Day 8 Task: Basic Git & GitHub for DevOps Engineers.
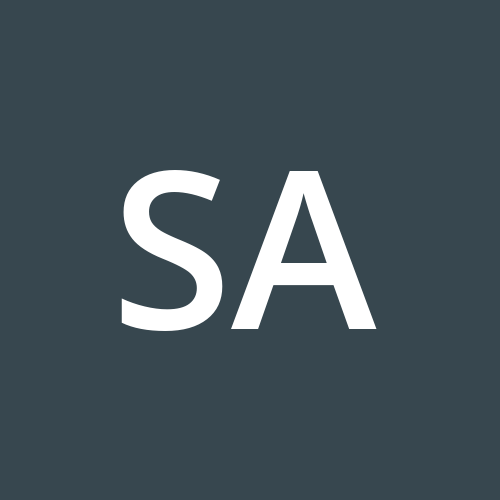
What is Git?
Git is a version control system that allows you to track changes to files and coordinate work on those files among multiple people. It is commonly used for software development, but it can be used to track changes to any set of files.
With Git, you can keep a record of who made changes to what part of a file, and you can revert back to earlier versions of the file if needed. Git also makes it easy to collaborate with others, as you can share changes and merge the changes made by different people into a single version of a file.
๐ฉโ๐ป Common Git Commands:
git init
: Initializes a new Git repository.git clone <repository>
: Copies a repository from a remote source to your local machine.git add <file>
: Adds changes in a file to the staging area.git commit -m "message"
: Records changes in the repository with a descriptive message.git push
: Uploads local changes to a remote repository.git pull
: Fetches changes from a remote repository and merges them into the current branch.git branch
: Lists all local branches in the current repository.git merge <branch>
: Combines changes from a specified branch into the current branch.git status
: Displays the status of changes as untracked, modified, or staged.
What is Github?
GitHub is a web-based platform that provides hosting for version control using Git. It is a subsidiary of Microsoft, and it offers all of the distributed version control and source code management (SCM) functionality of Git as well as adding its own features. GitHub is a very popular platform for developers to share and collaborate on projects, and it is also used for hosting open-source projects.
What is Version Control? How many types of version controls we have?
Version Control: Version control is a system that records changes to a file or a set of files over time so that you can recall specific versions later. It is especially crucial in software development, where multiple developers may be working on a project simultaneously. Version control systems help track changes, manage collaboration, and provide a history of modifications made to a codebase.
๐ Key Aspects of Version Control:
History Tracking: Records changes made to files, including who made the changes and when.
Collaboration: Facilitates simultaneous work by multiple developers on the same project.
Branching and Merging: Allows for the creation of branches for parallel development and easy merging of changes.
Revert to Previous States: Enables the ability to revert to a previous version of the codebase.
Types of Version Control Systems:
Centralized Version Control System (CVCS):
Description: Uses a central server to store versioned files, allowing collaboration.
Example: SVN (Apache Subversion).
Distributed Version Control System (DVCS):
Description: Each developer has a complete copy of the repository, enabling offline work and faster access.
Example: Git, Mercurial.
Why we use distributed version control over centralized version control?
Better collaboration: In a DVCS, every developer has a full copy of the repository, including the entire history of all changes. This makes it easier for developers to work together, as they don't have to constantly communicate with a central server to commit their changes or to see the changes made by others.
Improved speed: Because developers have a local copy of the repository, they can commit their changes and perform other version control actions faster, as they don't have to communicate with a central server.
Greater flexibility: With a DVCS, developers can work offline and commit their changes later when they do have an internet connection. They can also choose to share their changes with only a subset of the team, rather than pushing all of their changes to a central server.
Enhanced security: In a DVCS, the repository history is stored on multiple servers and computers, which makes it more resistant to data loss. If the central server in a CVCS goes down or the repository becomes corrupted, it can be difficult to recover the lost data.
Overall, the decentralized nature of a DVCS allows for greater collaboration, flexibility, and security, making it a popular choice for many teams.
Task:
Install Git:
Visit the official Git website: https://git-scm.com/downloads.
Download the appropriate version for your operating system (Windows, macOS, Linux).
Follow the installation instructions provided on the website.
Create a GitHub Account:
Visit the GitHub website: https://github.com/.
Click on the "Sign Up" button.
Fill in the required information, including your username, email address, and password.
Complete the account creation process by following the on-screen instructions.
Understanding Git and GitHub:
Now that you have Git installed and a GitHub account:
Git Basics:
Open a terminal or command prompt and type
git --version
to check if Git is installed correctly.Navigate to a directory where you want to initialize a Git repository using the command
cd <directory_path>
.Use
git init
to initialize a new Git repository in that directory.
Track Changes:
Create a new file using a text editor and save it in your Git repository directory.
Use
git status
to see the status of your repository, showing untracked files.Add the file to the staging area with
git add <filename>
.Commit the changes with
git commit -m "Your commit message"
.
Branching:
Create a new branch using
git branch <branch_name>
.Switch to the new branch with
git checkout <branch_name>
or usegit switch <branch_name>
(Git 2.23 and later).Make changes in the new branch, add, and commit them.
GitHub:
On GitHub, create a new repository by clicking on the "+" sign in the top right and selecting "New repository."
Follow the instructions to create a new repository.
Once created, copy the repository URL.
On your local machine, add the remote repository with
git remote add origin <repository_url>
.Push your changes to GitHub with
git push -u origin master
(or the branch you're working on).
Exercises:
Create a new repository on GitHub and clone it to your local machine
Creating a New Repository on GitHub:
Log in to GitHub:
- Go to GitHub and log in to your account.
Create a New Repository:
Click on the "+" sign in the top right corner of the GitHub page.
Select "New repository" from the dropdown menu.
Fill in Repository Details:
Enter a name for your repository in the "Repository name" field.
Add a description (optional).
Choose public or private visibility.
Initialize this repository with a README file (optional but recommended for initializing the repository with some content).
Click the "Create repository" button.
Cloning the Repository to Your Local Machine:
Copy Repository URL:
On the GitHub repository page, click the "Code" button.
Copy the repository URL (you can use HTTPS or SSH, depending on your preference).
Open Terminal or Command Prompt:
- Navigate to the directory where you want to clone the repository using the
cd
command.
- Navigate to the directory where you want to clone the repository using the
Clone the Repository:
Type the following command in the terminal, replacing
<repository_url>
with the URL you copied:git clone <repository_url>
Press Enter.
Navigate to the Cloned Directory:
Use the
cd
command to enter the cloned repository directory:cd <repository_name>
Now, you have successfully created a new repository on GitHub and cloned it to your local machine. You can start working on the project, make changes, and use Git commands to track and commit those changes. Remember to push your changes back to GitHub when you want to update the remote repository.
Example:
# Clone the repository
git clone https://github.com/your-username/your-repository.git
# Navigate to the cloned directory
cd your-repository
Make some changes to a file in the repository and commit them to the repository using Git.
Open the File:
- Use a text editor or any suitable tool to open the file you want to modify.
Make Changes:
- Add, modify, or delete content in the file according to your requirements.
Save the Changes:
- Save the changes you made to the file.
Check Status:
Open a terminal or command prompt.
Use the following command to check the status of your Git repository:
git status
This will show you the modified files.
Add Changes to Staging Area:
Use the following command to add the modified file to the staging area:
git add <filename>
Replace
<filename>
with the actual name of the file you modified.
Commit Changes:
Now, commit the changes with a meaningful commit message:
git commit -m "Your descriptive commit message here"
Verify Commit:
Verify that your commit was successful:
git log
- This will display the commit history, and you should see your latest commit at the top.
Push Changes to Remote (if needed):
If you're working with a remote repository on GitHub, push your changes to update the remote repository:
git push origin master
- Replace
master
with the branch name you are working on.
- Replace
Push the changes back to the repository on GitHub
Check the Status:
Open a terminal or command prompt.
Use the following command to check the status of your Git repository:
git status
This will ensure that you have the latest changes and that your working directory is clean.
Add Changes to Staging Area (if not done):
If you haven't added the changes to the staging area, use the following command:
git add <filename>
- Replace
<filename>
with the actual name of the file you modified.
- Replace
Commit Changes:
Commit the changes with a meaningful commit message:
git commit -m "Your descriptive commit message here"
Push Changes to GitHub:
Use the following command to push your changes to the GitHub repository:
git push origin master
- Replace
master
with the branch name you are working on.
- Replace
If it's your first time pushing, you might need to set the upstream branch. In that case, you can use:
git push -u origin master
Verify Changes on GitHub:
Go to your GitHub repository in a web browser.
You should see the changes you made reflected in the repository.
Subscribe to my newsletter
Read articles from Salman Hisamuddin Ansari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
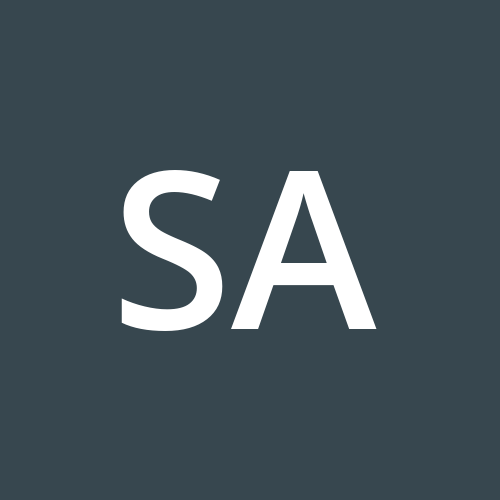