Mastering JavaScript Arrays: Unleashing the Power of 7 Essential Methods
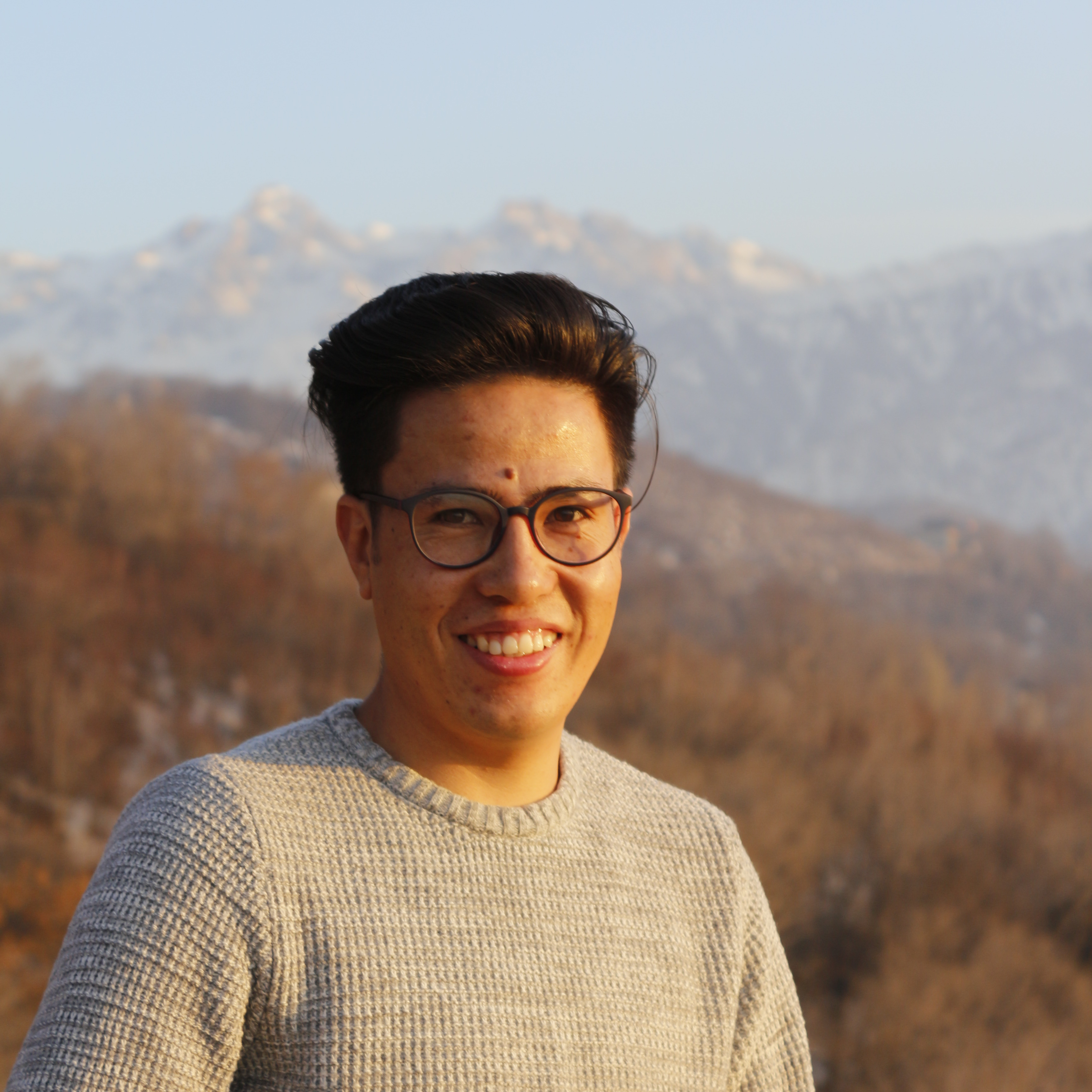
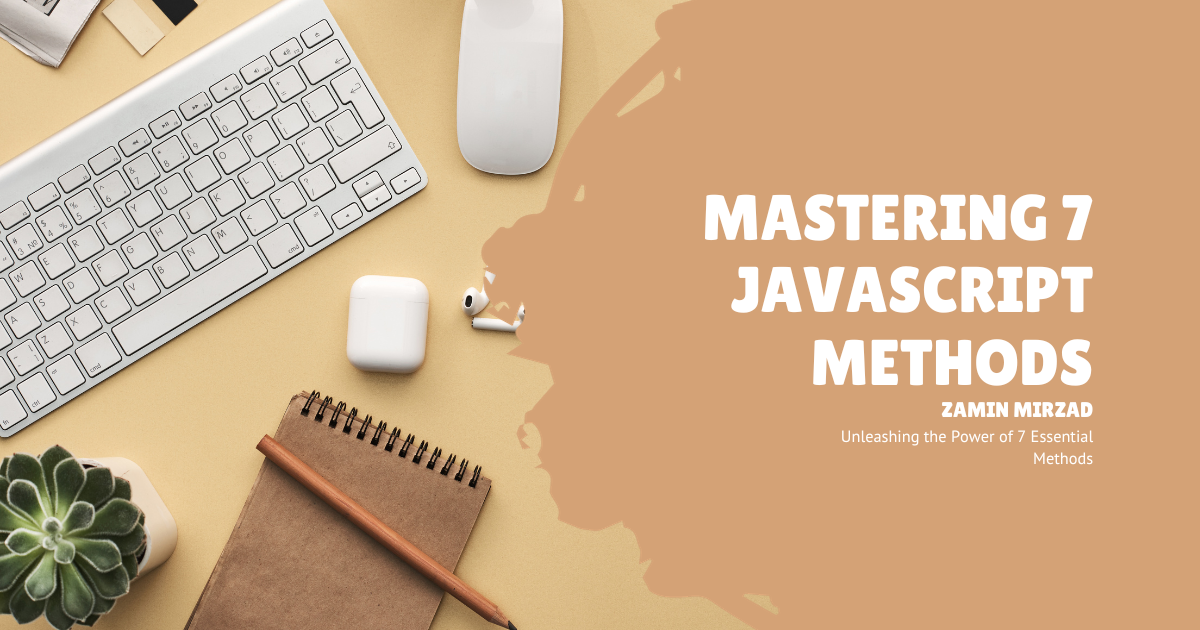
JavaScript Arrays are a fundamental component of web development, enabling developers to efficiently store and manipulate data. In this guide, we'll delve into 7 essential Array methods, providing detailed explanations and optimizing your code with two practical examples. Elevate your JavaScript proficiency as we explore the power of these methods.
map()
: Transforming Elements with EaseThe
map()
method is a powerful tool for transforming each element in an array without modifying the original array. It takes a callback function as an argument, applies it to each element, and returns a new array of the transformed elements.Example:
{
const originalArray = [1, 2, 3, 4];
const squaredArray =
originalArray.map
(num => num ** 2);
// Result: squaredArray = [1, 4, 9, 16]
}
filter()
: Filtering Arrays with PrecisionThe
filter()
method allows you to create a new array containing only elements that pass a specified condition. It takes a callback function that evaluates each element and returns true or false.Example:
{
const numbers = [10, 15, 20, 25];
const greaterThanFifteen = numbers.filter(num => num > 15);
// Result: greaterThanFifteen = [20, 25]
}
reduce()
: Condensing Arrays EffectivelyThe
reduce()
method condenses an array into a single value. It iterates through the array, applying a callback function that accumulates a result. The final result is the condensed value.Example:
{
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, num) => acc + num, 0);
// Result: sum = 10
}
forEach()
: Iterating with FinesseforEach()
is used for executing a provided function once for each array element. Unlike other methods, it does not create a new array but is excellent for performing actions on each element.Example:
{
const fruits = ['apple', 'banana', 'orange'];
fruits.forEach(fruit => console.log(I love ${fruit}s!));
// Result: Logs "I love apples!", "I love bananas!", "I love oranges!"
}
find()
: Discovering the Perfect MatchThe
find()
method returns the first array element that satisfies a specified condition. It takes a callback function and stops iterating once it finds a match.Example:
{
const numbers = [5, 10, 15, 20];
const found = numbers.find(num => num > 10);
// Result: found = 15
}
indexOf()
: Locating Elements EfficientlyindexOf()
returns the index of the first occurrence of a specified element in an array. If the element is not found, it returns -1.Example:
{
const colors = ['red', 'blue', 'green'];
const indexOfBlue = colors.indexOf('blue');
// Result: indexOfBlue = 1
}
slice()
: Extracting Subarrays with PrecisionThe
slice()
method extracts a section of an array and returns a new array. It takes two parameters: the starting index and the ending index (exclusive).Example:
{
const animals = ['lion', 'elephant', 'giraffe', 'zebra'];
const selectedAnimals = animals.slice(1, 3);
// Result: selectedAnimals = ['elephant', 'giraffe']
}
Conclusion:
Mastering these 7 essential JavaScript Array methods provides you with a robust toolkit for efficient data manipulation. Enhance your coding skills, optimize your applications, and elevate your development experience with these powerful techniques. Start incorporating these methods into your projects today for a cleaner, more effective JavaScript code!
Don't forget to Like โฅ๏ธ, Comment ๐ฌ and share ๐ฒ for good. Follow ๐ Mohammad Zamin Mirzad for more topics like this ๐
Subscribe to my newsletter
Read articles from Zamin Mirzad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
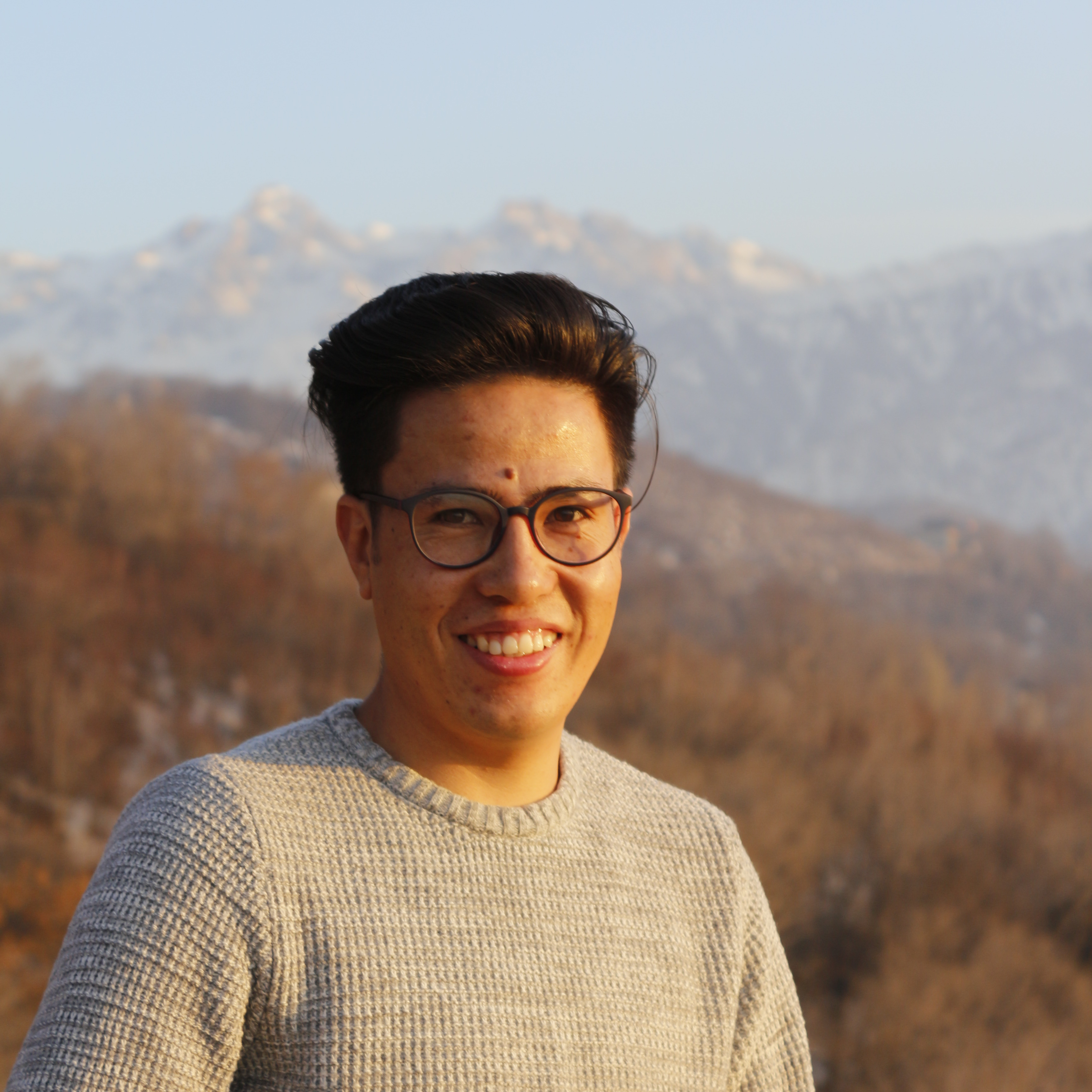
Zamin Mirzad
Zamin Mirzad
A Frontend Developer in all cycles of the software development life cycle from design, development to maintenance. I've worked on a variety of projects for clients in different cases. I'm passionate about programming with strong communication skills, creating great user interfaces and have a good understanding of modern tech stacks for web development. Enjoy working on challenging projects in an agile environment and in multi-cultural environment.