DSA - Remove Nth Node From End of List

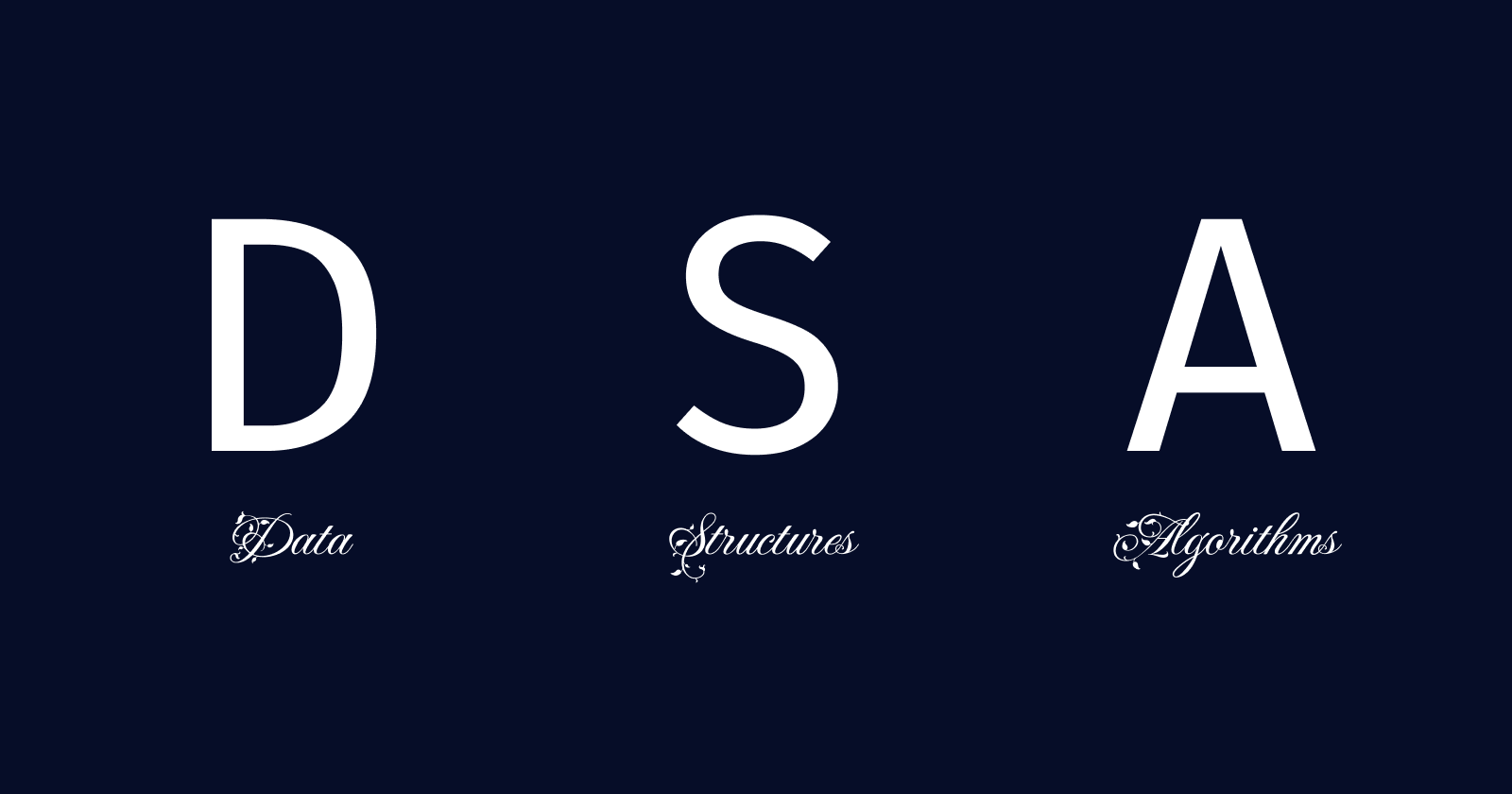
Problem
Given the head
of a linked list, remove the n<sup>th</sup>
node from the end of the list and return its head.
List: [1] -> [2] -> [3] -> [4] -> [5]
Value of N: 2
Output: [1] -> [2] -> [3] -> [5]
Given Code
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
Solution
Logical
Let's break down this code in simpler terms:
Check if
n
is 0:if n <= 0: return head
This part checks if the value of
n
is zero. If it is, the function immediately returns the original list (head
) without making any changes.Calculate the Length of the List:
curr = head len = 0 while curr: len += 1 curr = curr.next
This section counts how many nodes are in the given linked list (
head
). It does this by moving through the list node by node and keeping track of the count (len
).Check if
n
is Greater than the Length of the List:if n > len: return None
If the value of
n
is greater than the length of the list, the function returnsNone
because it means we can't remove then
-th node from the end of the list if the list is shorter thann
.Determine the Position of the Node to Remove from the Beginning:
nodeFromFirst = len - n + 1
It calculates the position of the node that needs to be removed from the beginning of the list. For example, if
n
is 2, and the list has 5 nodes, it calculates the position of the node to remove as5 - 2 + 1 = 4
.Initialize Two Lists:
returnList = updateList = ListNode()
It initializes two lists (
returnList
andupdateList
) with an empty node. These lists will be used to construct the modified list.Remove the N-th Node from the End:
for i in range(nodeFromFirst): if i == nodeFromFirst - 1: returnList.next = head.next break else: returnList.next = ListNode(head.val) returnList = returnList.next head = head.next
This loop iterates through the original list up to the position calculated earlier (
nodeFromFirst
). It creates a new list (returnList
) excluding the node to be removed. The loop uses a temporary list (updateList
) to keep track of the modified list.Return the Modified List:
return updateList.next
Finally, it returns the modified list starting from the node after the initially created empty node (
updateList
).
In simpler terms, this code removes the N-th node from the end of a linked list. It first checks if n
is zero or greater than the length of the list. Then, it calculates the position of the node to remove, creates a new list without that node, and returns the modified list.
Code
class Solution:
def removeNthFromEnd(self, head: Optional[ListNode], n: int) -> Optional[ListNode]:
if not bool(n):
return head
curr = head
len = 0
while curr:
len += 1
curr = curr.next
if n > len:
return None
nodeFromFirst = len - n + 1
returnList = updateList = ListNode()
for i in range(nodeFromFirst):
if i == nodeFromFirst - 1:
returnList.next = head.next
break
else:
returnList.next = ListNode(head.val)
returnList = returnList.next
head = head.next
return updateList.next
Subscribe to my newsletter
Read articles from Ritwik Math directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ritwik Math
Ritwik Math
As a seasoned senior web developer with a wealth of experience in Python, Javascript, web development, MySQL, MongoDB, and React, I am passionate about crafting exceptional digital experiences that delight users and drive business success.