Understanding Child Process Spawn Function in NodeJs: Exploring Options and Examples
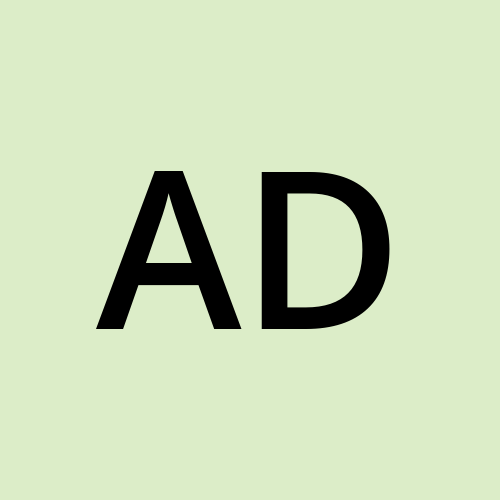
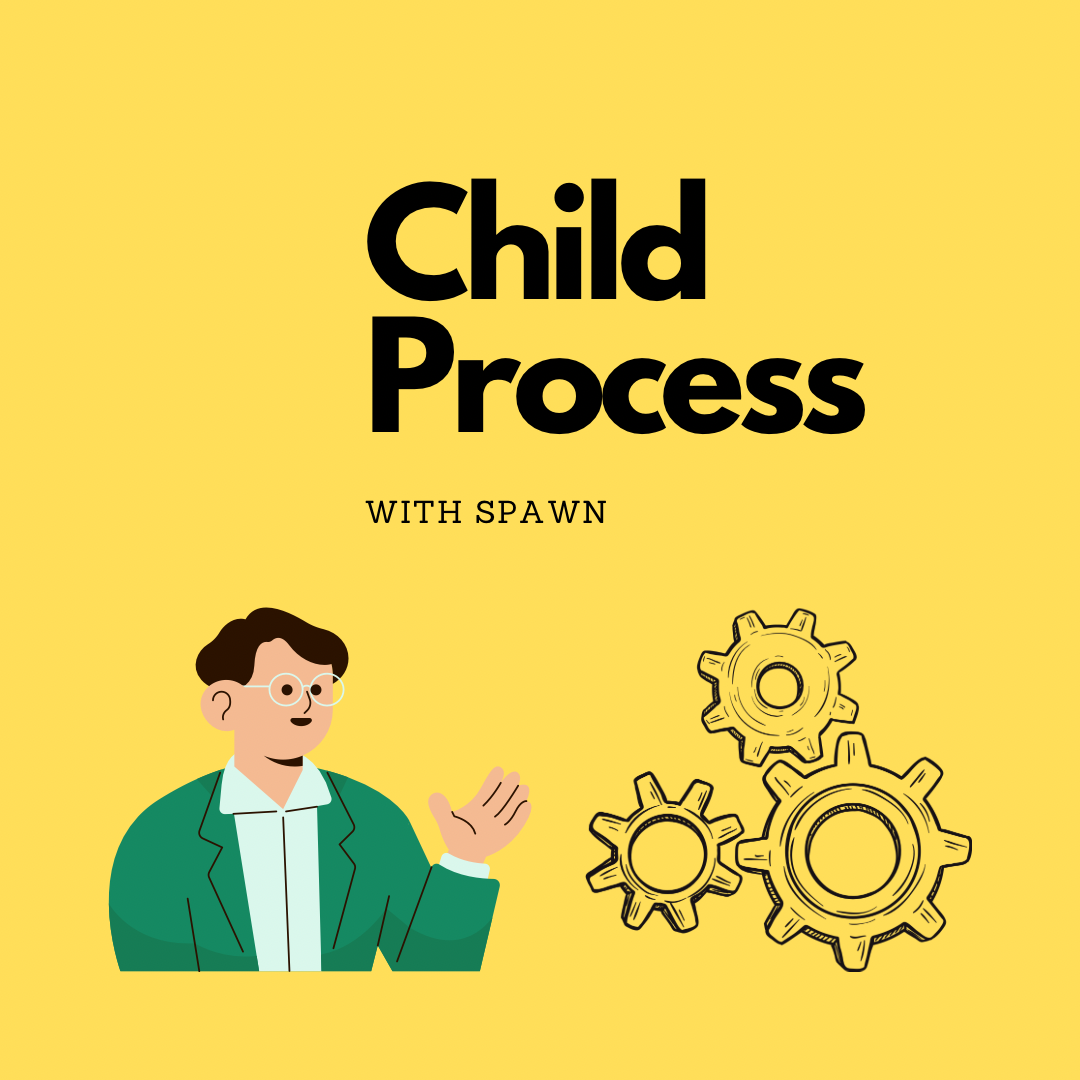
Introduction:
When working as a Node.js developer, it is essential to comprehend the child process module. This allows you to create new processes and execute commands within your Node.js applications, which in turn enables seamless interaction with the underlying operating system.
What is the Child Process Spawn Function?
The spawn
function belongs to Node.js's child process module, serving as a powerful tool to execute external commands in separate processes. It launches a new process with the specified command, allowing communication between the parent and child processes via streams. By default, there is no communication channel between a child process and its parent process.
const cp = require('child_process');
const child = cp.spawn(cmd, [args], {options});
Options in Child Process Spawn:
- Command (required): Specifies the command to execute.
const ls = cp.spawn('ls');
- Arguments (optional): Represents an array of arguments passed to the command.
const grep = cp.spawn('grep', ['hello']);
- Options (optional): Customizes the behavior of the spawned process. Example with
cwd
(current working directory):
const child = cp.spawn('pwd', [], {cwd: '/usr'});
- stdio (optional): Specifies the standard input/output of a child process, either as an option for stdio, an array of streams, or using ‘pipe’, ‘ignore’, or ‘inherit’ values.
// pipe: Connects the child's I/O to the parent's I/O streams.
const child = spawn('ls', ['-a'], { stdio: 'pipe' });
// ignore: Discards the child's I/O streams.
const child = spawn('ls', ['-a'], { stdio: 'ignore' });
// inherit: Shares the parent's I/O streams with the child.
const child = spawn('ls', ['-a'], { stdio: 'inherit' });
// Array of Streams: Customize individual I/O streams for the child process.
const fs = require('fs');
const out = fs.openSync('./out.log', 'a');
const err = fs.openSync('./err.log', 'a');
const child = spawn('ls', ['-a'], { stdio: [ 'ignore', out, err ] });
- env (optional): Specifies environment key-value pairs.
const child = spawn('echo $ANSWER', { env: { ANSWER: 42 } });
- Shell (optional): Executes the command in a shell environment.
const child = spawn('ls', ['-lh', '/usr'], { shell: true });
- Detached (optional): Allows the child process to run independently of the parent.
const child = spawn('node', ['script.js'], { detached: true })
Creating a communication channel between Parent and Child Process:
// ./parent-process.js
const {spawn} = require('child_process');
const child = spawn('node', ['child-process.js'], {
stdio: ['ignore', 'inherit', 'ignore', 'ipc']
});
child.on('message', (data) => {
console.log('Message recieved from child to parent --->', data);
});
// ./child-process.js
console.log('Hello from child process');
process.send('Message from child process');
Conclusion:
The spawn
function in Node.js child processes offers incredible flexibility to interact with system commands, facilitating robust application development. Understanding and utilizing its options empowers developers to create efficient, responsive, and scalable Node.js applications.
By harnessing the power of spawn
, Node.js developers can seamlessly integrate external commands, ensuring enhanced application functionality and versatility.
Subscribe to my newsletter
Read articles from Ashutosh Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
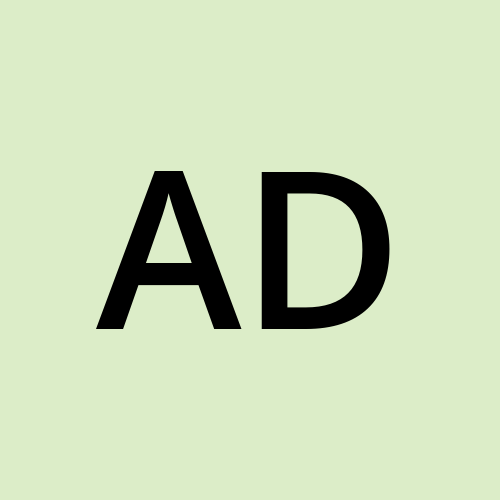