Go's Orchestra: A Symphony of Concurrency
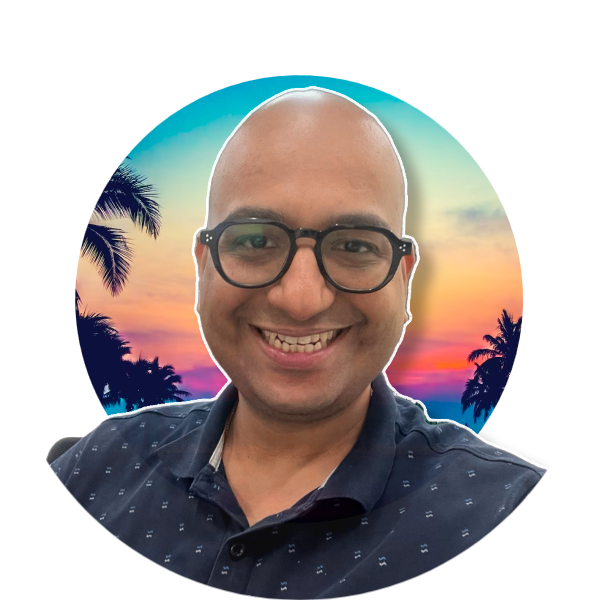
Table of contents
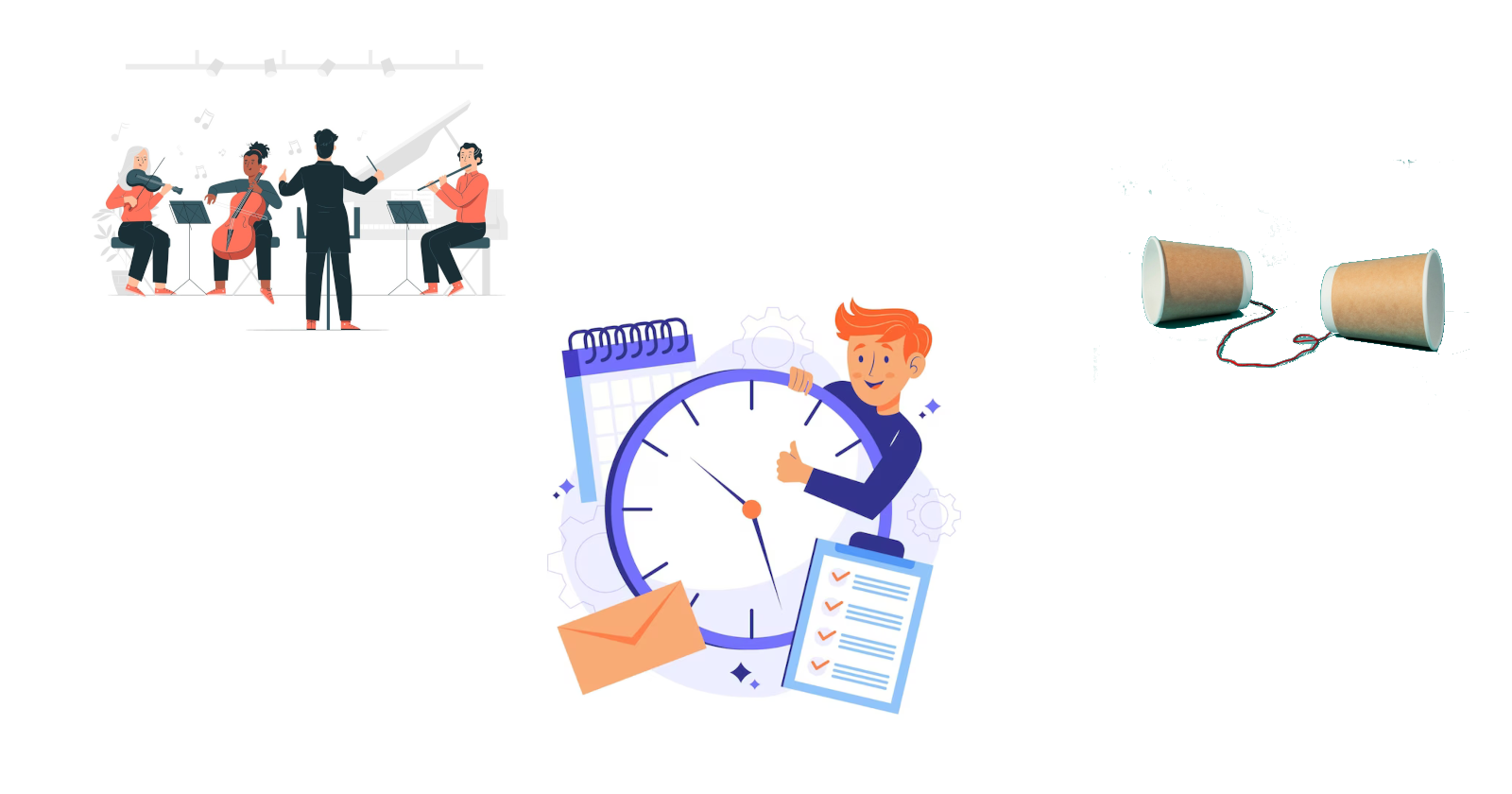
Introduction
As we venture into our Go learning journey, the focus shifts to the fascinating realm of concurrency. At the heart of Go's concurrency model are Go-routines and Channels, a dynamic duo that orchestrates parallelism with elegance.
Go routines are like little workers in your code, akin to having multiple tasks running concurrently. They operate independently, enabling seamless parallel execution. Imagine a team of chefs simultaneously preparing different parts of a meal in a well-coordinated kitchen. Channels, on the other hand, are communication pathways connecting these Go-routines. They allow workers to exchange information efficiently, like a network of conveyor belts in our kitchen ensuring each chef has the right ingredients.
Example Code
package main
import (
"fmt"
"time"
)
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d started job %d\n", id, j)
time.Sleep(time.Second) // Simulating work
fmt.Printf("Worker %d finished job %d\n", id, j)
results <- j * 2
}
}
func main() {
jobs := make(chan int, 5)
results := make(chan int, 5)
// Launch three worker goroutines
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
// Send jobs and close the jobs channel
for j := 1; j <= 5; j++ {
jobs <- j
}
close(jobs)
// Collect results from the workers
for a := 1; a <= 5; a++ {
result := <-results
fmt.Printf("Result received: %d\n", result)
}
}
In this code
We define a
worker
function that represents our concurrent workers (Go-routines).The
main
function initializes channelsjobs
andresults
.Three worker Go-routines are launched to process jobs concurrently.
Jobs are sent to the
jobs
channel, and it's closed to signal that no more jobs will be sent.Results are collected from the workers through the
results
channel.
Feel free to run and experiment with this code to get a hands-on experience with Go's concurrency features.
Reference Links:
Conclusion
As we immerse ourselves in the world of concurrency, Go-routines and Channels become indispensable tools for orchestrating parallel tasks. The analogy of a well-coordinated kitchen with chefs and conveyor belts helps visualize their synergy.
Can you think about real world use cases / implementation for go routines and channels? Let us know in the comments.
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
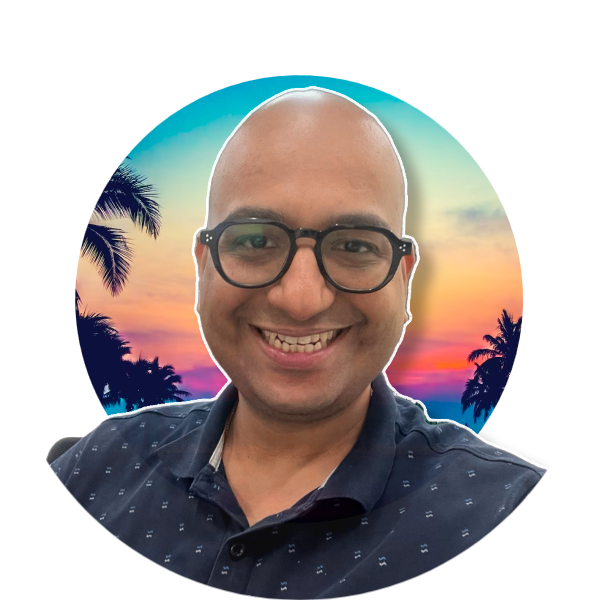
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.