Programming paradigms

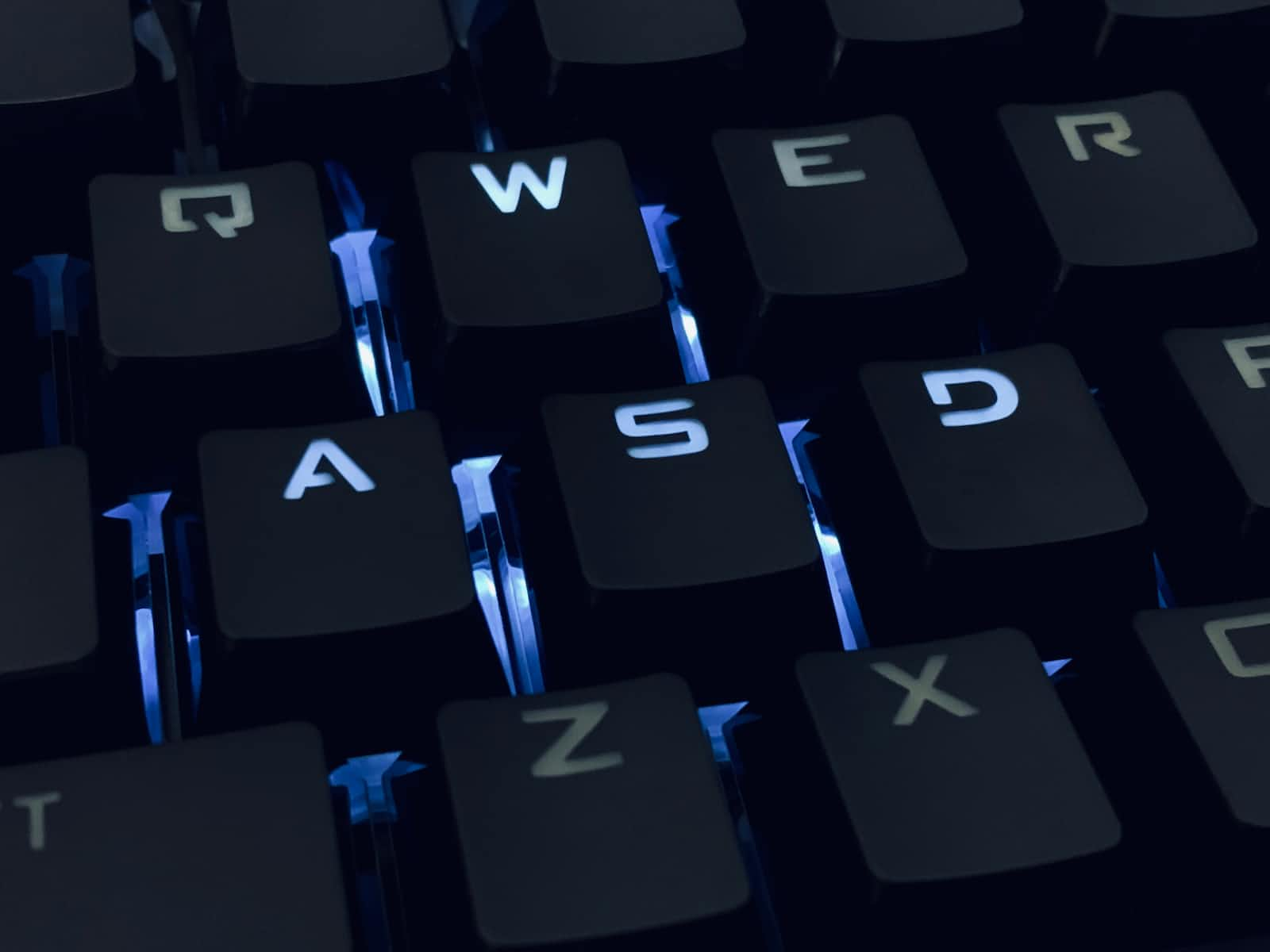
Programming paradigms
Programming paradigms are fundamental styles or approaches to writing computer programs. Each paradigm represents a distinct way of thinking about and structuring code. Here are some of the main programming paradigms:
Here are 15 programming paradigms:
Imperative Programming:
- Focuses on describing how a program operates through a sequence of statements that change a program's state. Common examples include C and Pascal.
Object-Oriented Programming (OOP):
- Based on the concept of "objects," encapsulating data and behavior. Involves concepts like encapsulation, inheritance, and polymorphism. Examples include Java and Python.
Functional Programming:
- Treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Examples include Haskell, Lisp, and Scala.
Declarative Programming:
- Specifies what needs to be achieved without explicitly stating how to achieve it. SQL is an example where you declare the desired result without specifying the steps to achieve it.
Event-Driven Programming:
- The flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs. JavaScript for web development is often used in an event-driven manner.
Parallel Programming:
- Focuses on the simultaneous execution of multiple tasks to improve performance. Can be achieved using multithreading or multiprocessing. Examples include C, C++, and Java.
Logic Programming:
- Programs are written in the form of logical statements. Prolog is a popular logic programming language.
Aspect-Oriented Programming (AOP):
- Allows the separation of cross-cutting concerns (e.g., logging, security) from the main business logic. AspectJ is an example of an aspect-oriented programming language.
Data-Driven Programming:
- Emphasizes the use and manipulation of data, often using databases and data structures as the central focus. SQL and some aspects of functional programming can be considered data-driven.
Concurrent Programming:
- Involves the execution of multiple tasks or processes simultaneously. This can be achieved through threading, multiprocessing, or other concurrency models. Languages like Python, Java, and Go support concurrent programming.
Distributed Programming:
- Involves writing programs that run on multiple interconnected computers. Socket programming, Remote Procedure Call (RPC), and message passing are common in distributed programming.
Service-Oriented Architecture (SOA):
- An architectural paradigm where software components, called services, communicate with each other over a network. Services are designed to provide specific business functionalities.
Reactive Programming:
- Focuses on asynchronous data streams and the propagation of changes. ReactiveX is a popular library for reactive programming.
Metaprogramming:
- Involves writing programs that manipulate other programs or treat programs as data. Macros in Lisp and template metaprogramming in C++ are examples.
Quantum Programming:
- Emerging paradigm for programming quantum computers. It involves using principles of quantum mechanics to perform computation. Qiskit (for IBM's quantum computers) and Cirq (for Google's quantum computers) are examples.
These paradigms represent different approaches to solving problems and building software, each with its strengths and weaknesses. The choice of paradigm often depends on the nature of the problem and the goals of the software project.
Here are five programming languages associated with each programming paradigm:
Imperative Programming:
C
Pascal
Fortran
Ada
COBOL
Object-Oriented Programming (OOP):
Java
C++
Python
Ruby
Smalltalk
Functional Programming:
Haskell
Lisp
Scala
Erlang
F#
Declarative Programming:
SQL
HTML
CSS
Prolog
XSLT
Event-Driven Programming:
JavaScript
Python (with event-driven libraries)
Java (with event handling)
C# (Windows Forms events)
Ruby (EventMachine)
Parallel Programming:
C
C++
Java
Python (with multiprocessing)
Go
Logic Programming:
Prolog
Mercury
Datalog
Oz
Logtalk
Aspect-Oriented Programming (AOP):
AspectJ (Java)
Spring AOP (Java)
PostSharp (C#)
AspectC++ (C++)
Python (with aspects library)
Data-Driven Programming:
SQL
Python (pandas library)
R
Apache Spark (using Scala or Python)
MATLAB
Concurrent Programming:
Java (with concurrency utilities)
Go
Erlang
Python (with threading)
C# (with Task Parallel Library)
Distributed Programming:
Java (RMI, JMS)
Python (with frameworks like Pyro)
C# (with WCF)
Erlang (natively supports distribution)
Scala (with Akka for distributed systems)
Service-Oriented Architecture (SOA):
Java (with JAX-WS, JAX-RS)
C# (with WCF)
Python (with Flask, Django REST framework)
Ruby (with Ruby on Rails)
Node.js (with Express)
Reactive Programming:
RxJava
ReactiveX (for multiple languages)
Akka (for Scala/Java)
ReactPHP (for PHP)
Bacon.js (for JavaScript)
Metaprogramming:
Lisp (with macros)
Ruby (with metaprogramming features)
C++ (with template metaprogramming)
Python (with metaclasses)
Groovy (with metaprogramming capabilities)
Quantum Programming:
Qiskit (for IBM's quantum computers, using Python)
Cirq (for Google's quantum computers, using Python)
Q# (for Microsoft's quantum computers)
Quipper (for quantum circuit description)
Rigetti Forest (for Rigetti Computing's quantum computers, using Python)
Subscribe to my newsletter
Read articles from Saifur Rahman Mahin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saifur Rahman Mahin
Saifur Rahman Mahin
I am a dedicated and aspiring programmer with a strong foundation in JavaScript, along with proficiency in key web development technologies like React, Next JS, Vue JS, Express JS, PHP, Laravel, MongoDB, and MySQL. I have a passion for creating interactive and dynamic web applications, and I'm committed to continuous learning and improvement in the ever-evolving world of programming. With my skills and enthusiasm, I'm excited to contribute to exciting projects and explore new opportunities in the field of web development.