
Instead of having separate addresses, all houses (containers) share the same address as the neighbourhood (host machine).
This makes it easy for houses to communicate with services outside the neighbourhood.
# Creating a container using the host network
docker run -d --network host my-app
Overlay Network:
Analogy: Interconnecting streets in different neighbourhoods (containers on different hosts) using a special bridge (overlay network).
Example:
You have two neighbourhoods (Docker hosts) with separate streets (bridge networks).
An overlay network is like a special bridge that connects these streets, allowing houses (containers) in different neighbourhoods to communicate.
# Creating an overlay network
docker network create --driver overlay my_overlay_network
# Creating containers on the overlay network
docker run -d --network my_overlay_network --name house1 my-app
docker run -d --network my_overlay_network --name house2 my-app
Macvlan Network:
Analogy: Giving each house (container) a separate street address (MAC address) as if it were a physical house on the street.
Example:
- Instead of sharing the same street address, each house (container) gets its unique address as if it were a standalone house on the street.
# Creating a container using a Macvlan network
docker network create -d macvlan --subnet=192.168.1.0/24 --gateway=192.168.1.1 -o parent=eth0 my_macvlan_network
docker run -d --network my_macvlan_network --name house1 my-app
None Network:
Analogy: A house (container) without any direct connection to a street (network).
Example:
- This is like a house that is not connected to any street; it has no direct way of communicating with the outside world.
# Creating a container with no network
docker run -d --network none my-app
- Custom Bridge Networks:
Analogy: A custom bridge network in Docker is like a private meeting room where a group of colleagues can communicate and collaborate. It provides a dedicated space with its own rules, allowing containers to share information in an organized and controlled environment.
Example:
Create a Custom Bridge Network:
docker network create --driver bridge my_custom_network
This command establishes a new bridge network named
my_custom_network
with its own isolated space for container communication.Run Containers on the Custom Network:
docker run --network my_custom_network --name container1 my-image docker run --network my_custom_network --name container2 my-image
Containers
container1
andcontainer2
are connected to themy_custom_network
bridge network. They can communicate with each other using their container names within this isolated space.
These are different analogies which provide a simple way to understand the concepts of Docker networking. Just like houses in a neighbourhood, Docker containers can have different ways of communicating with each other and the outside world, depending on the network configuration chosen.
How does Bridge Network work?
Here's a simple breakdown of how veth pairs work in Docker:
Creation of Veth Pairs:
When a Docker container is started, Docker creates a virtual network interface pair (veth pair).
One end of the pair is attached to the network namespace of the container (inside the container).
The other end is attached to the host machine's network namespace.
Isolation:
The veth pair helps maintain network isolation between the container and the host machine.
Communication between the container and the host is facilitated through the veth pair.
Bridge Networking:
- In Docker, the default networking mode is a bridge network. Each container is connected to a virtual bridge, and the veth pair is used to connect the container's network namespace to this bridge.
Communication Between Containers:
When containers on the same bridge network need to communicate, Docker uses veth pairs to establish this communication.
Each container gets its veth pair connected to the bridge, and communication occurs through these virtual interfaces.
Cleanup:
- When a container is stopped or removed, Docker cleans up the associated veth pair, ensuring that no remnants of the network connection persist.
The use of veth pairs helps Docker achieve network isolation and enables efficient communication between containers while maintaining separation from the host machine. It's an essential component of Docker networking that allows for the creation and management of network connections in a lightweight and scalable manner.
How does Host Network work?
In Docker, using the host network mode allows a container to share the network namespace with the host machine. This means the container does not get its isolated network stack; instead, it uses the network stack of the host directly. Let's break down how the host network mode works:
Running a Container with Host Network:
- When you run a Docker container with the
--network host
option, it uses the host network mode.
- When you run a Docker container with the
docker run -d --network host my-app
Shared Network Namespace:
In host network mode, the container shares the same network namespace as the host machine.
This means the container uses the same network interfaces, IP addresses, and ports as the host.
Direct Access to Host Services:
Containers in host network mode can directly access services running on the host machine without any port mapping.
If a service is listening on a particular port on the host, the container can access it on the same port without any additional configuration.
Host IP Address:
- The container in host network mode gets the same IP address as the host machine. This is different from other network modes, where containers typically have their own isolated IP addresses.
No Port Mapping Required:
- Since the container uses the host's network stack, there is no need for port mapping. If a service inside the container listens on port 80, it's accessible on port 80 of the host as well.
# The container's port 80 is directly accessible on the host's port 80
curl http://localhost
Performance Considerations:
Host network mode can offer better performance for certain types of applications, especially those that require low-latency communication or need to bind to many ports.
However, it might also reduce isolation between the container and the host.
Security Implications:
- While host network mode provides direct and efficient access to host services, it reduces the level of isolation between the container and the host. This may have security implications, and it's important to consider whether the benefits outweigh the potential risks.
Summary: When you run a Docker container in host network mode, it operates as if it's directly using the network stack of the host machine. This can simplify network configuration for certain applications but comes with the trade-off of reduced isolation between the container and the host.
(This is one of the most insecure way of creating networking in docker as anybody who have access to container can also have access to the host, which raises a security concern.)
How does the Overlay Network work?
In Docker, an overlay network is a network type that enables communication between containers running on different Docker hosts. This is particularly useful in distributed or swarm mode setups where containers are spread across multiple machines. The overlay network provides a way for containers on different hosts to seamlessly communicate with each other.
Network Creation:
- To create an overlay network, you use the
docker network create
command, specifying theoverlay
driver.
- To create an overlay network, you use the
docker network create --driver overlay my_overlay_network
This creates a virtual network that spans multiple Docker hosts.
Joining Containers to the Overlay Network:
- Containers that need to communicate with each other across different hosts can be joined to the overlay network.
docker run -d --network my_overlay_network --name container1 my-app
docker run -d --network my_overlay_network --name container2 my-app
Here, container1
and container2
are on different hosts but are part of the same overlay network.
Overlay Network Architecture:
Docker uses a combination of technologies, including the Virtual Extensible LAN (VXLAN) protocol, to create the overlay network.
VXLAN encapsulates Ethernet frames in UDP packets, allowing them to traverse different hosts in the overlay network.
Service Discovery:
Overlay networks come with built-in service discovery mechanisms.
Containers can discover and communicate with each other using their container names, similar to how it works on a bridge network within a single host.
# From within a container, you can ping another container by its name
ping container2
Security and Isolation:
- Overlay networks provide isolation between containers on different hosts. Communication between containers is encrypted, providing a secure communication channel.
Swarm Mode:
Overlay networks are commonly used in Docker Swarm mode, which allows you to orchestrate and manage a cluster of Docker hosts.
In Swarm mode, services can be deployed across the cluster, and the overlay network facilitates communication between services on different hosts.
Dynamic Container Discovery:
- Containers can join or leave the overlay network dynamically. The network adapts to changes in the cluster, allowing for automatic discovery of containers.
Scaling:
- As your application scales and additional containers are deployed across the cluster, the overlay network ensures seamless communication without manual configuration.
Overlay networks in Docker provide a powerful solution for inter-container communication in distributed and multi-host environments. They enable containers to communicate securely across different hosts, offering flexibility, scalability, and service discovery capabilities.
How to achieve logical isolation using Customer Bridge Network?
Let's think of an office space to explain how this logical isolation is achieved using a custom bridge network in Docker:
Analogy: Separating Teams with Different Projects in an Office
Creating Separate Meeting Rooms (Creating Custom Bridge Networks):
- Imagine you're managing an office, and you want to keep teams working on different projects separately. You create distinct meeting rooms for each project. In Docker, this is akin to creating custom bridge networks for different sets of containers.
# Create custom networks for different projects
docker network create --driver bridge project_a_network
docker network create --driver bridge project_b_network
Assigning Teams to Their Respective Rooms (Connecting Containers to Networks):
- Each team is assigned to its meeting room. Similarly, containers are connected to their designated custom bridge networks.
# Containers for Project A
docker run --network project_a_network --name container_a1 my-image
docker run --network project_a_network --name container_a2 my-image
# Containers for Project B
docker run --network project_b_network --name container_b1 my-image
docker run --network project_b_network --name container_b2 my-image
Team Communication Within the Same Room (Container Communication Within a Network):
- Teams within the same room can easily communicate and share information. Likewise, containers on the same custom bridge network can communicate with each other.
# Inside container_a1, you can communicate with container_a2 using its name
ping container_a2
Isolation Between Different Rooms (Logical Isolation):
- Teams in one room are isolated from teams in other rooms to maintain privacy and focus on their projects. Similarly, containers on one custom bridge network are logically isolated from containers on other networks.
# Containers on project_a_network cannot directly communicate with containers on project_b_network
ping container_b1
Custom Rules for Each Room (Network Configuration):
- Each meeting room might have its own rules and configurations. In Docker, custom bridge networks allow you to configure specific settings, such as IP address ranges or custom DNS, tailored to the needs of containers within each network.
# Configure a custom subnet and gateway for project_a_network
docker network create --driver bridge --subnet=192.168.1.0/24 --gateway=192.168.1.1 project_a_network
Achieving logical isolation using custom bridge networks in Docker is similar to separating teams with different projects into distinct meeting rooms in an office. Each network acts as a dedicated space for containers to work on specific tasks, ensuring communication within the same network while maintaining logical isolation from other networks.
A Simple Project Illustrating the concept of
Bridge Network Vs Host Network Vs Custom Bridge Network.
I have cloned an open-source Git repo and navigated to a repo that has a docker file.
Now let us create a docker container named "login". Let's say it stores login details of Customers.
Let us open an interactive bash by using "docker exec -it login /bin/bash".
Next, let's update the apt package manager by using "apt update" and install ping by using "apt-get install iputils-ping -y".
Check installation using "ping -V"
Now let us create another container named "logout".
Now we have two containers that can be viewed by using "docker ps".
Let us inspect the login container to see the ip address and network details by using "docker inspect login"
If we closely observe under Networks it shows "Bridge" (which is the default network) and ip address is "172.17.0.2".
Now let us inspect the logout container using "docker inspect logout".
If you closely observe the Networks section it shows that the "bridge" and ip address is "172.17.0.3".
So by this, we can see that both the "login" and "logout" are in the bridge network and are in the same subnet "172.17".
Let's try to communicate from the "login" container with the "logout" container since they are on the same default "bridge" network by using "ping 172.17.0.3 (ip address of logout container".
By this, it is evident that containers "login" and "logout" can communicate with each other.
To see networks in the docker we can use "docker network ls". This lists all the networks (the default ones, the customized networks etc).
To remove unwanted networks, we can simply type "docker network rm ls".
Let us create a network named "secure-network". The command is "docker network create secure-network" and do docker network ls.
Now that we have seen container-to-container communication. Now let us create a new docker container named "finance" and assume secure details of customers are stored in it. Now, we have to logically isolate this container. For this, we can use "docker run -d --name finance --network=secure-network nginx: latest".
So now we have three docker containers, this can be achieved by doing "docker ps".
Let us inspect this container by doing "docker inspect finance".
This shows that this container is under a customized bridge network "secure-network" which we created.
Let us try to ping this (finance container) from the container "login" and we should not be able to communicate, which means this finance container is logically separated.
Now let us create another container named "host-demo" within the host network. This can be achieved by using "docker run -d --name host-demo --network=host nginx: latest".
Now let us inspect this "host-demo" container using "docker inspect host-demo", doing this we can obtain network details
So, this is evident that this container is binded with the host. Hence it is displayed as a "host" in the networks sections and has no ip.
Conclusion: In unravelling Bridge Network vs Host Network vs Custom Bridge Network, we've navigated the art of container orchestration. Each choice, whether the Bridge's simplicity, the Host's directness, or a Custom Bridge's tailored elegance, paints a unique stroke in our network canvas. In this technological symphony, let's remember: that beauty lies not just in code but in thoughtful architecture. May your networks be robust, containers harmonious, and projects, works of art in technology's grand tapestry.
Subscribe to my newsletter
Read articles from Sarat Motamarri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
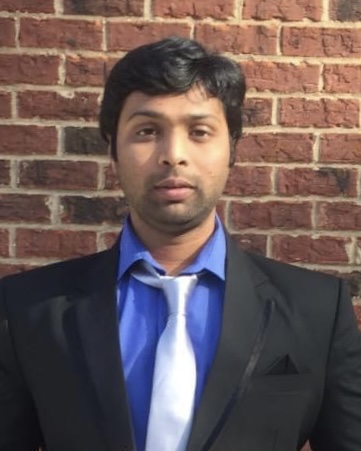
Sarat Motamarri
Sarat Motamarri
Hey there, I'm Sarat Motamarri, an aspiring engineer mastering the art of DevOps & AWS. If you're as passionate about the cloud and open-source wizardry as I am, you're in the right place! Let's explore the skies together ๐๐ฎ #CloudEnthusiast #DevOpsDreamer"