TextButton.styleFrom() in Flutter - Tutorial

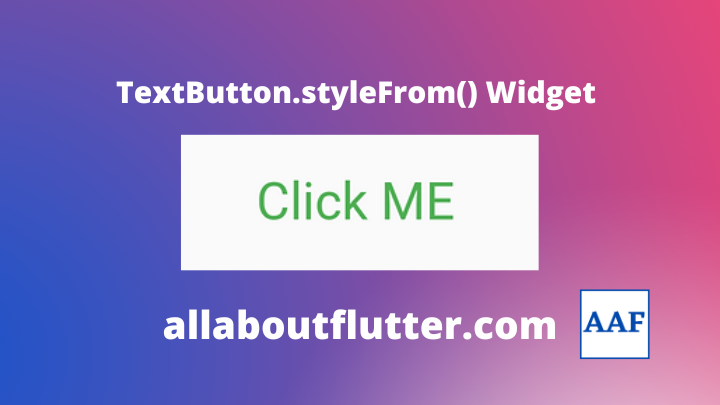
TextButton widget in Flutter is used to provide a transparent background by default and usually adds buttons containing the Text widget.
In this tutorial, we are going to learn to style the TextButton widget using the TextButton.styleForm() option.
Parameters: We use the following parameters:
primary: It is the text fill colour of the Button.
onPrimary: It is the default colour or the overlay colour of the Button Text.
onSurface: Similar to onPrimary.
shadowColor: It is used to set the shadowColor.
surfaceTintColor: It is used to set the surface tint colour.
elevation: It takes a double type of value and is used to set the shadow distance.
textStyle: It is used to set the TextStyle of the child widget if it is a TextWidget.
padding: It is used to set the padding of the Button with its child.
minimumSize: The minimum size the button should have.
fixedSize: It is used to fix the size of the button.
maximumSize: Here we need to specify the maximum size that the button could have.
side: It is used to set the border side.
shape: We can change the shape of the border with this field.
enabledMouseCursor: If true, the mouse cursor can be used on it.
disabledMouseCursor: If true, the mouse cursor is disabled.
visualDensity: It is used to set the visual density.
tapTargetSize: It is used to set the radius where the tap would be recognized from the button.
animationDuration: It is used to set the duration of the animation of the splash effect.
enableFeedback: If true, the HapticFeedback is turned on.
alignment: It is used to set the alignment of the contents of the Button.
splashFactory: We can set our own InkSplash with this.
Syntax: Apply the style of TextButton as follows:
TextButton( onPressed: () {}, style: TextButton.styleFrom( primary: Colors.green, ), child: const Text( "Click ME", ), ),
Example: In the following example, we have some TextButtons with different primary colours.
main.dart
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: 'AllAboutFlutter', theme: ThemeData( primarySwatch: Colors.blue, ), debugShowCheckedModeBanner: false, home: const TextButtonStyleFromTutorial(), ); } } class TextButtonStyleFromTutorial extends StatelessWidget { const TextButtonStyleFromTutorial({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('TextButton.styleFrom() Tutorial'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ TextButton( onPressed: () {}, style: TextButton.styleFrom( primary: Colors.green, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Click ME", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( primary: Colors.orange, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Click ME", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( primary: Colors.blue, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Click ME", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( primary: Colors.purple, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Click ME", ), ), ], ), ), ); } }
Output
Example: In the following example, we have buttons of different elevations.
main.dart
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: 'AllAboutFlutter', theme: ThemeData( primarySwatch: Colors.blue, ), debugShowCheckedModeBanner: false, home: const TextButtonStyleFromTutorial(), ); } } class TextButtonStyleFromTutorial extends StatelessWidget { const TextButtonStyleFromTutorial({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('TextButton.styleFrom() Tutorial'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ TextButton( onPressed: () {}, style: TextButton.styleFrom( elevation: 0.0, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Elevation 0.0", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( elevation: 5.0, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Elevation 5.0", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( elevation: 10.0, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Elevation 10.0", ), ), TextButton( onPressed: () {}, style: TextButton.styleFrom( elevation: 20.0, textStyle: const TextStyle(fontSize: 32.0), ), child: const Text( "Elevation 20", ), ), ], ), ), ); } }
Output
Reference: https://api.flutter.dev/flutter/material/TextButton/styleFrom.html
Subscribe to my newsletter
Read articles from Manav Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
