Day-11 | Python For DevOps | Python Real Time UseCase with Dictionaries
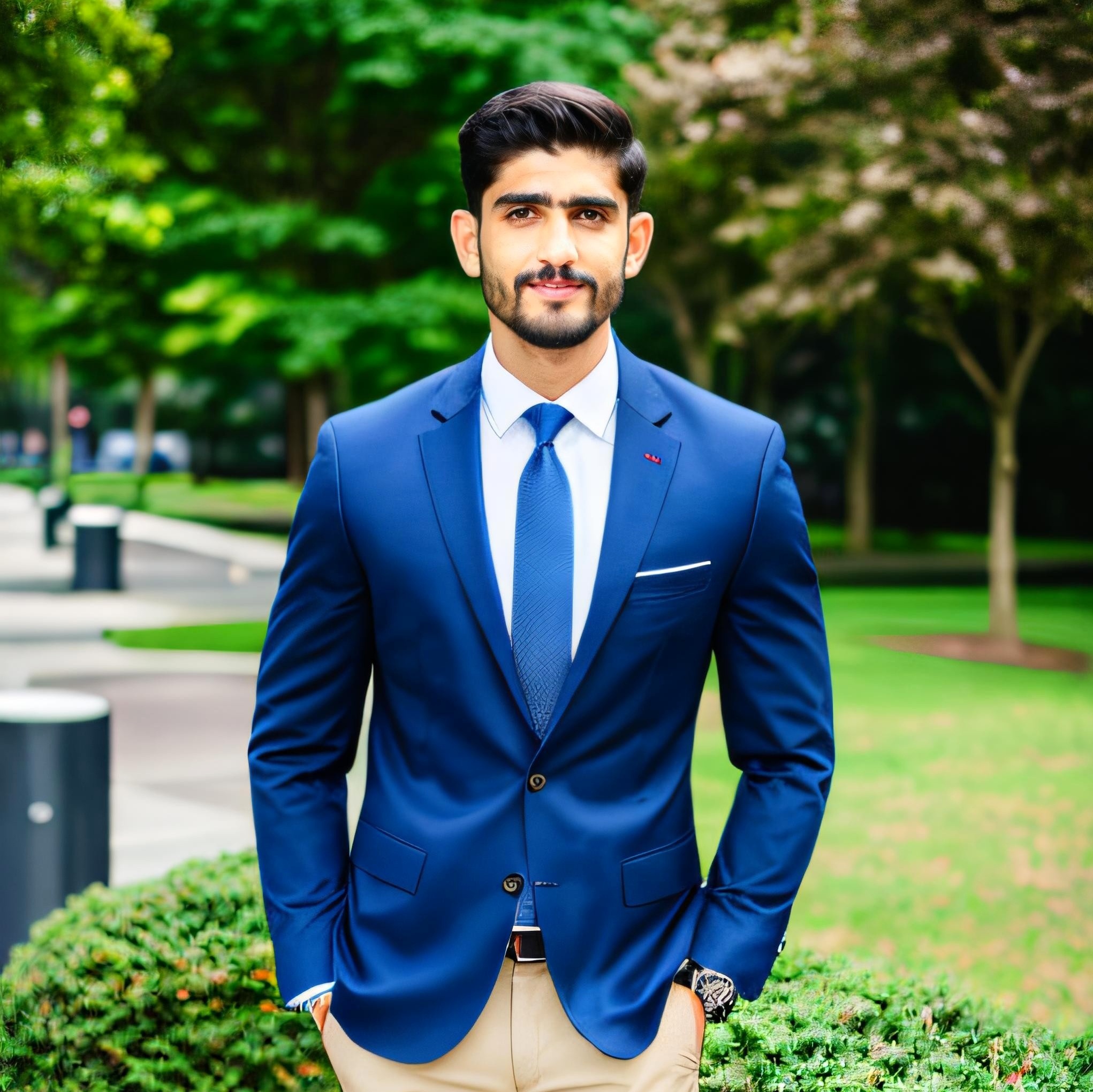
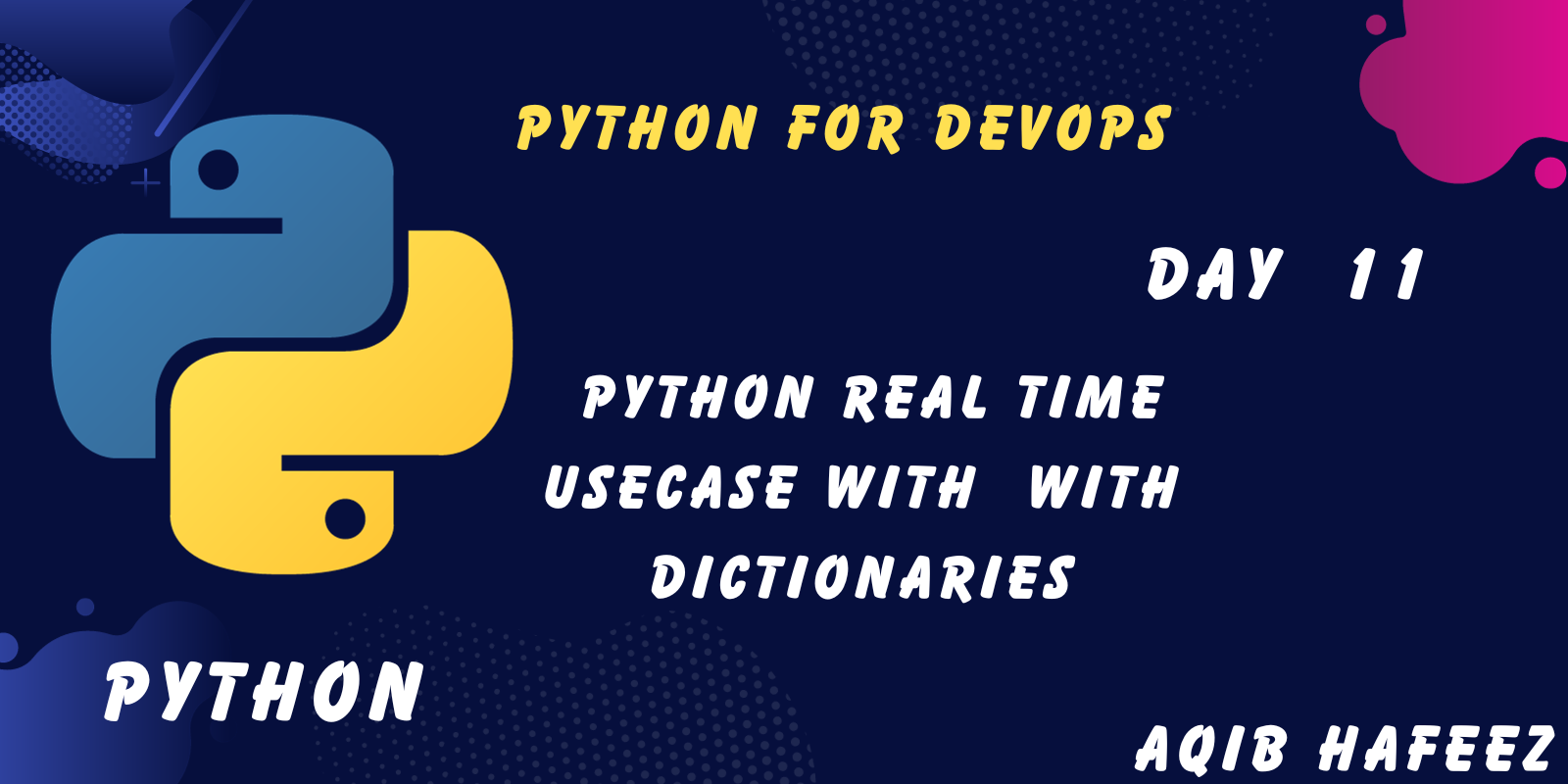
Dictionaries in Python
Definition: A dictionary in Python is an unordered collection of items. Each item in a dictionary has a key-value pair, where the key is a unique identifier and the value is the associated data. Dictionaries are defined using curly braces {}
and use a colon :
to separate keys and values.
Certainly! Let's start by discussing dictionaries in Python, comparing them with lists, and then exploring a use case in DevOps.
Example:
# Creating a dictionary
employee = {"name": "John", "age": 30, "role": "DevOps Engineer"}
Comparing Dictionaries with Lists
1. Uniqueness:
List: Elements in a list are ordered and can be accessed by index. Elements in a list can be duplicated.
Dictionary: Elements in a dictionary are accessed by keys. Keys must be unique, making each key associated with a unique value.
2. Order:
List: Elements in a list are ordered, and their order is maintained.
Dictionary: Elements in a dictionary are unordered, and their order is not guaranteed.
3. Use of Brackets:
List: Defined using square brackets
[]
.Dictionary: Defined using curly braces
{}
.
Use Case in DevOps
In the context of DevOps, both lists and dictionaries are valuable, but dictionaries offer a specific advantage when dealing with key-value pairs.
Example: Managing Configuration Parameters
Consider a scenario where you need to manage configuration parameters for multiple servers in your infrastructure.
# Using a list for server configurations
server_list = [
{"name": "web_server", "ip": "192.168.1.10", "port": 80},
{"name": "database_server", "ip": "192.168.1.20", "port": 3306},
{"name": "app_server", "ip": "192.168.1.30", "port": 8080}
]
# Using a dictionary for server configurations
server_dict = {
"web_server": {"ip": "192.168.1.10", "port": 80},
"database_server": {"ip": "192.168.1.20", "port": 3306},
"app_server": {"ip": "192.168.1.30", "port": 8080}
}
In this example, using a dictionary allows you to quickly retrieve the configuration of a specific server using its name as the key. This makes the code more readable and efficient compared to searching through a list.
Python for DevOps: A Real-world Use Case
Step 1: Managing EC2 Instance Information
Let's start by looking at a Python script that manages information about EC2 instances. The script uses dictionaries to store details about each EC2 instance, such as name, ID, and type.
ec2_instance_info = [
{
"Name": "ec2_1",
"id": "4567",
"type": "t2.micro"
},
{
"Name": "ec2_2",
"id": "9876",
"type": "t2.micro"
},
{
"Name": "ec2_3",
"id": "190423",
"type": "t2.micro"
}
]
# Accessing and printing information from the list of dictionaries
print(ec2_instance_info[2]["type"], ec2_instance_info[1]["id"])
In this script, we define a list called ec2_instance_info
, where each element is a dictionary representing an EC2 instance. The print
statement then extracts and prints specific information from the third and second instances.
Step 2: Making Requests to the GitHub API
Now, let's dive into making requests to the GitHub API using the requests
library. Before doing that, it's important to ensure that the library is imported. In an Integrated Development Environment (IDE), this is typically done at the beginning of the script.
import requests
This line tells Python to make the requests
library available for use in the script. Now, we can proceed to make a GET request to the GitHub API to fetch information about pull requests in the Kubernetes repository.
import requests
# Making a GET request to the GitHub API
response = requests.get("https://api.github.com/repos/kubernetes/kubernetes/pulls")
# Printing the JSON response
print(response.json())
In this snippet, we use the get
function from the requests
library to make a GET request to the specified GitHub API endpoint. The response is stored in the response
variable, and we print the JSON content of the response.
Subscribe to my newsletter
Read articles from AQIB HAFEEZ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
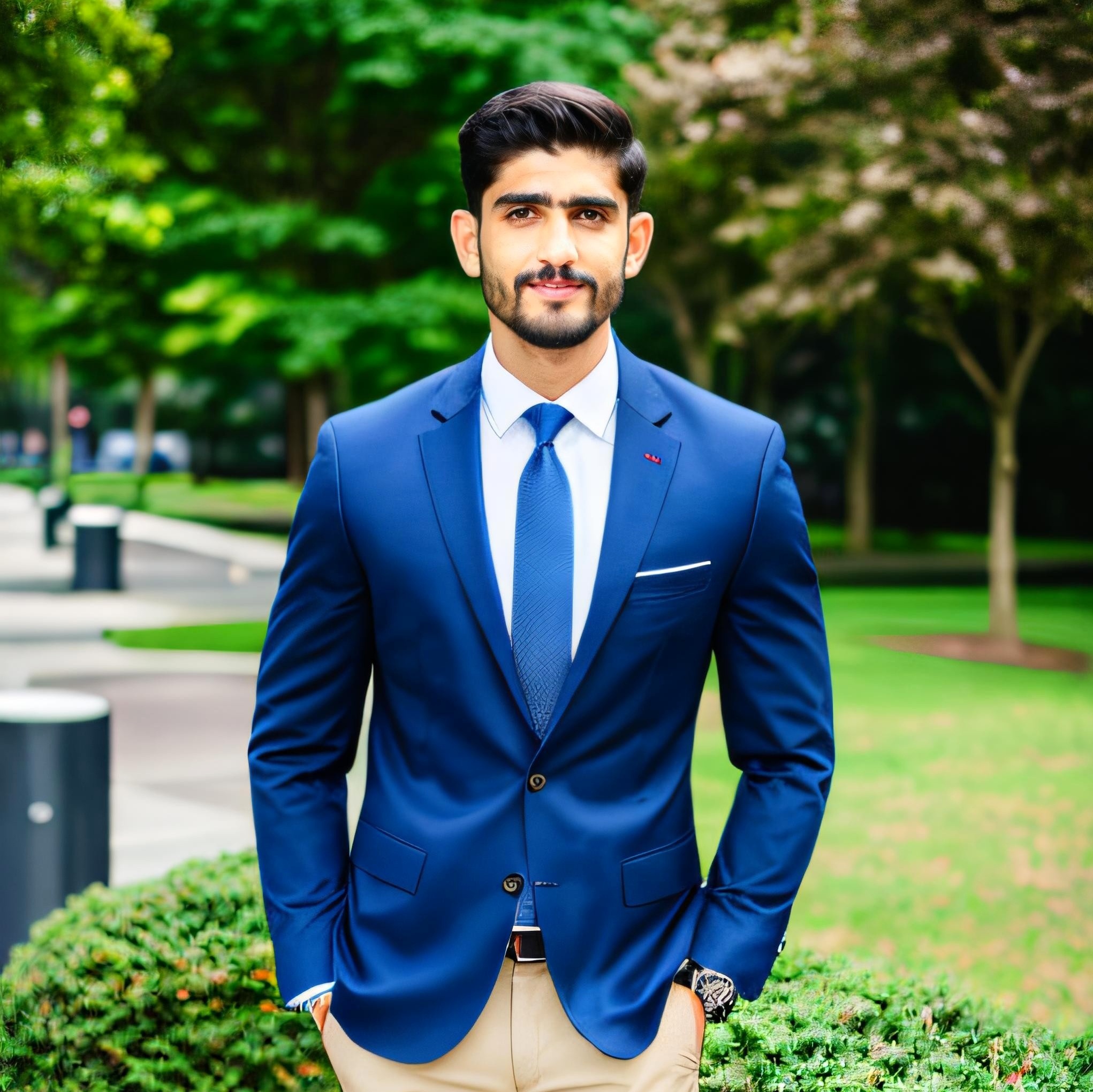
AQIB HAFEEZ
AQIB HAFEEZ
DevOps Engineer, Linux, Git, GitHub, GitLab, CI/CD pipelines, Jenkins, Docker, Kubernetes, Ansible & AWS. Practical experience in these DevOps tools enhances my passion for streamlined workflows, automated processes, and holistic development solutions. Proficient in digital and Facebook marketing, aiming to merge technical acumen with marketing finesse.