Use OOPS in Java to create a number guessing game from 1 to 100?
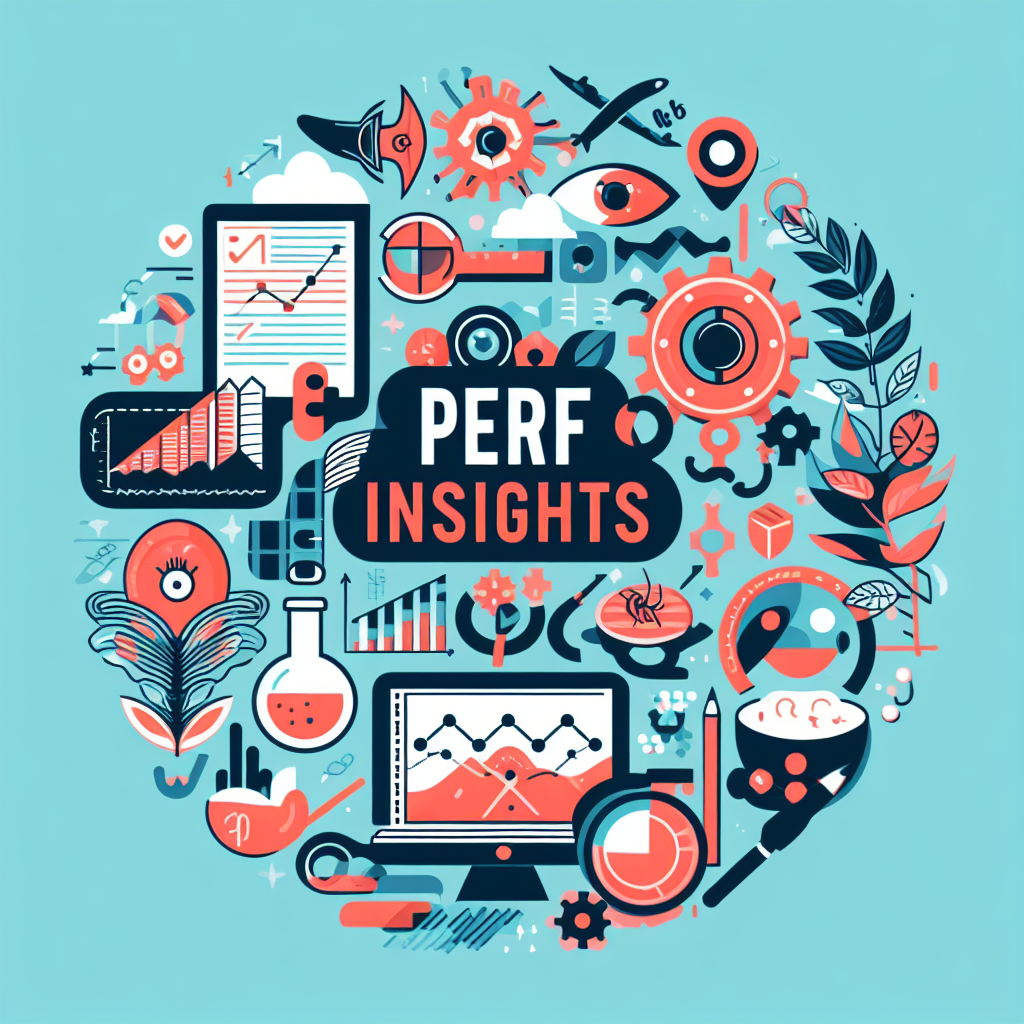
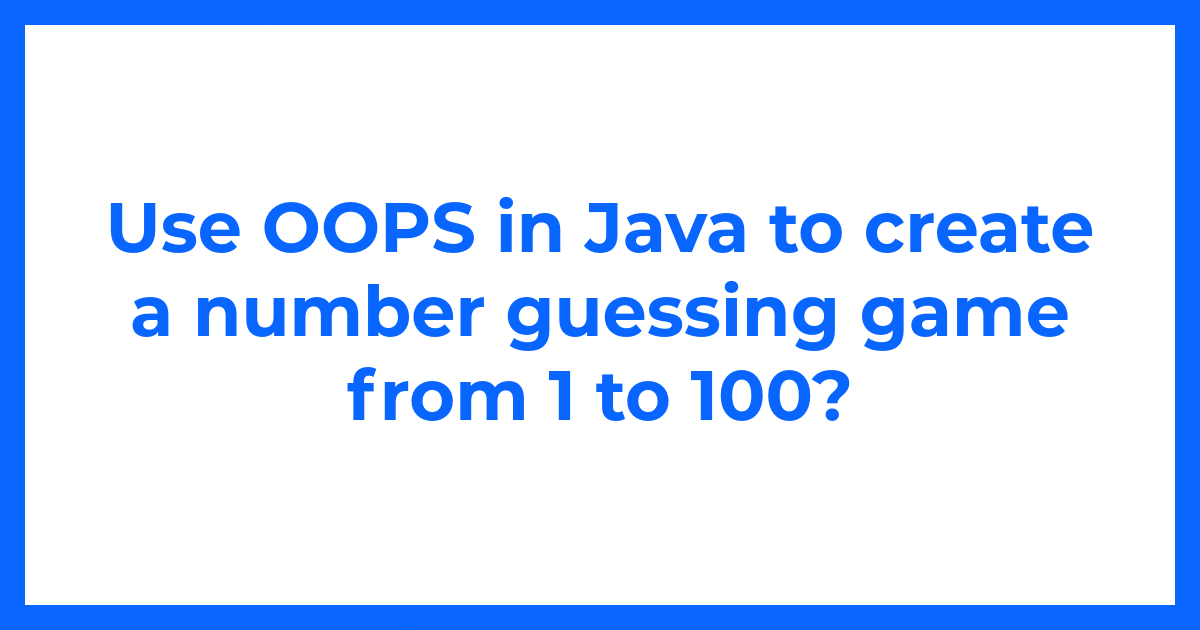
Taking user input and generating a random number in the below class.
// Importing required libraries
import java.util.Random;
import java.util.Scanner;
import static java.lang.System.in;
// Creating a class GuessNumb
class GuessNumb {
int randomNum; // Variable to store a random number
int userInput; // Variable to store user input
int attempt = 1; // Variable to track the number of attempts
boolean match = true; // Boolean to check if the guessed number matches
// Constructor to initialize the game
public GuessNumb() {
Random random = new Random();
this.randomNum = random.nextInt(1, 100); // Generating a random number between 1 and 100
System.out.println("Please guess numbers between 1-100 only");
}
// Method to take user input
public void takeUserInput() {
Scanner input = new Scanner(in);
this.userInput = input.nextInt();
}
// Method to check if the user's guess is correct
public void isCorrectNumber() {
while (match) { // Enters a loop until the correct number is guessed
if (userInput == randomNum) {
// If the user's guess matches the random number
System.out.println("Correct! Guessed " + userInput + " in " + attempt + " try");
match = false; // Set match flag to false to exit the loop
} else if (userInput < randomNum) {
// If the user's guess is smaller than the random number
System.out.println("Guess a number between 1-100 & larger than " + userInput);
takeUserInput(); // Take user input again
} else if (userInput > randomNum) {
// If the user's guess is larger than the random number
System.out.println("Guess a number between 1-100 & smaller than " + userInput);
takeUserInput(); // Take user input again
}
attempt++; // Increment the attempt counter
}
}
}
Initializing GuessNumb class for user input and number verification.
// Main class demo
public class demo {
public static void main(String[] args) {
GuessNumb game = new GuessNumb(); // Creating an instance of GuessNumb class
game.takeUserInput(); // Taking user input
game.isCorrectNumber(); // Checking if the guessed number is correct
} // End of main method
} // End of main class
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
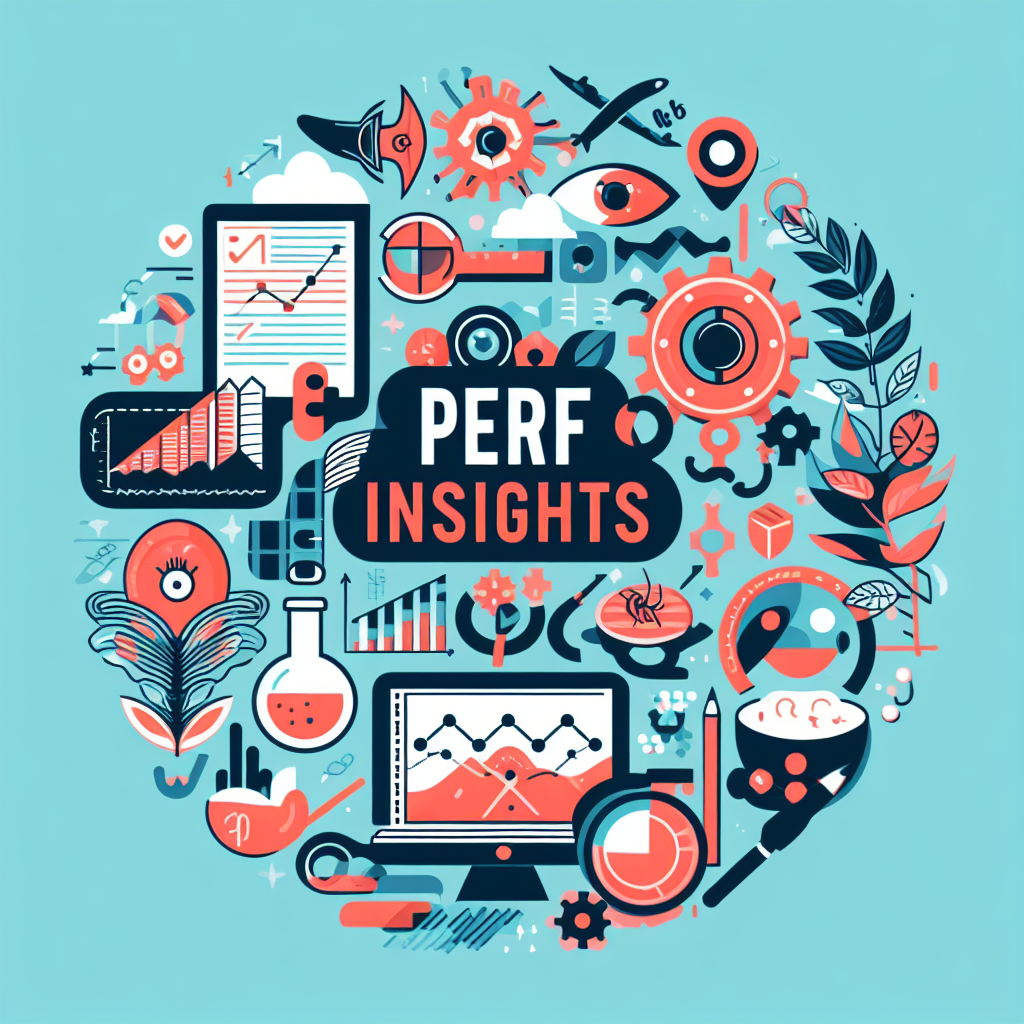
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.