My map function
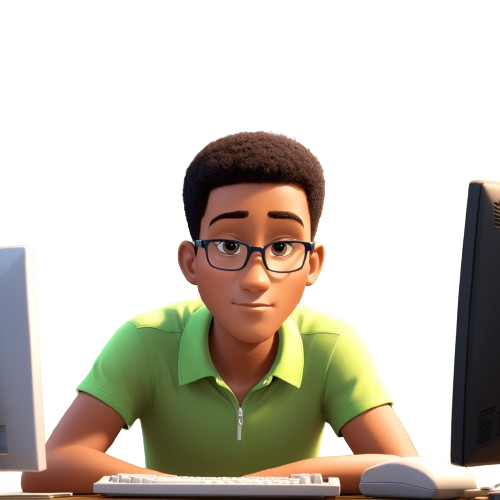
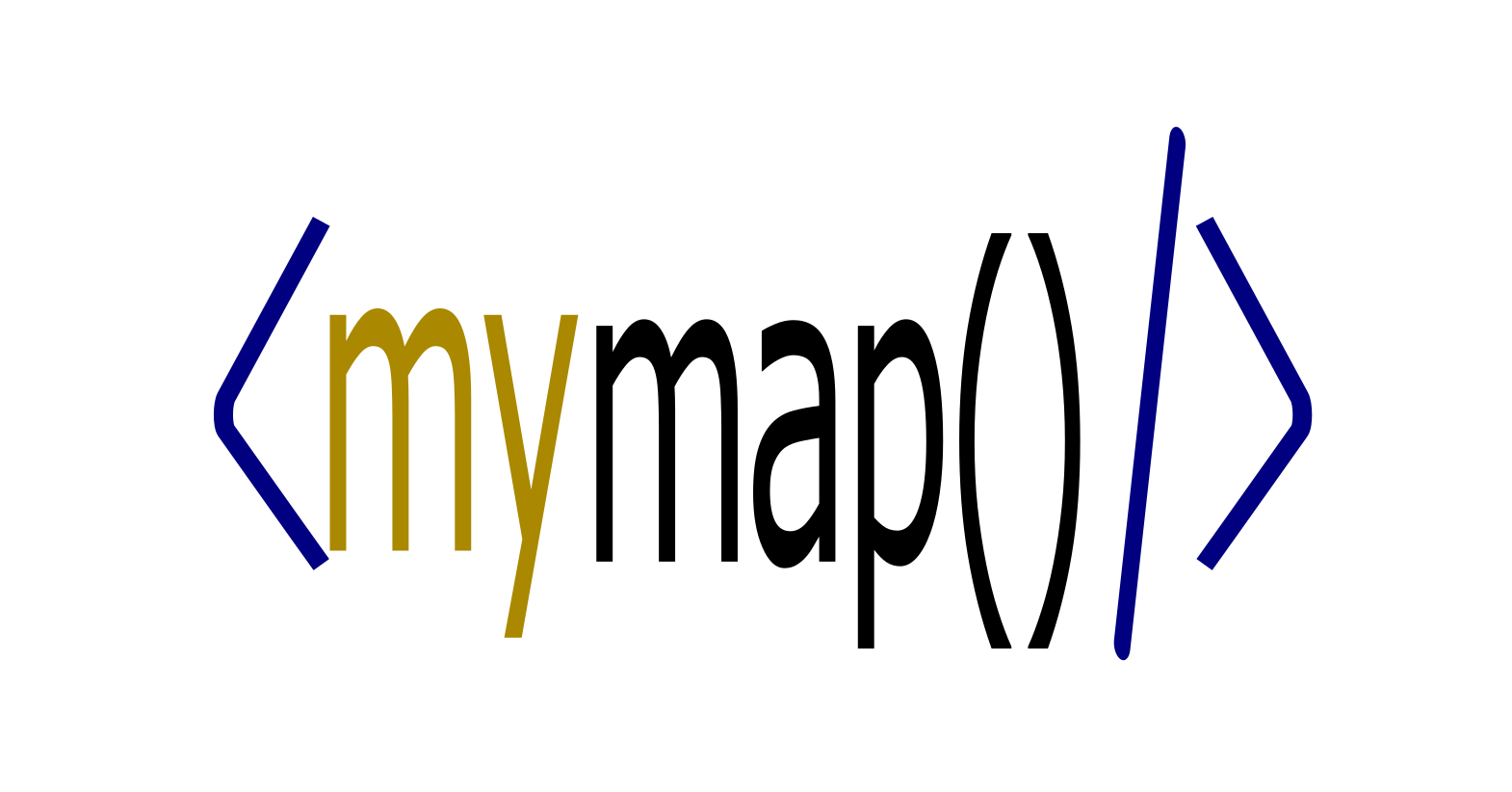
Now, let's create our version of the map method.
As mentioned in the introduction, to avoid the complexity of classes, we will use functions that take the array as a parameter to perform operations on it. So, the first param of our map function will be... the array itself and the second parameter will be the parameter of the map method(the callback):
function myMap(array, callback) {
}
Now, let's reflect on the functioning of our function.
As we see in the last chapter, the map method creates a new array and returns it. So let's do that.
function myMap(array, callback) {
const newArray = [];
/*
other code
...
*/
return newArray;
}
The new array is filled with the output of the callback for each element of the array. We could think like this then:
function myMap(array, callback) {
const newArray = [];
for (let item of array) {
//We obtain the output of the callback for the current item
const newItem = callback(item);
//Then, we fill newArray with newItem
newArray.push(newItem);
}
//These instruction will be perform for each item of 'array'
return newArray;
}
This code will work but it's not complete. Indeed, the callback can take three parameters but we send only one. The remaining two, are the index of the current item and the array.
For the array, it is not a problem because we already know it: it's the parameter array
. But for the index, we need to be cunning a little. According to you, how might we find out the index of the current element?
We have two options, we can create a variable index
that will represent the index and that we will increment on each iteration.
function myMap(array, callback) {
const newArray = [];
let index = 0;
for (let item of array) {
// We obtain the output of the callback for the current item
const newItem = callback(item, index, array);
// Then, we populate newArray with newItem
newArray.push(newItem);
// Let's increment the index for the next item
index++; // i.e., index = index + 1
}
// These instructions will be performed for each item in 'array'
return newArray;
}
Or we can simply use the loop for
like this:
function myMap(array, callback) {
const newArray = [];
for (let index = 0; index < array.length; index++) {
// We obtain the output of the callback for the current item
const newItem = callback(array[index], index, array);
// Then, we populate newArray with newItem
newArray.push(newItem);
}
// These instructions will be performed for each item in 'array'
return newArray;
}
Here, the loop for
will auto-increment the index for us and will take care of managing the index variable. Either way, both approaches achieve the same result.
With this implementation, our myMap
function replicates the basic functionality of the map
method in JavaScript. You can use it to create a new array by applying a provided function to each element of an existing array.
Feel free to test your myMap
function with different arrays and callbacks to explore its capabilities further. By understanding the inner workings of fundamental methods like map
, you gain a deeper insight into the foundations of JavaScript programming.
Let's see you in the next chapter to learn about the method filter.
Happy coding! ๐๐
Subscribe to my newsletter
Read articles from Rayane TOKO directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
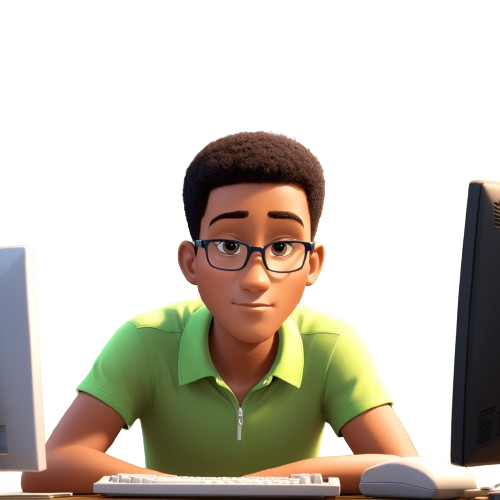
Rayane TOKO
Rayane TOKO
๐จโ๐ป Computer Scientist | ๐ Physicist Enthusiast ๐ก Always exploring new technologies and seeking to bridge the gap between science and technology.