FluentValidation Introduction and Setup In .NET
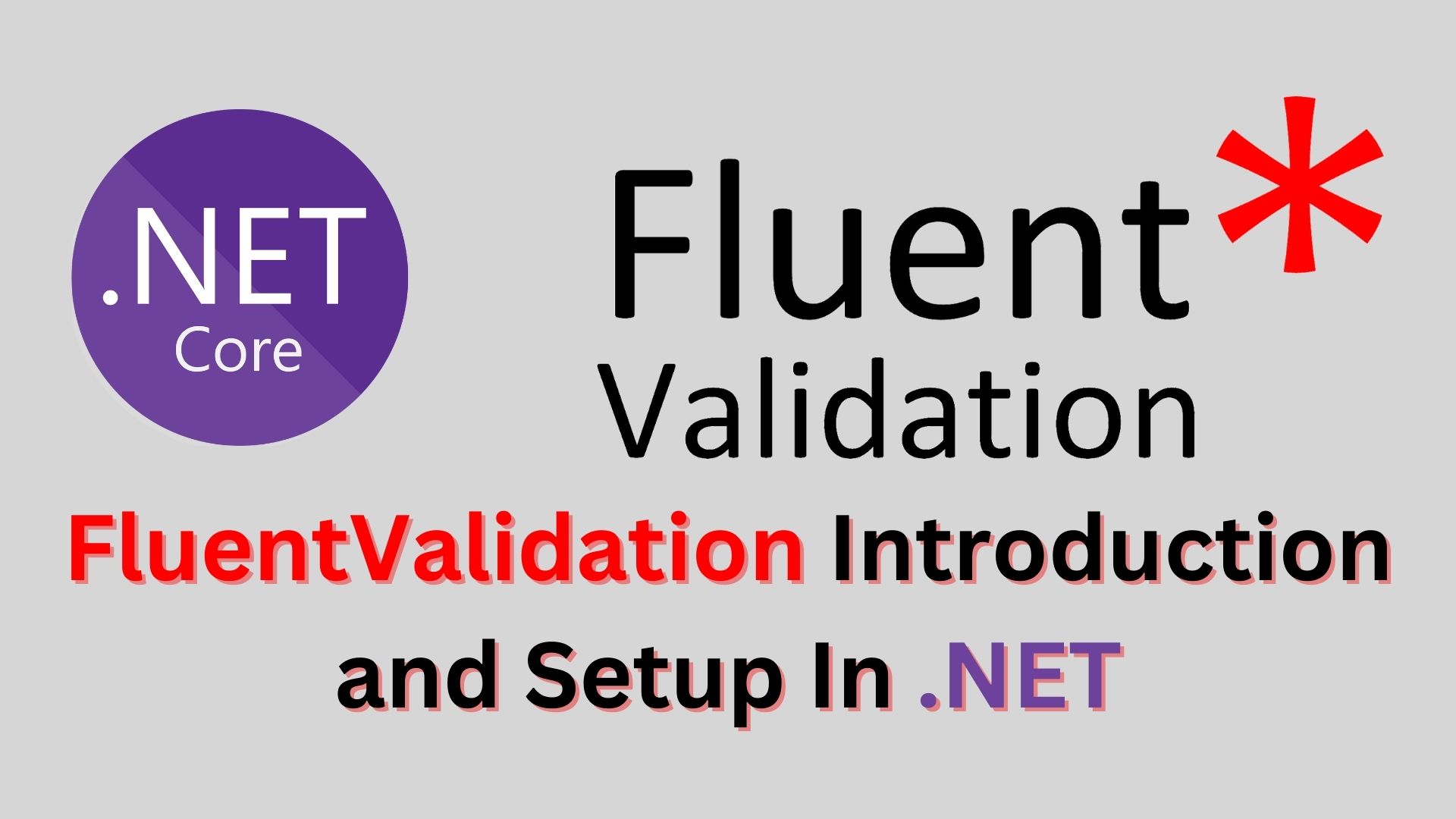
What is FluentValidation?
FluentValidation is a .NET library for building strongly-typed validation rules using a fluent interface.
It allows you to define validation rules for your domain models using a simple, readable syntax.
It can be used to validate user input, data from a database, or any other data in your application.
FluentValidation supports both synchronous and asynchronous validation and can be integrated with various frameworks and libraries such as ASP.NET Core, MVC, and Web API.
Installation
Using the NuGet package manager console within Visual Studio run the following command:
Install-Package FluentValidation
Goto NuGet package manager and Install FluentValidation Package.
Basic Syntax
using FluentValidation;
namespace Project.Validators
{
public class Validator_Name : AbstractValidator<Model_Name>
{
public Validator_Name()
{
RuleFor(x => x.Name).NotEmpty(); // Empty check
RuleFor(x => x.Length).GreaterThan(0); // GreaterThan check
}
}
}
Setup
Dependencies
FluentValidation
FluentValidation.AspNetCore
FluentValidation.DependencyInjectionExtensions
Folder Structure:
AddContactRequest.cs (Models)
namespace Contact.API.Models
{
public class AddContactRequest
{
public string Name { get; set; }
public double Phone { get; set; }
public string Email { get; set; }
public string Address { get; set; }
}
}
AddContactRequestValidator.cs (Validators)
using FluentValidation;
namespace Contact.API.Validators
{
public class AddContactRequestValidator: AbstractValidator<Models.AddContactRequest>
{
public AddContactRequestValidator()
{
RuleFor(x => x.Name).NotEmpty(); // Not Empty check
RuleFor(x => x.Length).GreaterThan(0); // GreaterThan check
}
}
}
Program.cs
using FluentValidation.AspNetCore;
... // other contents
builder.Services.
AddFluentValidation(options => options.RegisterValidatorsFromAssemblyContaining<Program>());
... // other contents
var app = builder.Build();
... // other contents
Built-in Validators
- Not Null Validator
Ensures that the specified property is not null.
RuleFor(customer => customer.Surname).NotNull();
- Not Empty Validator
Ensures that the specified property is not null, an empty string, or whitespace (or the default value for value types, e.g., 0 for int). When used on an IEnumerable (such as arrays, collections, lists, etc.), the validator ensures that the IEnumerable is not empty.
RuleFor(customer => customer.Surname).NotEmpty();
- Not Equal
Ensures that the value of the specified property is not equal to a particular value (or not equal to the value of another property).
//Not equal to a particular value
RuleFor(customer => customer.Surname).NotEqual("Foo");
//Not equal to another property
RuleFor(customer => customer.Surname).NotEqual(customer => customer.Forename);
Equal Validator
Ensures that the value of the specified property is equal to a particular value (or equal to the value of another property).
//Equal to a particular value
RuleFor(customer => customer.Surname).Equal("Foo");
//Equal to another property
RuleFor(customer => customer.Password).Equal(customer => customer.PasswordConfirmation);
Length Validator
Ensures that the length of a particular string property is within the specified range. However, it doesn’t ensure that the string property isn’t null.
RuleFor(customer => customer.Surname).Length(1, 250); //must be between 1 and 250 chars (inclusive)
Max Length Validator
Ensures that the length of a particular string property is no longer than the specified value.
RuleFor(customer => customer.Surname).MaximumLength(250); //must be 250 chars or fewer
Min Length Validator
Ensures that the length of a particular string property is longer than the specified value.
RuleFor(customer => customer.Surname).MinimumLength(10); //must be 10 chars or more
Less Than Validator
Ensures that the value of the specified property is less than a particular value (or less than the value of another property) Example:
//Less than a particular value
RuleFor(customer => customer.CreditLimit).LessThan(100);
//Less than another property
RuleFor(customer => customer.CreditLimit).LessThan(customer => customer.MaxCreditLimit);
Less Than Or Equal Validator
Ensures that the value of the specified property is less than or equal to a particular value (or less than or equal to the value of another property) Example:
//Less than a particular value
RuleFor(customer => customer.CreditLimit).LessThanOrEqualTo(100);
//Less than another property
RuleFor(customer => customer.CreditLimit).LessThanOrEqualTo(customer => customer.MaxCreditLimit);
Greater Than Validator
Ensures that the value of the specified property is greater than a particular value (or greater than the value of another property) Example:
//Greater than a particular value
RuleFor(customer => customer.CreditLimit).GreaterThan(0);
//Greater than another property
RuleFor(customer => customer.CreditLimit).GreaterThan(customer => customer.MinimumCreditLimit);
Greater Than Or Equal Validator
Ensures that the value of the specified property is greater than or equal to a particular value (or greater than or equal to the value of another property) Example:
//Greater than a particular value
RuleFor(customer => customer.CreditLimit).GreaterThanOrEqualTo(1);
//Greater than another property
RuleFor(customer => customer.CreditLimit).GreaterThanOrEqualTo(customer => customer.MinimumCreditLimit);
Predicate Validator
Passes the value of the specified property into a delegate that can perform custom validation logic on the value.
RuleFor(customer => customer.Surname).Must(surname => surname == "Foo");
Note that there is an additional overload for Must that also accepts an instance of the parent object being validated. This can be useful if you want to compare the current property with another property from inside the predicate:
RuleFor(customer => customer.Surname).Must((customer, surname) => surname != customer.Forename)
Regular Expression Validator
Ensures that the value of the specified property matches the given regular expression.
RuleFor(customer => customer.Surname).Matches("some regex here");
Email Validator
Ensures that the value of the specified property is a valid email address format.
RuleFor(customer => customer.Email).EmailAddress();
Credit Card Validator
Checks whether a string property could be a valid credit card number.
RuleFor(x => x.CreditCard).CreditCard();
Enum Validator
Checks whether a numeric value is valid to be in that enum. This is used to prevent numeric values from being cast to an enum type when the resulting value would be invalid. For example, the following is possible:
public enum ErrorLevel
{
Error = 1,
Warning = 2,
Notice = 3
}
public class Model
{
public ErrorLevel ErrorLevel { get; set; }
}
var model = new Model();
model.ErrorLevel = (ErrorLevel)4;
The compiler will allow this, but a value of 4 is technically not valid for this enum. The Enum validator can prevent this from happening.
RuleFor(x => x.ErrorLevel).IsInEnum();
Enum Name Validator
Checks whether a string is a valid enum name.
// For a case sensitive comparison
RuleFor(x => x.ErrorLevelName).IsEnumName(typeof(ErrorLevel));
// For a case-insensitive comparison
RuleFor(x => x.ErrorLevelName).IsEnumName(typeof(ErrorLevel), caseSensitive: false);
Empty Validator
Opposite of the NotEmpty validator. Checks if a property value is null, or is the default value for the type. When used on an IEnumerable (such as arrays, collections, lists, etc.), the validator ensures that the IEnumerable is empty.
RuleFor(x => x.Surname).Empty();
Null Validator
Opposite of the NotNull validator. Checks if a property value is null.
RuleFor(x => x.Surname).Null();
Exclusive Between Validator
Checks whether the property value is in a range between the two specified numbers (exclusive).
RuleFor(x => x.Id).ExclusiveBetween(1,10);
Inclusive Between Validator
Checks whether the property value is in a range between the two specified numbers (inclusive).
RuleFor(x => x.Id).InclusiveBetween(1,10);
Precision Scale Validator
Checks whether a decimal value has the specified precision and scale.
RuleFor(x => x.Amount).PrecisionScale(4, 2, false);
Happy Coding ✨ Fellows 🙂
If you enjoy my content, please consider buying me a coffee to support my work: https://www.buymeacoffee.com/atdilakshan
Subscribe to my newsletter
Read articles from Dilakshan Antony Thevathas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by