Day 12: Python Tasks for DevOps (Part 1) - File Operations
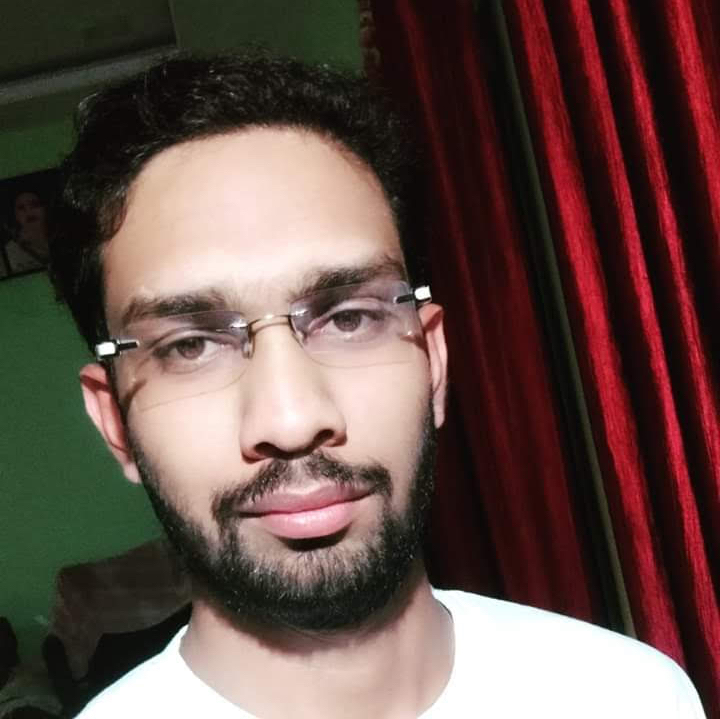
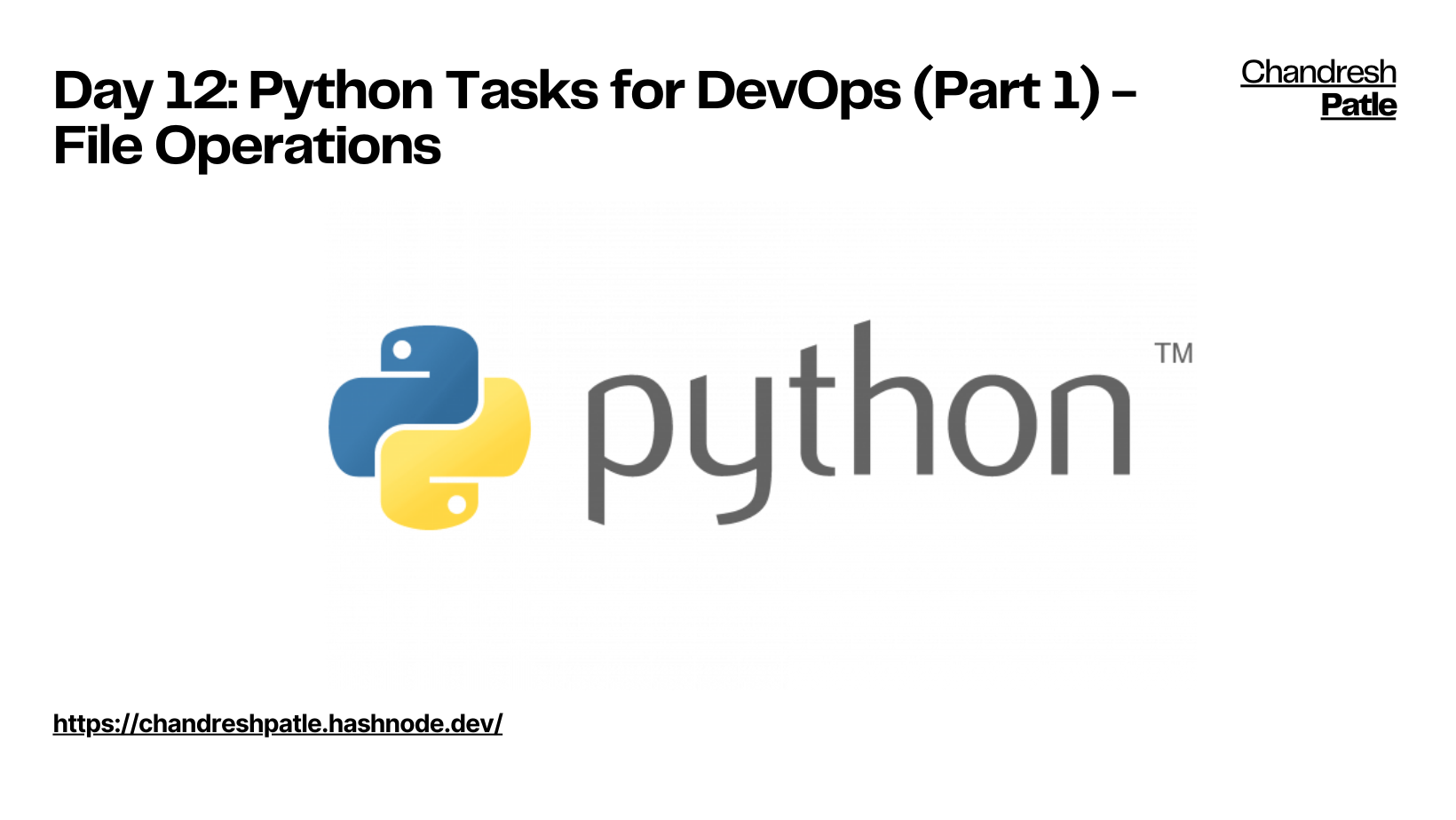
Welcome back to our Python for DevOps series! In today's installment, we'll focus on a crucial aspect of DevOps automation - File Operations. We'll explore the basics of working with files in Python. Let's dive into the world of automating file operations to enhance your DevOps toolkit.
🔶 Introduction to File Operations 🔶
✅ File Operations in Python
File operations are fundamental in the world of DevOps. Whether it's updating configuration files, analyzing log data, or managing server resources, understanding how to manipulate files programmatically is a key skill.
In Python, the os
modules provide powerful tools for file and directory operations. We'll explore how to read, write, and manipulate files using these modules, ensuring efficient and reliable automation in your DevOps scripts.
✅ Automating File Operations
Let's get hands-on with automating file operations using Python. We'll cover tasks such as reading and writing files, updating configuration files, and handling exceptions gracefully.
# Server Configuration File
# Network Settings
PORT = 8080
MAX_CONNECTIONS=1000
TIMEOUT = 30
# Security Settings
SSL_ENABLED = true
SSL_CERT = /path/to/certificate.pem
# Logging Settings
LOG_LEVEL = INFO
LOG_FILE = /var/log/server.log
# Other Settings
ENABLE_FEATURE_X = true
def update_server_config(file_path, key, value):
"""
Update a specific key-value pair in a configuration file.
Parameters:
- file_path (str): Path to the configuration file.
- key (str): The key to be updated.
- value (str): The new value to set for the key.
Returns:
- None
This function reads the content of the specified configuration file, searches for
the provided key, and updates its corresponding value. The updated content is then
written back to the file.
Example:
update_server_config("server.conf", "MAX_CONNECTIONS", "1200")
"""
# Read the content of the file
with open(file_path, "r") as file:
lines = file.readlines()
# Write back the updated content to the file
with open(file_path, "w") as file:
for line in lines:
if key in line:
# Update the line with the new key-value pair
file.write(key + "=" + value + "\n")
else:
# Keep the original line unchanged
file.write(line)
# Example usage
update_server_config("server.conf", "MAX_CONNECTIONS", "1200")
🔶 Conclusion
Mastering file operations in Python and leveraging Boto3 for cloud-based tasks opens up endless possibilities for DevOps automation. In Part 2, we'll explore more advanced Python tasks for DevOps, so stay tuned!
Note: I am following Abhishek Verraamalla's YouTube playlist for learning.
GitHub Repo: https://github.com/Chandreshpatle28/python-for-devops-av
Happy Learning :)
Stay in the loop with my latest insights and articles on cloud ☁️ and DevOps ♾️ by following me on Hashnode, LinkedIn (https://www.linkedin.com/in/chandreshpatle28/), and GitHub (https://github.com/Chandreshpatle28).
Thank you for reading! Your support means the world to me. Let's keep learning, growing, and making a positive impact in the tech world together.
#Git #Linux Devops #Devopscommunity #PythonforDevOps #python
Subscribe to my newsletter
Read articles from CHANDRESH PATLE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
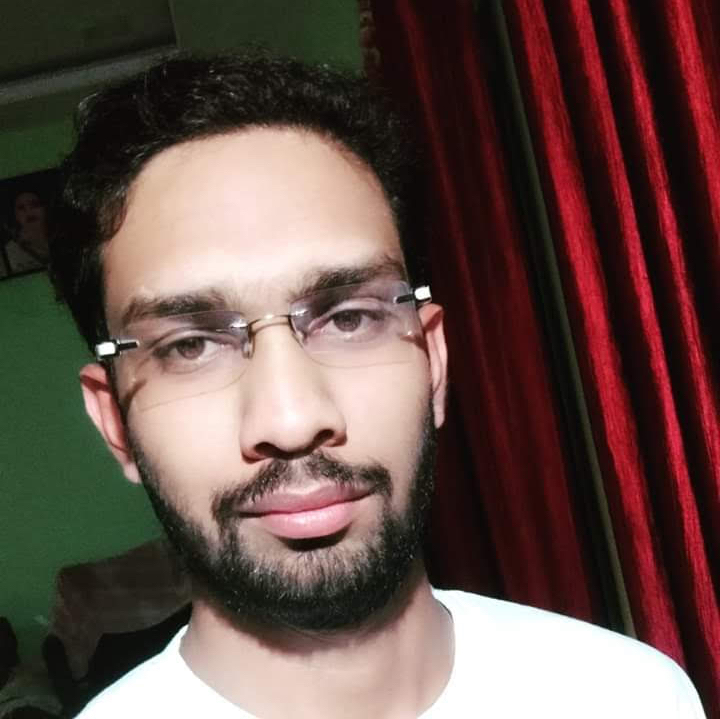
CHANDRESH PATLE
CHANDRESH PATLE
Hi, I'm Chandresh Patle, an aspiring DevOps Engineer with a diverse background in field supervision, manufacturing, and service consulting. With a strong foundation in engineering and project management, I bring a unique perspective to my work. I recently completed a Post Graduate Diploma in Advanced Computing (PG-DAC), where I honed my skills in web development, frontend and backend technologies, databases, and DevOps practices. My proficiency extends to Core Java, Oracle, MySQL, SDLC, AWS, Docker, Kubernetes, Ansible, Linux, GitHub, Terraform, Grafana, Selenium, and Jira. I am passionate about leveraging technology to drive efficient and reliable software delivery. With a focus on DevOps principles and automation, I strive to optimize workflows and enhance collaboration among teams. I am constantly seeking new opportunities to expand my knowledge and stay up-to-date with the latest industry trends. If you have any questions, collaboration ideas, or professional opportunities, feel free to reach out to me at patle269@gmail.com. I'm always open to connecting with fellow tech enthusiasts and exploring ways to contribute to the DevOps community. Let's build a better future through innovation and continuous improvement!