Ada: Loops
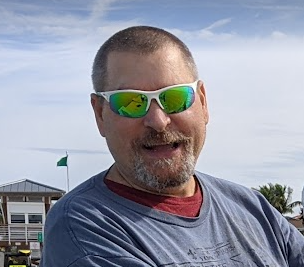
Table of contents
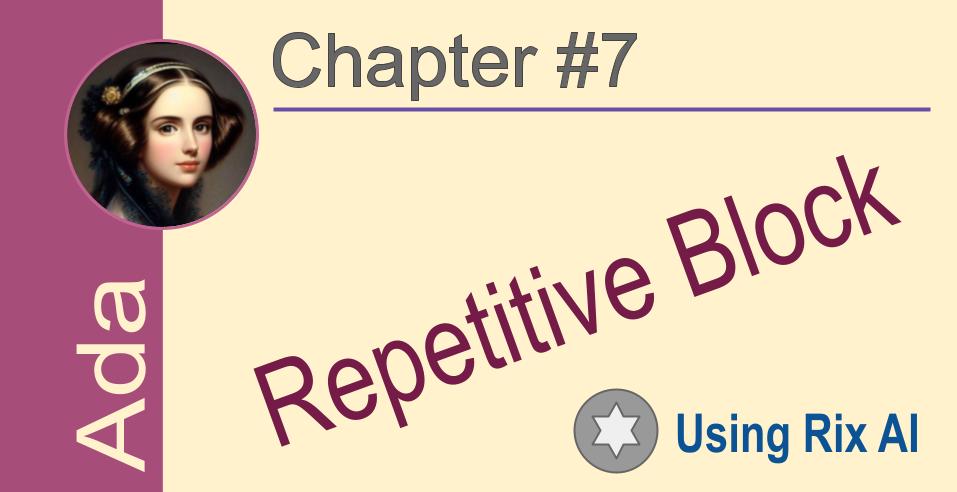
Repetitive Blocks
Repetitive blocks in Ada are used to execute a block of code repeatedly for a fixed number of times or until a condition is met. They have the following forms:
For loop:
for iterator in [reverse] loop_range loop -- Block of code end loop;
Where:
iterator is the loop variable
loop_range specifies the number of iterations, e.g. 1..10
reverse makes the loop count down instead of up
Example:
for i in 1..5 loop
Put_Line(i);
end loop;
This will print numbers from 1 to 5.
While loop:
while condition loop -- Block of code end loop;
Example:
counter := 1;
while counter <= 5 loop
Put_Line(counter);
counter := counter + 1;
end loop;
This will also print numbers from 1 to 5.
Exit loop: This statement exits the innermost enclosing loop.
Next loop: This statement skips the rest of the current iteration and starts the next iteration.
So in summary, Ada uses for loops, while loops, exit and next statements to execute a block of code repeatedly. The block of code can contain declarations and statements.
Labels
Labels in Ada are used to identify loops so that they can be targeted by exit and next statements. They have the following syntax:
label: for/while loop
Where label is an identifier for the loop.
For example:
outer:
for i in 1..3 loop
inner:
for j in 1..3 loop
if j = 2 then
exit inner; -- Exits the inner loop
end if;
end loop inner;
end loop outer;
Here we have:
An outer for loop labeled as outer
An inner for loop labeled as inner
An exit statement that exits the inner labeled loop using its label inner
This allows exiting specific loops when nested loops are used.
An example of nested loops with labels would be:
outer:
for i in 1..3 loop
inner:
for j in 1..3 loop
Put_Line(i || " " || j);
exit outer when i = 2;
exit inner when j = 2;
end loop inner;
end loop outer;
This will print:
1 1
1 2 1 3 2 1
Since we exit the outer loop when i becomes 2.
So in summary, labels in Ada allow:
Identifying specific loops
Exiting specific loops using exit statements targeting their labels
Useful when nested loops are used
Local scope
A control variable is a variable that is used to control the iterations of a for loop. It is usually declared within the for loop and has a local scope.
For example, in the following for loop:
for I in 1..5 loop
-- Loop body
end loop;
Here I is the control variable. It is declared within the for loop and has a local scope, meaning:
I is only visible and accessible within the for loop.
After the loop exits, I goes out of scope and cannot be accessed anymore.
This local scope of the control variable has a few benefits:
Encapsulation: The control variable is encapsulated within the for loop and does not pollute the outer scope.
Memory management: Since the control variable goes out of scope after the loop exits, its memory is automatically released.
Avoiding variable name clashes: Since the control variable has a local scope, the same variable name can be used in other for loops without clashes.
Simplicity: The local scope keeps the control variable tightly coupled to the for loop, making the code simpler.
To achieve a wider scope for the control variable, we can declare it before the for loop:
I : Integer := 0;
for I in 1..5 loop
-- Loop body
end loop;
-- I is still accessible here
Here I has a wider scope since it is declared before the for loop.
So in summary, the control variable of a for loop in Ada:
Is usually declared within the for loop, giving it a local scope
This local scope has several benefits like encapsulation, memory management and simplicity
But a wider scope can be achieved by declaring the variable before the for loop
Hope this explanation of control variables and local scope for loops in Ada was helpful! Let me know if you have any other questions.
Disclaim: This article was created with Rix (AI).
Subscribe to my newsletter
Read articles from Elucian Moise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
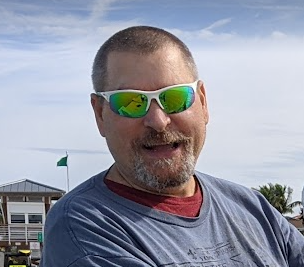
Elucian Moise
Elucian Moise
Software engineer instructor, software developer and community leader. Book author. Computer enthusiast and experienced programmer. Born in Romania, living in US.