How to Integrate CKEditor5 into a Vue.js Application: A Developer Guide

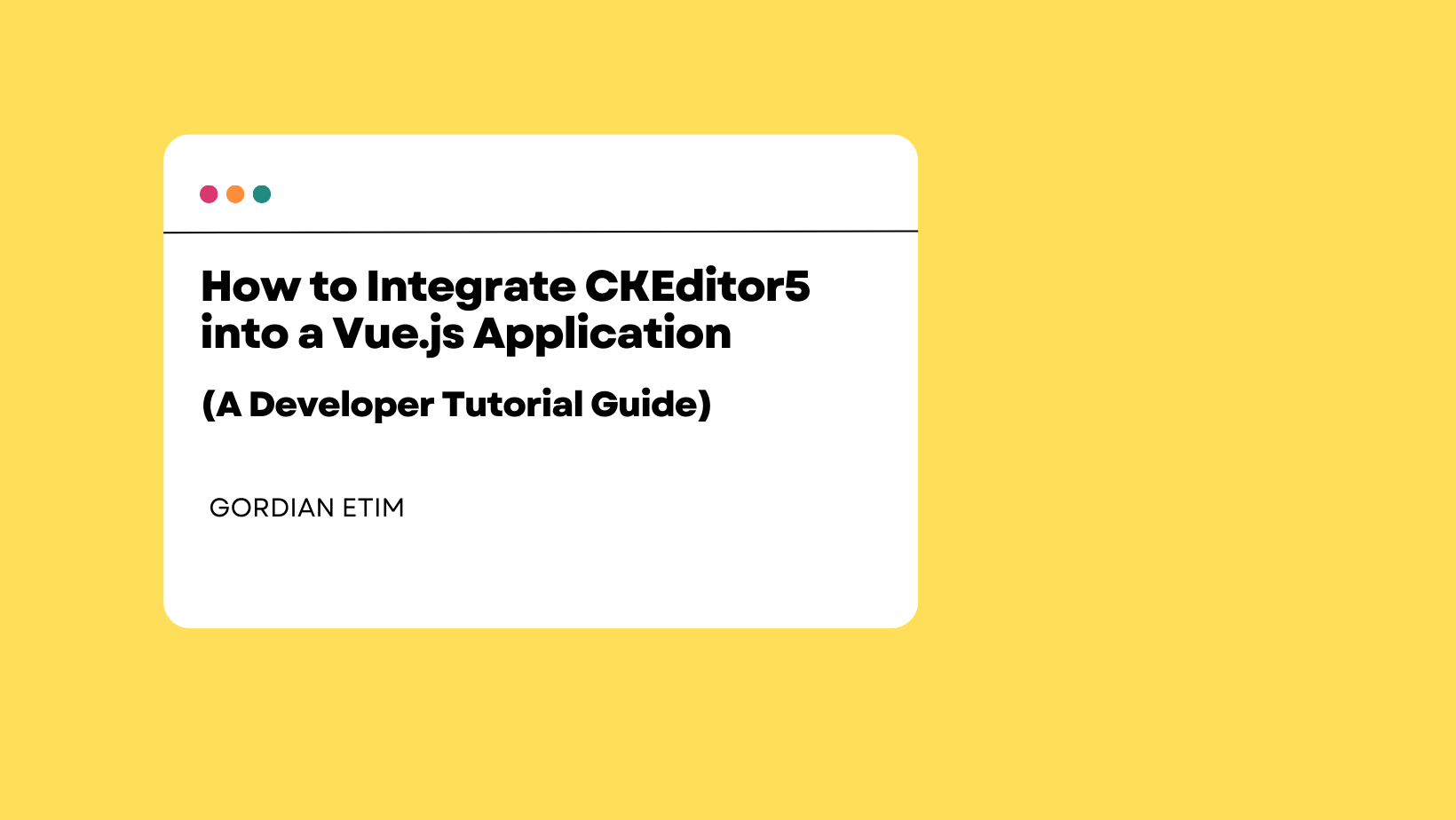
Introduction
CKEditor 5 is a versatile WYSIWYG text editor, and integrating it into a Vue.js application can enhance the content creation experience. In this guide, we'll walk through the process of integrating CKEditor into a Vue.js application, covering the setup, component integration, and data binding with Vue.js.
Prerequisites
Before we start, make sure you have the following installed:
Node.js and npm (Node Package Manager): Download Node.js
Vue CLI: Install using
npm install -g @vue/cli
Troubleshooting npm Installation Issues
If you encounter an error running the Vue CLI installation command, it may be related to insufficient permissions. Here are two solutions to address this issue:
1. Fix npm Permissions:
It seems like your npm global packages directory (/usr/local/lib/node_modules/
) is owned by the root user. You can change the ownership of the directory to your user account. Open a terminal and run:
sudo chown -R $(whoami) /usr/local/lib/node_modules/
This command recursively changes the ownership of the npm global packages directory to the current user.
After running this command, try installing the package again:
npm install -g @vue/cli
2. Use Node Version Manager (nvm):
Instead of using the system-wide npm, you can use Node Version Manager (nvm) to install and manage Node.js and npm versions. NVM installs packages in your home directory, avoiding the need for superuser permissions.
Follow these steps:
Install nvm:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
Close and reopen your terminal to start using nvm.
Install Node.js and npm using nvm:
nvm install node
This command installs the latest version of Node.js along with npm.
Use the installed Node.js version:
nvm use node
After using nvm, try installing the package again:
npm install -g @vue/cli
This step avoids the need for superuser permissions when installing global packages.
Step 1: Setting Up Your Vue Project
Create a new Vue project using Vue CLI:
vue create vue-ckeditor-integration
cd vue-ckeditor-integration
Step 2: Installing CKEditor
Install CKEditor and the CKEditor Vue component:
npm install --save @ckeditor/ckeditor5-vue @ckeditor/ckeditor5-build-classic
Step 3: Creating the CKEditor Component
Create a new file, CKEditor.vue
, in the src/components
directory. This file will contain your CKEditor component. Here's a basic setup:
<!-- src/components/CKEditor.vue -->
<template>
<div>
<ckeditor :editor="editor" v-model="data" @input="onEditorInput" />
</div>
</template>
<script>
import CKEditor from '@ckeditor/ckeditor5-vue';
import ClassicEditor from '@ckeditor/ckeditor5-build-classic';
export default {
components: {
ckeditor: CKEditor.component,
},
data() {
return {
editor: ClassicEditor,
data: '',
};
},
methods: {
onEditorInput({ editor, data }) {
this.data = data;
},
},
};
</script>
<style scoped>
/* Add any necessary styling for your CKEditor component */
</style>
Step 4: Using the CKEditor Component in Your App
In your src/App.vue
file, import and use the CKEditor
component:
<!-- src/App.vue -->
<template>
<div id="app">
<h1>CKEditor + Vue.js Integration</h1>
<CKEditor />
<div>
<h2>Editor Content:</h2>
<div v-html="editorContent"></div>
</div>
</div>
</template>
<script>
import CKEditor from './components/CKEditor.vue';
export default {
components: {
CKEditor,
},
data() {
return {
editorContent: '',
};
},
watch: {
'CKEditor.data': {
handler(newValue) {
this.editorContent = newValue;
},
immediate: true,
},
},
};
</script>
<style>
/* Add any necessary global styling for your app */
</style>
Step 5: Testing CKEditor Integration
Run your Vue app:
npm run server
Now, visit http://localhost:8080
in your browser. You should see your CKEditor successfully integrated into your Vue.js application. Start typing and formatting, and you'll notice the real-time updates.
Conclusion
This guide provides a step-by-step procedure on how to set up and integrate your CKEditor5 with VueJs. Learn more and get access to more docs and code guides in CKEditor documentation.
Subscribe to my newsletter
Read articles from Gordian Etim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gordian Etim
Gordian Etim
Developer Relations Advocate specializing in content creation, community management, and simplifying technologies for developers and users.