#Day 15 - Python Libraries

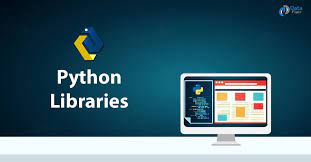
Reading JSON and YAML in Python
As a DevOps Engineer, one of the essential skills you need to have is the ability to work with various file formats, including JSON and YAML. These formats are commonly used in configuration management, infrastructure as code, and other DevOps tasks. In this article, we'll explore how to perform two crucial tasks:
Creating a Python Dictionary and Writing it to a JSON File.
Reading a JSON file and printing the service names of different cloud service providers.
Reading a YAML file using Python and converting it to JSON.
Libraries for DevOps in Python
Python is a versatile language with a rich ecosystem of libraries that are incredibly useful for DevOps tasks. Some of the key libraries include:
os: The os module allows you to interact with the operating system, making it essential for tasks such as file and directory manipulation.
sys: The sys module provides access to Python interpreter variables and functions, helping you control and customize Python runtime environments.
json: The json library is invaluable for working with JSON data, which is commonly used for configuration and data exchange in DevOps.
yaml: The yaml library allows you to work with YAML data, which is another popular format in the DevOps world for configuration files.
Now, let's dive into the tasks.
Task 1: Create a Dictionary and Write it to a JSON File
To create a Python dictionary and write it to a JSON file, you can use the json library. Here's an example:
import json
data = {
"aws": "ec2",
"azure": "VM",
"gcp": "compute engine"
}
with open('services.json', 'w') as json_file:
json.dump(data, json_file)
print("Dictionary written to services.json")
In this code snippet, we define a dictionary data with cloud service provider names and their corresponding services. We then use json.dump to write this dictionary to a file named services.json.
Task 2: Read a JSON File and Print Service Names
To read a JSON file and print the service names of different cloud providers, you can use the json library again. Here's an example:
import json
with open('services.json', 'r') as json_file:
data = json.load(json_file)
for provider, service in data.items():
print(f"{provider} : {service}")
This code reads the services.json file and prints the cloud service provider and the associated service name.
Task 3: Read a YAML File and Convert it to JSON
To read a YAML file using Python and convert it to JSON, you can use the pyyaml library, which is commonly used for working with YAML data. First, you need to install the library using pip:
pip install pyyaml
Now, let's read a YAML file and convert it to JSON:
import yaml
import json
with open('services.yaml', 'r') as yaml_file:
yaml_data = yaml.safe_load(yaml_file)
# Convert YAML data to JSON
json_data = json.dumps(yaml_data, indent=2)
print(json_data)
In this code, we read a YAML file using yaml.safe_load and then use json.dumps to convert the YAML data to JSON format.
As a DevOps engineer, these tasks and libraries are just the beginning of what you can do with Python. With the power of Python and its extensive library ecosystem, you can automate and streamline many DevOps processes, making your job more efficient and effective.
Subscribe to my newsletter
Read articles from Shefali Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
