Converting RGB to HEX
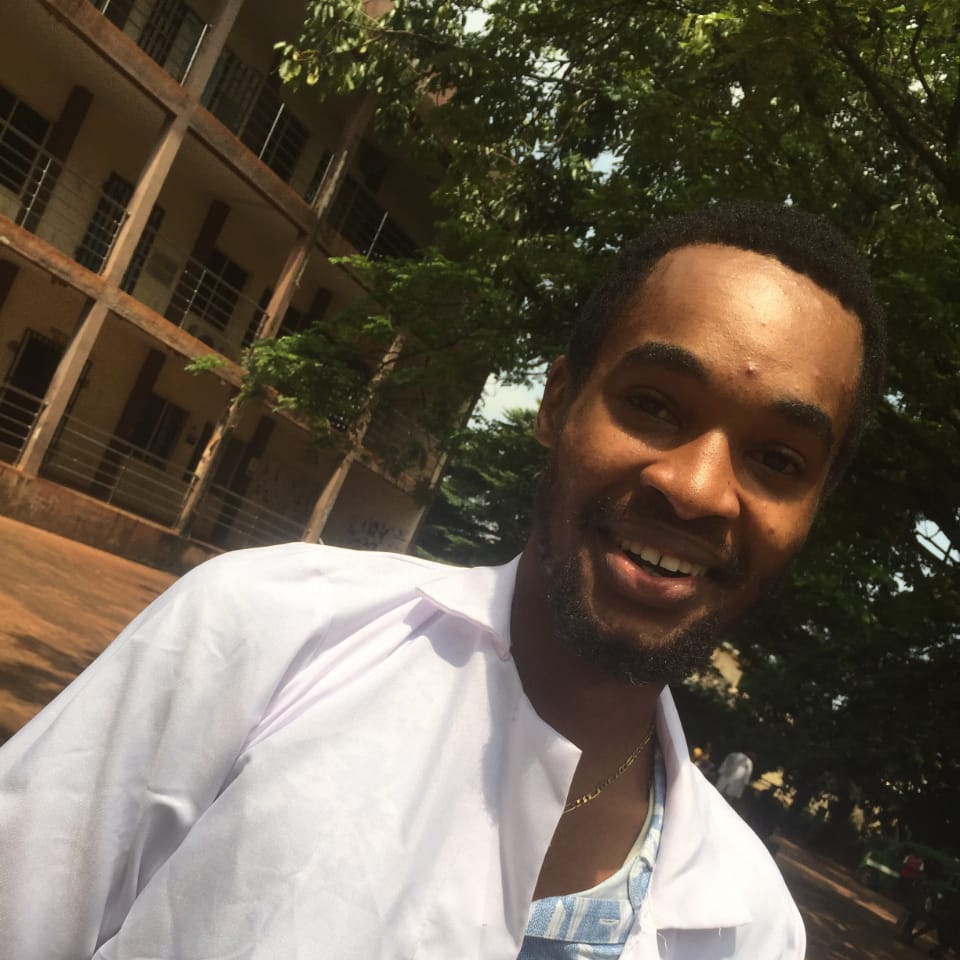
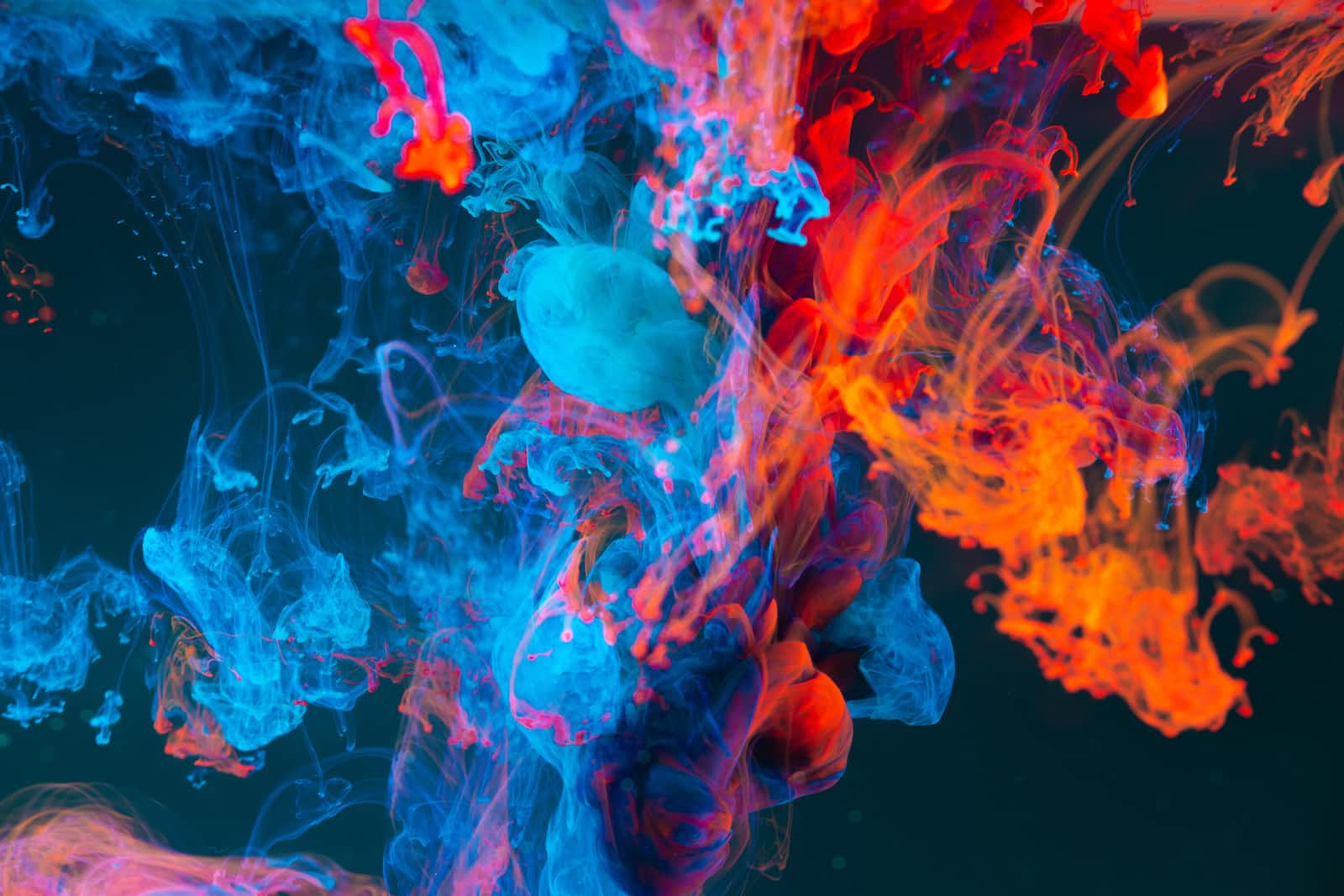
You might probably find this post irrelevant, but it's my first and I didn't know what to offer to the public, so I decided to share sth that has been an issue to me.
why ?
I just wanted to know how must of the conversation were done behind the scenes, I have been writing css and JavaScript for over 2 years now unproffesionally
, and funny enough operations like this ones seemed a little tricky.
so I will be using Typescript JavaScript
for this, which I know a good number if at all you read this post
of you are familiar with.
Examples
rgb(204, 56, 90) = #cc385a
rgb(0 247 91 / 50) = #00f75b7f
[4, 90, 200, 60] = #045ac899
60 in the array denotes the alpha channel
which ranges from 0-100 or 0-1
let's get started
export function rgbToHex(arg: string | number[]): string { }
our function rgbToHex
can accept two types a string such as "rgb(x, y, z)", "x y z"
or an array of numbers [0, 0, 0 ,0
// within our rgbToHex function
const param = typeof arg === "string" ? arg : Array.isArray(arg) ? arg : null;
next we check for the type of our arg and see if it corresponds to what we want , any thing other than a string or an array will be treated as null, the type checking is a weak one
// within our rgbToHex function
// make this object read-only.
const hexLetters = Object.freeze({ a: 10, b: 11, c: 12, d: 13, e: 14, f: 15 });
next we save some letters which are used in representing hexadecimals above 9
, the letters could be lower or uppercase css doesn't see a difference anyways
let hex: string = "#",
arr: any = param, // lol , just want to safe some time
len: number,
calc1: number | string ,
calc2: number | string;
this are the variables we are going to be manipulating and working with.
if (typeof param === "string") arr = arr?.split(/\D/).filter((num: string) => num.length !== 0).map(Number)
so what's actually going on here ?
first we check for the type , if it's a string which we've been doing right from the beginning.
we assign
arr
to a newarray
by splitting the string , by removing any character other than a numberthen we filter all the empty strings in the new array
and finally we transform those strings into actual numbers ๐ข
Now if the param
or arg
is already an array
I won't do much here just to ensure that the alpha channel isn't above 100
and proceed by changing the alpha channel
to a format which will assist in our calculations, that is keeping it internationally in the range of 0
The actual math โโ
the code above demonstrate the actual or minimalist math going on, lol it took me two hours to figure this shit out ๐ฅฒ.
using our examples
- [4, 90, 200, 60] (X, Y, Z, a)
this is how I pictured it.
RrGgBb(Aa)
when looking at the hex color #045ac899 you can start to imagine the similarities between it and RrGgBbAa. keeping in mind that numbers above 9
are represented using alphabetical letters a-f
see hexLetters constant
,
the equation is gotten as such. NB: using our example above
R = X / 16 and if there's a remainder it's used to determine for r = remainder * 16. if there is no remainder then r = 0
same method applies for Gg , Bb and so on.
R = (4 / 16) = 0 and remainder = .25 , so r = (.25 * 16) = 4, hence Rr = 04
G = (90 / 16) = 5 and remainder = .625, so g = (.625 * 16) = a , where a = 10
hence Gg = 5a
B = (200 / 16) = c, where c = 12
and remainder = .5, so b = (.5 * 16) = 8, hence Bb = c8
with this you should be able to work out for Aa.
joining everything together we end up with #045ac899.
//finally we return our result.
return hex;
here is the compiled version ( sorry not machine code)
Am open for criticism, and I will like to be guided by seniors as well, still have much to learn and much to perfect.
in case you made it down here, thanks. ๐
Subscribe to my newsletter
Read articles from Nonso Martin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
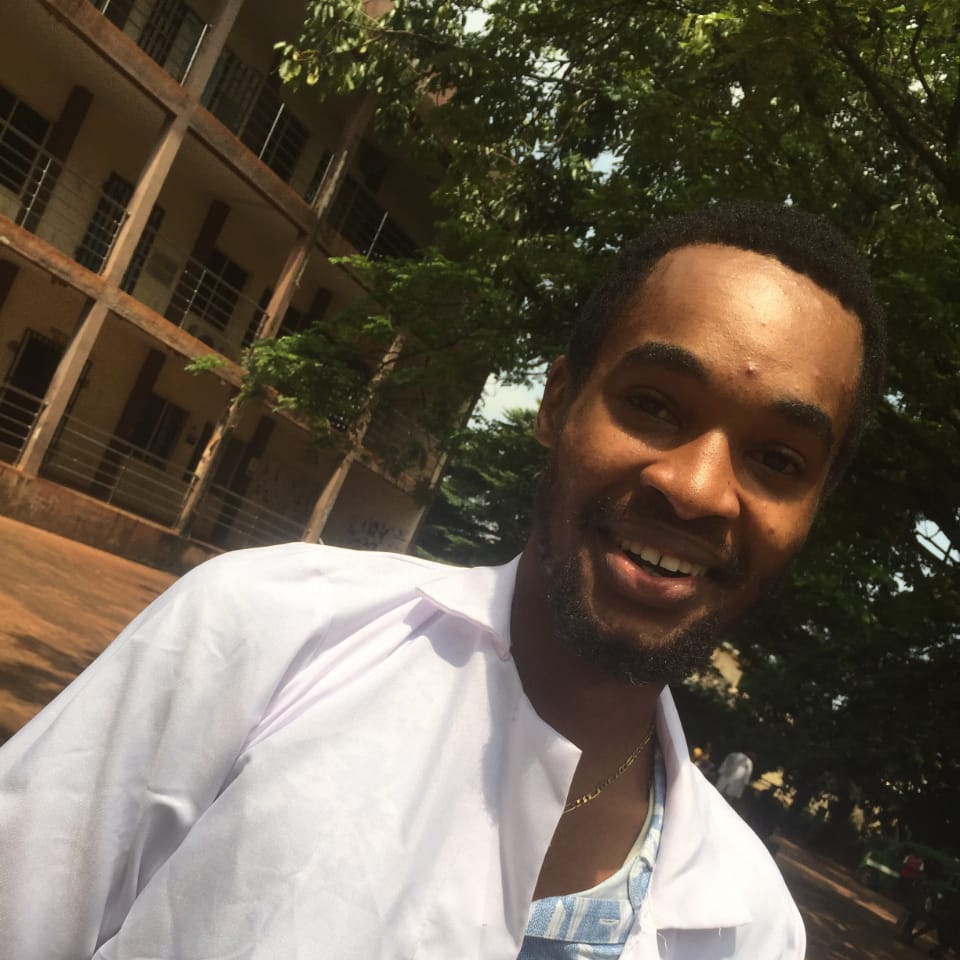
Nonso Martin
Nonso Martin
Just another random dev