Filter, Map, and Reduce in JavaScript

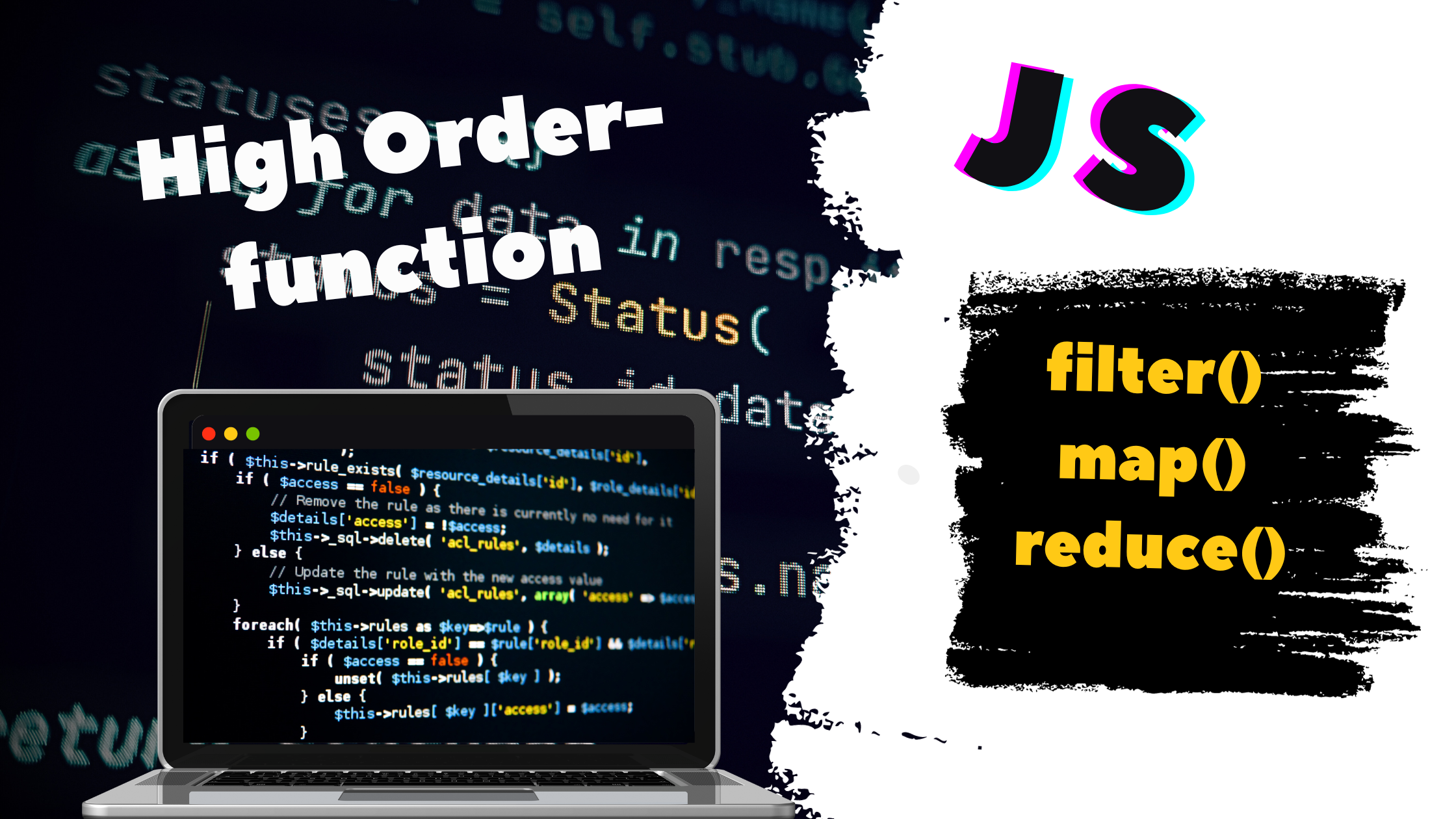
Introduction
A function that can take another function as an argument, or that returns a function as a result, is known as a Higher-Order Function(HOF).
They are a special type of function that can be used to create more complex and powerful functions.
Mostly used HIGH ORDER FUNCTIONS
filter()
map()
reduce()
filter() method
filter()
method is used to create a new array with elements that pass a certain condition or criteria from an existing array.It does not modify the original array but instead returns a new array containing only the elements that meet the specified condition.
Syntax:
const newArray = arr.filter((
currentValue
, index, array) => {// condition
});
callback
: Function is a predicate, to test each element of the array. Returntrue
to keep the element,false
otherwise. It accepts three arguments:currentValue
: The current element being processed in the array.index
(optional): The index of the current element being processed.array
(optional): The arrayfilter
was called upon.
Example -
// 1. filter() method
// What is filter()?
// Filter method takes each element in an array and it applies an conditional
/// statement against it, if condition returns true element gets push into
// the array and if the condition return false the element does not push into
// the output array.
// => filter() accepts 3 arguments same as forEach().
// => filter() return value, but forEach does not return any value.
// Syntax:
// filter((currentVal, index, arr) => {
// condition
// });
// Example 1 : filter even number
const arr1 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const res = arr1.filter((num) => num % 2 == 0); // Implicit scope (not use of {} and return keyword)
// console.log(res); // output: [ 2, 4, 6, 8, 10 ]
// There are 2 different way to use arrow function
// Implicit Function (No use of return keyword)
// Explicit Function (Use of return keyword)
//Note: Checkout my Function in JavaScript blog
// <=====####=====>
// Compare filter() with forEach()
const ans = [];
arr1.forEach((num) => {
if (num % 2 === 0) {
ans.push(num);
}
});
// console.log(ans);
// <=====####=====>
// Example 2 : return more than 2 elements only
const arr2 = [1, 2, 3, 4, 5, 6];
const moreThanTwo = arr2.filter((arrayElement, index, actualArray) => {
// no need to write index and actualArray
return arrayElement > 2; // Explicit scope (using {} with return keyword)
});
// console.log(moreThanTwo);
// Example 3 :
let students = [
{ name: "Piyush", rollNumber: 31, marks: 80 },
{ name: "Jenny", rollNumber: 15, marks: 69 },
{ name: "Kaushal", rollNumber: 16, marks: 35 },
{ name: "Dilpreet", rollNumber: 7, marks: 55 },
];
// using filter
// Question 2 : Return only details of those students who scored more than 60
let marksMoreThanSixty = students.filter((stuMarks) => stuMarks.marks > 60);
// console.log(marksMoreThanSixty);
// Question 2 : Return only details of those students who scored more than
// and roll no > 15
const details = students.filter(
(stuMarks) => stuMarks.marks > 60 && stuMarks.rollNumber > 15
);
// console.log(details);
// <=====####=====>
(IMPORTANT) Example
// #IMPORTANT: Example => filter out array of object according to some
// conditions
const data = [
{
title: "Mythology of the Vedas: Gods of the Rigveda",
genre: "Mythology",
publishDate: "2019",
editionDate: "2020",
},
{
title: "The Mahabharata: Gods, Heroes, and Epic Battles",
genre: "Epic Poetry",
publishDate: "2021",
editionDate: "2021",
},
{
title: "Gods and Goddesses of Ancient India: Stories from the Puranas",
genre: "Mythology",
publishDate: "2018",
editionDate: "2019",
},
{
title: "Shiva: The Eternal God of Destruction",
genre: "Biography",
publishDate: "2017",
editionDate: "2018",
},
{
title: "Ganesha: The Remover of Obstacles",
genre: "Mythology",
publishDate: "2020",
editionDate: "2021",
},
{
title: "Devi: The Divine Feminine in Hindu Mythology",
genre: "Mythology",
publishDate: "2019",
editionDate: "2019",
},
{
title: "Vishnu: The Preserver of the Universe",
genre: "Biography",
publishDate: "2016",
editionDate: "2017",
},
{
title: "Hanuman: The Devotee Warrior",
genre: "Mythology",
publishDate: "2018",
editionDate: "2018",
},
];
let result = data.filter((book) => book.genre === "Mythology"); //Implicit Scope
// console.log(result);
result = data.filter((book) => {
return book.publishDate > 2015 && book.genre === "Mythology"; //Explicit Scope
})
// console.log(result);
result = data.filter((book) => {
return book.publishDate > 2020; //Explicit Scope
});
console.log(result);
// <=====####=====>
map() method
map()
method is used to create a new array by applying a given function to each element of an existing array.It doesn't modify the original array but instead returns a new array where each element is the result of applying the provided function to the corresponding element of the original array.
Syntax:
const newArray = arr.map((
currentValue
, index, array) => {// condition
})
callback
: Function is a function that is called for each element in the array. It accepts three arguments:currentValue
: The current element being processed in the array.index
(optional): The index of the current element being processed.array
(optional): The arraymap
was called upon.
Example -
//map() method
// What is map()?
// It is a method to create a new array from existing one by applying
// a function to each one of the elements of the first array.
// Syntax
// map((currentVal, index, array) => {
// condition
// })
// Example 1 : multiplying each element of the array by 5 and returning the new array
const nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const res = nums.map((arrayElements, index, actualArray) => arrayElements * 5); // Returning implicitly(without return keyword)
// console.log(res);
const addTwo = nums.map((arrayElements, index, actualArray) => {
return arrayElements + 2; // Returning explicitly (using return keyword)
});
// <=====####=====>
// Example 2 : Output only names of students in uppercase
let students = [
{ name: "Vihant", rollNo: 30, marks: 95 },
{ name: "Raj", rollNo: 25, marks: 80 },
{ name: "Piyush", rollNo: 20, marks: 85 },
{ name: "Rohan", rollNo: 40, marks: 70 },
{ name: "Aryan", rollNo: 35, marks: 75 },
];
//normal way
let names = [];
for (let i = 0; i < students.length; i++) {
names.push(students[i].name.toUpperCase());
}
// console.log(names);
// Using map
let stuNames = students.map((arrElement) => arrElement.name.toUpperCase());
console.log(stuNames);
// <=====####=====>
reduce() method
reduce()
method is used to accumulate or reduce the elements of an array into a single value.It iterates over the array and applies a function to each element, updating an accumulator along the way.
The result is a single value that is the accumulated result of applying the function to each element of the array.
Syntax:
reduce((accumulator,
currentValue
, currentIndex, array) => {// condition
}, initialValue)
callback
: A function that is called for each element in the array. It accepts four arguments:accumulator
: The accumulated result of the previous iterations.currentValue
: The current element being processed in the array.index
(optional): The index of the current element being processed.array
(optional): The arrayreduce
was called upon.
initialValue
(optional): An initial value for the accumulator. If not provided, the first element of the array is used as the initial accumulator value.
Example -
// reduce() method
// What is reduce()?
// Reduce method reduces an array of values down to just one value.
// Syntax:
// reduce((accumulator, currentVal, currentIndex, array) => {
// condition
// }, initialVal)
// Example 1 : Return the sum of array
const arr = [1, 2, 3, 4, 5, 6];
// const sum = arr.reduce((acc, currentElement, index, actualArray) => {
// // accumulator(acc) = result of the previous computation or result of array elements till the current element
// // currentElement = current element
// // index = indexing of the element
// // actualArray = Input Array
// console.log(`Acc : ${acc} | CurrentVal : ${currentElement} `);
// return acc + currentElement;
// }, 0); // 0 represent second parameter that is initial value which added with accumulator and it runs only once
const sum = arr.reduce((acc, currVal) => acc + currVal, 0);
// console.log(sum);
// Example 2 : Return TotalBillToPay in shopping cart
let cart = [
{ product: "Watch", price: 2500 },
{ product: "Headphone", price: 4000 },
{ product: "Shoes", price: 2000 },
{ product: "Shirt", price: 750 }
];
const TotalBillToPay = cart.reduce((acc, item) => acc + item.price, 0);
console.log(TotalBillToPay);
Method Chaining Example
Method chaining in JavaScript is a programming technique where multiple methods are called on an object sequentially.
This is possible when each method returns an object (typically the object on which the method was called) so that additional methods can be invoked on the result.
This pattern is often used to create more readable and concise code.
Example -
// Method Chaining
// Syntax:
// map(() => cond1).filter(() => cond2).reduce((acc, item) => cond3);
// Example 1 : Return only names of students who scored more than 60
let students = [
{ name: "Vihant", rollNo: 30, marks: 95 },
{ name: "Raj", rollNo: 25, marks: 80 },
{ name: "Piyush", rollNo: 20, marks: 55 },
{ name: "Rohan", rollNo: 40, marks: 65 },
{ name: "Aryan", rollNo: 35, marks: 45 },
];
const res = students
.filter((stu) => {
return stu.marks > 60
})
.map((stu) => {
return stu.name
});
console.log(res);
// Example 2 : Return total marks for students with marks greater than 60
// after 20 marks have been added to those who scored less than 60.
const totMarks = students
.map((stu) => {
if (stu.marks < 60) {
stu.marks += 20;
}
return stu;
})
.filter((stu) => stu.marks > 60)
.reduce((acc, curr) => acc + curr.marks, 0);
console.log(totMarks);
Subscribe to my newsletter
Read articles from Pranay Sanjule directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranay Sanjule
Pranay Sanjule
Learner, Tech-Enthusiast