Operators In JavaScript: Comparison and Logical Operators In JavaScript
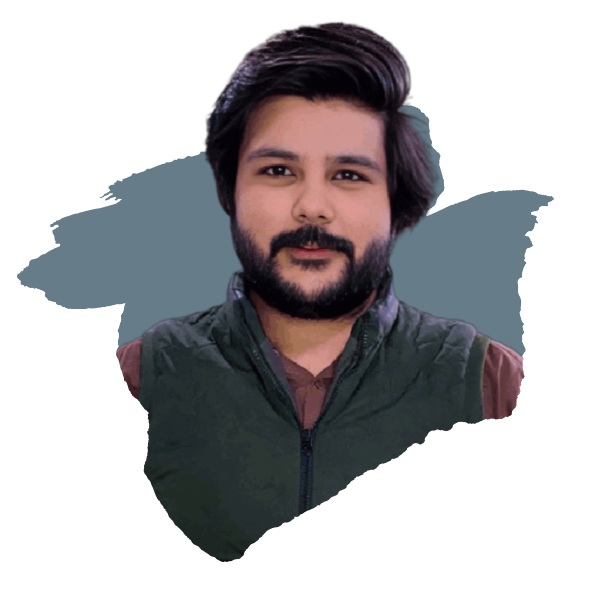
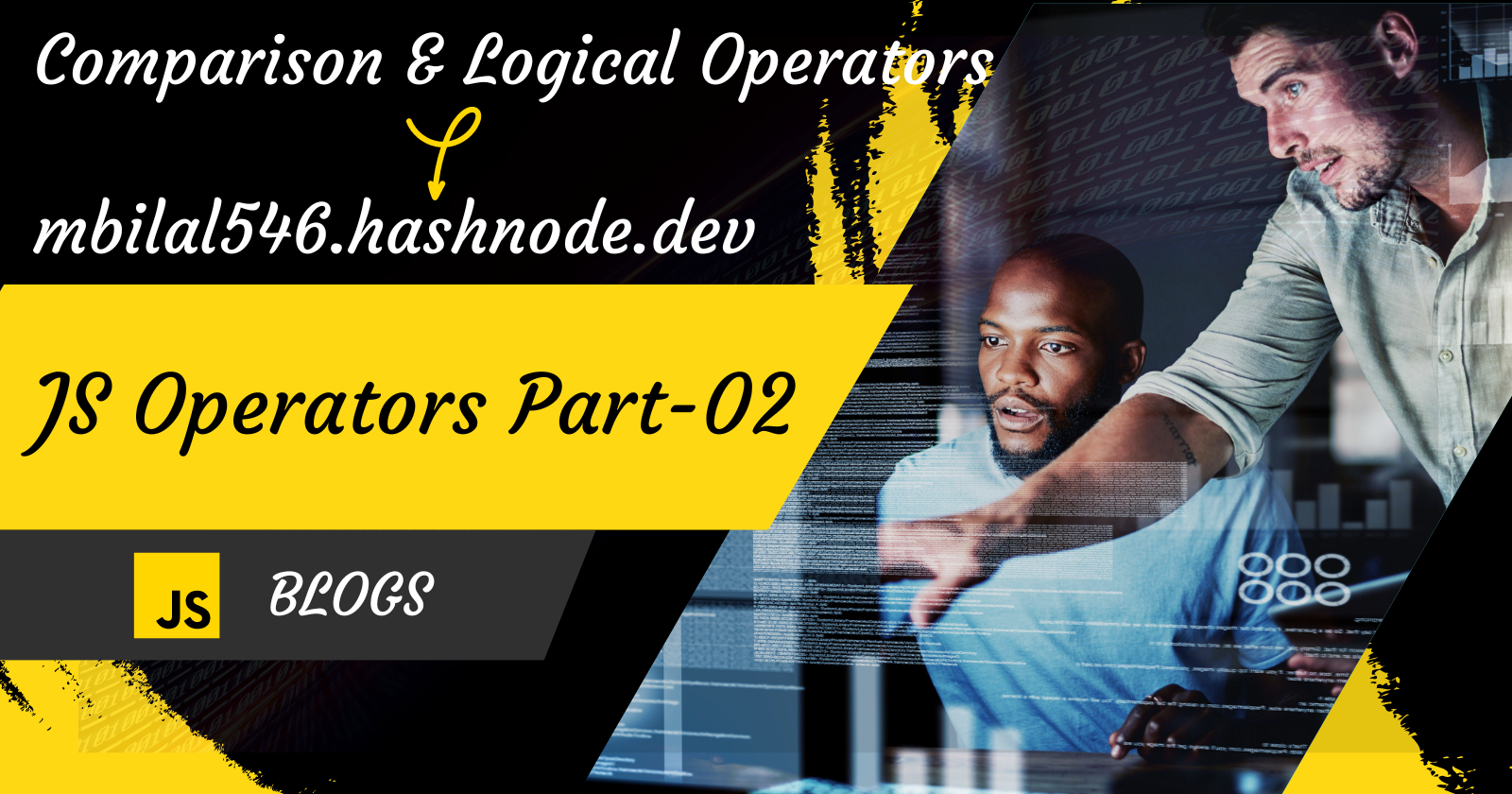
Comparison Operators in JavaScript
JavaScript provides several comparison operators to compare values and evaluate logical expressions. These operators return either true or false based on the comparison result. Here's a breakdown of the most common ones:
Equality (==):
It returns true if the values are equal, even if they are of different types.
For example:
let a = 2;
let b = "2";
if (a == b) {
console.log(true);
}else{
console.log(false);
}
// Output: true
// Because == operator doesn't check the type strictly
Strict Equality (===):
It returns true only if the values are equal and the datatypes are also equal.
For example:
let a = 2;
let b = "2";
if (a === b) {
console.log(true);
}else{
console.log(false);
}
// Output: false
// Because === operator check the type strictly.
Inequality Operator (!=):
It returns true if the values are not equal, regardless of type.
For example:
let a = 3;
let b = "2";
if (a != b) {
console.log(true);
}else{
console.log(false);
}
// Output: true
Strict inequality (!==):
It returns true only if the values are not equal or of different types.
For example:
let a = 3;
let b = "2";
if (a !== b) {
console.log(true);
}else{
console.log(false);
}
// Output: true
Comparison:
Less than (<):
It returns true if the left operand is less than the right operand.
For example:
let a = 2;
let b = 3;
if (a < b) {
console.log(true);
}else{
console.log(false);
}
// Output: true // Because 2 isless than 3
Less than or equal to (<=):
It returns true if the left operand is less than or equal to the right operand.
For example:
let a = 3;
let b = 3;
if (a <= b) {
console.log(true);
}else{
console.log(false);
}
// Output: true // Because they are equal
Greater than (>):
It returns true if the left operand is greater than the right operand.
For example:
let a = 4;
let b = 3;
if (a > b) {
console.log(true);
}else{
console.log(false);
}
// Output: true
Greater than or equal to (>=):
It returns true if the left operand is greater than or equal to the right operand.
For example:
let a = 3;
let b = 2;
if (a >= b) {
console.log(true);
}else{
console.log(false);
}
// Output: true
Note ๐:
When comparing strings, JavaScript converts them to numbers if possible. For example, '10' > 5 is true.
Comparison operators can be chained. For example, 1 < 2 < 3 is true.
The comparison operators are used in conditional statements like if and while to control program flow.
Logical Operators in JavaScript
JavaScript also offers a set of logical operators to combine multiple conditions and create more complex logical expressions. These operators help build conditional statements, control program flow and handle different situations based on various factors. Let's explore the main ones:
AND Operator (&&):
This operator returns true
only if both operands are true
. Otherwise, it returns false
.
Example:
let raining = true; // Operand 1
let cold = false; // Operand 2
if (raining && cold) {
console.log("Stay home, it's both rainy and cold!");
} else {
console.log("Both Conditions are not true!");
}
// Output: Both Conditions are not true!
OR Operator (||):
This operator returnstrue
if at least one operand is true
. It only returnsfalse
if both operands are false
.
Examples:
If one is true:
let money = false; // Operand 1
let card = true; // Operand 2
if (money || card) {
console.log("One is true so, You can buy that coffee!");
} else {
console.log("Better luck next time...");
}
// Output: One is true so, You can buy that coffee!
If both are true:
let money1 = true; // Operand 1
let card1 = true; // Operand 2
if (money || card) {
console.log("Both are true so, You can buy that coffee!");
} else {
console.log("Better luck next time...");
}
// Output: Both are true so, You can buy that coffee!
If both are false:
let hasMoney = false; // Operand 1
let hasCard = false; // Operand 2
if (hasMoney || hasCard) {
console.log("You can buy that coffee!");
} else {
console.log("Both Operands are false so, Better luck next time...");
}
// Output: Both Operands are false so, Better luck next time...
NOT (!):
This operator flips the logical value of its operand. true
becomesfalse
, and false
becomes true
.
Examples:
let isLoggedIn = false;
if (!isLoggedIn) {
console.log("You are LoggedIn!");
} else {
console.log("Go back!");
}
// Output: You are LoggedIn!
let isLoggedOut = true;
if (!isLoggedOut) {
console.log("You are LoggedOut!");
} else {
console.log("Go back!");
}
// Output: Go back!
Nullish Coalescing (??): Introduced in ES2020, this operator returns the first operand if it's not null
or undefined
. Otherwise, it returns the second operand.
Example:
let userName = null;
let defaultUserName = "Muhammad Bilal";
let actualUserName = userName ?? defaultUserName;
console.log(`Welcome, ${actualUserName}`);
// Output: Welcome, Muhammad Bilal
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
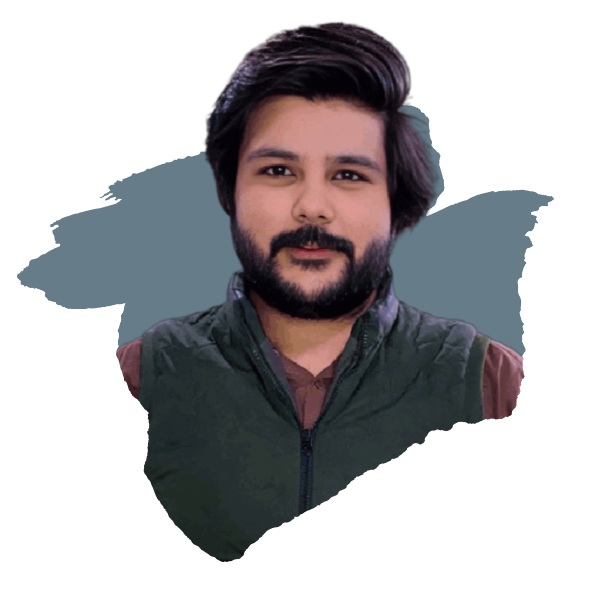
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.